Resolving Thread Safety Issues in Java Concurrency
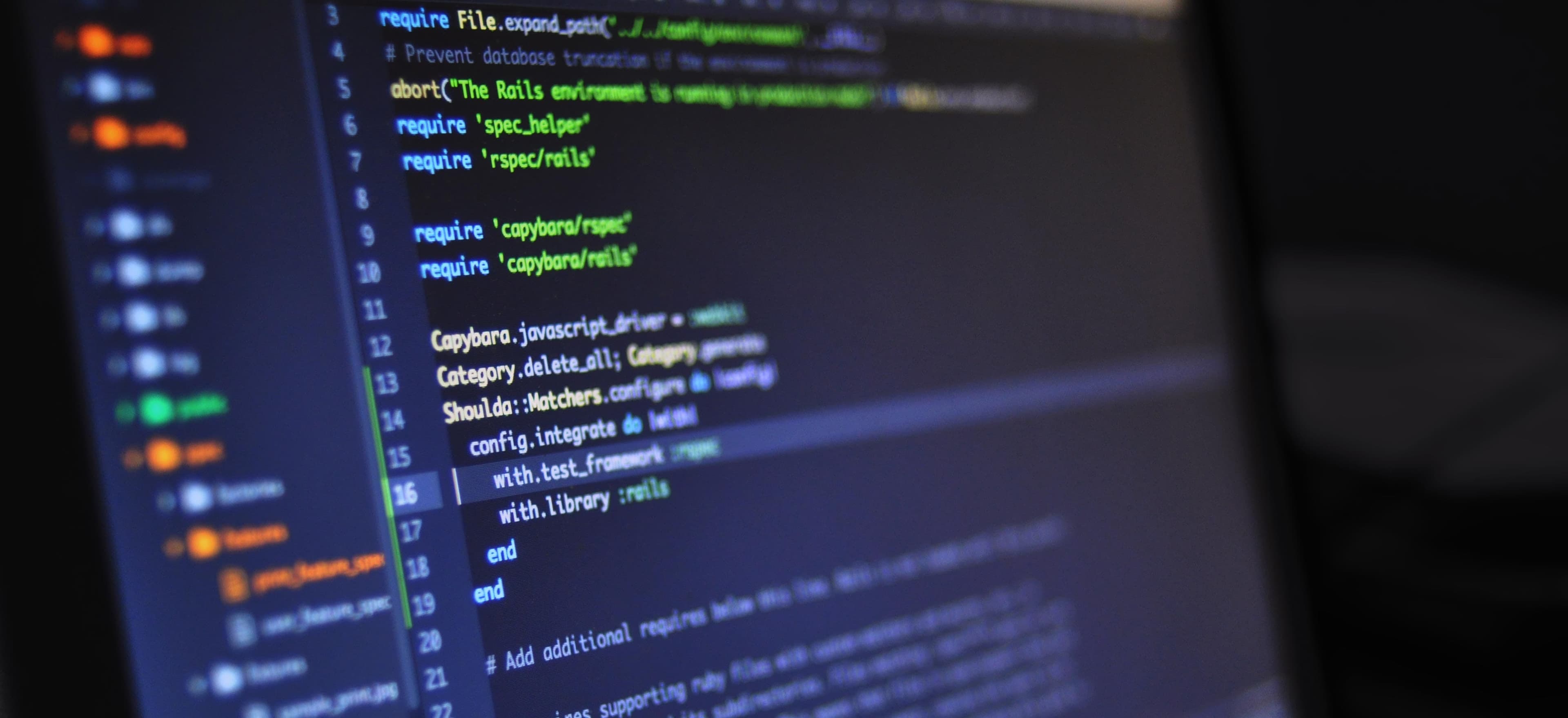
- Published on
Resolving Thread Safety Issues in Java Concurrency
Concurrency in Java is a powerful feature that allows multiple threads to execute simultaneously, ensuring efficient utilization of processor resources and improved application performance. However, it comes with its own set of challenges, particularly when it comes to thread safety. In this blog post, we will delve into thread safety issues in Java concurrency and explore practical strategies for resolving them.
Understanding Thread Safety
At its core, thread safety means that a class or a piece of code functions correctly when accessed by multiple threads. When a data structure or some shared state is not thread-safe, it can lead to unpredictable behavior, corrupted state, or even crashes.
Why is Thread Safety Important?
- Data Integrity: When multiple threads interact with shared data, ensuring the correctness of that data is paramount.
- Application Stability: Unhandled concurrency issues can cause apps to behave unpredictably, leading to potential downtime.
- User Experience: Applications that hang or crash under load can frustrate users and lead to loss of trust.
Common Thread Safety Issues
1. Race Conditions
A race condition occurs when two or more threads access shared data and attempt to change it at the same time. The outcome depends on the unpredictable timing of how the threads are scheduled.
class Counter {
private int count = 0;
public void increment() {
count++;
}
public int getCount() {
return count;
}
}
In the above Counter
class, if two threads call increment()
simultaneously, they might read the same value of count
before either updates it, leading to lost increments.
2. Deadlocks
A deadlock happens when two or more threads are each waiting for the other to release a resource, resulting in a total halt of execution.
class Resource {
public synchronized void resourceOne(Resource resource) {
resource.resourceTwo();
}
public synchronized void resourceTwo() {
// Method logic
}
}
In this example, if two threads each hold one resource and attempt to call a method on the other, they will both be stuck waiting, causing a deadlock.
3. Livelocks
Livelocks are similar to deadlocks but involve threads actively changing states in response to each other. They are still stuck in a cycle where no progress can be made.
Strategies to Resolve Thread Safety Issues
1. Use Synchronized Blocks
The simplest way to ensure thread safety is to use the synchronized
keyword. This prevents multiple threads from executing a block of code simultaneously.
class SafeCounter {
private int count = 0;
public synchronized void increment() {
count++;
}
public synchronized int getCount() {
return count;
}
}
In this SafeCounter
class, synchronized
ensures that only one thread can execute the increment()
or getCount()
method at a time. However, be cautious about overusing synchronization, as it can lead to performance bottlenecks.
2. Use Locks
Java provides high-level concurrency utilities like ReentrantLock
, which offer more flexibility than synchronized
methods.
import java.util.concurrent.locks.ReentrantLock;
class LockCounter {
private final ReentrantLock lock = new ReentrantLock();
private int count = 0;
public void increment() {
lock.lock(); // Acquire the lock
try {
count++;
} finally {
lock.unlock(); // Always release the lock
}
}
public int getCount() {
return count;
}
}
In this example, ReentrantLock
allows the thread to try to acquire the lock and ensures that it is always released in a finally
block—this is critical to avoid deadlocks.
3. Atomic Variables
Java provides a set of atomic classes—like AtomicInteger
, AtomicBoolean
, etc.—in the java.util.concurrent.atomic
package. These classes use low-level atomic operations, offering efficient thread-safe operations without the heavy overhead of synchronization.
import java.util.concurrent.atomic.AtomicInteger;
class AtomicCounter {
private AtomicInteger count = new AtomicInteger(0);
public void increment() {
count.incrementAndGet(); // Atomically increments by 1
}
public int getCount() {
return count.get();
}
}
In this example, AtomicInteger
provides a built-in thread-safe method for incrementing the count without explicit locking.
4. Immutable Objects
Creating immutable objects can be a powerful strategy for avoiding thread safety issues since immutable objects do not change state after their creation.
final class ImmutablePoint {
private final int x;
private final int y;
public ImmutablePoint(int x, int y) {
this.x = x;
this.y = y;
}
public int getX() {
return x;
}
public int getY() {
return y;
}
}
In this ImmutablePoint
class, once an instance is created, its state cannot be altered, making it inherently thread-safe.
5. Using Concurrent Collections
Java offers a suite of concurrent collections (like ConcurrentHashMap
, CopyOnWriteArrayList
, etc.) that simplify handling concurrency without the need for explicit synchronization.
import java.util.concurrent.ConcurrentHashMap;
class UserStore {
private ConcurrentHashMap<String, String> userMap = new ConcurrentHashMap<>();
public void addUser(String id, String name) {
userMap.put(id, name);
}
public String getUser(String id) {
return userMap.get(id);
}
}
Here, ConcurrentHashMap
allows concurrent access without locking the entire map, improving performance in multi-threaded scenarios.
Best Practices for Achieving Thread Safety
- Minimize Shared State: Reduce shared variables as much as possible by using local thread data or employing thread-local storage.
- Favor Immutability: Use immutable objects for shared data whenever possible.
- Use High-Level Concurrency Utilities: Leverage existing concurrency classes from the Java Concurrency framework.
- Monitor and Test: Thoroughly test multi-threaded behavior using tools like JUnit and concurrency testing libraries to uncover hidden issues.
Lessons Learned
Thread safety in Java concurrency is a broad and complex topic. Understanding common problems like race conditions, deadlocks, and livelocks is crucial for developing reliable multi-threaded applications. Implementing strategies such as synchronized blocks, locks, atomic variables, immutability, and concurrent collections can help mitigate these issues. By adhering to best practices and employing existing Java concurrency utilities, developers can create efficient, reliable, and thread-safe Java applications.
For more extensive reading on Java concurrency, consider checking out Java Concurrency in Practice and The Java Tutorials on Concurrency.
By prioritizing thread safety, you prepare your applications for the complexities of concurrent execution, ensuring they remain robust and responsive even under high loads.
Checkout our other articles