Mastering Mockito: Delaying Stubbed Method Responses
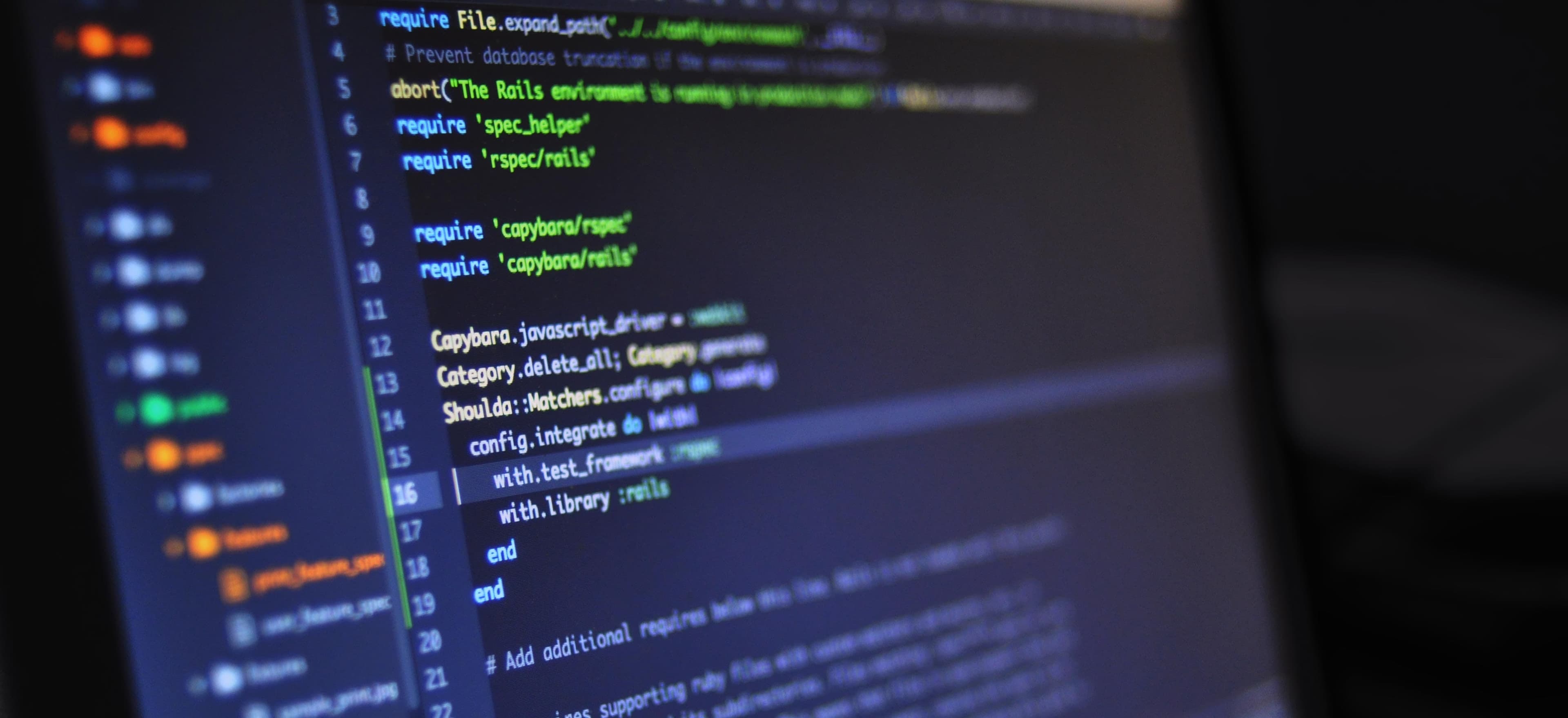
- Published on
Mastering Mockito: Delaying Stubbed Method Responses
When working on Java unit tests, Mockito has become a go-to library for many developers. It provides various functionalities for mocking objects, verifying behaviors, and simplifying test-driven development. One interesting aspect of Mockito is its ability to delay stubbed method responses. Understanding how to implement delayed responses can help create more robust and realistic tests.
In this blog post, we will explore the concept of delaying stubbed method responses using Mockito, provide code snippets, and discuss various scenarios where this feature might be helpful. Let’s dive in!
Why Delay Method Responses?
In some cases, you may want to simulate conditions that occur in real-life applications. For example:
- Testing Async Operations: In applications that deal with asynchronous processes, delays might occur due to network calls, I/O operations, etc. By simulating these delays, you can ensure that your code handles them correctly.
- Timeout Scenarios: If your application requires handling timeouts, simulating them through method delays can help ensure that your application behaves correctly under those conditions.
Setting Up Mockito
Before we dive into examples, let's ensure you have Mockito set up in your project. Add the following dependency to your pom.xml
if you're using Maven:
<dependency>
<groupId>org.mockito</groupId>
<artifactId>mockito-core</artifactId>
<version>4.0.0</version> <!-- Check for the latest version -->
<scope>test</scope>
</dependency>
If you're using Gradle, include this in your build.gradle
:
testImplementation 'org.mockito:mockito-core:4.0.0' // Check for the latest version
Delaying Stubbed Responses in Mockito
Mockito provides several ways to simulate delays in your tests. You can use the thenAnswer()
method, which allows you to create a custom stub that can execute code when the method is called. Here’s how to do it:
Example Code Snippet
Let’s say we have a service that simulates fetching user data. We want to delay the response to test how our application manages the waiting period.
import static org.mockito.Mockito.*;
import java.util.concurrent.TimeUnit;
public interface UserService {
User fetchUserData(int userId);
}
public class User {
private int id;
private String name;
// Constructor and Getters/Setters
}
// In your test class
@Test
public void testFetchUserDataWithDelay() {
// Arrange
UserService userService = mock(UserService.class);
// Simulate a delay of 2 seconds
when(userService.fetchUserData(anyInt())).thenAnswer(invocation -> {
TimeUnit.SECONDS.sleep(2); // Delay for 2 seconds
return new User(1, "John Doe");
});
long startTime = System.currentTimeMillis();
// Act
User user = userService.fetchUserData(1);
long duration = System.currentTimeMillis() - startTime;
// Assert
assertNotNull(user);
assertEquals("John Doe", user.getName());
assertTrue(duration >= 2000); // Duration should be at least 2000 ms
}
Explanation of the Code
- Mocking the Service: We create a mock of
UserService
, which allows us to control its behavior in tests. - Setting up the Delay: By using the
thenAnswer()
method, we introduce a 2-second sleep when thefetchUserData
method is called. This simulates the delay. - Counting the Duration: We measure the time taken for the method call to verify that our delay behaves as expected.
- Assertions: We check if the returned User object is valid and whether the duration meets our delay expectations.
Limiting the Scope of Delays
It’s important to control the scope of your delays. You typically wouldn't want to delay every interaction within your tests. Hence, use delays sparingly and only when needed, primarily for simulating specific scenarios or handling potential edge cases.
Practical Applications of Delayed Responses
- Simulating High-latency Networks: If your application queries an external API, you can mimic a slow network to ensure your application handles it gracefully.
- Thread Handling: For applications that involve concurrency (like multi-threaded execution), delays can help determine if your code can handle race conditions effectively.
- User Experience Testing: Introducing artificial delays helps emulate real-world usage conditions, improving your application's resilience to slow responses.
Caution Relying on Delays
While introducing delays can be useful, it’s crucial to maintain the focus of your tests. Mocked delays can lead to:
- Increased Test Times: If overused, delays can bloat your testing time, leading to frustration for developers.
- Mocking Over Real Implementation: Ensure you keep a balance; real-life tests alongside mocked ones give the best results in terms of accuracy and reliability.
Closing the Chapter
Delaying stubbed method responses in Mockito is a powerful technique for creating robust and realistic tests. It allows developers not just to test the correctness of the code against expected values but also to assess how their application behaves in various conditions.
By leveraging Mockito’s capabilities, you can create more reliable unit tests, ensuring your application is well-equipped to handle real-world scenarios.
For more detailed information on Mockito, check out the official documentation. There, you will find extensive resources to enhance your testing skills and perfect your testing strategies.
Happy Testing!