Converting Char to Byte in Java: Common Pitfalls Explained
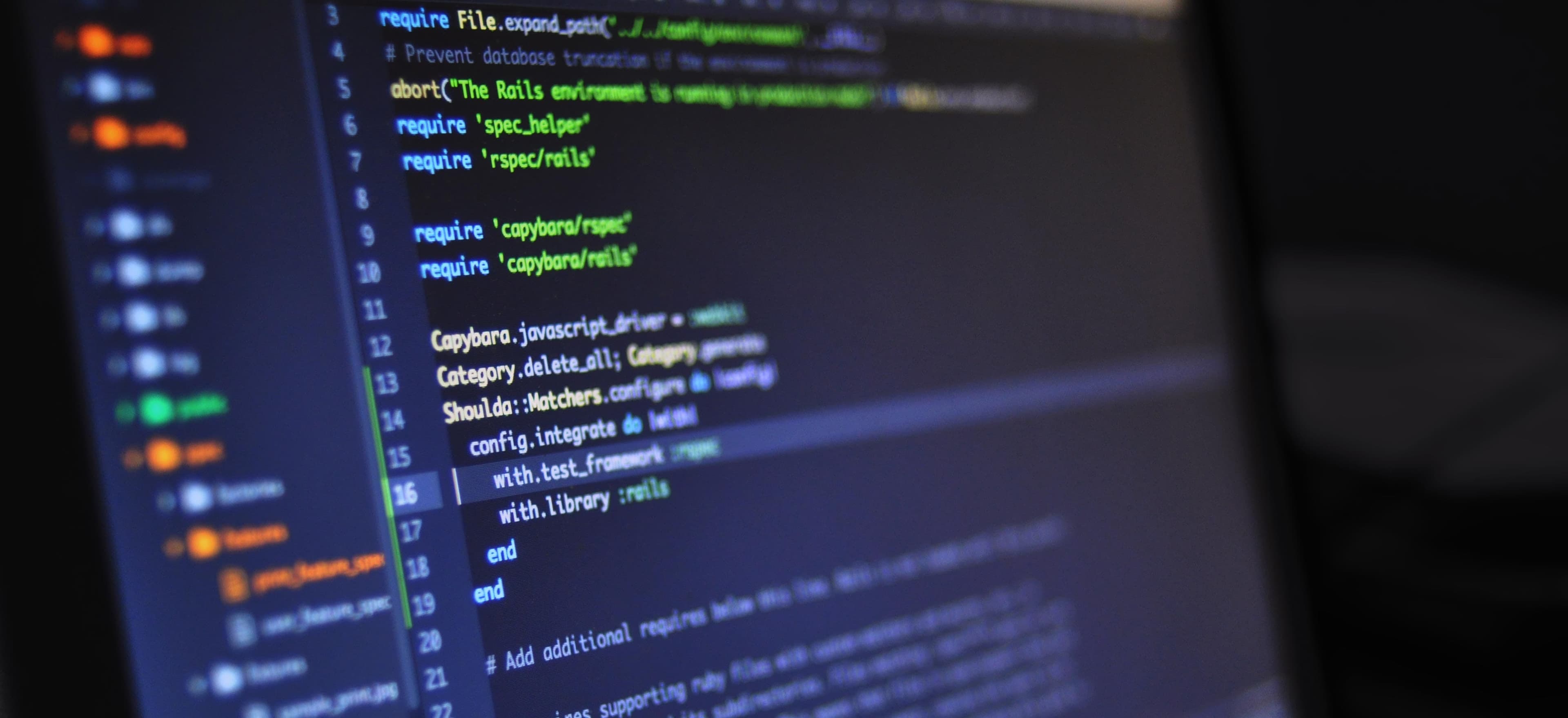
- Published on
Converting Char to Byte in Java: Common Pitfalls Explained
When working with Java, one of the frequent tasks is converting characters (char) to bytes. Char types in Java are 16-bit Unicode characters, while bytes are 8-bit values. This difference in size can lead to several common pitfalls that developers, especially beginners, may encounter. This blog post aims to clarify the nuances involved in this conversion and provide you with best practices while coding in Java.
Understanding the Basics of Char and Byte
Before diving into the conversion process, let's take a moment to clarify what char and byte actually are in Java.
-
Char: A data type in Java that represents a single 16-bit Unicode character. It can hold a value from 0 to 65,535 (0 to 2^16 - 1).
-
Byte: A Java data type that is an 8-bit signed integer. This data type ranges from -128 to 127 (or 0 to 255 when unsigned).
Given these definitions, it is essential to realize that not all char values can be smoothly converted to byte values without information loss. Let’s explore some of the common pitfalls encountered during this conversion.
Common Pitfalls When Converting Char to Byte
1. Character Overflow
One of the most obvious pitfalls is the risk of character overflow. As mentioned previously, a character can have a value between 0 and 65,535, while a byte can only hold values from -128 to 127. Attempting to convert char values outside this range will lead to unexpected results.
Example of Character Overflow:
char ch = 200; // This is valid
byte b = (byte) ch; // This will truncate the value
System.out.println(b); // Output: -56
Here, 200 does not fit within the byte's range. The byte representation could result in negative values due to overflow, demonstrating the unpredictable nature of direct casting.
2. Using Unicode Characters
Since Java employs Unicode to represent characters, some characters may not convert directly to a byte due to encoding issues. Consider characters that fall outside the standard ASCII range.
Example with Unicode:
char unicodeCh = 1000; // Unicode characters can exceed byte range
byte b = (byte) unicodeCh;
System.out.println(b); // Output: -112
In this example, Unicode character 1000 does not fit into the byte limits, leading to an unpredictable output again. Always be aware of the Unicode value you are dealing with when performing such conversions.
3. Loss of Data During Conversion
Converting from a char to a byte can result in a loss of data. This issue can lead to logic errors as the intended value is changed.
Example of Data Loss:
char originalChar = 'A';
byte convertedByte = (byte) originalChar;
System.out.println(convertedByte); // Output: 65
While this result appears fine, consider altering the character to a value such as '¥', which falls outside the byte range.
4. Implicit Casting
Implicit casting of char to byte lacks explicit conversion and can lead to confusion for developers who might expect the value to be preserved exactly.
Example of Implicit Casting Issue:
char ch = 'Z';
byte b = ch; // Compile-time error
Here, you will receive a compile-time error since Java requires an explicit cast to convert a larger type to a smaller one, preventing silent failures.
Best Practices for Char to Byte Conversion
Now that we've established the pitfalls let's turn our focus to practical techniques for converting char to byte safely and effectively.
1. Check the Value Range
Always verify that the char value lies within the acceptable byte range before conversion. This will prevent overflow and data loss.
char ch = 'B'; // Sample character
if (ch >= Byte.MIN_VALUE && ch <= Byte.MAX_VALUE) {
byte b = (byte) ch;
System.out.println("Converted byte: " + b);
} else {
System.out.println("Character out of byte range.");
}
2. Using Charset Encoding
If the goal is to encode characters properly into bytes, consider using Java's Charset
class from java.nio.charset
. This approach is particularly useful when dealing with multiple characters or strings.
Example Using Charset:
import java.nio.charset.StandardCharsets;
String str = "Hello";
byte[] byteArray = str.getBytes(StandardCharsets.UTF_8);
System.out.println(Arrays.toString(byteArray)); // Outputs: [72, 101, 108, 108, 111]
This example ensures that the conversion accurately reflects the byte representation according to the selected charset encoding, avoiding pitfalls typical of direct casting.
3. Use Warning Logs for Potential Issues
It may be useful to log warnings or exceptions when encountering situations where data may be lost. The presence of proper logging can help identify and manage conversion problems easily.
char ch = 'Ω'; // An example of character outside ASCII range
if (ch > 127) {
System.out.println("Warning: Character " + ch + " exceeds byte limit.");
}
This strategy allows developers to be proactive about data risks from conversions.
4. Testing with JUnit
When dealing with conversions as part of your application's codebase, write unit tests using JUnit. This practice will ensure that edge cases are handled and any conversion issues are captured during testing, reducing the chance of surprises later.
Wrapping Up
Converting char to byte in Java is not merely about casting a value. It requires an understanding of the limitations and characteristics of both data types. By addressing the common pitfalls discussed here - overflow, Unicode issues, data loss, and the complications of casting - developers can perform these conversions safely and effectively.
For more in-depth discussions about Java's data types, check out the official Java documentation.
By adhering to the best practices outlined in this post, you can convert chars to bytes with confidence and avoid costly mistakes in your applications. Always be cautious and deliberate about your data conversions, and your Java applications will be all the more robust for it. Happy coding!
Checkout our other articles