Common Spring MVC Annotation Mistakes to Avoid
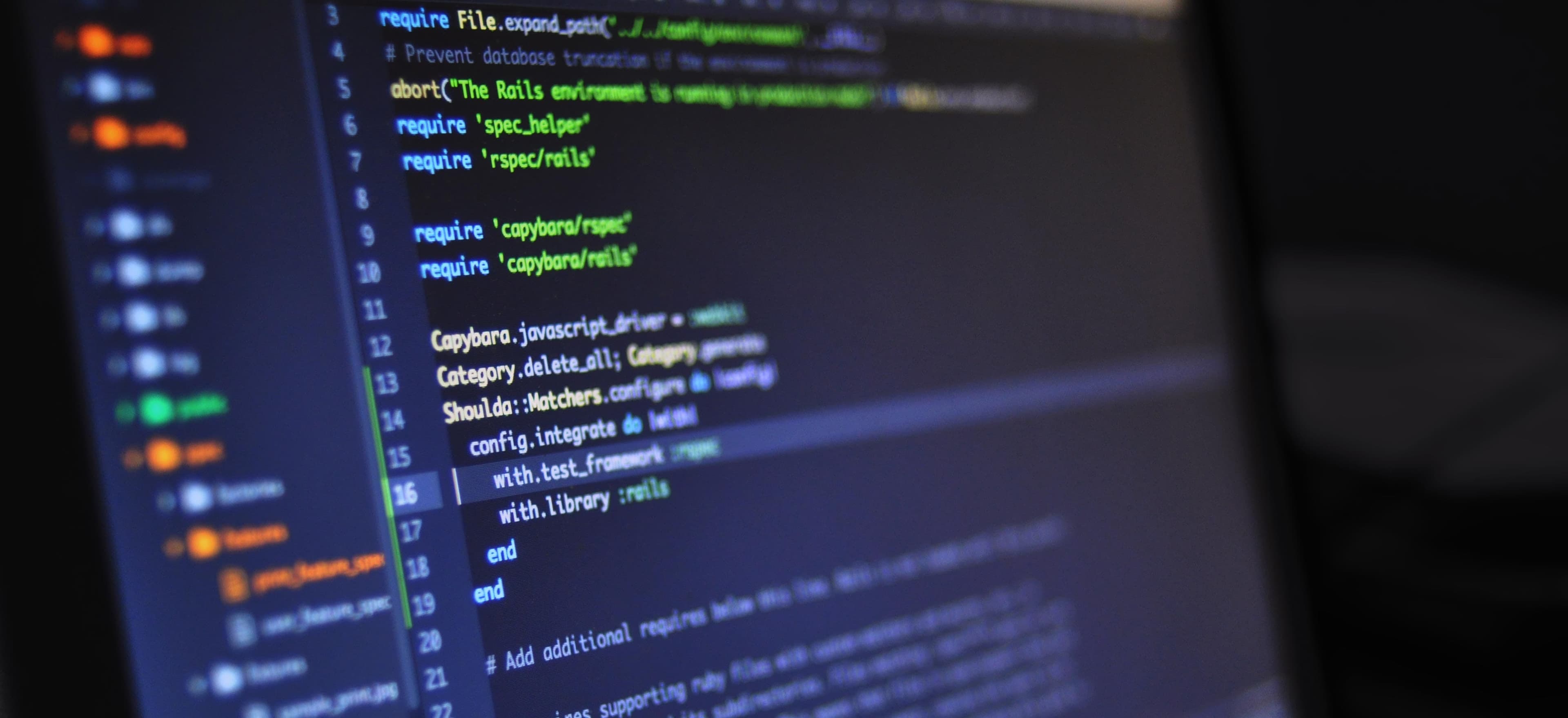
- Published on
Common Spring MVC Annotation Mistakes to Avoid
Spring MVC is a powerful framework for building web applications in Java. Its use of annotations simplifies configuration and minimizes boilerplate code. However, as with any powerful tool, it's easy to misstep if you’re not familiar with the intricacies. Whether you’re a newcomer or a seasoned developer, there are common mistakes you should avoid when working with Spring MVC annotations.
1. Ignoring the @Controller Annotation
The first mistake is neglecting the @Controller
annotation on your class. This annotation is essential for marking your class as a Spring MVC controller.
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
@Controller
public class MyController {
@GetMapping("/welcome")
public String welcome() {
return "welcome"; // Returns the name of the view
}
}
Why This Matters
Without this annotation, Spring doesn't treat your class as a controller, meaning that your endpoints won't be mapped correctly. Always ensure you annotate your controller classes.
2. Misusing @RequestMapping
A common pitfall is the improper use of the @RequestMapping
annotation. It's crucial to apply it on the method level while also recognizing how to use the class level.
Example of Misuse
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
@RequestMapping("/api")
public class MyApiController {
@RequestMapping("/data") // This is okay
public String getData() {
return "data";
}
@RequestMapping(value = "/data", method = RequestMethod.POST) // This is also okay
public String postData() {
return "data saved";
}
}
Mistake
Many developers forget to specify the method type when using @RequestMapping
. By not specifying methods, developers expose endpoints to all HTTP methods, which may inadvertently allow undesired access.
The Right Way
Instead, use the more specific @GetMapping
, @PostMapping
, @PutMapping
, and @DeleteMapping
to clearly define intended behaviors.
@GetMapping("/data") // Correct for GET requests
public String getData() {
return "data";
}
@PostMapping("/data") // Correct for POST requests
public String postData() {
return "data saved";
}
3. Overlooking @PathVariable and @RequestParam
Another frequent error involves misunderstanding the purpose of @PathVariable
and @RequestParam
.
Example
@GetMapping("/items/{id}")
public String getItem(@PathVariable String id) {
return "Item ID: " + id;
}
Common Mistake
Using @RequestParam
instead of @PathVariable
for URL segments is a mistake that can lead to confusion and incorrect behavior.
Correct Usage
- Use
@PathVariable
for dynamic segments in your URL. - Use
@RequestParam
for query parameters.
@GetMapping("/items") // URL: /items?id=10
public String getItems(@RequestParam int id) {
return "Item ID: " + id;
}
4. Failing to Handle Exceptions
Many developers overlook the importance of exception handling in Spring MVC. When an exception arises, it’s essential to manage it gracefully.
Common Practice
Using a global exception handler is a good practice that avoids clutter in individual controllers.
import org.springframework.web.bind.annotation.ControllerAdvice;
import org.springframework.web.bind.annotation.ExceptionHandler;
import org.springframework.http.ResponseEntity;
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(Exception.class)
public ResponseEntity<String> handleException(Exception e) {
return ResponseEntity.status(500).body("An error occurred: " + e.getMessage());
}
}
Why This Matters
Failure to handle exceptions properly may lead your application to crash or return HTTP 500 errors without meaningful messages for the client.
5. Forgetting @ResponseBody
Using @ResponseBody
can be easy to overlook when returning JSON or XML responses. In a traditional view-based application, returning views might be the norm, but in an API-centric application, using @ResponseBody
becomes essential.
Example
@GetMapping("/api/user")
@ResponseBody
public User getUser() {
return new User("John", 30);
}
Note
With @RestController
, there’s no need for @ResponseBody
, as it is implied. However, when using @Controller
, always keep it in mind.
6. Not Validating Input Properly
Neglecting input validation in your controllers can lead to security vulnerabilities.
Example of Input Validation
import org.springframework.validation.annotation.Validated;
@Validated
@PostMapping("/user")
public ResponseEntity<String> createUser(@Valid @RequestBody User user) {
return ResponseEntity.ok("User created successfully");
}
Importance
Incorporating validation not only secures your application but also enhances the user's experience by providing immediate feedback on incorrect input.
7. Not Structuring Packages Properly
Code organization is crucial in Spring applications. Often, you’ll find developers placing controllers, services, and repositories in a single package.
Recommended Practice
Use the following structure:
com.example.app
├── controller
├── service
└── repository
Why It Matters
This organization fosters a clearer understanding of your application’s architecture and helps maintainability over time.
8. Over-Annotation
Finally, don’t overload your controller methods with too many annotations. While Spring MVC provides numerous annotations to customize behavior, using too many makes your code harder to read.
Example of Over-Annotation
@GetMapping(path = "/info", consumes = "application/json", produces = "application/json")
public String getInfo() {
return "Info";
}
Better Approach
Only include what is necessary:
@GetMapping("/info") // Simpler and clear
public String getInfo() {
return "Info";
}
The Closing Argument
Spring MVC’s annotation-based configuration is a tremendous asset, but like any tool, it demands a level of expertise to wield effectively. By avoiding the common mistakes outlined above, you can enhance the reliability and maintainability of your Spring applications.
If you're interested in further reading or seeking deeper learning resources, consider these links:
- Spring MVC Documentation
- Java Design Principles
Developing a strong grasp of these fundamentals will not only make you a more competent developer but will also lead to more robust and resilient applications. Happy coding!
Checkout our other articles