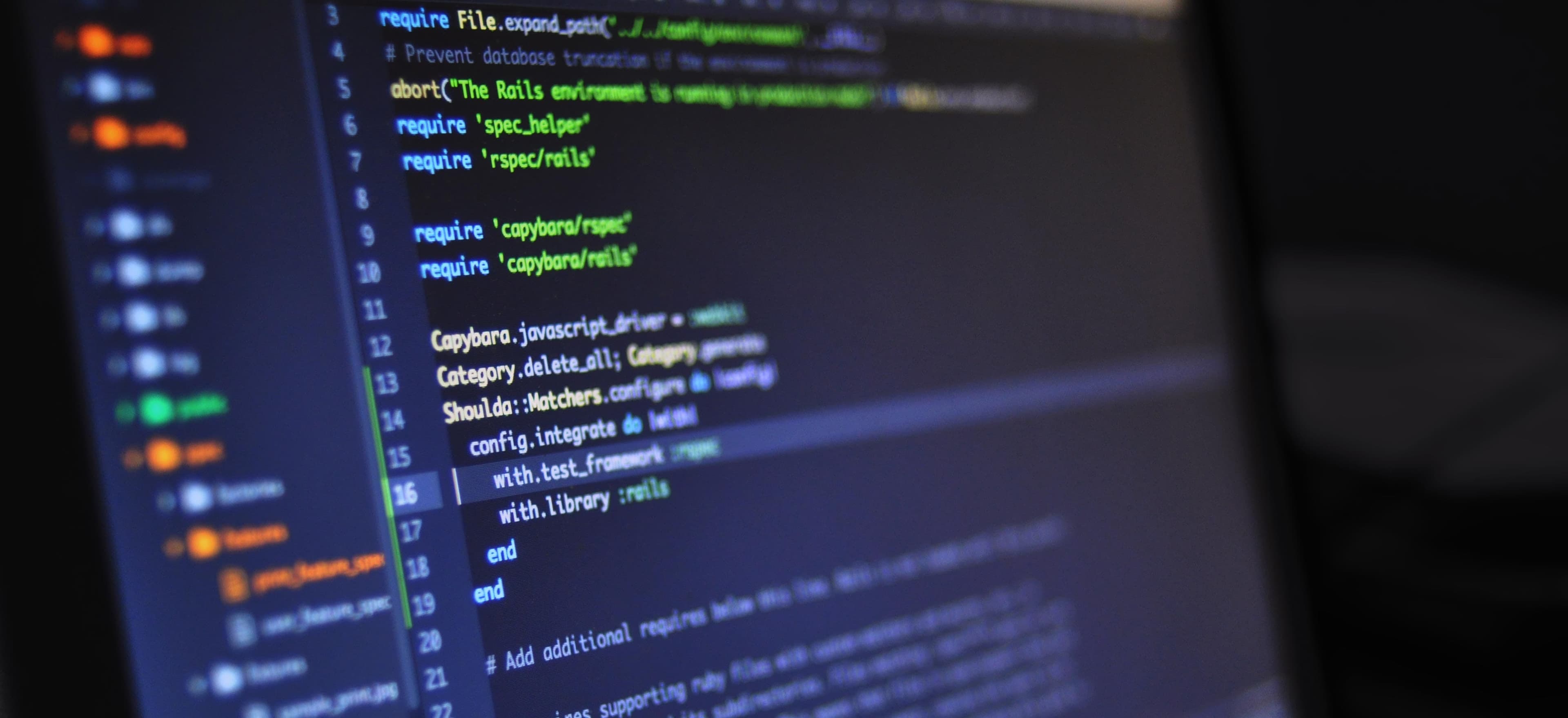
- Published on
Common Spring Security Misconfigurations to Avoid
Spring Security is a powerful and customizable authentication and access-control framework for Java applications. However, even seasoned developers can inadvertently misconfigure it, potentially leading to significant security vulnerabilities. In this post, we'll explore common misconfigurations you should avoid to ensure your Spring application remains secure.
1. Insecure Password Storage
Why It's Important
One of the most critical aspects of security is how passwords are stored. Storing plain text passwords is a glaring mistake and can lead to catastrophic breaches.
Correct Approach
Always use industry-standard hashing algorithms alongside a secure salt. Spring Security provides mechanisms to manage password storage securely.
Example
import org.springframework.security.crypto.bcrypt.BCryptPasswordEncoder;
public class PasswordUtil {
private static final BCryptPasswordEncoder passwordEncoder = new BCryptPasswordEncoder();
public static String hashPassword(String rawPassword) {
return passwordEncoder.encode(rawPassword);
}
public static boolean checkPassword(String rawPassword, String hashedPassword) {
return passwordEncoder.matches(rawPassword, hashedPassword);
}
}
In this example, BCryptPasswordEncoder
is used to hash passwords securely, ensuring that they are not stored in plain text. This is effective against rainbow table attacks, thanks to its salting mechanism.
Additional Reading
For more on password security practices, check out OWASP's Password Storage Cheat Sheet.
2. Allowing Open Redirects
Why It's Important
Open redirects can exploit user trust, sending users to malicious sites without their knowledge.
Correct Approach
Always validate URLs before redirecting. Use a whitelist to ensure that redirects only go to trusted locations.
Example
@RequestMapping("/redirect")
public String redirect(@RequestParam String url) {
if (isValidRedirect(url)) {
return "redirect:" + url;
}
return "redirect:/error"; // Fallback to a safe page
}
private boolean isValidRedirect(String url) {
List<String> safeUrls = Arrays.asList("http://safe-site.com", "http://another-safe-site.com");
return safeUrls.contains(url);
}
By validating against a predefined list of safe URLs, you mitigate the risk of unintentional redirects to harmful sites.
Additional Reading
To delve deeper into redirect vulnerabilities, refer to the OWASP Open Redirect page.
3. Weak CSRF Protection
Why It's Important
Cross-Site Request Forgery (CSRF) attacks trick users into submitting unwanted requests to a web application where they are authenticated.
Correct Approach
Spring Security helps shield your application from CSRF by default, but you must ensure it is not disabled, especially in forms and Ajax requests.
Example
@Override
protected void configure(HttpSecurity http) throws Exception {
http.csrf()
.csrfTokenRepository(CookieCsrfTokenRepository.withHttpOnlyFalse());
}
The use of CookieCsrfTokenRepository
creates CSRF tokens that can be sent through cookies, which can be easier to handle in client-side applications.
Additional Reading
Read more about CSRF protection on the Mozilla Developer Network.
4. Misconfigured CORS
Why It's Important
Cross-Origin Resource Sharing (CORS) allows restricted resources on a web page to be requested from another domain. Misconfiguring CORS can lead to unauthorized access to sensitive endpoints.
Correct Approach
Define specific origins instead of using wildcards, and ensure you only allow methods and headers that are necessary.
Example
@Override
protected void addCorsMappings(CorsRegistry registry) {
registry.addMapping("/api/**")
.allowedOrigins("https://your-safe-domain.com")
.allowedMethods("GET", "POST")
.allowedHeaders("Authorization", "Content-Type")
.allowCredentials(true);
}
This setup allows only specific domains to access your API, reducing exposure to potential attacks.
Additional Reading
For more information about CORS, see the MDN CORS documentation.
5. Forgetting to Secure HTTPS
Why It's Important
Using HTTP instead of HTTPS can expose users to man-in-the-middle attacks where sensitive data can be intercepted.
Correct Approach
Enforce HTTPS in your Spring Security configuration.
Example
@Override
protected void configure(HttpSecurity http) throws Exception {
http.requiresChannel()
.anyRequest()
.requiresSecure();
}
This code snippet forces HTTPS connections for all requests, ensuring that data in transit is encrypted.
Additional Reading
A detailed guide on HTTPS can be found in the Google Security Blog.
6. Overly Permissive Security Configurations
Why It's Important
Permissive security settings can leave your application vulnerable to attacks. If users are granted too many permissions, they may exploit unwanted pathways.
Correct Approach
Follow the principle of least privilege; only grant users the access they need.
Example
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/admin/**").hasRole("ADMIN")
.antMatchers("/user/**").hasAnyRole("USER", "ADMIN")
.antMatchers("/", "/home").permitAll()
.anyRequest().authenticated();
}
In this setup, only users with the ADMIN
role can access administrative endpoints, while less privileged users can only access user-specific routes.
Additional Reading
For a better understanding of user permissions, see the Spring Security Reference.
7. Not Using Method Security
Why It's Important
Method-level security complements URL-based security and can enforce business rules at a granular level.
Correct Approach
Use annotations such as @PreAuthorize
and @PostAuthorize
to control access based on the business logic.
Example
@PreAuthorize("hasRole('USER')")
public void viewUserProfile() {
// Logic to view user profile
}
By applying @PreAuthorize
, you can ensure that only users with the appropriate role can invoke this method, adding an extra security layer.
Additional Reading
Learn more about Spring's method security in the Spring Security Documentation.
Lessons Learned
Misconfigurations in Spring Security are not merely theoretical risks; they are gateways to real vulnerabilities. By adhering to secure practices and employing a proactive approach, you can mitigate these risks effectively.
Security is an ongoing process; staying updated with the latest security practices is essential. Follow best practices, heed the advice shared, and explore additional resources to create a resilient application environment.
If you want to learn more about Spring Security, I recommend checking the official Spring Security documentation for in-depth knowledge.
By understanding and avoiding these common pitfalls, you can empower your application with robust security and foster trust among your users.
Checkout our other articles