Optimizing AWS DynamoDB Queries in Reactive Spring WebFlux
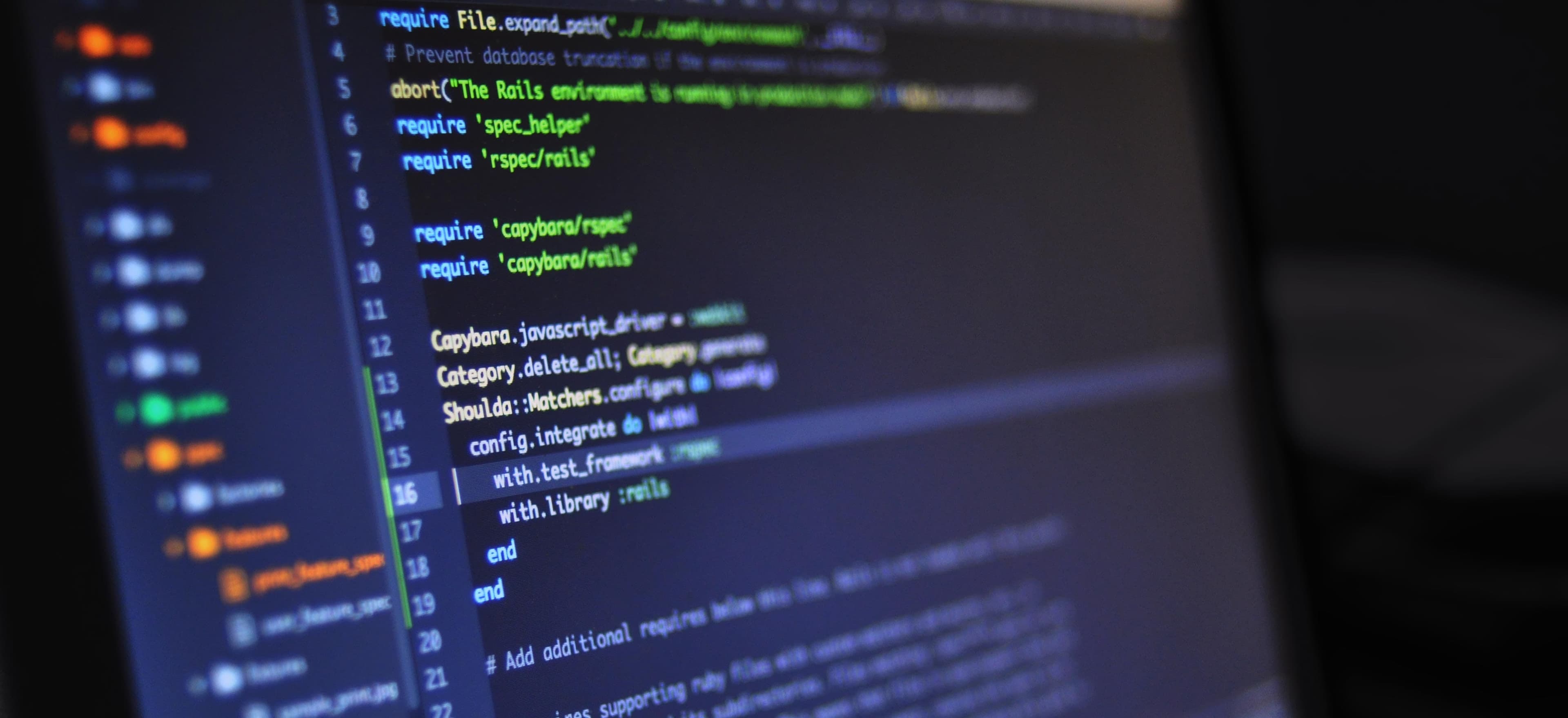
- Published on
Optimizing AWS DynamoDB Queries in Reactive Spring WebFlux
In the world of modern application development, the combination of AWS DynamoDB and Reactive Spring WebFlux can deliver highly responsive and efficient applications. While both technologies offer several advantages, optimizing DynamoDB queries in a reactive environment requires a detailed understanding of how to best leverage the interplay between them. In this post, we will explore effective strategies to optimize your DynamoDB queries using Reactive Spring WebFlux, enhancing the overall performance of your application.
Understanding Reactive Spring WebFlux
Spring WebFlux is part of the Spring Framework that enables non-blocking, asynchronous programming. This model is essential for applications that require handling numerous concurrent connections with efficient resource utilization. By choosing Reactive Spring WebFlux, developers can build applications that respond instantly without holding up threads.
Key Features of Spring WebFlux
- Reactive Programming: Utilizes reactive programming principles, providing a more natural fit for asynchronous and event-driven systems.
- Backpressure: Supports backpressure, enabling the system to manage the flow of data and avoid overwhelming subscribers.
- Non-blocking I/O: Allows for handling multiple requests without the overhead associated with traditional multi-threading.
Let us Begin to AWS DynamoDB
Amazon DynamoDB is a fully managed NoSQL database service that provides fast and predictable performance with seamless scalability. It is designed to handle both unstructured and structured data while ensuring low-latency reads and writes.
Key Features of DynamoDB
- Fully Managed: AWS handles maintenance tasks such as backups and scaling, allowing developers to focus on their application.
- Scalable: Automatically scales throughput capacity based on traffic demands.
- Flexible Schema: Supports flexible data models, making it suitable for a variety of use cases.
Setting Up a Reactive Spring WebFlux with DynamoDB
To start querying DynamoDB in a non-blocking fashion, you'll need to set up your project with the required dependencies. Add the following dependencies to your pom.xml
if you are using Maven:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-webflux</artifactId>
</dependency>
<dependency>
<groupId>software.amazon.awssdk</groupId>
<artifactId>dynamodb</artifactId>
</dependency>
<dependency>
<groupId>io.projectreactor</groupId>
<artifactId>reactor-core</artifactId>
</dependency>
Optimizing DynamoDB Queries
Once your basic setup is complete, you can focus on optimizing the querying mechanism. Here are some strategies to enhance the performance of DynamoDB queries:
1. Use Efficient Query Patterns
DynamoDB offers two primary ways to query data: GetItem and Query. Each has its own performance considerations:
- GetItem: Fetches a single item in constant time, which is optimal. It is perfect when you know the exact primary key.
public Mono<Item> getItem(String primaryKey) {
GetItemRequest getItemRequest = GetItemRequest.builder()
.tableName("YourTableName")
.key(Collections.singletonMap("PrimaryKey", AttributeValue.builder().s(primaryKey).build()))
.build();
return Mono.fromFuture(() -> dynamoDbClient.getItem(getItemRequest))
.map(GetItemResponse::item)
.map(this::mapToItem);
}
This method is efficient for retrieving unique records, thereby minimizing read capacity units (RCUs).
- Query: Utilizes partition keys and can filter results by sort keys. Ensure you are using secondary indexes effectively if you need to query beyond primary key lookups.
public Flux<Item> queryItems(String partitionKey) {
QueryRequest queryRequest = QueryRequest.builder()
.tableName("YourTableName")
.keyConditionExpression("PartitionKey = :v1")
.expressionAttributeValues(Collections.singletonMap(":v1", AttributeValue.builder().s(partitionKey).build()))
.build();
return Flux.fromFuture(() -> dynamoDbClient.query(queryRequest))
.flatMap(queryResponse -> Flux.fromIterable(queryResponse.items()))
.map(this::mapToItem);
}
Using indexed queries ensures that you only retrieve the data you need, optimizing throughput.
2. Pagination for Large Result Sets
When dealing with large datasets, always consider implementing pagination. DynamoDBn terms, use the LastEvaluatedKey to track your position in the dataset and retrieve results in chunks.
public Flux<Item> paginateQuery(String partitionKey, String startKey) {
Map<String, AttributeValue> lastKey = startKey != null ?
Collections.singletonMap("PrimaryKey", AttributeValue.builder().s(startKey).build()) :
null;
QueryRequest queryRequest = QueryRequest.builder()
.tableName("YourTableName")
.exclusiveStartKey(lastKey)
.keyConditionExpression("PartitionKey = :v1")
.expressionAttributeValues(Collections.singletonMap(":v1", AttributeValue.builder().s(partitionKey).build()))
.build();
return Flux.defer(() -> {
return Mono.fromFuture(() -> dynamoDbClient.query(queryRequest))
.flatMapMany(queryResponse -> {
if (queryResponse.hasItems()) {
// Next page might be available
items = Flux.fromIterable(queryResponse.items()).map(this::mapToItem);
return items.concatWith(paginateQuery(partitionKey, queryResponse.lastEvaluatedKey()));
}
return Flux.empty();
});
});
}
This approach minimizes the data retrieved per request, enhancing efficiency.
3. Use Projections Wisely
By default, DynamoDB retrieves all attributes of an item. However, if you only need specific fields, use projection expressions to limit the attributes returned. This reduces the amount of data transferred and improves performance.
public Mono<Item> getSpecificAttributes(String primaryKey) {
GetItemRequest getItemRequest = GetItemRequest.builder()
.tableName("YourTableName")
.key(Collections.singletonMap("PrimaryKey", AttributeValue.builder().s(primaryKey).build()))
.projectionExpression("id, name")
.build();
return Mono.fromFuture(() -> dynamoDbClient.getItem(getItemRequest))
.map(GetItemResponse::item)
.map(this::mapToRequiredItem);
}
Using projections can drastically reduce the I/O and memory overhead involved in processing large items.
4. Use Caching
Integrate a caching layer between your application and DynamoDB. Use solutions like Redis or AWS ElastiCache to cache frequently accessed data, avoiding unnecessary DynamoDB queries.
Consider this simple caching strategy using a Map
.
private final Map<String, Item> cache = new ConcurrentHashMap<>();
public Mono<Item> getCachedItem(String primaryKey) {
return Mono.justOrEmpty(cache.get(primaryKey))
.switchIfEmpty(getItem(primaryKey)
.doOnNext(item -> cache.put(primaryKey, item)));
}
This not only improves response times but also lowers the costs associated with DynamoDB queries.
5. Monitor and Adjust Throughput
Utilize AWS CloudWatch to monitor your DynamoDB table's Read and Write Capacity Units. Based on your application’s usage patterns, adjust the provisioned capacity or switch to Auto Scaling to dynamically adapt to demand — keeping costs reasonable while ensuring performance.
A Final Look
Optimizing AWS DynamoDB queries in a Reactive Spring WebFlux application can significantly enhance your application's responsiveness and reliability. By implementing efficient query patterns, utilizing pagination, applying projection expressions, leveraging caching mechanisms, and actively monitoring throughput, developers can create a high-performing application that meets user expectations in today’s fast-paced environment.
For further reading on related topics, consider checking:
By following these strategies and best practices, you'll ensure that your applications can handle workloads effectively and efficiently, maximizing the potential of both DynamoDB and Spring WebFlux.
By understanding the synergy between DynamoDB and Spring WebFlux, and utilizing proper optimization techniques, you'll be well-placed to create applications that deliver outstanding performance and user experience. Happy coding!
Checkout our other articles