Troubleshooting Common Issues with Camel File Transfers
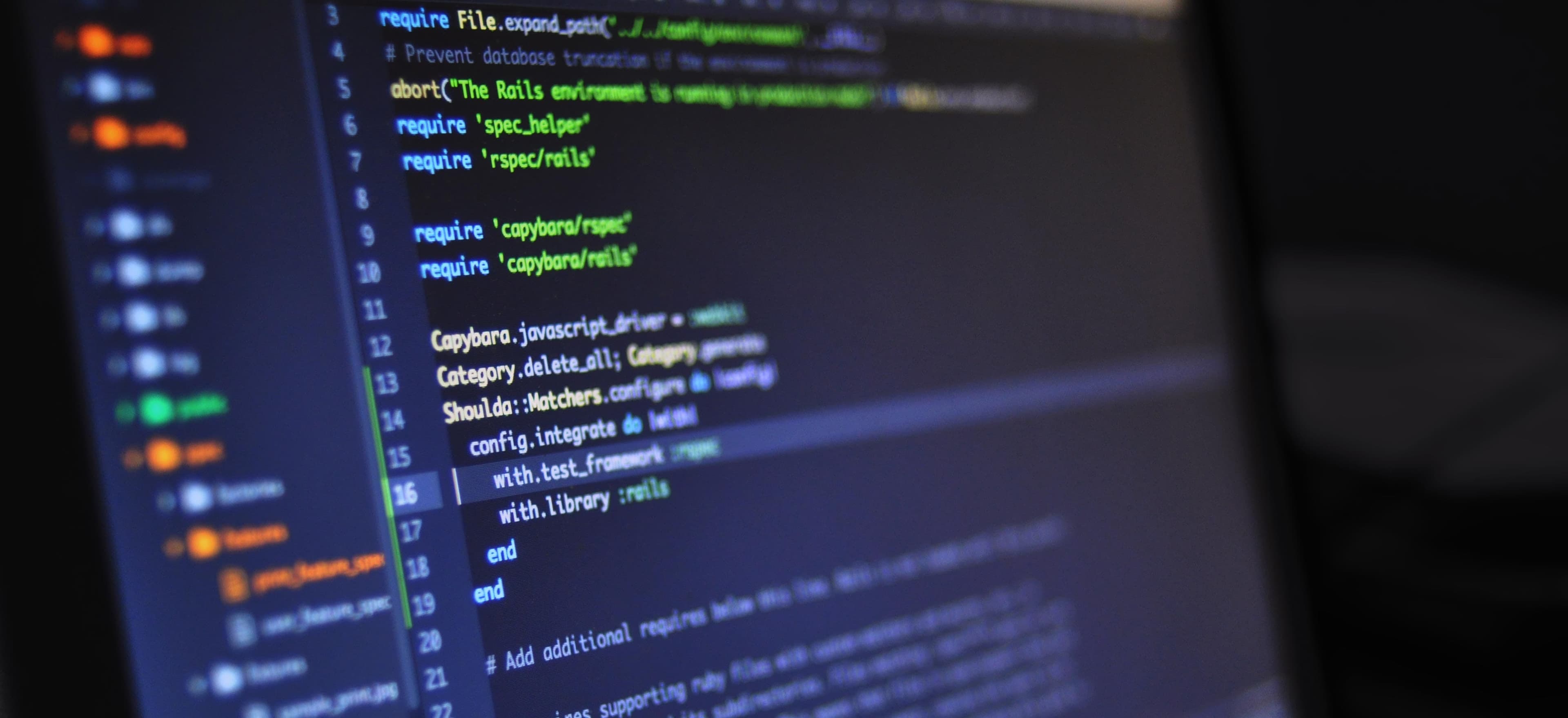
- Published on
Troubleshooting Common Issues with Camel File Transfers
Apache Camel is an open-source integration framework that greatly simplifies the process of integrating various systems and applications. One of the commonly used components in Camel is the file component, which allows for reading from and writing to files within a given directory. While it provides great functionality, users might encounter various issues during file transfers. This guide delves into the most common problems and how to troubleshoot them effectively.
Understanding Apache Camel File Component
Before diving into troubleshooting, it is crucial to have a working knowledge of how the Camel file component functions. The file component can manage file manipulations asynchronously, allowing for efficient monitoring of files at specified directories.
Here’s how you set up a basic file producer in Camel:
from("direct:start")
.to("file:outputDirectory");
In this example, Camel listens to the direct:start
endpoint and transfers incoming messages directly to an output directory.
Key Features of the File Component:
- Directory Monitoring: Camel continuously monitors a specified directory for any new files or changes.
- Automatic File Deletion: Once transfer completes, files can be deleted to prevent reprocessing.
- Polling Strategy: Configurable strategies determine the frequency of polling.
With the fundamental principles established, let's move into common issues faced while working with the Camel file component.
1. Directory Not Found
Issue Description:
One of the most frequent problems users encounter is the "Directory Not Found" error. This typically happens when the path specified in the to()
method does not exist or is mistyped.
Troubleshooting Steps:
- Check Path Spelling: Ensure that the directory name is correctly spelled in the code.
- Correct Path: Verify that the directory you are routing to actually exists on the filesystem.
- Permissions: Ensure that the application has the necessary permissions to write to the target directory.
Code Example:
from("direct:start")
.to("file:///nonexistentDirectory");
In the above example, Camel will fail if nonexistentDirectory
does not exist. Fix this by correcting the directory path.
2. Insufficient Permissions
Issue Description:
If the Camel application doesn’t have the right permissions to access the file system, it will lead to transfer failures.
Troubleshooting Steps:
- Permission Checking: Check the read and write permissions of the directories involved.
- Run As Administrator: For local development, running the application with elevated privileges might resolve permission issues.
- Use ROOT Paths: In cloud environments or Docker containers, ensure container/VM permissions align with the file system structure.
Code Example:
from("file:inputDirectory?delete=true")
.to("file:outputDirectory");
In this case, ensure that both inputDirectory
and outputDirectory
are accessible and writable.
3. Encoding Issues
Issue Description:
When dealing with text files, encoding mismatches can lead to data being read incorrectly, resulting in corrupted file outputs.
Troubleshooting Steps:
- Specify Encoding: Use the
charset
parameter in the file endpoint configuration to ensure the correct encoding is used. - Check Input Files: Ensure that the original files are encoded correctly to avoid misinterpretation during transfers.
Code Example:
from("file:inputDirectory?charset=UTF-8")
.to("file:outputDirectory");
Specifying the charset
parameter ensures that files are read in the correct character encoding.
4. File Locking Issues
Issue Description:
When accessing files that are locked by another process, Camel can fail to read or write to them.
Troubleshooting Steps:
- Identify Locking Processes: Use tools (like
lsof
on Linux or Process Explorer on Windows) to determine which process is locking the file. - Retry Mechanism: Implement a retry mechanism that periodically attempts to access the file until it is released.
Code Example:
from("file:inputDirectory?noop=true&retryAttemptCount=5")
.to("file:outputDirectory");
The noop=true
option allows Camel to leave the original file unchanged, while the retryAttemptCount
permits multiple access retries.
Additional Resources
5. Message Processing Failures
Issue Description:
Sometimes messages may not be processed as expected due to transformations or failure in downstream endpoints.
Troubleshooting Steps:
- Enable Logging: Turn on Camel logging for deeper insight into what happens during the transfer.
- Validation: Validate messages before processing to ensure all required fields are present and correct.
- Handling Exceptions: Use error handling mechanisms such as onException to better manage exceptions and implement fallback strategies.
Code Example:
onException(Exception.class)
.log("An error occurred: ${exception.message}")
.handled(true);
from("file:inputDirectory")
.process(exchange -> {
// your processing logic
})
.to("file:outputDirectory");
The above error handling mechanism will log any exceptions, helping track failures during file processing.
My Closing Thoughts on the Matter
Troubleshooting file transfer issues in Apache Camel may seem daunting, but with a methodical approach, you can resolve most common problems efficiently. By focusing on identifying the root causes—be it directory path issues, permissions, encoding, file locks, or message processing—you can ensure smoother operation of your file integrations.
Each of the code snippets and troubleshooting steps provided above can be adapted for your specific use case. Evaluating and refining your configurations based on these insights will pave the way for successful Camel file transfers.
For more detailed information, consider exploring the Apache Camel documentation or engaging with the community through forums to get real-time advice on unique issues you may encounter.
Utilize these strategies, and tackle file transfer issues with confidence!