Enhancing Java Application Monitoring with Prometheus Alerts
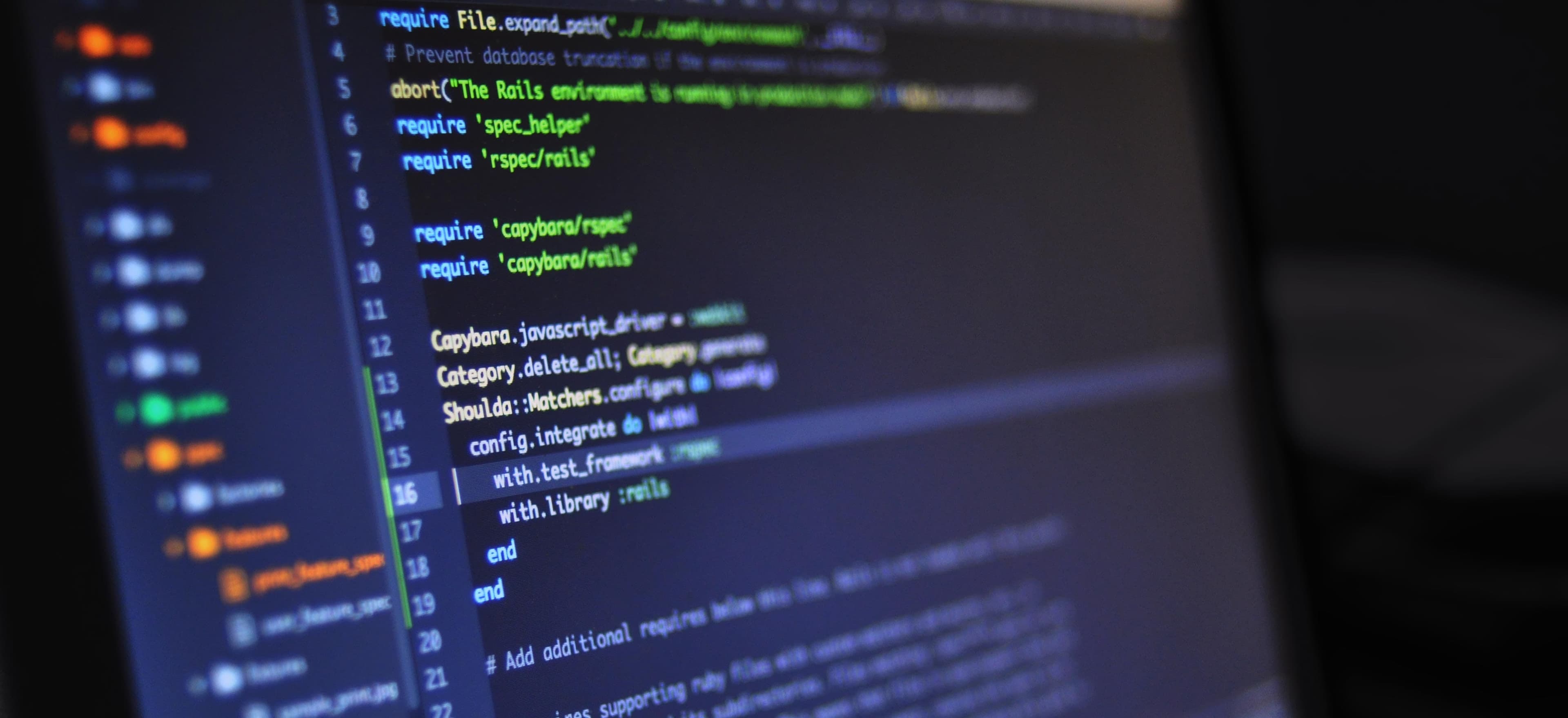
- Published on
Enhancing Java Application Monitoring with Prometheus Alerts
Monitoring is a critical aspect of maintaining the health and performance of any application, especially in complex systems that involve multiple services. As applications evolve, so does the need for more advanced monitoring solutions. One of these solutions is Prometheus, a powerful monitoring and alerting toolkit widely used in the software development community. In this blog post, we will explore how to harness the power of Prometheus alerts specifically for Java applications.
What is Prometheus?
Prometheus is an open-source monitoring solution that collects metrics from configured targets at specified intervals, evaluates rule expressions, and can trigger alerts if certain conditions are met. Its pull-based model, combined with robust querying capabilities, makes it an excellent choice for monitoring modern cloud-native architectures.
Why Use Prometheus for Java Applications?
Using Prometheus with Java applications offers several advantages:
- Scalability: Prometheus can scrape metrics from many instances of your Java application, enabling it to scale as your services grow.
- Rich Ecosystem: Prometheus has a rich ecosystem of exporters, making it easy to collect metrics from various sources.
- High Availability: With Prometheus, you can set alerts based on metrics, ensuring that important issues are notified promptly to your engineering team.
Getting Started with Prometheus in Java
To start monitoring your Java applications using Prometheus, Integrate the Micrometer library, which acts as a facade for various monitoring systems, including Prometheus. Here's a quick guide to focus on setup and configuration.
Step 1: Adding Dependencies
Ensure you have the relevant dependencies added to your pom.xml
file if you're using Maven:
<dependencies>
<dependency>
<groupId>io.micrometer</groupId>
<artifactId>micrometer-core</artifactId>
</dependency>
<dependency>
<groupId>io.micrometer</groupId>
<artifactId>micrometer-registry-prometheus</artifactId>
</dependency>
</dependencies>
Here, the micrometer-registry-prometheus
dependency allows you to export metrics to Prometheus. By utilizing Micrometer, we can keep our application lightweight while offering rich metrics functionality.
Step 2: Configuring the Prometheus Endpoint
To expose metrics, you need to configure an HTTP endpoint in your application. Here's a basic Spring Boot configuration:
import io.micrometer.core.instrument.MeterRegistry;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@SpringBootApplication
public class MonitoringApplication {
public static void main(String[] args) {
SpringApplication.run(MonitoringApplication.class, args);
}
}
@RestController
class MetricsController {
private final MeterRegistry meterRegistry;
public MetricsController(MeterRegistry meterRegistry) {
this.meterRegistry = meterRegistry;
}
@GetMapping("/metrics")
public String getMetrics() {
return meterRegistry.getMeters().toString();
}
}
In this code snippet, we expose an HTTP endpoint /metrics
that returns the metrics collected by Micrometer, which can then be scraped by Prometheus. Using a controller helps us to handle HTTP requests and serve metrics on demand.
Why This Matters
Exposing metrics allows Prometheus to pull performance data about your application, enabling the generation of insightful dashboards and alerts.
Prometheus Configuration
After setting up your Java application, you need to configure your Prometheus server to scrape these metrics. This is done in the prometheus.yml
configuration file:
scrape_configs:
- job_name: 'java_application'
static_configs:
- targets: ['localhost:8080']
This configuration tells Prometheus to scrape data from the /metrics
endpoint of your running Java application.
Step 3: Defining Alert Rules
Once Prometheus is scraping the metrics successfully, it’s time to define alerting rules. Alerts can be configured based on various conditions such as error rates, latency, or resource usage. Here's an example alert rule in alerts.yml
:
groups:
- name: example_alerts
rules:
- alert: HighErrorRate
expr: rate(http_server_errors_total[5m]) > 0.05
for: 5m
labels:
severity: critical
annotations:
summary: "High error rate detected in Java application"
description: "The error rate has exceeded 5% in the last 5 minutes."
Why Use Alerts?
Alerts provide real-time notification when certain thresholds are exceeded. This enables your team to address potential issues proactively, which can significantly reduce downtime and improve user experience.
Integrating with Alertmanager
Although Prometheus can handle basic alerting, for a more robust notification system, you should integrate with Alertmanager. Alertmanager can group alerts, manage silences, and route notifications to various endpoints like email, Slack, or PagerDuty.
To set up Alertmanager, configure the following in the Alertmanager config file:
route:
group_by: ['alertname']
group_wait: 30s
group_interval: 5m
repeat_interval: 1h
receiver: 'slack-notifications'
receivers:
- name: 'slack-notifications'
slack_configs:
- channel: '#alerts'
send_resolved: true
Communication is Key
Integrating with notification systems ensures that alert notifications reach team members instantly, enabling a quicker response to critical incidents.
Best Practices for Monitoring Java Applications with Prometheus
Now that you have the basic setup and configurations, let’s summarize some best practices to follow while using Prometheus to monitor Java applications:
-
Instrument Your Code: Always avoid collecting more data than necessary. Focus on key performance indicators that truly reflect the health and performance of your application.
-
Use Labels Effectively: Utilize labels to provide more context around your metrics. Examples include environment (production or staging), service name, and version.
-
Define Clear Alerting Rules: Make sure your alerts are actionable and pertinent. Avoid alert fatigue by tuning thresholds and prioritizing critical alerts.
-
Regularly Review Metrics: Set aside time to review the metrics captured throughout your application lifecycle. This analysis can guide performance improvements and capacity planning.
-
Leverage Existing Resources: Explore available resources to optimize your alert configurations. For example, you can refer to the article titled Improving Prometheus Alert Notifications for further ideas.
Final Thoughts
Prometheus and its alerting capabilities significantly enhance your ability to monitor Java applications effectively. With the right configuration, alert rules, and integration into your workflow, you can ensure a robust monitoring strategy that keeps your applications healthy and responsive.
By implementing these practices, you not only improve the resilience of your application but you also provide a better experience for your users. The proactive nature of alerts ensures that issues are detected and dealt with promptly, contributing to higher customer satisfaction and service reliability.
With the explosive growth of cloud-native architectures, the importance of sophisticated monitoring solutions like Prometheus cannot be overstated. As you implement these strategies in your Java applications, you will cultivate a deeper understanding of your metrics, enabling you to make informed decisions and enhance your applications further.
Checkout our other articles