Naming Boolean Variables: Java Best Practices for Clarity
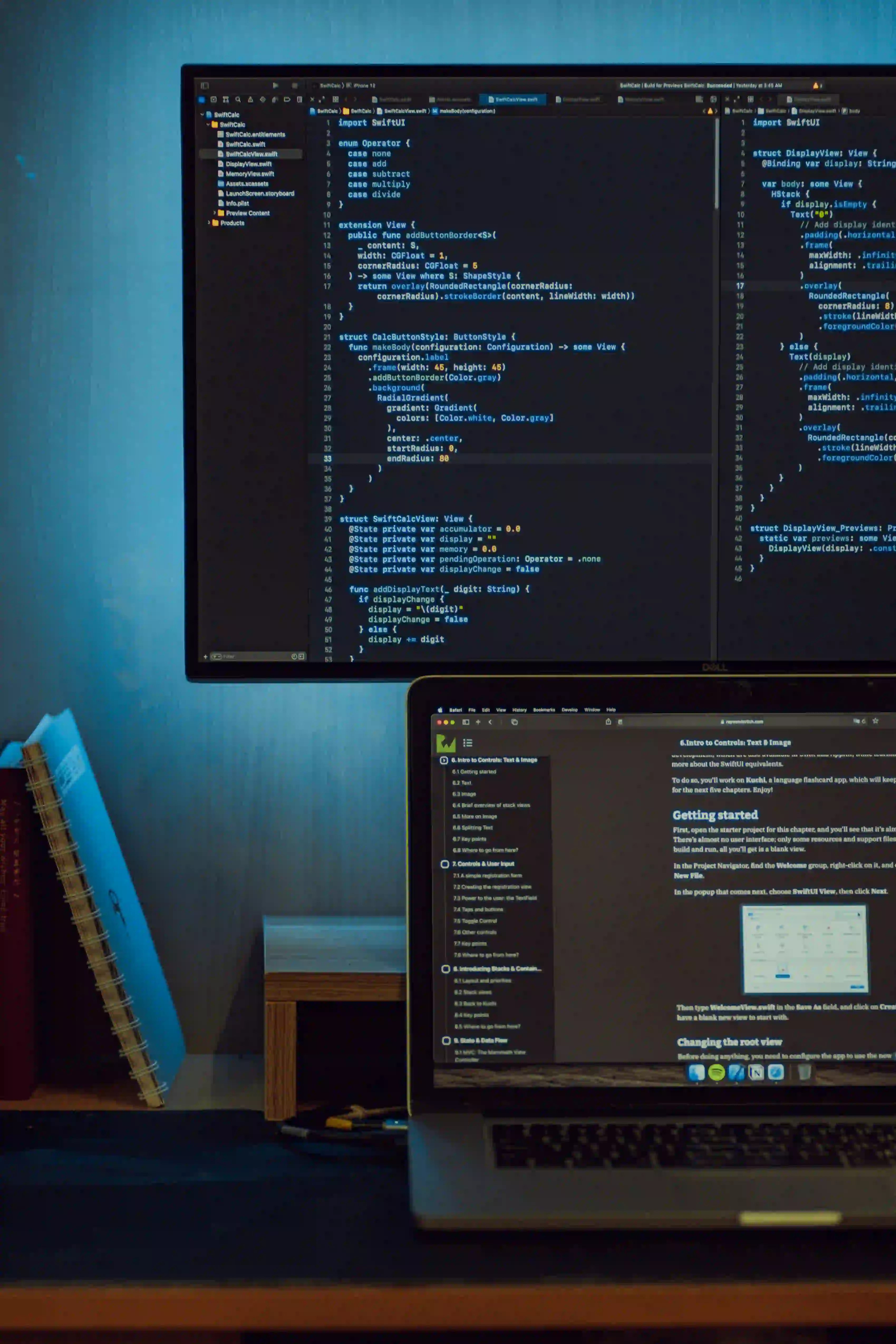
Naming Boolean Variables: Java Best Practices for Clarity
When it comes to writing clean and maintainable code in Java, naming conventions play a crucial role. Among various variable types, Boolean variables deserve special attention. A well-named Boolean variable can greatly enhance the readability of your code, making it easier for others (and yourself) to understand the logic without excessive commenting.
In this article, we will delve into the best practices for naming Boolean variables in Java. We will explore why proper naming is important, provide clear examples, and link to further resources, including the insightful article, "Mastering Boolean Variable Names for Readable Code," which can be found here.
Why Naming Matters
Why should we care about naming, especially for Boolean variables? Well, naming directly influences code quality. A clear name can eliminate confusion, while a vague name can lead to misinterpretation. In many cases, a poorly named variable can result in hours of debugging or miscommunication among team members.
Consider two Boolean variables:
boolean isActive;
boolean hasPermission;
These names immediately convey their purpose. Someone reading the code can quickly infer that isActive
signifies whether an entity is currently active, while hasPermission
clarifies if access rights exist.
The Importance of Clarity in Boolean Naming
When naming Boolean variables, the goal should be clarity and intent. You want the name to reflect the state or condition that the variable is managing. This is especially critical when your codebase scales or when multiple developers are involved.
Use Positive Naming Conventions
One of the most effective practices is using positive language for your Boolean variable names. A positive name eliminates double negatives, which can be confusing.
Instead of:
boolean isNotOverdue;
Use:
boolean isOverdue;
The preferred naming method creates a natural understanding of what the variable signifies, thereby reducing cognitive load on the reader.
Prefixing with "is", "has", or "can"
Prefixes convey the type of check being performed or the condition being evaluated. Here are some common prefixes:
- is: Used for states or conditions.
- has: Implies possession or availability.
- can: Implies ability.
boolean isVisible;
boolean hasAccess;
boolean canEdit;
Deploying these prefixes adds an immediate context and structure to your naming conventions, guiding the reader through your intended logic.
Avoiding Ambiguity
Avoid using ambiguous names that do not offer insight into their purpose.
Instead of:
boolean status;
Choose a descriptive name:
boolean isUserLoggedIn;
This change helps clarify the variable's role instantly, avoiding potential confusion later.
Examples in Context
Example 1: User Authentication
Let's look at a simplistic login check.
boolean isUserAuthenticated;
In this case, the variable clearly states if a user has been authenticated. The choice of the prefix is
conveys a state and complements the Boolean true/false values effectively.
Example 2: Feature Flags
When implementing feature toggles in a Java application, consider structuring your Boolean variables meaningfully:
boolean isFeatureXEnabled;
boolean isBetaAccessGranted;
These variable names immediately communicate their purpose. They manage the state of features and access, and a developer can easily comprehend their roles.
Example 3: Flagging Permissions
Managing permissions can often lead to cluttered and confusing code.
Instead of using general names:
boolean permissionStatus;
Choosing a specific name brings clarity:
boolean canDeletePost;
Such naming improves the readability of conditional checks throughout the application.
Refactoring for Improved Naming
If you analyze your existing code and find poorly named Boolean variables, don't hesitate to refactor. Refactoring is essential for:
- Enhancing future maintainability
- Making code self-documenting
- Reducing the chance of errors during revisions
For example, if you find a Boolean variable named status
, consider refactoring it to something more descriptive:
boolean isEmailVerified;
// Instead of
boolean status;
Refactoring can lead to clearer, more maintainable code that others can easily understand.
Tools for Handling Naming Conventions
Several tools and IDE features can aid in maintaining naming conventions. For instance:
Code Linters
Using tools such as Checkstyle or PMD can help enforce naming conventions in your Java code. These linters can catch naming violations that deviate from the standards you've set for your codebase.
IDE Features
Most modern IDEs, including IntelliJ IDEA and Eclipse, provide built-in inspections for code quality and can suggest naming conventions. These suggestions can be a great starting point when naming variables, including Boolean types.
The Last Word
The way you name your Boolean variables can significantly impact the readability and maintainability of your Java code. By using clear, descriptive naming conventions, properly applying prefixes, avoiding ambiguity, and refactoring as needed, you can ensure your codebase remains clean and understandable.
Always remember the importance of clarity in code—after all, you are not only writing for the machine but also for your future self and other developers. For a deeper understanding of this topic, consider reading the article, "Mastering Boolean Variable Names for Readable Code," available here.
By following these best practices, you will foster a culture of clean code, allowing for efficient collaboration and enhancing the overall quality of your software development process. Happy coding!