Common Spring Boot Setup Errors in IntelliJ Community Edition
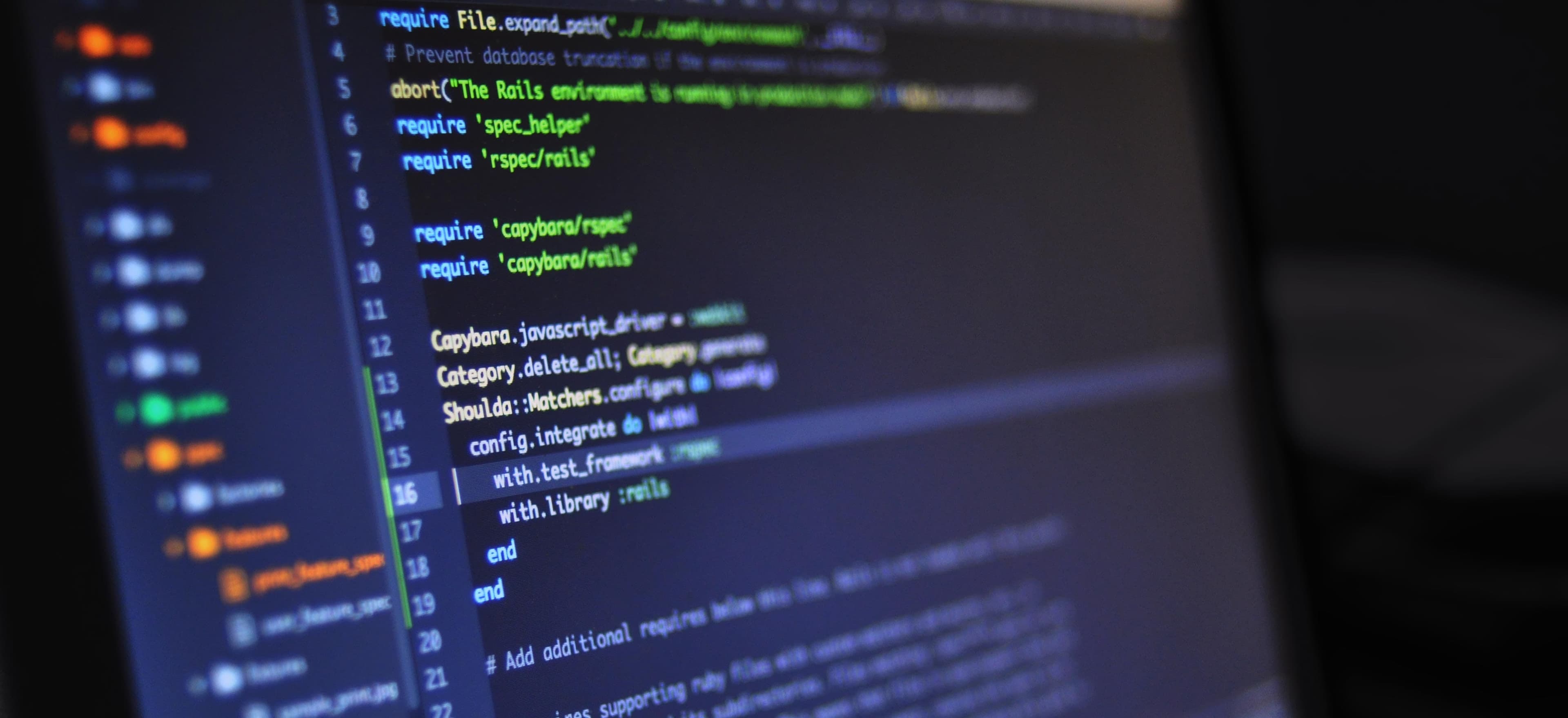
- Published on
Common Spring Boot Setup Errors in IntelliJ Community Edition
Spring Boot has revolutionized Java development, allowing developers to create stand-alone, production-grade applications quickly. However, when setting up a Spring Boot project in IntelliJ Community Edition, you may encounter several common errors. This blog post will explore these errors, providing solutions and best practices so you can hit the ground running with your Spring Boot application.
1. Incorrect Artifacts Configuration
One of the most frequent problems developers face is the improper configuration of the artifact in the IntelliJ project settings.
Symptoms
You may see error messages related to missing dependencies or issues with the project structure.
Solution
- Right-click on your project in the Project Explorer window.
- Select
Open Module Settings
or simply pressF4
. - Navigate to the
Artifacts
section. - Make sure that you have a proper JAR or WAR artifact configured that specifies your main class, resources, and libraries.
Example Code Snippet
When configuring your pom.xml
, ensure you have Spring Boot dependencies correctly set up:
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-devtools</artifactId>
<scope>runtime</scope>
<optional>true</optional>
</dependency>
<!-- Add your other dependencies here -->
</dependencies>
By ensuring your pom.xml
is correctly structured, you prevent unresolved dependency issues.
2. Java Version Compatibility
Java version mismatches are another common error. Spring Boot has specific requirements for the Java version being used.
Symptoms
Compilation errors or runtime exceptions can occur if you use a Java version that is incompatible with your Spring Boot version.
Solution
Verify your Java version with the following command:
java -version
Make sure that your pom.xml
specifies a compatible version of Java, such as:
<properties>
<java.version>17</java.version>
</properties>
You should also configure IntelliJ to use the correct SDK:
- Go to
File
>Project Structure
. - Select
Project
. - Ensure the Project SDK matches the Java version you intend to use.
3. Maven Wrapper Issues
Many developers struggle with Maven setup, especially concerning the Maven wrapper files.
Symptoms
When you try to execute commands, you might receive a message indicating the Maven wrapper doesn't work properly.
Solution
If your project doesn't have a Maven Wrapper (mvnw
file), you can generate it using:
mvn -N io.takari:maven:wrapper
This creates the files you need. Now, ensure you execute Maven commands via the wrapper using:
./mvnw clean install
4. Dependency Resolution Issues
IntelliJ sometimes struggles with dependency resolution, particularly in Community Edition without additional plugins.
Symptoms
You may experience build errors indicating that certain classes or packages cannot be found.
Solution
Try the following approaches:
-
Invalidate Caches:
- Go to
File
>Invalidate Caches / Restart
. - This action can solve various caching issues that sometimes obstruct IntelliJ's ability to recognize updated dependencies.
- Go to
-
Update Your Dependencies: Make sure that all your dependencies are resolved properly. Use:
mvn clean install
-
Check Your
pom.xml
: Ensure your dependencies are well-defined and do not conflict.
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
5. Spring Boot Configuration Errors
Configuration properties in Spring Boot can cause applications to fail if not set correctly.
Symptoms
You’ll see exceptions indicating that Spring could not start due to misconfigured properties.
Solution
Double-check your application properties or YAML file:
For application.properties
:
spring.datasource.url=jdbc:mysql://localhost:3306/yourdatabase
spring.datasource.username=root
spring.datasource.password=yourpassword
spring.jpa.hibernate.ddl-auto=update
This file exposes essential configurations that can affect the underlying behavior of your Spring Boot application.
6. Embedded Container Issues
Spring Boot supports embedded servers, such as Tomcat or Jetty, but sometimes, issues arise during startup.
Symptoms
You may encounter stack traces when trying to access your application in a browser.
Solution
Make sure that you have set up the dependencies required for embedded containers. Additionally, check your main
function:
@SpringBootApplication
public class MySpringBootApplication {
public static void main(String[] args) {
SpringApplication.run(MySpringBootApplication.class, args);
}
}
Ensure your main method is correctly annotated. A wrong entry point can lead to initialization errors.
7. Spring Boot DevTools Not Working
If you are using Spring Boot DevTools but still experience application reload delays, you might not have it configured correctly.
Symptoms
Changes to your application code do not trigger a reload.
Solution
Add the spring-boot-devtools
dependency:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-devtools</artifactId>
<scope>runtime</scope>
</dependency>
Make sure that your IDE is set to compile your code before running. In IntelliJ:
- Go to
Settings
>Build, Execution, Deployment
>Compiler
. - Check the option
Build project automatically
.
Closing the Chapter
Setting up a Spring Boot project can sometimes lead to frustrating errors, especially in IntelliJ Community Edition. However, with the guidance provided in this article, you should be better equipped to tackle these common issues. Through careful configuration, proper dependency management, and diligent checking of your project structure, you can lay a solid foundation for developing Spring Boot applications.
For additional support, refer to the official Spring Boot documentation and IntelliJ's JetBrains documentation.
Happy coding!
Checkout our other articles