Nesting Java Functions: A Guide to Cleaner Code
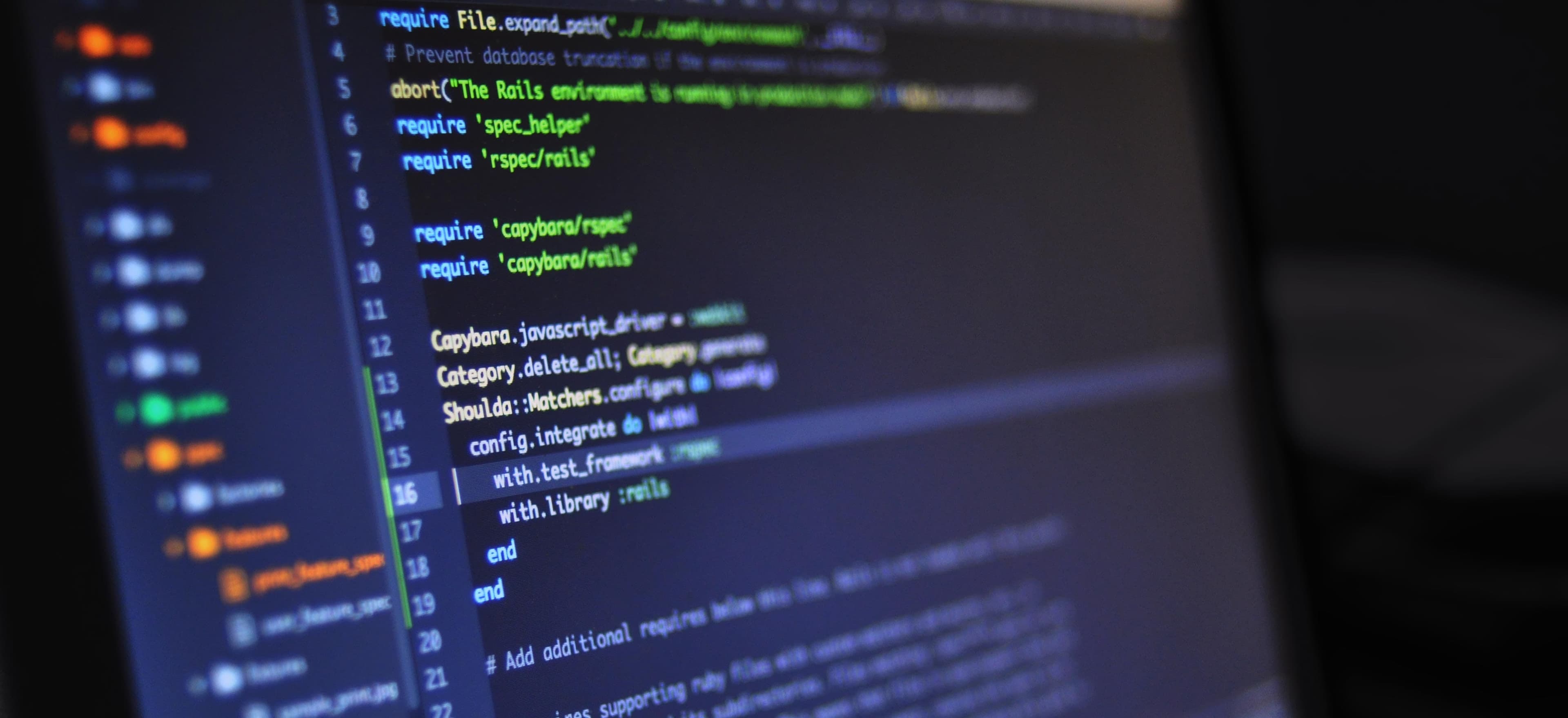
- Published on
Nesting Java Functions: A Guide to Cleaner Code
Nesting functions in Java can be an effective way to tidy up your code, enhance readability, and promote better organization within your applications. In this blog post, we'll delve deep into the concept of nested functions in Java, offering practical examples and highlighting why employing this technique can elevate your programming skills.
What Are Nested Functions?
Nesting functions simply refers to the practice of defining a function within another function. This technique is not as straightforward in Java as it is in some other languages like JavaScript or Python, which allow for more flexible definitions. However, Java provides several mechanisms to achieve similar results, including inner classes, anonymous classes, and lambda expressions.
Why Use Nested Functions?
- Encapsulation: By defining functions within functions, you can limit their scope to where they're needed. This minimizes the risk of conflicting variable names and enhances code encapsulation.
- Increased Readability: Having logic that is closely related grouped together can enhance comprehension.
- Reduction of Redundancy: Nested functions can help encapsulate repetitive tasks, reducing code duplication.
Now that we have a grasp of nested functions, let's explore different approaches in Java.
1. Inner Classes
Java allows you to define classes within classes, known as inner classes. These inner classes can contain methods that would effectively act like nested functions.
Example: Using Inner Classes
public class OuterClass {
private String outerField = "Outer Field";
// Inner class
public class InnerClass {
public void innerMethod() {
System.out.println("Accessing: " + outerField);
}
}
public void outerMethod() {
InnerClass inner = new InnerClass();
inner.innerMethod();
}
}
Commentary:
In the code above, InnerClass
is defined within OuterClass
. The method innerMethod
can access outerField
, which is a field of OuterClass
. This demonstrates a clear example of encapsulation and scope. To invoke innerMethod
, you have to first create an instance of InnerClass
in your outerMethod
. This pattern helps keep related logic closely tied together.
2. Anonymous Classes
Anonymous classes enable you to define a class instance without a named class. They can be useful for one-time use functions.
Example: Using Anonymous Classes
public class Main {
public static void main(String[] args) {
Runnable runnable = new Runnable() {
@Override
public void run() {
System.out.println("Running from an anonymous class!");
}
};
Thread thread = new Thread(runnable);
thread.start();
}
}
Commentary:
In this example, we created an anonymous class that implements the Runnable
interface. The run
method acts as a nested function that can be executed in a separate thread. It serves as a perfect example of when to use anonymous classes: when you need a class for a short tasks without the overhead of a full class definition.
3. Lambda Expressions
Since Java 8, you can use lambda expressions to create instances of functional interfaces. These are very close to the concept of nested functions and provide a clean, succinct way to implement behavior.
Example: Using Lambda Expressions
import java.util.Arrays;
import java.util.List;
public class Main {
public static void main(String[] args) {
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
names.forEach(name -> {
System.out.println("Hello, " + name);
});
}
}
Commentary:
Here, we have a list of names, and we’re using a lambda expression with the forEach
method. The lambda acts like a nested function in that it's defined inline and works directly with the items in names
. It's concise and improves readability significantly compared to a traditional loop.
Best Practices for Using Nested Functions
- Limit Scope: Only use nested functions where necessary to maintain the clarity of function purposes.
- Favor Readability Over Cleverness: While nesting functions can compact code and make it more efficient, it should not come at the cost of readability.
- Use Meaningful Names: Whether you’re working with inner classes, anonymous classes, or lambda expressions, naming matters. Give your functions meaningful names to enhance understandability.
When Not to Use Nested Functions
While nested functions can be beneficial, they are not always the best solution. Here are scenarios where you might want to avoid them:
- Over-Nesting: Too many levels of nesting can lead to confusion. Keep it simple.
- Performance Considerations: Although less common in modern Java, certain patterns of nested functions may lead to performance issues. Always consider the implications.
The Closing Argument
Nesting functions in Java is a powerful technique that can lead to cleaner, more maintainable code. Whether you're using inner classes, anonymous classes, or lambda expressions, the key to effective nesting is encapsulation and readability. Additionally, implementing these techniques can significantly streamline your Java applications.
For more tips on structured programming and command nesting, check out this excellent article, Mastering the Art of Nesting in Bash Commands.
As you incorporate nested functions into your Java applications, remember to apply best practices and avoid over-complication. Happy coding, and may your Java projects benefit from clearer, more organized code!