Avoiding Async Pitfalls in Java: Best Practices Explored
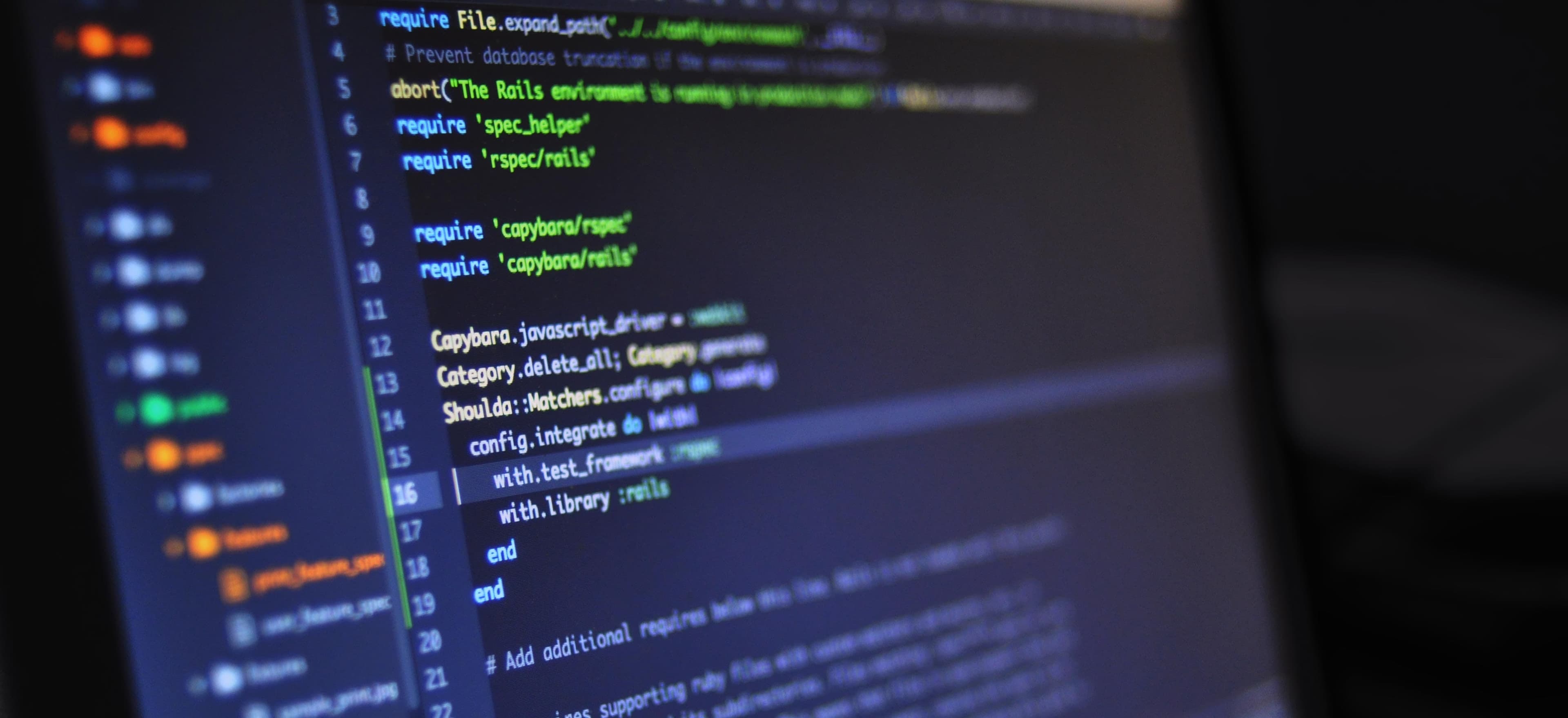
- Published on
Avoiding Async Pitfalls in Java: Best Practices Explored
As we delve into the intricacies of asynchronous programming in Java, it's imperative to choose the right practices to build efficient and resilient applications. While Java provides robust tools for asynchronous programming, developers often encounter various pitfalls. Learning to navigate these is crucial for elevating your code quality. This post provides insights into common challenges and best practices to avoid pitfalls while utilizing asynchronous programming.
Understanding Asynchronous Programming in Java
In the realm of programming, asynchronous operations allow one task to execute independently from another, helping in optimizing the application's performance. In Java, asynchronous programming can be accomplished via several constructs, including CompletableFuture
, ExecutorService
, and reactive programming frameworks like Spring WebFlux.
Using asynchronous patterns serves to improve performance, particularly in I/O-bound tasks. With the proper techniques, you can ensure that your applications are not only efficient but also maintainable.
The Importance of Asynchronous Operations
When tasks run asynchronously, the main thread does not have to wait for the task to complete. This is particularly useful when dealing with operations such as:
- Database queries
- Remote service calls
- File I/O operations
The key benefits include:
- Improved Responsiveness: Applications can process multiple requests concurrently without blocking.
- Better Resource Utilization: By efficiently using the resources, applications can handle more requests simultaneously.
Common Pitfalls in Asynchronous Programming
While asynchronous programming has numerous benefits, it isn’t without its challenges. Some common pitfalls include:
- Ignoring Exceptions: Failing to handle exceptions in your asynchronous tasks can leave your application in an inconsistent state.
- Callback Hell: Nesting too many callbacks can make your code difficult to read and maintain.
- Blocking Calls: Mixing synchronous calls with asynchronous logic can lead to unresponsive applications.
- Resource Leaks: Not properly managing threads can exhaust system resources and lead to application crashes.
For a detailed exploration of these pitfalls in the context of modern Java, I highly recommend checking out the article titled Mastering Async/Await: Common Pitfalls to Avoid.
Best Practices for Avoiding Async Pitfalls
1. Use CompletableFuture for Better Control
CompletableFuture
is a versatile tool that allows non-blocking asynchronous programming in Java. It makes working with callbacks more manageable, resulting in cleaner code.
Example Code:
import java.util.concurrent.CompletableFuture;
public class AsyncExample {
public static void main(String[] args) {
CompletableFuture.supplyAsync(() -> {
// Simulating a long-running job.
try {
Thread.sleep(2000);
} catch (InterruptedException e) {
throw new IllegalStateException("Task interrupted", e);
}
return "Result from asynchronous task";
}).thenAccept(result -> System.out.println("Received: " + result));
System.out.println("Doing other work while waiting...");
// Prevent the program from exiting immediately
try {
Thread.sleep(3000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
Commentary:
In this code, supplyAsync()
starts a task asynchronously. The thenAccept()
method allows us to define what to do with the result once it's available, eliminating the need for nested callbacks. This approach enhances readability and maintainability.
2. Exception Handling with Asynchronous Tasks
When working with asynchronous programming, don't ignore exceptions. Proper handling ensures your application can recover gracefully.
Example Code:
import java.util.concurrent.CompletableFuture;
public class ErrorHandlingExample {
public static void main(String[] args) {
CompletableFuture<Integer> future = CompletableFuture.supplyAsync(() -> {
throw new RuntimeException("Simulated exception");
});
future
.exceptionally(ex -> {
System.out.println("Error occurred: " + ex.getMessage());
return -1; // Fallback value
})
.thenAccept(result -> System.out.println("Result: " + result));
// Prevent the program from exiting immediately
try {
Thread.sleep(2000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
Commentary:
In this example, we demonstrate exception handling by utilizing the exceptionally()
method. This allows the program to catch exceptions thrown during the task execution and provides a fallback mechanism, maintaining stability in your application.
3. Keep Asynchronous and Synchronous Code Separate
Mixing synchronous and asynchronous code can cause unintentional blocking, leading to poor performance. To avoid this, always maintain a clear separation of concerns.
Example Code:
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class SeparateThreadsExample {
private static final ExecutorService executor = Executors.newFixedThreadPool(2);
public static void main(String[] args) {
executor.submit(() -> {
performAsyncTask();
});
System.out.println("Main thread continues without blocking...");
}
private static void performAsyncTask() {
// Much of your I/O-bound or long-running code can go here.
try {
Thread.sleep(2000);
System.out.println("Processing completed in the async task.");
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
Commentary:
This code snippet utilizes an ExecutorService
to manage threads better. By submitting tasks to the executor, we keep our main logic straightforward while isolating the asynchronous logic, which contributes to cleaner code.
4. Limit the Number of Concurrent Tasks
It's easy to overwhelm resources by running too many tasks simultaneously. Make use of CompletableFuture.allOf()
to coordinate multiple asynchronous tasks while managing concurrency limits.
Example Code:
import java.util.concurrent.CompletableFuture;
public class ConcurrencyLimitingExample {
public static void main(String[] args) {
CompletableFuture<Void> future1 = CompletableFuture.runAsync(() -> {
simulateWork("Task 1");
});
CompletableFuture<Void> future2 = CompletableFuture.runAsync(() -> {
simulateWork("Task 2");
});
CompletableFuture<Void> future3 = CompletableFuture.runAsync(() -> {
simulateWork("Task 3");
});
CompletableFuture<Void> allFutures = CompletableFuture.allOf(future1, future2, future3);
allFutures.join(); // Wait for all futures to complete
System.out.println("All tasks completed.");
}
private static void simulateWork(String taskName) {
try {
Thread.sleep(2000);
System.out.println(taskName + " completed.");
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
Commentary:
In this example, we manage multiple asynchronous tasks by running them in parallel. CompletableFuture.allOf()
ensures that we wait for all tasks to signal completion without overwhelming the system resources.
5. Apply Timeouts Where Necessary
In scenarios involving external systems, timeouts are vital for managing latency issues.
Example Code:
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.TimeUnit;
public class TimeoutExample {
public static void main(String[] args) {
CompletableFuture<String> future = CompletableFuture.supplyAsync(() -> {
// Simulating long-running task (beyond timeout).
try {
TimeUnit.SECONDS.sleep(5);
return "Completed";
} catch (InterruptedException e) {
throw new RuntimeException("Task interrupted", e);
}
});
try {
// Timeout after 3 seconds
String result = future.orTimeout(3, TimeUnit.SECONDS).join();
System.out.println(result);
} catch (Exception e) {
System.out.println("Task did not complete in time: " + e.getMessage());
}
}
}
Commentary:
In this scenario, we use the orTimeout()
method to specify a timeout for the async task. If the task doesn't complete within the allotted time, an exception is thrown, allowing for graceful error handling.
Closing the Chapter
Mastering asynchronous programming in Java hinges on understanding the core concepts and being aware of potential pitfalls. By adhering to the best practices highlighted in this post, you can create more reliable, efficient, and maintainable applications. Always remember the importance of exception handling, separating concerns, and the value of well-structured codes, such as those demonstrated through CompletableFuture
.
For further reading on avoiding common pitfalls in Java's asynchronous programming, I highly encourage visiting Mastering Async/Await: Common Pitfalls to Avoid.
With these principles in mind, you can confidently navigate the world of asynchronous programming in Java and create applications that are not only performant but also robust in the face of challenges. Happy coding!