The Perils of JPA Lazy Loading: When to Draw the Line
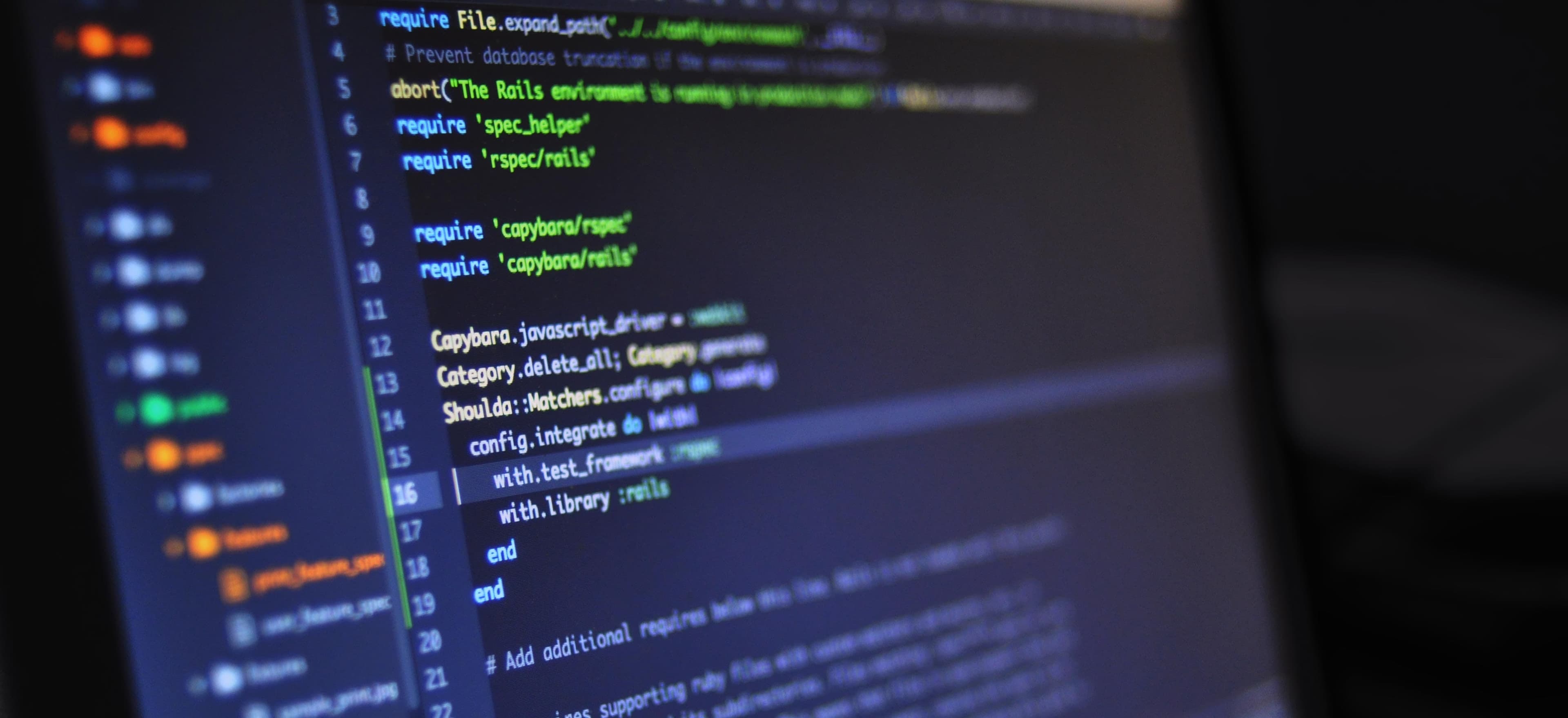
- Published on
The Perils of JPA Lazy Loading: When to Draw the Line
Java Persistence API (JPA) is an essential component for managing relational data in Java applications. It provides a way to interact with databases using Java objects, simplifying data access and manipulation. One of its most powerful features is lazy loading. While lazy loading can optimize performance and memory usage, it also comes with its own set of challenges and pitfalls. In this post, we will delve into the intricacies of JPA lazy loading, highlighting its advantages, potential issues, and best practices to draw the line effectively.
Understanding Lazy Loading in JPA
What is Lazy Loading?
Lazy loading is a design pattern used to defer the initialization of an object until the point at which it is needed. In JPA, this means that related entities are not immediately fetched from the database when the parent entity is loaded. Instead, they are loaded on-demand, which can lead to significant performance improvements in cases where related data is large or infrequently accessed.
How Does It Work?
When you fetch an entity from the database using JPA, any relationships marked with @OneToMany
, @ManyToMany
, or @OneToOne
can be configured for lazy loading using the fetch = FetchType.LAZY
attribute.
Here is a simple code example:
@Entity
public class Order {
@Id
private Long id;
@OneToMany(fetch = FetchType.LAZY)
private List<OrderItem> items;
// Getters and Setters
}
In this example, an Order
entity will not load its OrderItem
collection until you explicitly access it. This can save memory and improve application startup time.
The Pros of Lazy Loading
-
Performance Optimization: By not loading all related entities up front, you save on database queries and reduce the memory footprint of your application, especially when dealing with large datasets.
-
Reduced Initial Load Time: Entities can be loaded faster because only the required data is retrieved, enabling quicker user interactions and a snappier user experience.
-
Flexibility: Lazy loading allows developers to define which relationships should be loaded, providing greater control over data access.
The Cons of Lazy Loading
While lazy loading can be beneficial, it comes with its risks.
- N+1 Select Problem: This occurs when a single query to load parent entities leads to additional queries for each of the related entities. This can substantially degrade performance:
List<Order> orders = orderRepository.findAll(); // 1 query
for(Order order : orders) {
order.getItems(); // N additional queries
}
In this scenario, if there are 100 orders, this leads to 101 database queries, significantly impacting performance.
- LazyInitializationException: This occurs when an entity is accessed outside of an active Hibernate session. If you try to access a lazily-loaded collection after the session has closed, an exception will be thrown:
@Transactional
public Order getOrder(Long id) {
Order order = orderRepository.findById(id);
// Session closes here
List<OrderItem> items = order.getItems(); // Throws LazyInitializationException
return order;
}
- Over-fetching: Sometimes, developers overuse lazy loading without considering the implications, fetching more data than necessary, leading to performance degradations.
Best Practices to Manage Lazy Loading
Here are some guidelines to manage lazy loading effectively in your JPA applications:
1. Use @Transactional
Wisely
Ensure that entity access happens within a transactional context. Use the @Transactional
annotation on service methods to keep the session open:
@Transactional
public List<Order> getAllOrders() {
return orderRepository.findAll();
}
2. Consider Join Fetching
If you know that you will need the related entities, you can use join fetching to load them in a single query, thus preventing the N+1 problem:
@Query("SELECT o FROM Order o JOIN FETCH o.items")
List<Order> findAllWithItems();
Using join fetching can significantly reduce the number of total database queries, improving application performance.
3. Eager Loading Where Appropriate
Sometimes, certain relationships are always required, and lazy loading may not be the best choice. Consider using fetch type EAGER
in such cases — although with caution due to potential performance issues:
@OneToMany(fetch = FetchType.EAGER)
private List<OrderItem> items;
4. Use DTOs for Specific Queries
Data Transfer Objects (DTOs) can be effective when you need specific information from multiple related entities without triggering lazy loading. This can optimize data retrieval:
public class OrderDTO {
private Long orderId;
private List<OrderItemDTO> items;
// Constructor, getters, setters...
}
// Use a query to populate the DTO
@Query("SELECT new com.example.OrderDTO(o.id, i) FROM Order o JOIN o.items i WHERE o.id = :id")
OrderDTO findOrderDtoById(@Param("id") Long id);
5. Monitor Database Performance
It's crucial to keep an eye on your database performance when using lazy loading. Tools such as Hibernate's statistics can provide insight into query performance, allowing you to identify potential bottlenecks:
SessionFactory sessionFactory = ...; // Obtain session factory
Statistics stats = sessionFactory.getStatistics();
stats.setStatisticsEnabled(true);
// Perform database operations
System.out.println("Number of queries executed: " + stats.getQueryExecutionCount());
6. Use Caching
Implement caching mechanisms to avoid repeated queries for the same data. JPA providers like Hibernate offer first-level and second-level caching.
@Entity
@Cacheable
public class Order {
// Entity definition
}
Final Thoughts
JPA lazy loading is a powerful feature that can enhance the performance and scalability of your applications when used correctly. However, it is crucial to understand its implications and the potential pitfalls that can arise from careless implementation. By applying best practices like careful transaction management, appropriate fetching strategies, and monitoring performance, you can effectively draw the line.
Always remember that every application's needs are unique. Therefore, evaluate the trade-offs of lazy loading on a case-by-case basis to ensure optimal database access patterns.
For more insight into JPA and related technologies, consider exploring additional resources:
- JPA Documentation
- Hibernate ORM Documentation
- Deep Dive into JPA Performance
With thoughtful application of JPA's capabilities, you can elevate your Java applications to meet performance expectations while maintaining a high standard of code quality. Happy coding!
Checkout our other articles