Crafting Clear Boolean Names in Java for Better Readability
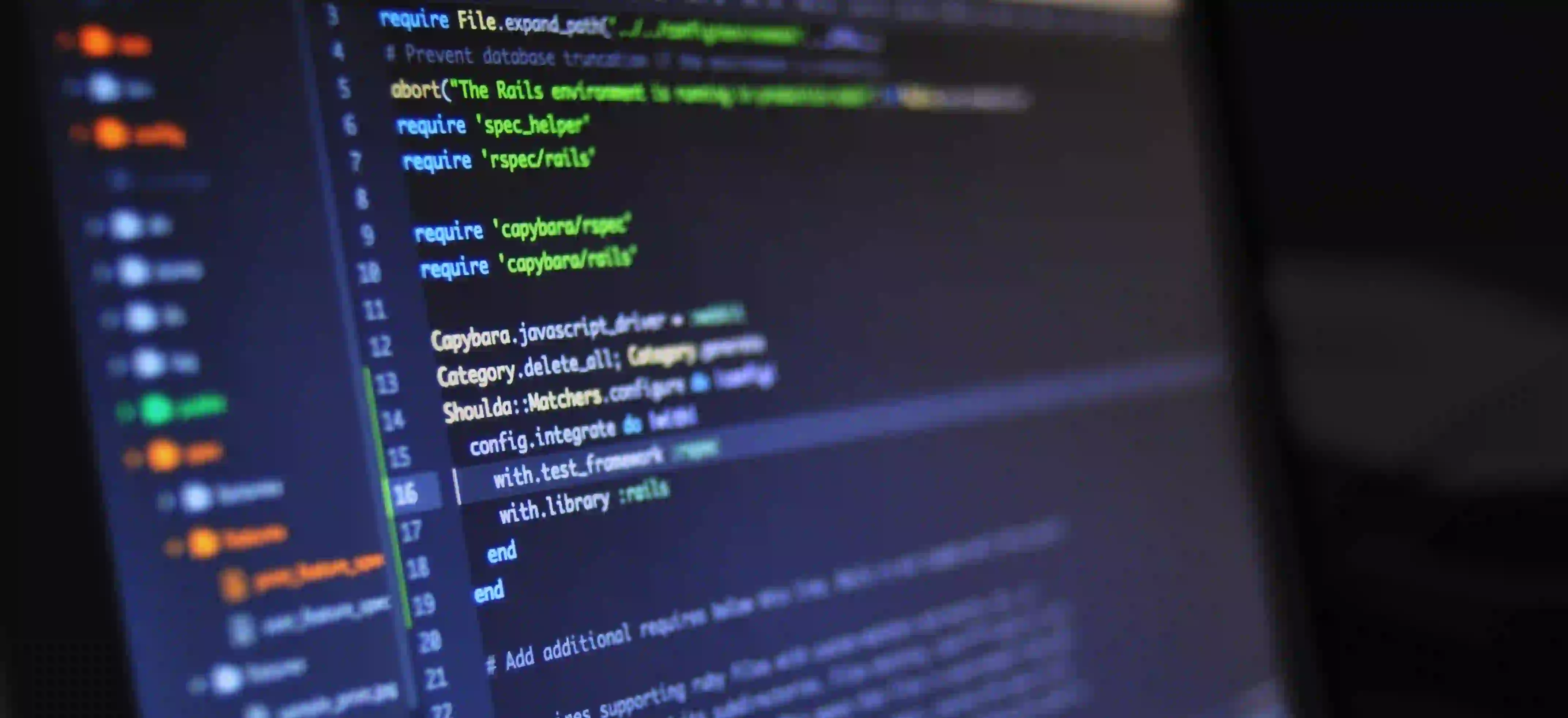
Crafting Clear Boolean Names in Java for Better Readability
In the world of programming, clarity is paramount. When it comes to Boolean variables in Java, well-chosen names can greatly enhance code readability and maintainability. This article will explore the significance of crafting clear Boolean variable names, the best practices for doing so, and how it integrates into the broader context of readable code. We will draw upon concepts from the existing article "Mastering Boolean Variable Names for Readable Code" (available at infinitejs.com/posts/mastering-boolean-variable-names) to provide a comprehensive perspective on this important matter.
Understanding the Role of Boolean Variables
Boolean variables represent a binary state: true or false. They are often used for decision-making and flow control in your Java applications. The naming of these variables should provide clarity about what ‘true’ or ‘false’ signifies. This clarity not only enhances your code’s readability but also aids in its maintainability.
Why Naming Matters
Consider a scenario where a developer must decide whether to allow access based on user roles. Using vague names like isAllowed
or flag
can cause confusion. Instead, a more descriptive name such as hasAdminPermissions
provides immediate insight into the variable's purpose.
boolean hasAdminPermissions = checkUserRole(user);
In this example, the variable name succinctly communicates what the code intends to determine.
Best Practices for Naming Boolean Variables
-
Use Affix Conventions
For boolean variables, prefixes are instrumental in enhancing readability. The most common prefixes include:
is
has
can
should
was
Each prefix serves a specific purpose and suggests the expected value:
- is: Used for state-checking or status verification (e.g.,
isActive
,isComplete
). - has: Indicates possession or ownership (e.g.,
hasChildren
,hasAccess
). - can: Suggests capability or permission (e.g.,
canEdit
,canDelete
). - should: Implies a recommended state or best practice (e.g.,
shouldNotify
). - was: Used for tracking past states (e.g.,
wasInitialized
).
Consider the following function that checks if a user is active:
☕snippet.javaboolean isActive = user.getStatus() == AccountStatus.ACTIVE;
Here,
isActive
immediately conveys the intention to check the user’s status. -
Be Specific
Vague names do not aid any developer's understanding. Instead, ensure that variable names are specific and convey precise meaning.
Poor Example:
☕snippet.javaboolean status;
Better Example:
☕snippet.javaboolean isUserLoggedIn;
The latter conveys a clear purpose, making it instantly understandable.
-
Avoid Negation in Naming
When naming boolean variables, avoid using negative forms. Negative names, such as
isNotActive
, can lead to confusion. It’s better to use a positive affirmation or a clearer statement.Poor Example:
☕snippet.javaboolean isNotAdmin;
Better Example:
☕snippet.javaboolean isRegularUser;
By avoiding negation, you minimize cognitive load while reading through your code.
-
Utilize Context
Providing context enhances variable clarity. Especially in larger classes or functions, contextual naming helps developers grasp what the boolean represents without needing to sift through the implementation.
☕snippet.javaboolean isDiscountAvailableForUser;
This name makes clear that the status relates directly to a discount applicable to a user.
-
Consistent Naming Patterns
Just as important as individual names is the consistency throughout your codebase. Following a consistent naming convention across all your boolean variables enhances readability and makes your code more predictable.
If you decide on using
can
for capability checks, likecanEdit
, stick to this format wherever applicable. Consistency aids comprehension.
Code Examples in Real Application
Let’s implement some of these principles in real-life Java code scenarios. Here’s a simple user role-checking example.
public class User {
private String role;
public User(String role) {
this.role = role;
}
public boolean hasAdminPermissions() {
return "ADMIN".equals(role);
}
public boolean isActive() {
return !role.isEmpty();
}
}
Here’s what we are doing:
hasAdminPermissions()
useshas
to indicate access rights.isActive()
employsis
to show the check on the state of the user's role.
By following these naming conventions, the code is clearer, making it easier for future developers or even yourself to understand the logic at a glance.
Testing Boolean Functionality
Good practice requires testing your Boolean functionalities. You want to ensure your naming directly corresponds to the expected result.
User adminUser = new User("ADMIN");
assert adminUser.hasAdminPermissions() : "Admin user should have admin permissions";
User regularUser = new User("USER");
assert !regularUser.hasAdminPermissions() : "Regular user should not have admin permissions";
In this test case, we leverage the clarity provided by the boolean names. Our assertions are self-explanatory, making it easy to comprehend what they're testing.
Closing the Chapter
Crafting clear Boolean names is crucial for enhancing the readability of code in Java. By employing clear naming conventions, adhering to best practices, and maintaining a consistent approach, developers can contribute to a codebase that is easily understood and maintained. The insightful methods detailed in this article not only reflect the principles found in "Mastering Boolean Variable Names for Readable Code" but also set a foundation for writing more readable, maintainable code.
For more in-depth insights into writing clear boolean variable names, reference the article Mastering Boolean Variable Names for Readable Code.
Implement these practices, and watch your code transform into a more readable, understandable, and maintainable form. Happy coding!