Conditionally Executing CommandLineRunner in Spring Boot
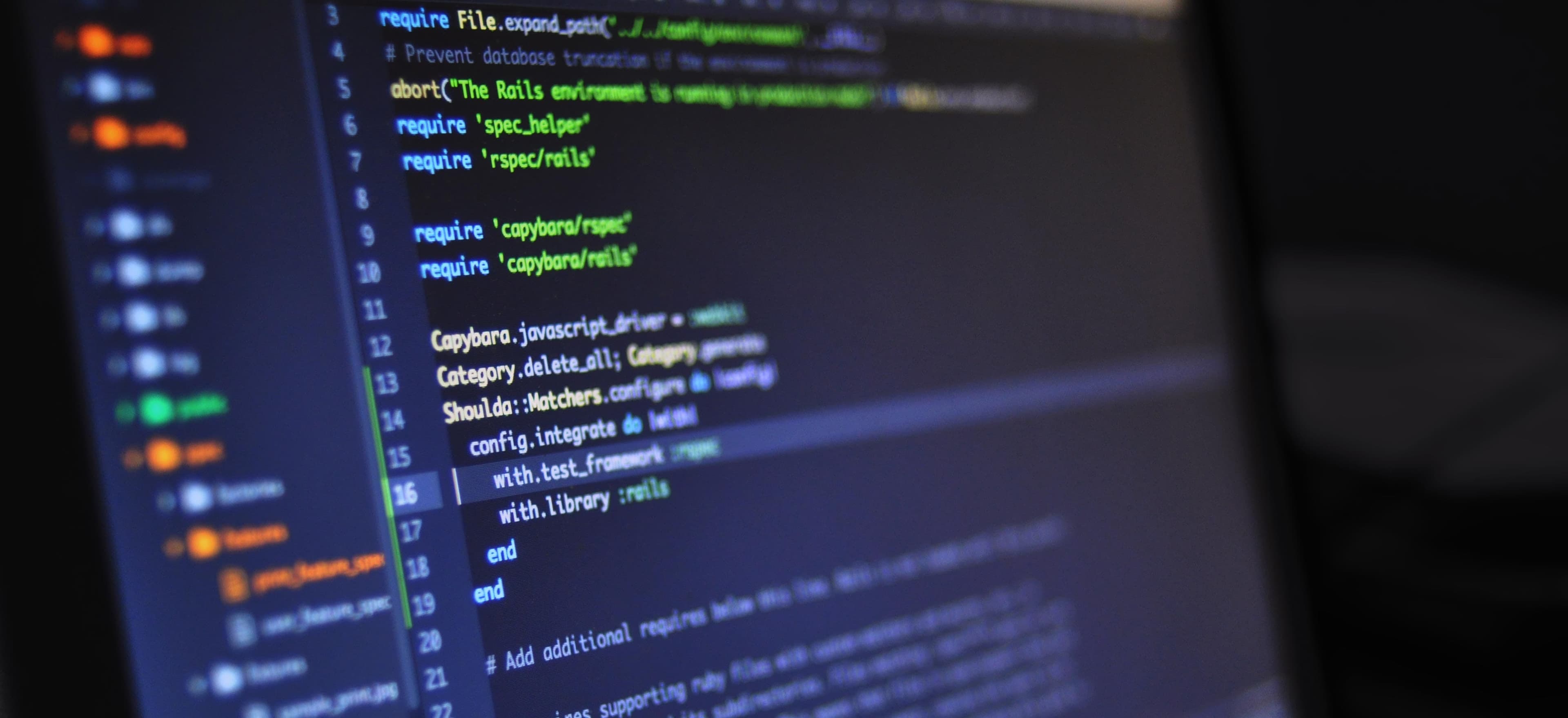
- Published on
Conditionally Executing CommandLineRunner in Spring Boot
Spring Boot is a powerful tool for developing applications quickly and efficiently. One of its many features is the CommandLineRunner
interface, which provides a way to execute code after the application has started. This is particularly useful for initializing data or executing code that needs to be run once the application context is fully loaded. However, there are times when you may not want to run certain logic under specific conditions. In this post, we'll explore how to conditionally execute code in a CommandLineRunner
.
What is CommandLineRunner?
CommandLineRunner
is a functional interface in Spring Boot. By implementing this interface, you can define a run
method that Spring will automatically execute after the application context is fully loaded.
Here is a simple example:
import org.springframework.boot.CommandLineRunner;
import org.springframework.stereotype.Component;
@Component
public class MyStartupRunner implements CommandLineRunner {
@Override
public void run(String... args) throws Exception {
System.out.println("Running startup logic...");
}
}
In this case, upon starting the application, you would see the message "Running startup logic..." in the console.
Why Use Conditional Execution
While CommandLineRunner
can be handy for seamless execution at startup, you may want to run certain logic conditionally. This can help you avoid unintended behaviors, such as running database migrations or seed data insertion in a production environment when it isn't required or could lead to errors.
For example:
- You may have a given setting in your
application.properties
file that toggles development and production modes. - You might want to check whether a certain environment variable is set before executing the logic.
- You can also react based on certain service states or configurations.
Implementing Conditional Execution
Let's consider a scenario where we conditionally execute code in our CommandLineRunner
based on a property set in application.properties
. For instance, we want our startup logic to run only if we're in development mode.
Step 1: Configure your application.properties
First, add a property to your application.properties
file:
app.mode=development
Step 2: Modify the CommandLineRunner
Now, let's modify our MyStartupRunner
class to only run the logic when the application mode is set to "development".
import org.springframework.boot.CommandLineRunner;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Component;
@Component
public class MyStartupRunner implements CommandLineRunner {
@Value("${app.mode}")
private String appMode;
@Override
public void run(String... args) throws Exception {
if ("development".equalsIgnoreCase(appMode)) {
System.out.println("Running startup logic in development mode...");
} else {
System.out.println("Skipping startup logic in production mode.");
}
}
}
Step 3: Explanation of the Code
- @Value Annotation: This annotation is used to inject properties from the application properties file.
- Equals Ignore Case: This checks for string equality while ignoring the case (to prevent issues due to different casing).
In this setup, if you set app.mode
to production
, the output would change, and the startup logic wouldn't run.
Utilizing Profiles for Conditional Execution
Spring also supports Profiles which can be used to define environments like development or production. Conditional execution can also be managed using these profiles which can provide even more structure to your application.
Step 1: Define a Profile
In your application.yml
or application.properties
file, you can define different profiles:
spring:
profiles:
active: dev
Step 2: Use Profile-specific Configurations
You can define your CommandLineRunner
in a profile-specific way:
import org.springframework.boot.CommandLineRunner;
import org.springframework.context.annotation.Profile;
import org.springframework.stereotype.Component;
@Profile("dev") // This component will only be registered in the dev profile
@Component
public class DevStartupRunner implements CommandLineRunner {
@Override
public void run(String... args) throws Exception {
System.out.println("Running startup logic for development profile...");
}
}
Step 3: Explanation of Profile Annotations
- @Profile Annotation: This annotation will ensure that the
DevStartupRunner
is only instantiated when thedev
profile is active.
If you switch to the prod
profile, you can have a different implementation or none at all, effectively controlling which startup logic runs based on your environment.
Using Conditional Annotations
Additionally, Spring provides conditional annotations like @ConditionalOnProperty
, which can help you achieve similar functionality without hardcoding values in your Java code.
Here’s how you can use @ConditionalOnProperty
:
import org.springframework.boot.CommandLineRunner;
import org.springframework.boot.autoconfigure.condition.ConditionalOnProperty;
import org.springframework.stereotype.Component;
@ConditionalOnProperty(name = "app.mode", havingValue = "development")
@Component
public class ConditionalStartupRunner implements CommandLineRunner {
@Override
public void run(String... args) throws Exception {
System.out.println("Running startup logic in development mode via ConditionalOnProperty...");
}
}
Explanation of ConditionalOnProperty
- ConditionalOnProperty: This allows you to specify when a bean should be created. In this case,
ConditionalStartupRunner
will only run startup logic ifapp.mode
is set todevelopment
.
Wrapping Up
Conditionally executing a CommandLineRunner
in Spring Boot is an excellent way to fine-tune your application's startup behavior based on specific requirements or environments. Whether through property checks, profiles, or conditional annotations, you can ensure that your application runs the necessary startup logic at the right time.
For more information on configuring your application with Spring Boot, consider reviewing the Spring Boot official documentation.
With the knowledge of conditional execution, you can make your Spring Boot applications even more robust and adaptable to varying environments. Happy coding!
Checkout our other articles