Preventing Resource Leaks: Key Debugging Techniques
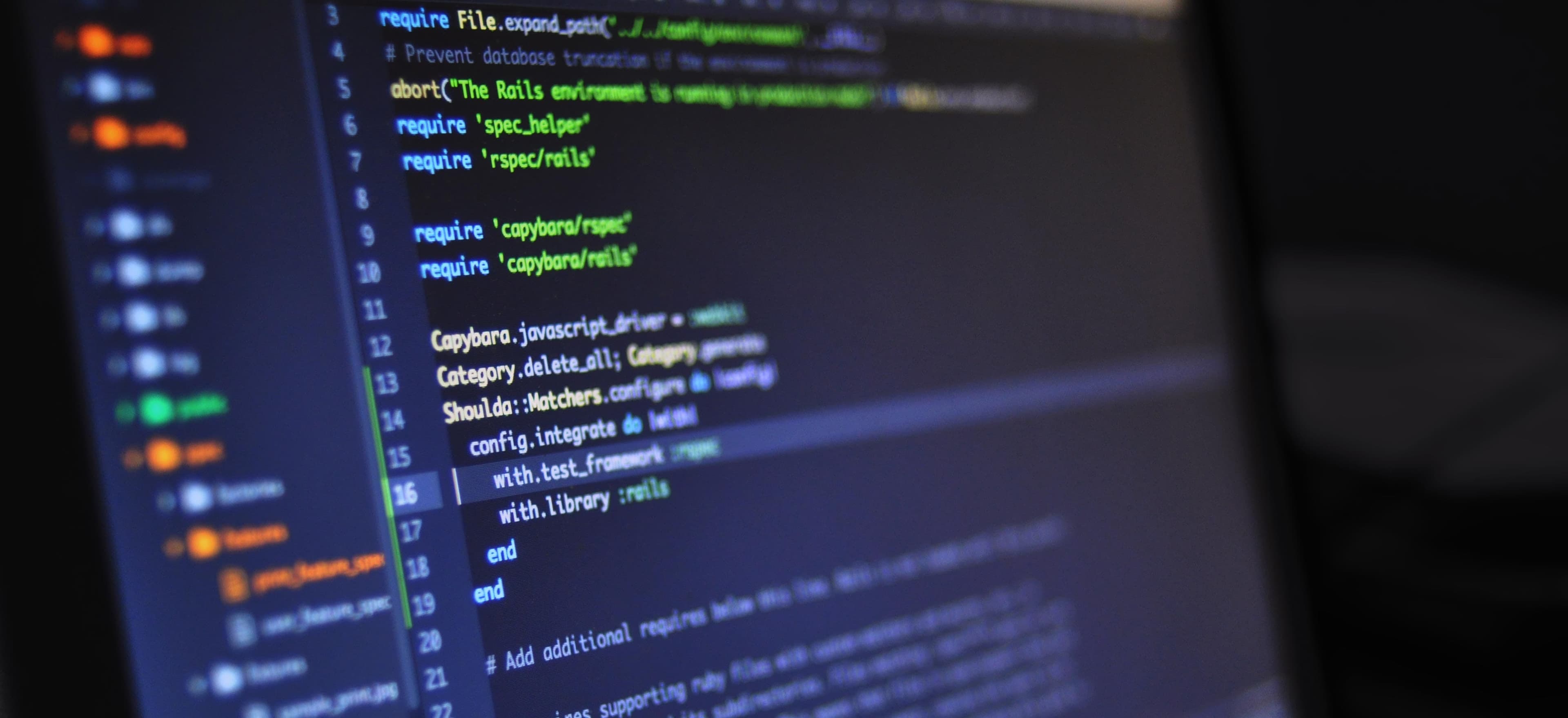
- Published on
Preventing Resource Leaks: Key Debugging Techniques in Java
Java is a powerful programming language that has become the backbone of many modern applications. However, even experienced developers can sometimes overlook one critical aspect: resource management. In particular, when resources such as memory, file handles, and network connections are not properly managed, they can lead to resource leaks. In this blog post, we will explore effective debugging techniques for preventing resource leaks in Java applications, enhancing performance and reliability.
What are Resource Leaks?
Resource leaks occur when an application fails to release resources it has acquired. This can manifest in various forms, such as:
- Memory leaks: Unreleased objects that occupy memory.
- File handle leaks: Open files that are not closed.
- Network connection leaks: Unused connections that remain open.
These leaks can lead to decreased performance, application crashes, and ultimately a poor user experience. Therefore, learning how to debug and prevent them is crucial for any Java developer.
Understanding Common Causes
Before diving into debugging techniques, it is essential to understand the common reasons behind resource leaks:
- Improper Resource Handling: Forgetting to close resources such as database connections and streams.
- Circular References: In situations where objects reference each other, memory cannot be released.
- Long-lived Objects: Keeping references to large objects in memory can prevent garbage collection.
Key Debugging Techniques
Now that we are familiar with resource leaks, let's discuss some key techniques for debugging and preventing them.
1. Utilizing Try-with-Resources Statement
One of the most effective ways to manage resources in Java is through the use of the try-with-resources statement, introduced in Java 7. This construct automatically closes resources when they are no longer needed.
Here is an example of how to use it:
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
public class FileReadExample {
public static void main(String[] args) {
String filePath = "example.txt";
try (BufferedReader br = new BufferedReader(new FileReader(filePath))) {
String line;
while ((line = br.readLine()) != null) {
System.out.println(line);
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
Why it Matters: The try-with-resources statement ensures that the BufferedReader
is closed automatically, even if exceptions occur. This reduces the chances of resource leaks in your code.
2. Using Java Profilers
Java profilers, such as VisualVM or YourKit, provide a real-time view of memory usage in Java applications. These advanced tools can help identify memory leaks by allowing developers to analyze memory heap dumps.
To get started with VisualVM:
- Download and install VisualVM.
- Start your Java application with the
-Dcom.sun.management.jmxremote
option. - Open VisualVM and connect to your application.
- Monitor the heap and perform memory analysis.
Why it Matters: Profilers help developers visualize and trace resource usage over time. They can pinpoint which objects are not being released, making it easier to identify potential leaks.
3. Employing Static Code Analysis Tools
Static code analysis tools such as SonarQube and FindBugs can detect potential resource leaks during the code review process. These tools analyze code for patterns that are known to cause resource leaks, such as unclosed streams or connections.
Here's how you can integrate FindBugs into your development workflow:
- Add FindBugs to your build system (Maven example):
<plugin>
<groupId>org.codehaus.mojo</groupId>
<artifactId>findbugs-maven-plugin</artifactId>
<version>3.0.5</version>
<executions>
<execution>
<goals>
<goal>check</goal>
</goals>
</execution>
</executions>
</plugin>
- Run the analysis by executing your Maven build.
Why it Matters: Static code analysis allows you to catch potential issues before they make it into production. Identifying and resolving these problems early can save time and reduce future debugging efforts.
4. Implementing Resource Tracking
While developing Java applications, creating a resource tracking utility can help manage and monitor resource usage. By implementing a simple tracking class, developers can log resource allocations and releases.
import java.util.HashSet;
import java.util.Set;
public class ResourceTracker<T> {
private Set<T> resources = new HashSet<>();
public void addResource(T resource) {
resources.add(resource);
}
public void removeResource(T resource) {
resources.remove(resource);
}
public void reportResources() {
System.out.println("Currently tracked resources: " + resources.size());
}
}
Why it Matters: This code snippet provides a way to keep track of resources that are added and removed. It allows for better visibility into the resource lifecycle and can help ensure that resources are always accounted for.
5. Regular Code Reviews
Regular code reviews are essential not only for improving code quality but also for spotting resource leaks that may have been overlooked by the original developer. During a code review session, focus on:
- Resource handling (e.g., looking for missing close statements).
- Identifying potential long-lived objects that should be refactored.
- Checking for circular references in data structures.
Why it Matters: Code reviews foster knowledge sharing among team members. They can expose areas of the code that require optimization or refactoring and help prevent resource leaks.
To Wrap Things Up
Preventing resource leaks is critical to producing high-quality, reliable Java applications. By utilizing debugging techniques such as try-with-resources, Java profilers, static code analysis tools, resource tracking, and regular code reviews, developers can effectively manage resources and enhance application performance.
Remember, preventing resource leaks not only improves performance but also enhances user satisfaction. For more resources on Java and preventing memory leaks, check out the following links:
- Java Memory Management
- Effective Java Programming
By incorporating these practices into your development workflow, you will significantly reduce the likelihood of resource leaks and set your Java applications up for success. Happy coding!
Checkout our other articles