Mastering Java 8 Predicates for Efficient Data Filtering
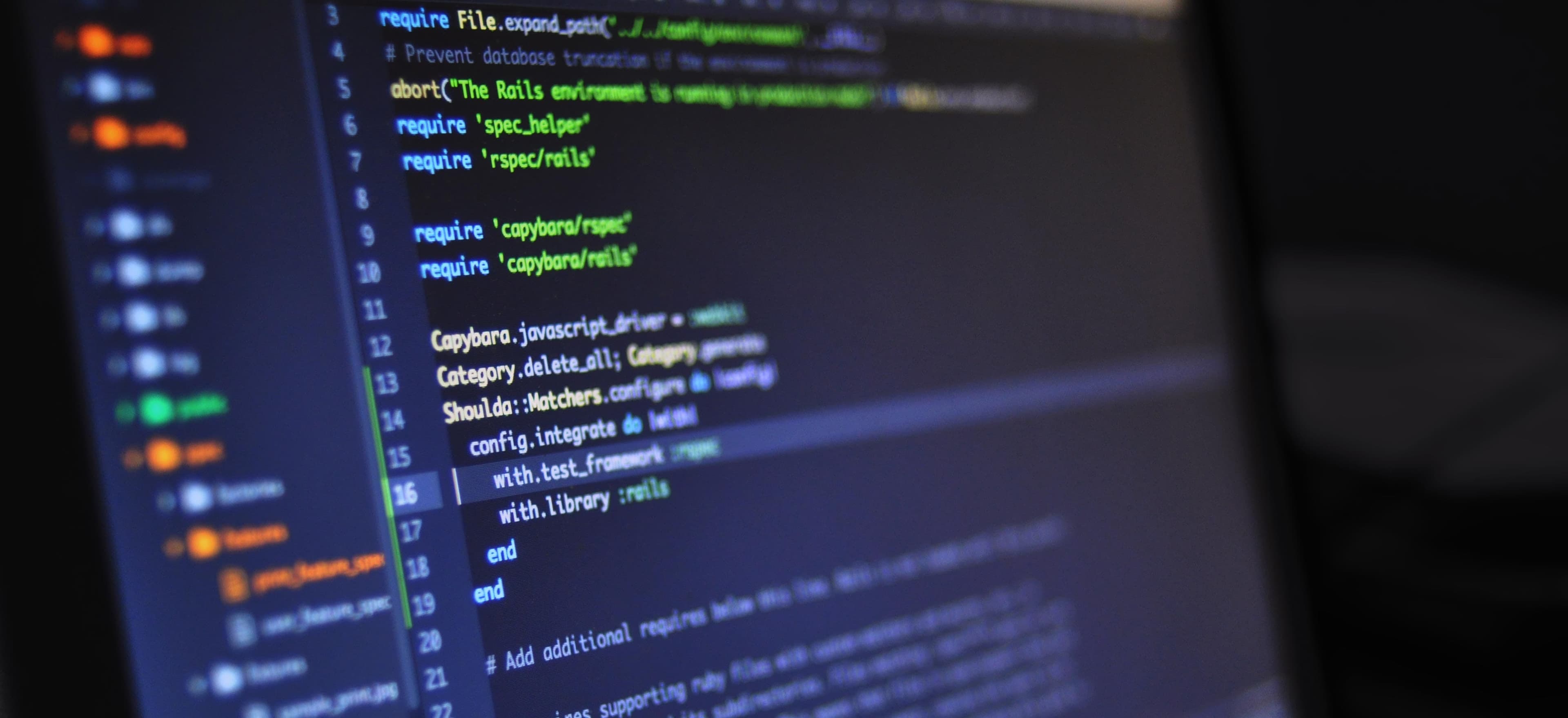
- Published on
Mastering Java 8 Predicates for Efficient Data Filtering
Java 8 introduced powerful tools to improve the way we work with collections and data. Among these tools are Predicates, which allow developers to write cleaner and more efficient code for data filtering. In this blog post, we'll dive deep into what predicates are, how to leverage them effectively, and provide practical examples showcasing their capabilities.
What is a Predicate in Java?
In Java, a Predicate is a functional interface defined in the java.util.function
package. It represents a boolean-valued function of one argument. In simpler terms, a Predicate evaluates a condition and returns true
or false
.
The signature of a Predicate looks like this:
@FunctionalInterface
public interface Predicate<T> {
boolean test(T t);
}
This concise design promotes rich functionality for filtering data and can be easily utilized in conjunction with Java Streams.
Why Use Predicates?
Predicates provide several advantages:
- Cleaner Code: Simplifies conditions without repetitive boilerplate code.
- Reduced Complexity: Allows more natural expression of conditions.
- Reusable Components: Easily passed around in code; can combine multiple predicates for complex conditions.
- Integration with Streams: Streams are made powerful when combined with predicates.
Example of a Basic Predicate
Let's look at a simple example for clarity.
import java.util.function.Predicate;
public class PredicateExample {
public static void main(String[] args) {
Predicate<Integer> isEven = num -> num % 2 == 0;
System.out.println(isEven.test(4)); // true
System.out.println(isEven.test(5)); // false
}
}
In this code snippet, we define a predicate called isEven
, which checks if a number is even. We then use the test
method to evaluate numbers.
Working with Collections and Streams
The real game-changer comes when you apply predicates in conjunction with Java Streams. Lets explore filtering collections using predicates.
Filtering a List with Predicates
Suppose you have a list of names, and you want to filter out the names that start with the letter "A".
import java.util.Arrays;
import java.util.List;
import java.util.function.Predicate;
import java.util.stream.Collectors;
public class FilterNames {
public static void main(String[] args) {
List<String> names = Arrays.asList("Alice", "Bob", "Adam", "Charlie", "Amanda");
Predicate<String> startsWithA = name -> name.startsWith("A");
List<String> filteredNames = names.stream()
.filter(startsWithA)
.collect(Collectors.toList());
System.out.println(filteredNames); // [Alice, Adam, Amanda]
}
}
In this example, we create a list of names and filter them using the startsWithA
predicate. This results in a simple and expressive filtering mechanism.
Combining Predicates
Predicates can be combined using the and
, or
, and negate
methods to create complex conditions without facing code bloat.
Example of Combining Predicates
Let’s create a more robust set of filtering criteria. We’ll filter numbers based on whether they are even and greater than 10.
import java.util.Arrays;
import java.util.List;
import java.util.function.Predicate;
import java.util.stream.Collectors;
public class CombinePredicates {
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(7, 12, 3, 6, 20, 15, 18);
Predicate<Integer> isEven = num -> num % 2 == 0;
Predicate<Integer> isGreaterThanTen = num -> num > 10;
// Combine both predicates using AND
List<Integer> filteredNumbers = numbers.stream()
.filter(isEven.and(isGreaterThanTen))
.collect(Collectors.toList());
System.out.println(filteredNumbers); // [12, 20, 18]
}
}
Here, we combine two predicates: isEven
and isGreaterThanTen
. The resulting filteredNumbers
list contains only the numbers that meet both criteria.
Using Predicate Negation
In some cases, you may want to filter out values that meet certain criteria. The negate
method can easily handle this:
import java.util.Arrays;
import java.util.List;
import java.util.function.Predicate;
import java.util.stream.Collectors;
public class NegatePredicate {
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(7, 12, 3, 6, 20, 15, 18);
Predicate<Integer> isEven = num -> num % 2 == 0;
List<Integer> oddNumbers = numbers.stream()
.filter(isEven.negate())
.collect(Collectors.toList());
System.out.println(oddNumbers); // [7, 3, 15]
}
}
In this snippet, isEven.negate()
filters for all odd numbers.
Practical Use Cases
Now that we’ve discussed the foundation of predicates, let’s take a look into a practical use case.
Filtering Users Based on Criteria
Imagine you are developing a user management system. You might have a list of users, and you want to filter them based on several attributes, such as age and status.
import java.util.Arrays;
import java.util.List;
import java.util.function.Predicate;
import java.util.stream.Collectors;
class User {
String name;
int age;
boolean active;
User(String name, int age, boolean active) {
this.name = name;
this.age = age;
this.active = active;
}
public String toString() {
return name + " (Age: " + age + ", Active: " + active + ")";
}
}
public class UserFilter {
public static void main(String[] args) {
List<User> users = Arrays.asList(
new User("Alex", 25, true),
new User("Bob", 30, false),
new User("Charlie", 20, true),
new User("David", 35, false),
new User("Eve", 22, true)
);
Predicate<User> isActive = user -> user.active;
Predicate<User> isAdult = user -> user.age >= 21;
List<User> filteredUsers = users.stream()
.filter(isActive.and(isAdult))
.collect(Collectors.toList());
filteredUsers.forEach(System.out::println);
}
}
In this example, we filter users to obtain a list of active adults. This approach not only reduces the complexity of our queries but also keeps the code business-relevant.
My Closing Thoughts on the Matter
Java 8 Predicates have revolutionized data filtering with Collections through their concise style and rich functionality. They allow for clean, expressive, and powerful code. Using predicates, you can create reusable conditions, combine them, and leverage their power with Java Streams effectively.
For further reading, check out these resources:
- Java 8 documentation on java.util.function.
- A deeper dive into Java Streams.
As you continue exploring Java, consider incorporating predicates into your data processing routines for more efficient and maintainable solutions. Happy coding!
Checkout our other articles