Securing Your API Gateway with Robust Lambda Authentication
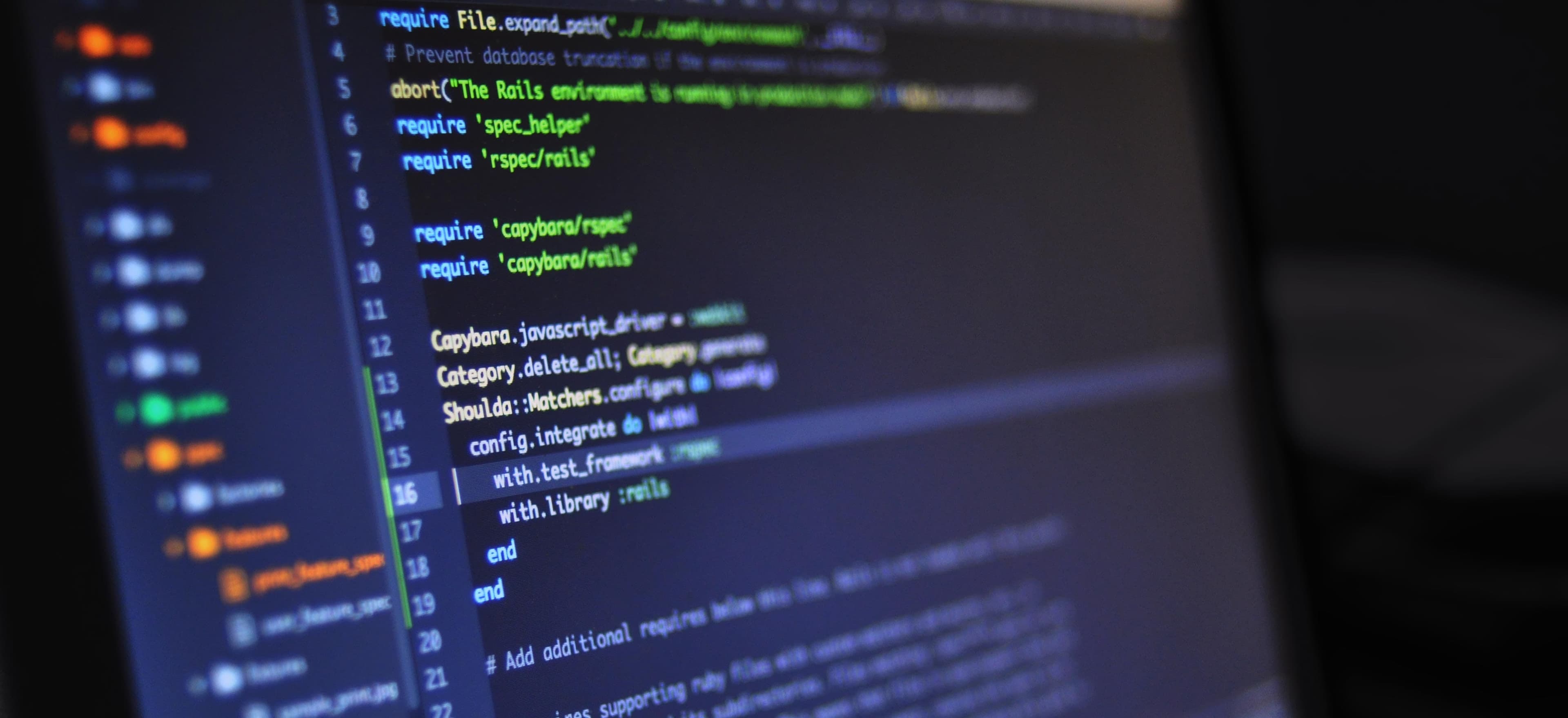
- Published on
Securing Your API Gateway with Robust Lambda Authentication
In today's interconnected world, the security of APIs (Application Programming Interfaces) is paramount. Whether you are building a microservices architecture or integrating third-party services, protecting your APIs from unauthorized access is vital to maintaining data integrity and user privacy. One effective approach in the realm of serverless applications is using AWS Lambda for authentication and authorization. In this post, we will explore how to secure your AWS API Gateway with robust Lambda authentication.
What is API Gateway?
Amazon API Gateway is a fully managed service that helps you create, publish, maintain, monitor, and secure APIs at any scale. It handles thousands of concurrent API calls and all the tasks associated with traffic management, authorization and access control, monitoring, and API version management.
Before diving deeper into securing our API, let us first understand the basic flow of API Gateway and AWS Lambda.
How API Gateway Works
- Client Request: The client makes a request to the API.
- API Gateway: The request hits the API Gateway, which serves as the entry point for clients.
- Lambda Function: If the request needs processing, it invokes the appropriate AWS Lambda function.
- Response: The function processes the input and returns a result back through the API Gateway to the client.
Why Use Lambda for Authentication?
Using AWS Lambda for authentication offers several advantages:
- Scalability: Serverless architecture allows your authentication logic to scale automatically with traffic.
- Cost-effectiveness: You are only charged for the compute time for your Lambda functions.
- Flexibility: You can implement custom authentication logic, including integrating with third-party identity providers.
- Ease of integration: Lambda works seamlessly with API Gateway, allowing you to create a unified solution.
Overview of Lambda Authentication with API Gateway
When securing an API, the typical pattern includes:
- Authorization: Verifying the identity of the user.
- Authentication: Confirming the user has permission to access a specific resource.
Lambda authorizers (formerly known as custom authorizers) are AWS Lambda functions that API Gateway calls to authorize requests before they reach your backend services.
Implementing Lambda Authentication
To implement Lambda authentication in API Gateway, you will follow these steps:
- Create a Lambda function.
- Set up the API Gateway to use the Lambda authorizer.
- Test the API to ensure it is secured properly.
Step 1: Create Your Lambda Function
Let's start by creating a basic Lambda function that will act as our authorizer.
exports.handler = async (event) => {
const token = event.authorizationToken; // Get the token from the event
const methodArn = event.methodArn; // Get the method ARN
// Example hardcoded token for demonstration
const validToken = 'your-secure-token';
// Check if the token is valid
if (token === validToken) {
console.log('User authorized');
return generatePolicy('user', 'Allow', methodArn);
} else {
console.log('User unauthorized');
return generatePolicy('user', 'Deny', methodArn);
}
};
// Helper function to generate IAM policy
const generatePolicy = (principalId, effect, resource) => {
const authResponse = {};
authResponse.principalId = principalId;
if (effect && resource) {
const policyDocument = {
Version: '2012-10-17',
Statement: [{
Action: 'execute-api:Invoke',
Effect: effect,
Resource: resource,
}],
};
authResponse.policyDocument = policyDocument;
}
return authResponse;
};
Explanation
Here’s a breakdown of the above code:
- Authorization Token: We get the token from the incoming request. In a real-world scenario, this would usually be a JWT (JSON Web Token).
- Token Validation: We compare the incoming token to a predefined valid token (for demonstration purposes). In production, you should validate against a secure source, such as a database or an identity provider.
- Generating IAM Policy: The
generatePolicy
function creates an IAM policy document to allow or deny access based on the token validation.
Step 2: Link Lambda Authorizer with API Gateway
Now that we have our Lambda function ready, we’ll link this function as an authorizer in the API Gateway.
- Go to your API Gateway console.
- Select your API and navigate to the Authorizers section.
- Create a new authorizer, and choose the Lambda function you created.
- Set the token source to the "Authorization" header.
Step 3: Testing Your Configuration
To test if your Lambda authorizer is properly securing your API Gateway, you can:
- Use Postman or curl to make requests.
- Send the request with the Authorization header set to your valid token.
- Verify that requests with an invalid token receive a 403 Forbidden response.
# Test with valid token
curl -X GET 'https://your-api-id.execute-api.region.amazonaws.com/stage/resource' -H 'Authorization: your-secure-token'
# Test with invalid token
curl -X GET 'https://your-api-id.execute-api.region.amazonaws.com/stage/resource' -H 'Authorization: invalid-token'
Best Practices for API Security
While the Lambda authorizer is a powerful tool for securing your API, keep the following best practices in mind:
- Use HTTPS: Always ensure your API Gateway is using HTTPS to encrypt data in transit.
- Implement Rate Limiting: To protect against DDoS attacks, implement throttling and rate limiting within API Gateway.
- Log Access and Errors: Enable CloudWatch logging to monitor access patterns and errors for better security insights.
- Regularly Rotate Secrets: If using tokens or API keys, regularly rotate them to minimize risk.
- Minimize Permissions: Follow the principle of least privilege when configuring IAM roles used by your API and Lambda functions.
The Bottom Line
Securing your API Gateway using AWS Lambda for authentication is a robust approach that leverages the serverless architecture's benefits. By implementing a custom Lambda authorizer, you gain flexibility and control over how your API is accessed, ensuring that only authorized users can interact with your services.
For more insights on securing APIs and utilizing AWS services effectively, consider exploring the AWS Security Best Practices documentation.
Final Thoughts
As developers, we must prioritize API security to safeguard user data and maintain the integrity of our applications. With tools like AWS API Gateway and Lambda, we can create scalable and secure authentication mechanisms that meet the demands of modern web applications.
Feel free to reach out in the comments with any questions or experiences you've had when securing your APIs! Happy coding!