Common Mistakes in Java Naming Conventions to Avoid
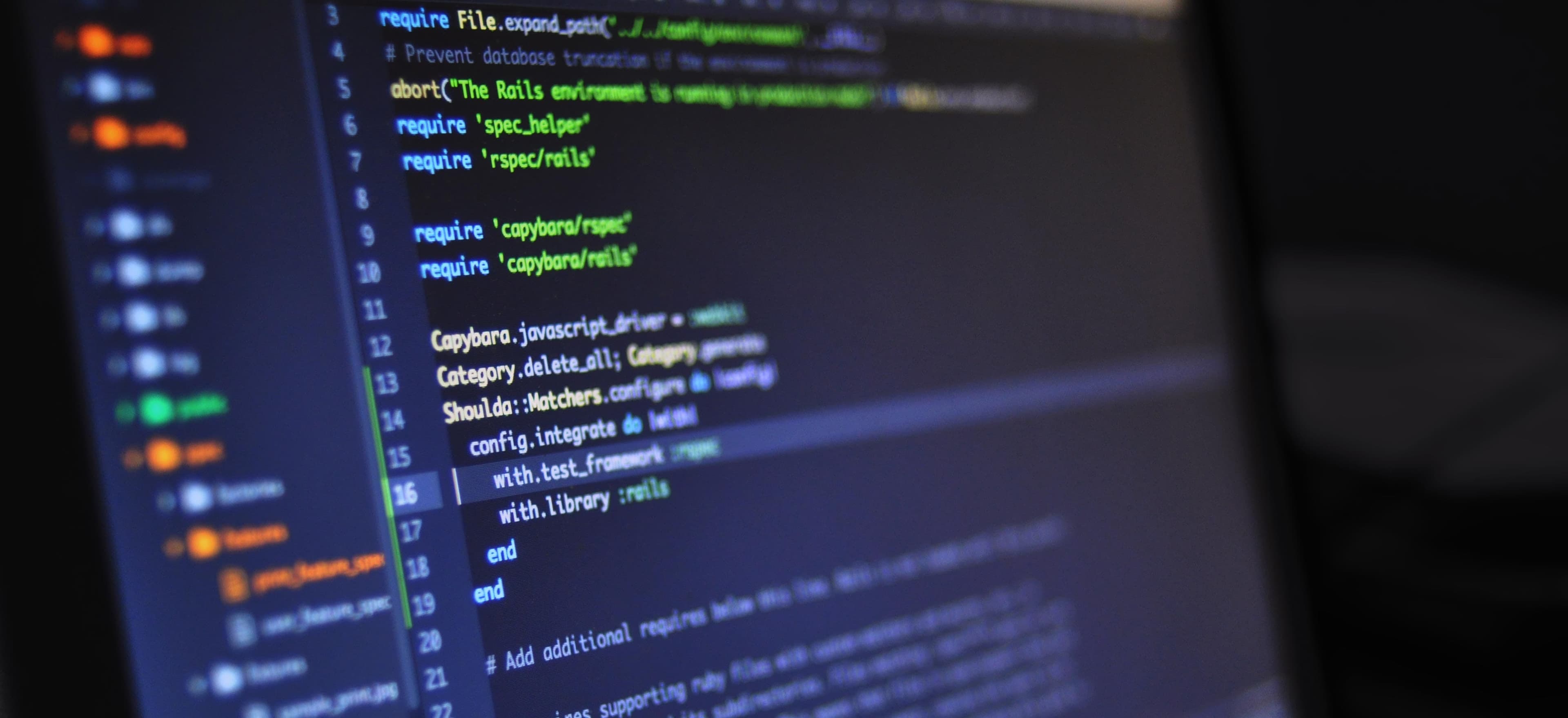
- Published on
Common Mistakes in Java Naming Conventions to Avoid
When writing Java code, one of the most critical yet often overlooked aspects is adhering to naming conventions. Proper naming conventions enhance code readability, maintainability, and professionalism. In this blog post, we will explore common mistakes developers make in naming conventions and how to avoid them. Not only will this elevate your coding standards, but it will also make your projects easier for others (and future you) to understand.
Importance of Naming Conventions
Before diving into specific mistakes, let’s first understand the importance of naming conventions in Java.
- Readability: Well-named variables, classes, and methods are easier to read and understand.
- Maintenance: Code that's easier to understand is also easier to maintain and update.
- Collaboration: In team environments, consistent naming conventions reduce confusion for team members.
- Professionalism: Adhering to common standards reflects your professionalism and attention to detail.
The Java community has established naming conventions that developers commonly follow. Here is a quick overview of these conventions:
- Classes: Use CamelCase, starting with an uppercase letter.
- Methods: Use camelCase, starting with a lowercase letter.
- Variables: Also use camelCase.
- Constants: Use uppercase letters with underscores.
Common Naming Convention Mistakes
Let's explore some common mistakes in Java naming conventions and how you can avoid them.
1. Inconsistent Case Usage
One of the biggest mistakes is inconsistent usage of cases. Developers sometimes switch between CamelCase and lowercase, leading to confusion.
Incorrect Example
public class userProfile {
private String UserName;
public void setUsername(String userName) {
this.UserName = userName;
}
}
Why This is Wrong: The class name userProfile
should be UserProfile
. The variable UserName
should be username
to maintain consistency.
Corrected Example
public class UserProfile {
private String username;
public void setUsername(String username) {
this.username = username;
}
}
2. Using Generic Names
Another common mistake is using overly generic names such as temp
, data
, or stuff
. These names do not convey any context about the variable's purpose.
Incorrect Example
int temp;
Why This is Wrong: Renaming temp
to a more descriptive name clarifies its purpose.
Corrected Example
int temperatureInCelsius;
3. Not Using Meaningful Names
It’s tempting to abbreviate names for brevity, but this often results in losing clarity. Using full, descriptive names takes a bit more time but pays off in the long run.
Incorrect Example
public void pr() {
// Print report logic
}
Why This is Wrong: The method name pr()
does not describe what it does.
Corrected Example
public void printReport() {
// Logic to print the report
}
4. Confusing Class and Interface Names
Some developers make the mistake of not differentiating class names from interface names. By convention, interfaces should have names that suggest an action or capability.
Incorrect Example
public interface List {
// interface methods
}
Why This is Wrong: List
can easily be confused with the Java Collections Framework class.
Corrected Example
public interface Printable {
void print();
}
5. Capitalizing Variables Incorrectly
Capitalization is essential in Java naming conventions. Many developers mistakenly use uppercase letters for non-constant variables.
Incorrect Example
public class Person {
public String Name; // incorrect capitalization
}
Why This is Wrong: Variables should be in lowercase.
Corrected Example
public class Person {
public String name; // corrected capitalization
}
6. Using Underscores in Variable Names
While some programming languages use underscores for separating words, Java does not. Instead, camel case should be employed.
Incorrect Example
public class Student_Score {
private int student_score;
}
Why This is Wrong: The Student_Score
class and student_score
variable should use camel case.
Corrected Example
public class StudentScore {
private int studentScore;
}
7. Avoiding the Use of Hungarian Notation
Hungarian Notation is a naming convention where variable names include prefixes indicating their data types. This practice is generally discouraged in Java.
Incorrect Example
public String strName;
Why This is Wrong: In Java, the data type is already known from the declaration.
Corrected Example
public String name; // cleaner and more readable
8. Names That Are Too Similar
Sometimes developers use names that are confusingly similar, making it hard to distinguish between them.
Incorrect Example
public class Order {
private String orderNumber;
private String orderNum;
}
Why This is Wrong: Having orderNumber
and orderNum
is confusing.
Corrected Example
public class Order {
private String orderNumber;
private String customerOrderNumber; // Descriptive and distinct
}
9. Not Following the Naming Convention for Constants
Constants should be entirely uppercase and utilize underscores to separate words. A frequent mistake is to omit uppercase letters.
Incorrect Example
public static final String defaultShape = "Circle"; // incorrect
Why This is Wrong: The constant name should be in uppercase.
Corrected Example
public static final String DEFAULT_SHAPE = "CIRCLE"; // corrected
10. Using Reserved Words
One crucial mistake is naming variables or methods with Java reserved keywords. Doing so will lead to compiler errors.
Incorrect Example
public class Class {
public void public() {
// logic
}
}
Why This is Wrong: public
is a reserved keyword in Java.
Corrected Example
public class MyClass {
public void handlePublic() {
// logic
}
}
Closing Remarks
Understanding and adhering to proper naming conventions is crucial for writing clean, maintainable Java code. By avoiding these common mistakes, your code will be more readable, and others will appreciate the time you invested in creating well-structured code.
For further reading, you can explore Oracle's Java Code Conventions for a deeper understanding of best practices in Java coding standards.
Additional Resources
- Effective Java by Joshua Bloch
- Clean Code: A Handbook of Agile Software Craftsmanship by Robert C. Martin
By following the principles outlined in this post, you will contribute to a more professional and efficient development environment, whether you are working solo or as part of a larger team. Happy coding!
Checkout our other articles