Creating Custom Enable Annotations in Spring Made Easy
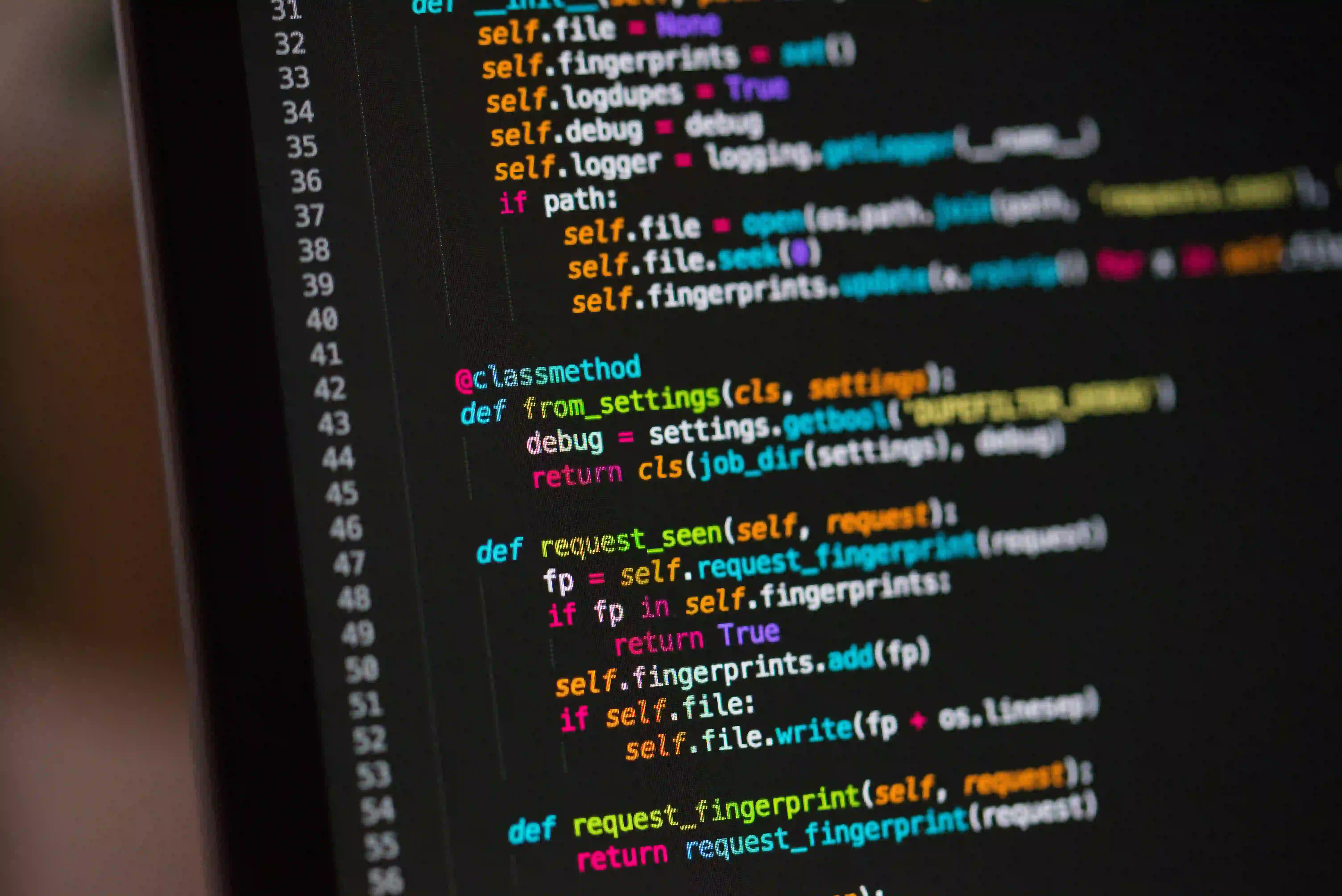
Creating Custom Enable Annotations in Spring Made Easy
Spring Framework has become a staple in modern Java development. One of its powerful features is the ability to create custom annotations, particularly @Enable
annotations that help configure beans and settings in a declarative manner. This post completes a deep dive into creating and using custom enable annotations in Spring, providing you with helpful code snippets along the way.
Understanding Enable Annotations
Enable annotations act as a flag to tell Spring to look for certain configurations or features annotated with them. The most common example is @EnableAutoConfiguration
, which enables the auto-configuration feature in Spring Boot. The ability to create your own enable annotation allows you to customize your application further and encapsulate specific behavior.
Why Use Custom Enable Annotations?
Custom enable annotations encapsulate configurations, making your code cleaner and more maintainable. Utilizing these annotations brings the following benefits:
- Encapsulation: Hide the complexity of conditional bean creation behind a simple annotation.
- Reusability: Create generic solutions that can be reused across projects.
- Readability: Improve your code's readability by clearly defining what features are enabled.
Step-by-Step Guide to Creating Custom Enable Annotations
Let’s walk through the creation of a custom enable annotation step by step.
Step 1: Create the Custom Annotation
Start by creating the annotation that will serve as our enable marker. For this example, we will create a simple custom annotation called @EnableFeatureX
.
import org.springframework.context.annotation.Import;
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
@Target(ElementType.TYPE)
@Retention(RetentionPolicy.RUNTIME)
@Import(FeatureXConfiguration.class)
public @interface EnableFeatureX {
}
Explanation:
@Target(ElementType.TYPE)
: Specifies that this annotation can only be applied to classes.@Retention(RetentionPolicy.RUNTIME)
: Indicates that the annotation will be available at runtime.@Import(FeatureXConfiguration.class)
: Imports the configuration class that will handle the bean definitions and settings.
Step 2: Create the Configuration Class
Next, we need a configuration class that will be responsible for managing the beans related to FeatureX
.
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class FeatureXConfiguration {
@Bean
public FeatureXService featureXService() {
return new FeatureXService();
}
}
Explanation:
- The
@Configuration
annotation indicates that this class has methods that define Spring beans. - The method
featureXService
creates and returns a new instance ofFeatureXService
, which we will define next.
Step 3: Create the Service Class
Now, let's define the FeatureXService
class that provides some functionality.
public class FeatureXService {
public void execute() {
System.out.println("Feature X is now active!");
}
}
Explanation: The execute
method simulates some functionality of Feature X.
Step 4: Use the Custom Enable Annotation
Now that we have our custom annotation and related components, let’s see how to apply it in a Spring application.
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
@EnableFeatureX
public class MySpringApplication {
public static void main(String[] args) {
var context = SpringApplication.run(MySpringApplication.class, args);
FeatureXService service = context.getBean(FeatureXService.class);
service.execute();
}
}
Explanation:
@EnableFeatureX
tells Spring to use the configuration defined inFeatureXConfiguration
.- Inside the
main
method, we retrieve theFeatureXService
bean and invoke theexecute
method. This outputs the message indicating that Feature X is active.
Additional Considerations
Conditional Annotations
If you want to add conditions for enabling certain features based on specific criteria, consider using @ConditionalOnProperty
.
For example:
import org.springframework.boot.autoconfigure.condition.ConditionalOnProperty;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class FeatureXConfiguration {
@Bean
@ConditionalOnProperty(name = "feature.x.enabled", havingValue = "true", matchIfMissing = true)
public FeatureXService featureXService() {
return new FeatureXService();
}
}
Explanation: The @ConditionalOnProperty
annotation allows the FeatureXService
bean to be created only if the property feature.x.enabled
is set to true in your configuration file (application.properties or application.yml).
Common Use Cases
Custom enable annotations can significantly simplify the configuration and management of common features such as:
- Integrating third-party libraries: Enable a library's features without boilerplate code.
- Module-based applications: Enable specific features according to the application modules being used.
For more in-depth details on Spring's conditional annotations, consider checking out the Spring Boot Reference Documentation.
The Last Word
Creating custom enable annotations in Spring can dramatically enhance your application's configurability and maintainability. This ability enables developers to encapsulate complex configurations into simple annotations, promoting better design.
In summary, your custom annotation is a powerful tool in the Spring landscape, facilitating better code management and modularity. As Spring continues to evolve, mastering these custom annotations will become increasingly essential for any Java developer.
Feel free to experiment with the concepts covered in this guide. Share your experiences and let us know how you’ve implemented custom enable annotations in your projects! Happy coding!