Enhancing JUnit Experiences with Annotations Made Easy
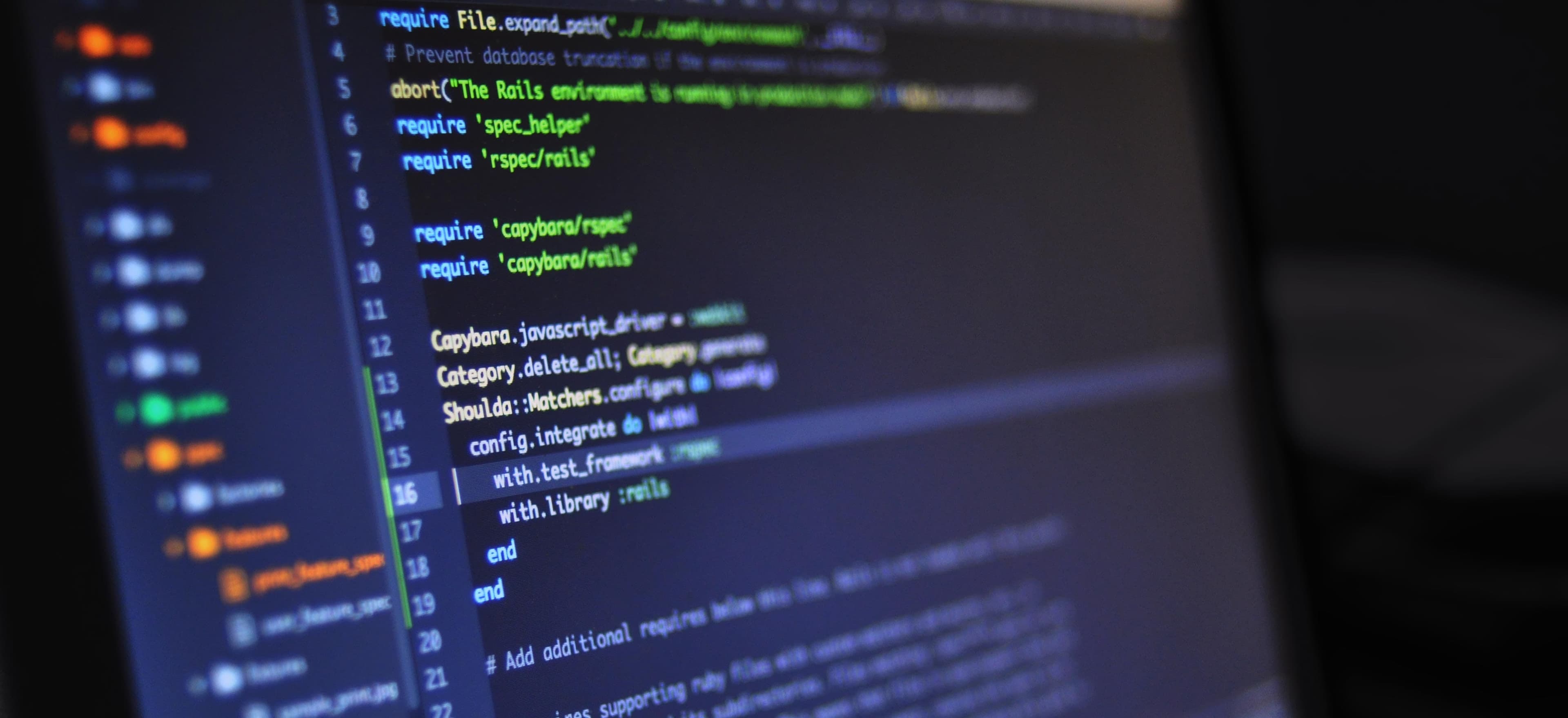
- Published on
Enhancing JUnit Experiences with Annotations Made Easy
JUnit is an essential tool in the Java development environment. It allows developers to create automated tests, ensuring that code behaves as intended. Although many developers are familiar with basic JUnit features, leveraging annotations can significantly enhance testing efficiency and overall experience. In this blog post, we will explore JUnit annotations, understand their purpose, and illustrate how they can improve your testing framework. Let’s dive in!
Understanding JUnit Annotations
JUnit annotations provide metadata to Java methods, signaling how the testing framework should execute them. These annotations help to clarify the purpose of tests, making your code cleaner and more maintainable.
Common JUnit Annotations
Here are some essential JUnit annotations that you should know:
@Test
@Before
@After
@BeforeClass
@AfterClass
@Ignore
1. @Test
The @Test
annotation is the foundation of any JUnit test. It identifies a method as a test case.
import org.junit.Test;
import static org.junit.Assert.assertTrue;
public class ExampleTest {
@Test
public void testAddition() {
int sum = 5 + 3;
assertTrue("5 + 3 should equal 8", sum == 8);
}
}
Why use @Test
?
Using @Test
clearly indicates which methods are intended for testing and allows JUnit to execute them automatically.
2. @Before and @After
The @Before
and @After
annotations are utilized to set up a testing environment and to clean up afterward. They execute before and after each test method respectively.
import org.junit.After;
import org.junit.Before;
import org.junit.Test;
public class DatabaseTest {
private DatabaseConnection connection;
@Before
public void setUp() {
connection = new DatabaseConnection();
connection.open();
}
@After
public void tearDown() {
connection.close();
}
@Test
public void testConnection() {
assertTrue(connection.isOpen());
}
}
Why use @Before
and @After
?
These annotations help minimize code duplication by allowing developers to set up and tear down resources for each test case without manually coding them in each method.
3. @BeforeClass and @AfterClass
In cases where you want to run methods only once before or after all tests, use @BeforeClass
and @AfterClass
.
import org.junit.AfterClass;
import org.junit.BeforeClass;
import org.junit.Test;
public class StaticResourceTest {
private static Resource resource;
@BeforeClass
public static void init() {
resource = new Resource();
resource.initialize();
}
@AfterClass
public static void cleanUp() {
resource.dispose();
}
@Test
public void testStaticResource() {
assertTrue(resource.isInitialized());
}
}
Why use @BeforeClass
and @AfterClass
?
These annotations allow you to allocate resources globally for the entire test class, improving performance by avoiding repetitive setup and teardown of resources.
4. @Ignore
Sometimes, certain test cases may need to be temporarily ignored. This is where @Ignore
comes in.
import org.junit.Ignore;
import org.junit.Test;
public class FeatureTest {
@Ignore("Pending implementation")
@Test
public void testPendingFeature() {
// Test code is not implemented yet
}
@Test
public void testCompletedFeature() {
assertTrue(true);
}
}
Why use @Ignore
?
Using @Ignore
provides clear documentation in your tests, signaling to other developers that the test is not ready for execution without deleting or commenting out the entire test method.
Creating Custom Annotations with JUnit
Although JUnit provides plenty of built-in annotations, creating custom annotations can further streamline testing processes tailored to your application needs.
Custom Annotations
Let’s create a simple custom annotation to log execution time for specific tests.
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
@Target(ElementType.METHOD)
@Retention(RetentionPolicy.RUNTIME)
public @interface TimedTest {
// Custom annotation for timing tests
}
After defining the annotation, we can use it alongside an Aspect or a JUnit Rule to measure execution time.
Using AspectJ for Logging
AspectJ allows us to implement cross-cutting concerns, like logging.
import org.aspectj.lang.ProceedingJoinPoint;
import org.aspectj.lang.annotation.Around;
import org.aspectj.lang.annotation.Aspect;
@Aspect
public class TimedAspect {
@Around("@annotation(TimedTest)")
public Object timeTestExecution(ProceedingJoinPoint joinPoint) throws Throwable {
long start = System.currentTimeMillis();
Object proceed = joinPoint.proceed();
long executionTime = System.currentTimeMillis() - start;
System.out.println(joinPoint.getSignature() + " executed in " + executionTime + "ms");
return proceed;
}
}
Why create a custom annotation?
Custom annotations enhance expressiveness and clarity in your codebase. In this example, a developer can quickly add timing functionality to a test case without cluttering the test method itself.
Why Embrace JUnit Annotations?
- Readability: Annotations clarify the intention of code, making it easily understandable.
- Maintainability: They reduce code duplication and complexity within test classes.
- Performance: Proper use of
@BeforeClass
and@AfterClass
can significantly improve test execution times. - Extensibility: Custom annotations empower developers to implement tailored behaviors as needed.
Best Practices When Using JUnit Annotations
- Keep Tests Isolated: Each test should be independent of others to maintain clean test runs.
- Avoid Overloading
@Before
/@After
: Keep setup and teardown code concise to prevent obscuring the test logic. - Use Descriptive Names: Ensure that your test methods convey their purpose clearly so that others can easily understand them.
Final Thoughts
Harnessing JUnit annotations as part of your testing framework can greatly enhance both your development experience and improve the quality of your tests. Through widespread use of annotations like @Test
, @Before
, @After
, and custom annotations, you can create a more organized and efficient testing environment.
For further reading on unit testing in Java, check out the JUnit 5 documentation or explore Effective Unit Testing.
Remember, effective unit testing is not just about writing tests but writing them in a way that enhances usability, readability, and maintainability. Happy testing!
Checkout our other articles