Boosting Spring Boot Performance: Handling Requests Efficiently
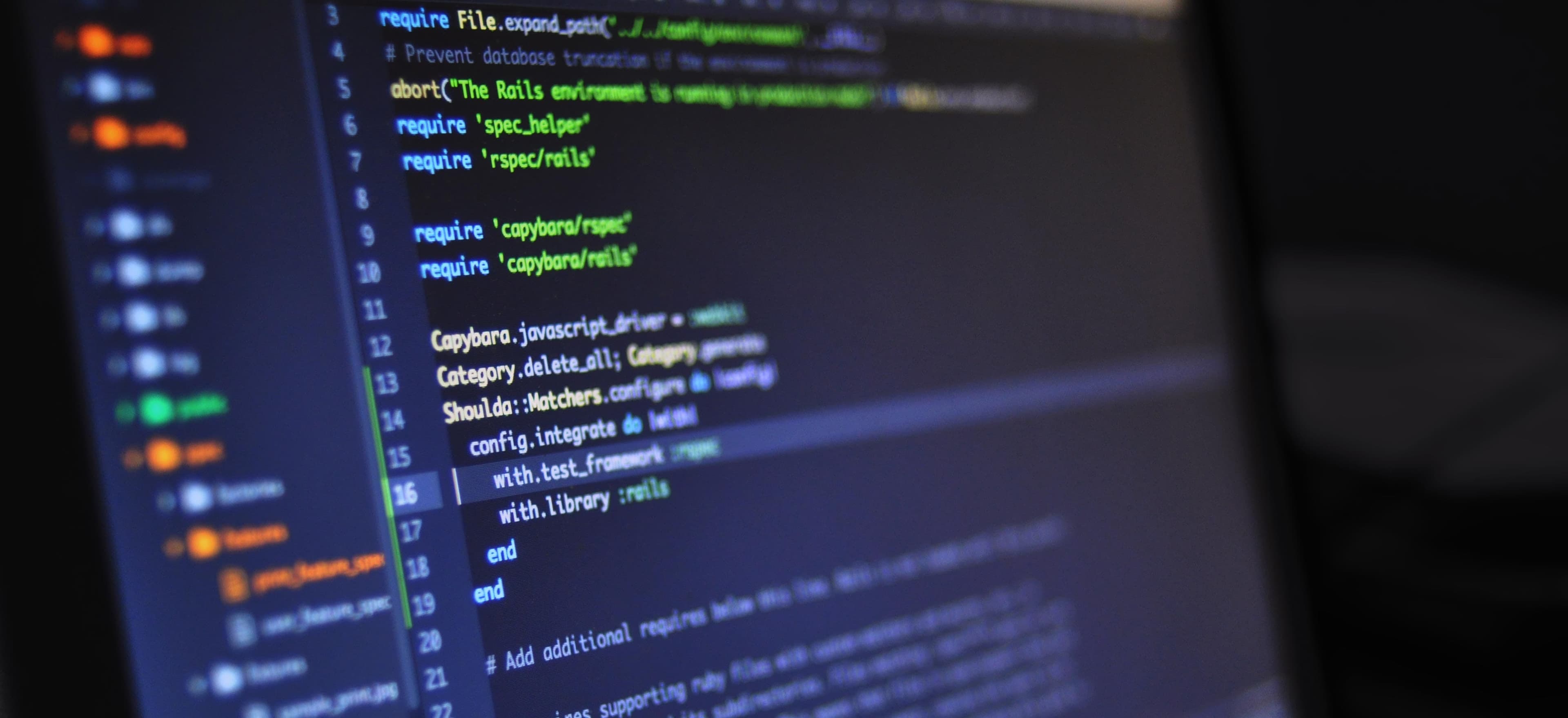
- Published on
Boosting Spring Boot Performance: Handling Requests Efficiently
Spring Boot has revolutionized the way developers create Java applications. Its simplicity and effectiveness empower developers to deploy robust applications quickly. However, with great power comes great responsibility. As applications scale, performance can become a challenge. In this article, we will explore various strategies for optimizing the performance of Spring Boot applications, specifically focusing on how to handle requests efficiently.
Understanding Spring Boot's Request Handling
At its core, Spring Boot is built on the Spring Framework, and it employs a powerful request-response architecture. When a client makes a request, the server processes it based on predefined routes. This involves data processing, inter-service communication, and rendering responses. Given the numerous steps involved, it’s crucial to optimize each phase to boost performance effectively.
1. Asynchronous Request Processing
One of the most effective ways to handle requests efficiently is by making them asynchronous. Non-blocking I/O allows the server to handle other requests while waiting for responses from an external service, thus maximizing resource utilization.
Example: Asynchronous Controller
Here's a simple example using Spring's @Async
annotation:
import org.springframework.scheduling.annotation.Async;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
import java.util.concurrent.CompletableFuture;
@RestController
public class MyAsyncController {
@Async
@GetMapping("/async-endpoint")
public CompletableFuture<String> asyncEndpoint() {
// Simulate a long-running task
try {
Thread.sleep(5000);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
return CompletableFuture.completedFuture("Async Response");
}
}
Why Use @Async
?
The @Async
annotation allows Spring to handle the method in a separate thread, which means that the server can continue processing other requests while waiting for the long-running task to finish. This leads to better scalability and responsiveness.
2. Connection Pooling
Managing database connections efficiently is another critical performance factor. Using a connection pool allows your application to reuse database connections instead of creating new ones for every request, reducing latency significantly.
Implementing Connection Pooling
You can configure connection pooling in your application.properties
file:
spring.datasource.url=jdbc:mysql://localhost:3306/mydb
spring.datasource.username=myuser
spring.datasource.password=mypassword
spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver
# HikariCP settings (default connection pool in Spring Boot)
spring.datasource.hikari.maximum-pool-size=10
spring.datasource.hikari.minimum-idle=5
spring.datasource.hikari.idle-timeout=30000
Why Connection Pooling Matters? By pooling connections, you minimize the overhead associated with establishing and tearing down connections to your database. This results in reduced response times and better resource management.
3. Caching Strategies
Implementing caching is a fundamental technique to improve application performance. Caching reduces the need to fetch data repeatedly from a database or service, significantly speeding up response times.
Here’s an example of using Spring’s caching capabilities:
Adding Caching to Your Application
First, annotate your main application class to enable caching:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cache.annotation.EnableCaching;
@SpringBootApplication
@EnableCaching
public class MyApplication {
public static void main(String[] args) {
SpringApplication.run(MyApplication.class, args);
}
}
Then, you can use the @Cacheable
annotation in your service methods:
import org.springframework.cache.annotation.Cacheable;
import org.springframework.stereotype.Service;
@Service
public class UserService {
@Cacheable("users")
public User findUserById(Long id) {
// Simulating slow database call
try {
Thread.sleep(3000);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
return fetchUserFromDatabase(id);
}
private User fetchUserFromDatabase(Long id) {
// Perform database fetching logic here
return new User(id, "John Doe");
}
}
Why Cache?
When you use @Cacheable
, Spring caches the method's return value, and for subsequent calls with the same parameter, it instantly retrieves the result without invoking the method again. This leads to substantial performance gains.
4. Compression Middleware
When dealing with large responses, consider using compression. By enabling GZIP compression on your server responses, you can reduce the amount of data transmitted over the network.
Enabling GZIP Compression in Spring Boot
You can easily enable GZIP compression by modifying the application.properties
:
spring.servlet.multipart.enabled=true
server.compression.enabled=true
server.compression.mime-types=text/html,text/xml,text/plain,text/css,text/javascript,application/javascript,application/json
server.compression.min-response-size=2048
Why GZIP Compression? GZIP compression significantly reduces the size of responses, which leads to faster load times and improved resource utilization. Clients also benefit from reduced download times.
5. Profiling and Monitoring
To truly understand where performance bottlenecks exist, consider implementing profiling and monitoring solutions. Tools like Spring Boot Actuator provide endpoints to monitor and gain insights into your application's health, metrics, and more.
Implementation
Add the dependency to your pom.xml
:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
In your application.properties
, you can expose specific endpoints:
management.endpoints.web.exposure.include=health,metrics
Why Monitor? By continuously monitoring application performance and health, you can identify bottlenecks and apply targeted optimizations. Regular profiling also uncovers potential memory leaks or inefficient algorithms.
Summary
Optimizing the request-handling capabilities of a Spring Boot application is crucial for maintaining performance as user demands grow. Implementing strategies like asynchronous processing, connection pooling, effective caching, response compression, and robust monitoring can lead to substantial improvements.
Utilizing these techniques not only enhances the user experience but also ensures efficient usage of resources, contributing to the longevity and scalability of your applications. For more in-depth performance tuning strategies, you might want to explore additional resources such as Spring Performance Tuning.
By writing efficient code and effectively leveraging Spring Boot's features, your Java applications can handle requests more gracefully, providing a seamless experience for users.
Checkout our other articles