How to Fix Hibernate's Dirty Checking Performance Issues
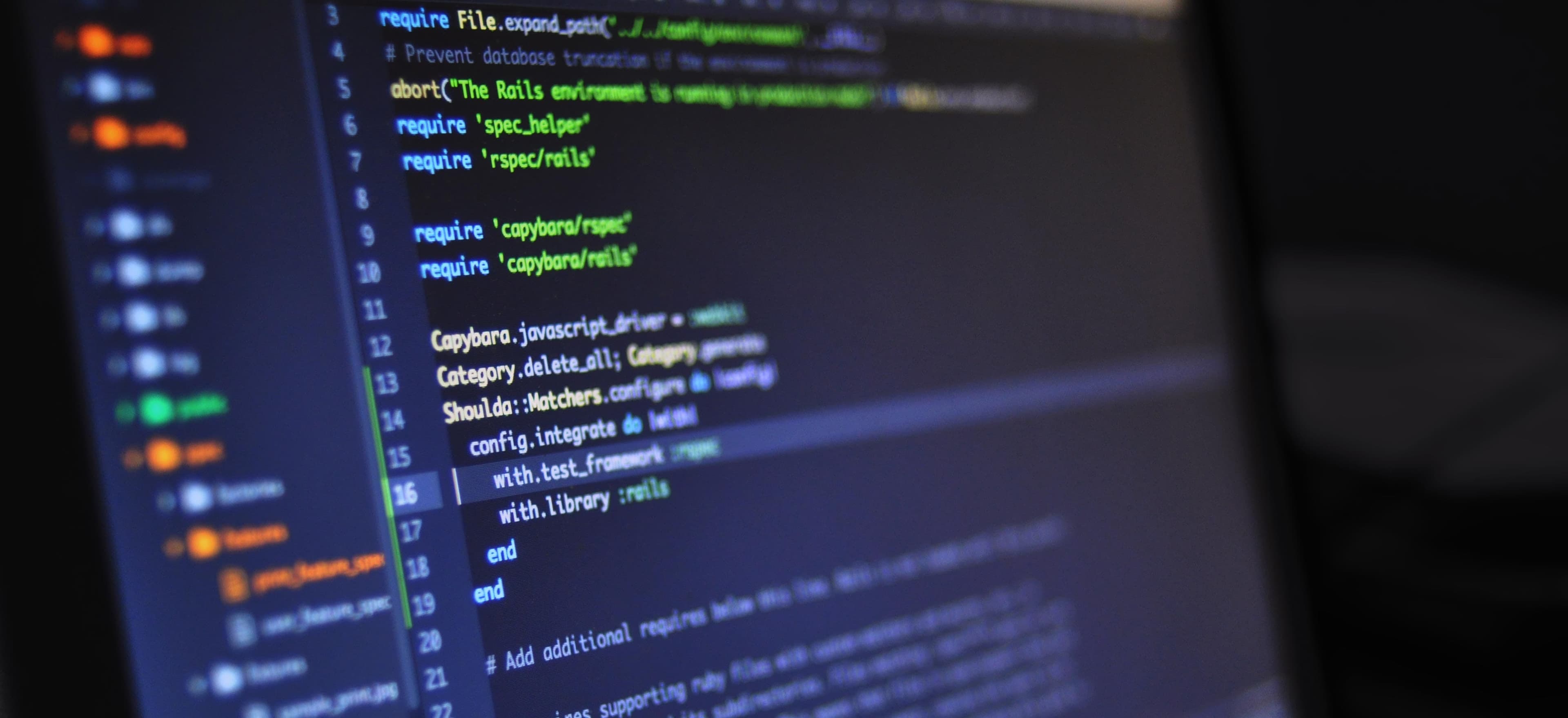
- Published on
How to Fix Hibernate's Dirty Checking Performance Issues
Hibernate is one of the most widely used ORM (Object-Relational Mapping) frameworks in the Java ecosystem. It simplifies database interactions by mapping Java objects to database tables, thus allowing developers to work with persistent data without writing complex SQL queries. One of its core features is "dirty checking," which tracks changes made to an entity and updates the database only when necessary. While this can be beneficial, it can also lead to performance issues under certain circumstances. In this post, we will discuss how to identify and fix Hibernate's dirty checking performance issues.
Understanding Dirty Checking
Before diving into solutions, let’s understand what dirty checking is. In Hibernate, dirty checking refers to the ability of the framework to detect changes made to an entity’s state during a session. This detection is crucial because Hibernate needs to decide which changes to persist to the database when a transaction is committed.
How Dirty Checking Works
Hibernate utilizes the following mechanism for dirty checking:
- Entity State: It keeps a snapshot of the entity when it is loaded into the session.
- Change Detection: During the flush operation (usually at commit), Hibernate compares the current state of the entity with the stored snapshot.
- Update Generation: If differences are found, Hibernate generates SQL update statements synchronously to the database.
While this process is beneficial in reducing unnecessary database transactions, it can become a bottleneck if not managed correctly, particularly in applications with heavy data manipulation or large entity graphs.
Identifying Performance Issues
Before we can fix issues, we need to identify them. Here are several signs that you are facing performance issues due to dirty checking:
- High Memory Usage: Keeping a snapshot of large and complex entities can lead to high memory consumption.
- Slow Transaction Times: If flushing takes longer than expected, it may indicate that dirty checking is taking too much time.
- Long-Running Queries: Excessive dirty checking can contribute to long-running SQL statements, affecting overall application performance.
Profiling Hibernate
Tools like Hibernate Profiler, JPA Buddy, or your application server logs can help you understand performance bottlenecks related to dirty checking.
Strategies to Optimize Dirty Checking
1. Minimize Entity State Changes
Modifying only the necessary attributes of an entity can help reduce the complexity of dirty checking. For example:
public class User {
private String username;
private String email;
private String address;
// Only update email if email has changed
public void updateEmail(String newEmail) {
if (!this.email.equals(newEmail)) {
this.email = newEmail;
}
}
}
In the above code, the updateEmail
method ensures that dirty checking is only performed when the email actually changes. This approach minimizes state changes within the entity and may lead to fewer dirty checks.
2. Consider @DynamicUpdate
When you have large entities with many attributes, using @DynamicUpdate
can optimize the generated update statements. This annotation tells Hibernate to generate SQL updates that include only changed columns.
@Entity
@DynamicUpdate
public class Order {
@Id
private Long id;
private BigDecimal total;
private String status;
}
With @DynamicUpdate
, Hibernate will create SQL updates that only include modified fields, potentially reducing the amount of data sent over the network.
3. Adjust Flush Mode
Hibernate provides different flush modes that control when entities are synchronized with the database. Adjusting the flush mode appropriately can help improve performance.
By default, Hibernate operates in FlushMode.AUTO
, but you can switch it to FlushMode.COMMIT
:
Session session = sessionFactory.openSession();
Transaction tx = session.beginTransaction();
session.setFlushMode(FlushModeType.COMMIT);
// Perform some operations
tx.commit();
session.close();
In FlushMode.COMMIT
, flushing occurs only during the commit phase, which may reduce unnecessary database operations.
4. Use Detached Objects Carefully
In some cases, you might want to use detached objects to optimize performance. When you detach objects, they are no longer managed by the session, and Hibernate will not perform dirty checking on them. However, you lose automatic persistence, so use this method judiciously.
session.evict(user);
user.setEmail("newemail@example.com"); // No dirty checking occurs.
5. Batch Size Configuration
Setting the right batch size for operations can significantly improve performance. By default, Hibernate does not batch operations, which can lead to multiple round trips to the database. Configure the batch size in your Hibernate configuration file:
hibernate.jdbc.batch_size=20
This setting allows Hibernate to send updates in batches, reducing the number of database round trips.
6. Session Management
Managing your session lifecycle effectively can also help with performance. Session per request or session per transaction is recommended practices. Make sure to close sessions when they are no longer needed:
try (Session session = sessionFactory.openSession()) {
Transaction tx = session.beginTransaction();
// Perform your operations
tx.commit();
}
This structure ensures that each operation has a proper lifecycle, preventing session-related memory leaks.
7. Lazy Initialization
Using lazy initialization for collections can prevent unnecessary memory usage related to dirty checking. Configure collections to load only when referenced:
@Entity
public class User {
@OneToMany(fetch = FetchType.LAZY)
private Set<Order> orders;
}
By loading collections lazily, you’ll minimize the data loaded into memory, thus reducing the complexity of dirty checking.
8. Use Stateless Sessions
For batch processing, consider using Stateless Sessions. They do not track changes and perform better for read-only scenarios:
StatelessSession statelessSession = sessionFactory.openStatelessSession();
This option works best when you do not require dirty checking or other ORM features.
A Final Look
Performance issues related to Hibernate's dirty checking can hinder application responsiveness. However, with careful consideration and adjustments, these issues can be mitigated. By minimizing entity state changes, using @DynamicUpdate
, adjusting flush modes, managing sessions effectively, configuring batch sizes, and more, you can optimize your Hibernate applications.
For further reading on Hibernate performance optimization techniques, refer to the Hibernate User Guide.
Optimizing Hibernate's dirty checking doesn't have to be complex. Understanding your application's specific requirements and carefully applying these strategies will undoubtedly lead to improved performance. Happy coding!