Understanding Static Context: Calling getClass() Issues
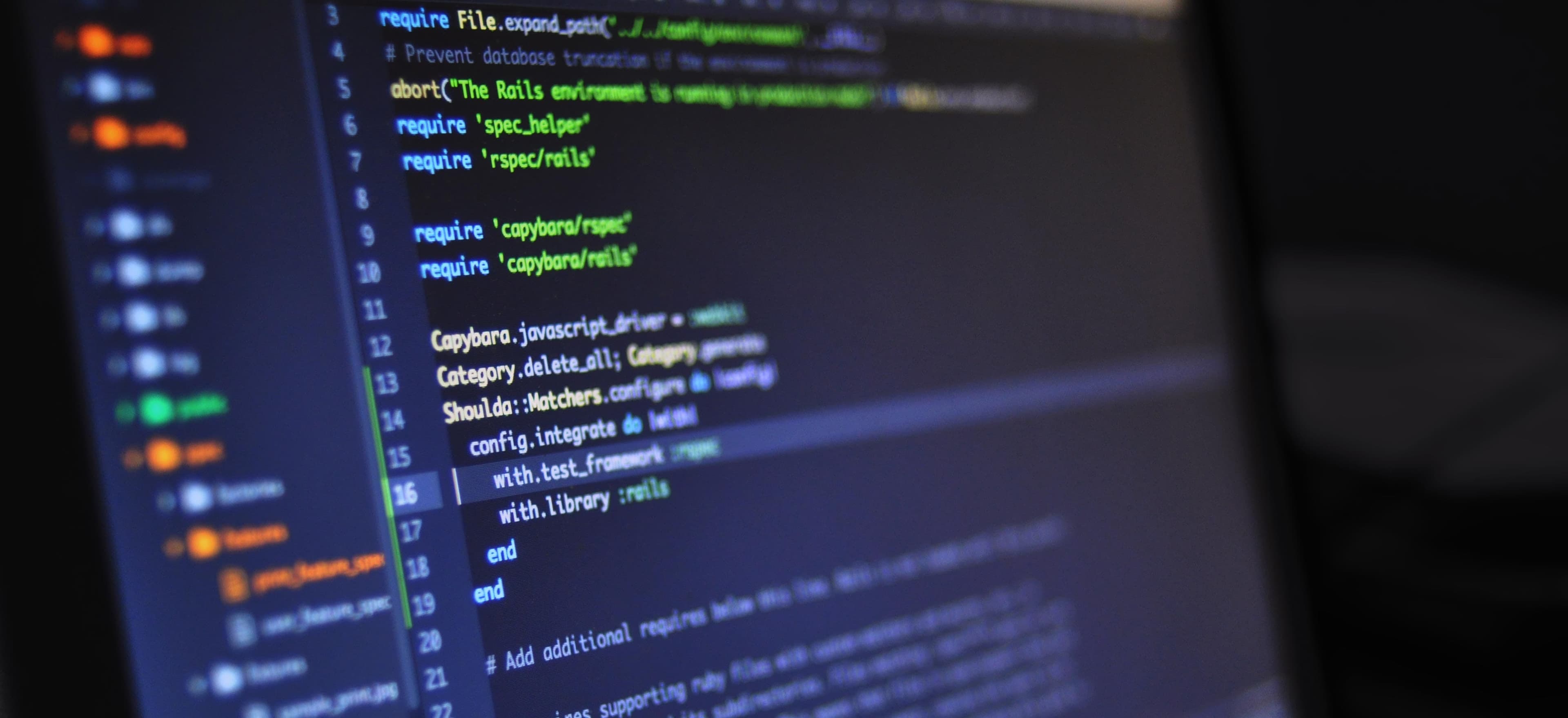
- Published on
Understanding Static Context: Calling getClass() Issues in Java
Java is a powerful programming language that provides developers with various tools to create flexible and efficient applications. However, navigating its concepts can sometimes lead to confusion. One such concept is the interaction between static context and instance methods, particularly with the getClass()
method. In this blog post, we will discuss the nature of the static context, the meaning of getClass()
, scenarios where issues may arise, and how to handle them effectively.
What is Static Context?
In Java, the term "static context" refers to the scope of static members. Static methods and variables belong to the class level rather than any specific instance. This means that they can be called on the class itself, without needing to create an object first.
Consider the following example:
public class StaticContext {
static int count = 0;
static void incrementCount() {
count++;
}
public static void main(String[] args) {
StaticContext.incrementCount();
System.out.println("Count: " + count); // Outputs: Count: 1
}
}
In the StaticContext
class above, both count
and incrementCount()
are static members. The main
method demonstrates that we can access them without instantiating the StaticContext
class.
Understanding getClass()
The getClass()
method is an instance method defined in the java.lang.Object
class. It returns the runtime class of the current object. In simpler terms, it tells you what class the object is an instance of.
Here's a brief example:
public class GetClassExample {
public void displayClass() {
System.out.println("Class: " + getClass());
}
public static void main(String[] args) {
GetClassExample example = new GetClassExample();
example.displayClass(); // Outputs: Class: class GetClassExample
}
}
In this code, when displayClass()
is called on an instance of GetClassExample
, it correctly outputs the class of that instance.
Static Context and getClass()
The main issue arises when we try to call getClass()
from a static context. Because getClass()
is an instance method, attempting to call it in a static method will lead to a compilation error. Let's see an example:
public class StaticGetClass {
static void staticMethod() {
System.out.println("Class: " + getClass()); // This will result in a compilation error
}
public static void main(String[] args) {
staticMethod();
}
}
The above code will give us a compile-time error: "non-static method getClass() cannot be referenced from a static context." This error occurs because getClass()
needs an instance of the class to work with, and static methods do not have access to instance-specific data.
Why This Matters
Understanding why you cannot call getClass()
from a static context is critical for proper object-oriented programming. Static methods operate independently from instances, while getClass()
depends on instances. Misusing these can lead to runtime errors or unexpected behavior in larger applications.
How to Work Around the Issue
If you need to access the class type within a static context, you can either:
- Pass an instance to the static method: You can create a static method that accepts an instance of the class as a parameter.
public class StaticGetClassCorrected {
static void staticMethod(StaticGetClassCorrected instance) {
System.out.println("Class: " + instance.getClass()); // Works because we now have an instance
}
public static void main(String[] args) {
StaticGetClassCorrected example = new StaticGetClassCorrected();
staticMethod(example);
}
}
- Use the class name: If you want to refer to the class type statically, you can directly use the class name.
public class StaticClassExample {
static void staticMethod() {
System.out.println("Class: " + StaticClassExample.class); // Outputs the class type directly
}
public static void main(String[] args) {
staticMethod(); // Works without any instance
}
}
Practical Applications
The proper understanding of static context and getClass()
can enhance your coding skills and minimize common pitfalls. Consider the following practical applications:
- Factory patterns: When creating objects in factory patterns, you can utilize the class name to create instances statically.
- Reflection: Understanding class types aids in using Java's reflection capabilities, which allow for inspecting classes at runtime.
For additional insights into class manipulation and reflection, check out the Java Reflection Tutorial.
The Closing Argument
In summary, static context and instance methods can be tricky if not understood correctly. getClass()
is an instance method requiring an actual object, while static members operate at the class level. By learning to navigate the complexities of Java, you can write more efficient, robust code.
To further enhance your Java programming skills, delve deeper into topics like interfaces, inheritance, and polymorphism. Understanding these foundational aspects will make you a confident developer in the Java ecosystem.
Make sure to practice these concepts in your projects, and you'll find yourself utilizing them effortlessly in various application scenarios. For more in-depth discussions and tutorials on Java programming, consider visiting Java Code Geeks.
Happy coding!