Understanding Class Loader Issues in JDK with jcmd
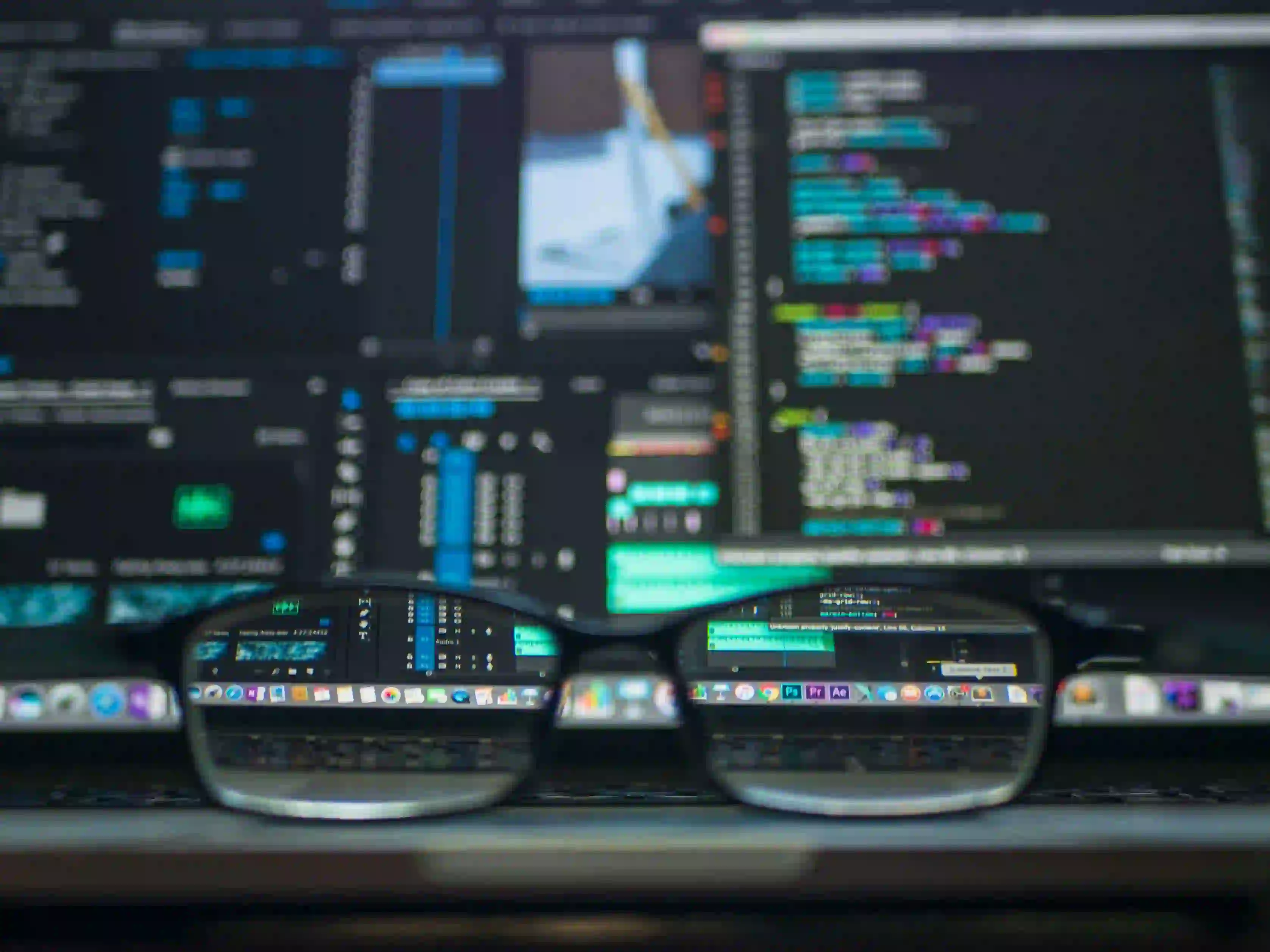
Understanding Class Loader Issues in JDK with jcmd
Java's class loading mechanism is a sophisticated component of the Java Virtual Machine (JVM). It plays a crucial role in how Java applications load classes at runtime. But with this complexity comes challenges, such as class loader issues, which can lead to undesirable behavior or performance in your applications. In this blog post, we will delve into class loader issues, how they arise, and how to use the Java Diagnostic Command (jcmd
) tool to diagnose and troubleshoot those problems.
What Is a Class Loader?
Before diving into issues, it's essential to understand what a class loader is. In Java, a class loader is responsible for loading class files into the JVM, where they are converted into runtime representations. Java’s class loading is hierarchical and consists of several built-in class loaders, namely:
- Bootstrap Class Loader: Loads core Java libraries located in the
jre/lib
directory. - Extension Class Loader: Loads classes from the JDK’s extension directory,
jre/lib/ext
. - Application Class Loader: Loads classes from the application classpath, which includes user-defined classes and libraries.
The interesting aspect of the class loader hierarchy is the delegating mechanism: when a class loader receives a request to load a class, it first delegates the request to its parent loader before attempting to load the class itself. This ensures that classes are only loaded once and helps prevent class versioning issues.
Why Do Class Loader Issues Occur?
Class loader issues typically arise due to the following reasons:
- Classpath Conflicts: If the same class definition is present in multiple JAR files, it can lead to conflicts. The class loaded first can overshadow others.
- Class Visibility Issues: Classes loaded by different class loaders are considered different even if they have the same name and content. This can lead to
ClassCastException
. - Class Loader Memory Leaks: Improperly managed references to classes can prevent class loaders from being garbage collected, leading to memory issues.
Detecting Class Loader Issues Using jcmd
The jcmd
tool is part of the JDK that provides diagnostic capabilities. It allows you to send commands to a running JVM process for monitoring, debugging, and profiling.
To get started with jcmd
, you'll first need to identify the process ID (PID) of your Java application. You can achieve this through the following command:
jps -l
This will list all Java processes along with their PIDs. Take note of the one you're interested in.
Getting Class Loader Information
Once you have the PID, you can get detailed class loader information using the jcmd
command:
jcmd <pid> VM.class_loader
This command will provide you insights into the various class loaders in use, including their names and the classes they have loaded.
Example of Using jcmd to Identify Class Loader Issues
Consider an example where you suspect class loader issues in your application. Here's how you can investigate further using jcmd
.
-
Run the Command: Start by executing the command mentioned above.
🔧snippet.shjcmd <pid> VM.class_loader
-
Analyze the Output: The output will include class loader details. Look for duplicate classes that might be causing conflicts or any unusual class loaders that appear not to match your application design.
-
Use the
jcmd
GC.heap_dump
Command: If you suspect a memory leak related to class loaders, use the following command to create a heap dump.🔧snippet.shjcmd <pid> GC.heap_dump <file_path>
After generating the heap dump, you can analyze it using tools like Eclipse Memory Analyzer (MAT) to check for class loader leaks.
Code Snippet: Demonstrating Class Loader Conflict
To illustrate a simple class loader conflict scenario, let's assume we have two JARs with the same class name.
Step 1 - Create Two JARs
Let's create two simple JAR files, libA.jar
and libB.jar
, both containing a class called MyClass
.
libA/MyClass.java:
package libA;
public class MyClass {
public void display() {
System.out.println("Hello from libA MyClass");
}
}
libB/MyClass.java:
package libB;
public class MyClass {
public void display() {
System.out.println("Hello from libB MyClass");
}
}
Compile and package them into JAR files:
javac libA/MyClass.java -d libA
jar -cvf libA.jar -C libA/ .
javac libB/MyClass.java -d libB
jar -cvf libB.jar -C libB/ .
Step 2 - Create the Main Class
Now, let's create a main application that loads both JAR files.
import libA.MyClass;
import libB.MyClass as MySecondClass; // Renaming to avoid conflict
public class Main {
public static void main(String[] args) {
MyClass classA = new MyClass();
classA.display();
MySecondClass classB = new MySecondClass();
classB.display();
}
}
Incorporating this snippet into your application will demonstrate how multiple class loaders can load classes with the same name without conflict as long as you manually manage dependencies.
Step 3 - Running the Application with Classpath Issues
When running your application, include both JARs:
java -cp "libA.jar:libB.jar:." Main
You may encounter unexpected behavior or exceptions, highlighting class loading conflicts.
Closing Remarks
Class loading is an integral part of the Java ecosystem, but its complexity can lead to various issues. Understanding how to leverage tools like jcmd
allows developers to diagnose and troubleshoot class loader-related problems effectively.
For more details on learning about Java performance tuning and diagnostics, you can check out Java Performance Tuning and Understanding Java Classloaders.
By mastering these tools and concepts, you can ensure smoother deployments and a more robust Java application architecture. Happy coding!