Boosting Database Stream Performance with Java GraalVM
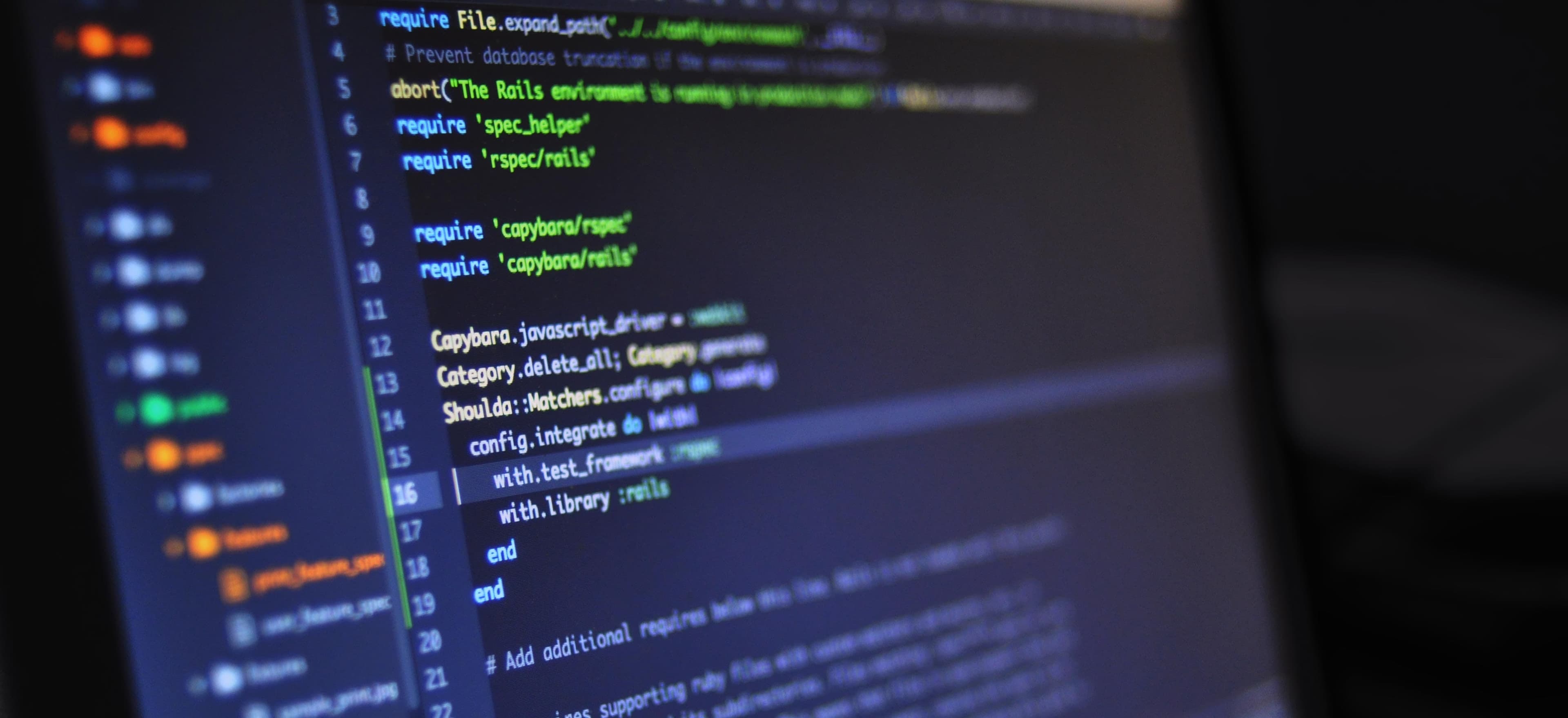
- Published on
Boosting Database Stream Performance with Java GraalVM
In today's data-driven world, the ability to efficiently manage and process large streams of data has become critical. Java, with its robust ecosystem, provides numerous tools and frameworks to accomplish this. However, what if you could heighten your Java application's performance multifold? Enter GraalVM, an innovative high-performance runtime that can compile Java applications to native images, providing faster execution and lower memory consumption. This post explores how to leverage GraalVM to boost database stream performance in Java applications.
What is GraalVM?
GraalVM is an advanced polyglot virtual machine that allows you to run Java applications alongside those written in other languages like JavaScript, Python, Ruby, and R. The standout feature of GraalVM is its ability to compile programs ahead of time into native executables. This reduces startup time and improves runtime performance, making it particularly beneficial for microservices or serverless endpoints that require high efficiency.
Why is Database Stream Performance Important?
The need for efficient database stream processing arises from numerous domains like e-commerce, finance, social media, and IoT systems. As data inflow increases, database bottlenecks can severely hamper application performance. This is where using GraalVM can make a significant difference.
Getting Started with GraalVM
To utilize GraalVM, you must first install it on your machine. You can download it from the official GraalVM website. Once installed, you can choose your IDE or Java editor, but ensure you also configure your environment variables to point to the GraalVM installation.
For example on Unix-like systems, configure your environment as follows:
export JAVA_HOME=/path/to/graalvm
export PATH=$JAVA_HOME/bin:$PATH
For Windows users, adjust JAVA_HOME
accordingly through System Properties.
Setting Up a Database and Streaming Framework
For our examples, we will establish a simple Java application that streams data from a mock database using JDBC and Java Streams. Let's set up a mock database.
Dependencies
First, you'll need the following dependencies in your pom.xml
if you are using Maven:
<dependency>
<groupId>org.postgresql</groupId>
<artifactId>postgresql</artifactId>
<version>42.2.20</version>
</dependency>
This will allow us to connect and interact with a PostgreSQL database.
Connecting to PostgreSQL
Let’s create a simple method to connect to our PostgreSQL database.
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class Database {
private static final String URL = "jdbc:postgresql://localhost/testdb";
private static final String USER = "user";
private static final String PASSWORD = "password";
public static Connection connect() {
Connection conn = null;
try {
conn = DriverManager.getConnection(URL, USER, PASSWORD);
System.out.println("Connected to the database");
} catch (SQLException e) {
System.err.println("Connection failed: " + e.getMessage());
}
return conn;
}
}
Streaming Data with Java Streams
Now that we have the connection established, let’s write code to stream data from a table.
This example assumes we have a table called users
with at least the columns id
, name
, and email
.
import java.sql.Connection;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
import java.util.stream.Stream;
import java.util.stream.StreamSupport;
public class DatabaseStream {
public static Stream<String> streamUsers(Connection connection) {
try {
Statement statement = connection.createStatement();
String query = "SELECT id, name, email FROM users";
ResultSet resultSet = statement.executeQuery(query);
return StreamSupport.stream(new ResultSetIterable(resultSet).spliterator(), false)
.map(row -> row.getString("name"));
} catch (SQLException e) {
throw new RuntimeException("Error fetching users", e);
}
}
}
In this example, we utilize a custom ResultSetIterable
class that you will need to implement to convert ResultSet
into an iterable format.
Custom Iterable for ResultSet
Here’s how you can implement this; it’s crucial for streaming from JDBC.
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.Iterator;
import java.util.NoSuchElementException;
public class ResultSetIterable implements Iterable<ResultSet> {
private final ResultSet resultSet;
public ResultSetIterable(ResultSet resultSet) {
this.resultSet = resultSet;
}
@Override
public Iterator<ResultSet> iterator() {
return new Iterator<ResultSet>() {
@Override
public boolean hasNext() {
try {
return !resultSet.isLast();
} catch (SQLException e) {
throw new RuntimeException("Error checking for next result", e);
}
}
@Override
public ResultSet next() {
try {
if (!hasNext()) throw new NoSuchElementException();
resultSet.next();
return resultSet;
} catch (SQLException e) {
throw new RuntimeException("Error fetching next result", e);
}
}
};
}
}
Performance Considerations
The most significant performance gain comes from GraalVM’s ability to optimize your code. By compiling your application into a native image, you can realize the potential of below-average database response times and memory overheads.
To compile your Java project with GraalVM, you can use the native-image
command:
native-image --no-fallback -cp target/your-app-1.0-SNAPSHOT.jar com.example.Main
Key Advantages:
- Reduced Startup Time: Native images can start in the order of milliseconds.
- Lower Memory Footprint: Avoids the overhead of JVM features not required for your application.
- Improved Execution Speed: GraalVM includes advanced optimizations that can significantly improve your code's performance.
Example: Putting It All Together
Here’s how you can run our program.
public class Main {
public static void main(String[] args) {
Connection connection = Database.connect();
DatabaseStream.streamUsers(connection)
.forEach(System.out::println);
}
}
Now compile the application into a native image, and run it. The program should list all user names from the users
table promptly, exhibiting the efficiency and prowess of GraalVM in enhancing database stream performance.
Final Thoughts
Incorporating GraalVM into your Java projects can enhance database streaming performance exceptionally. By leveraging GraalVM's unique features, developers can achieve faster execution times and reduced memory consumption—promising a seamless experience for applications dealing with large volumes of data.
For additional resources and further reading, check out the official GraalVM documentation and explore more about optimizing your Java applications.
As you start leveraging GraalVM, remember that performance tuning and optimization is an ongoing process. Stay updated with the latest developments, sharing knowledge with peers, and explore community-driven solutions.
Happy coding!
Checkout our other articles