Common API Design Pitfalls and How to Avoid Them
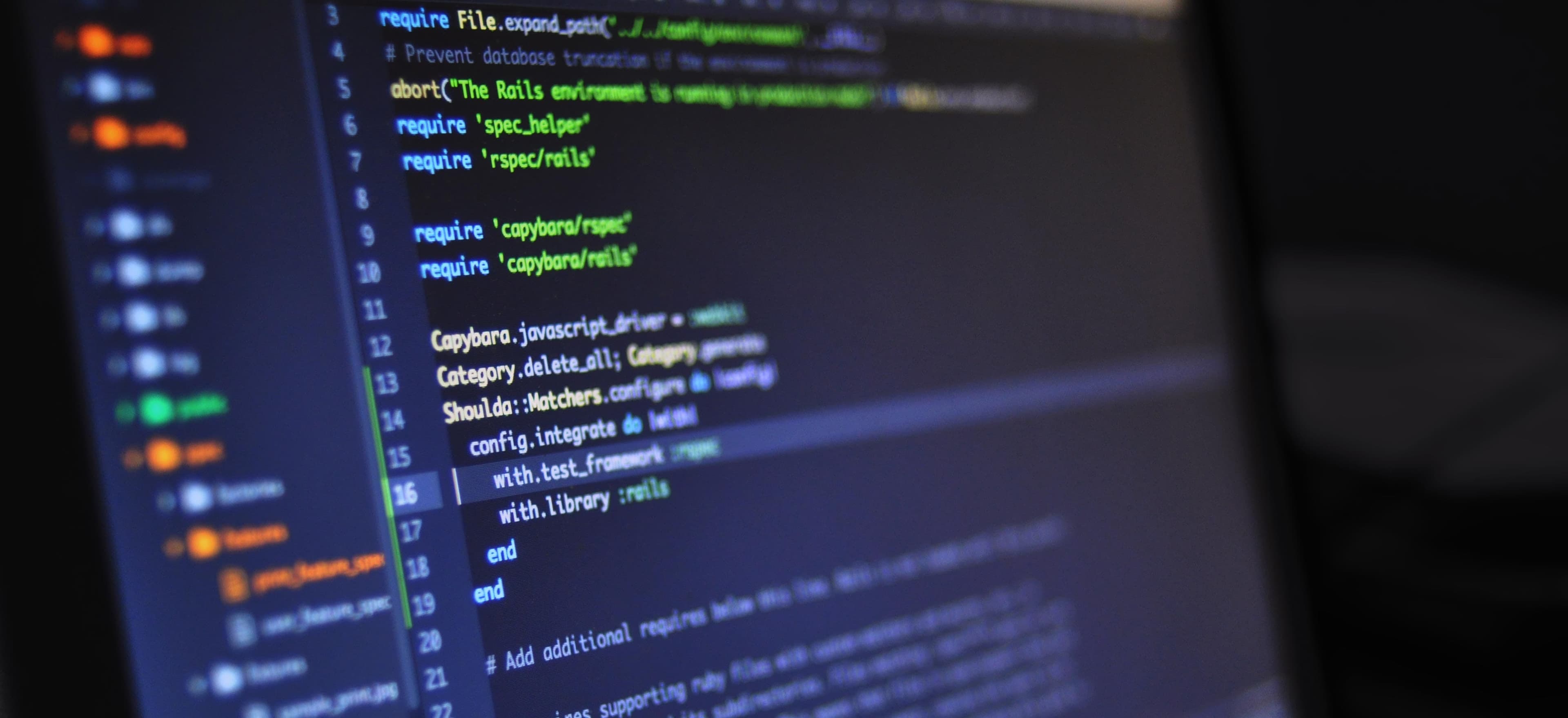
- Published on
Common API Design Pitfalls and How to Avoid Them
In the vast world of software development, Application Programming Interfaces (APIs) serve as crucial bridges that allow different software applications to communicate with one another. However, designing an effective API is not always straightforward. It involves careful consideration of various factors to ensure usability, scalability, and performance. In this blog post, we will explore some common API design pitfalls and provide strategies to avoid them, all backed by Java code examples to illustrate best practices.
Understanding API Design
API design is about crafting user-friendly interfaces that communicate effectively with others. The best APIs are intuitive and offer a clear contract between the provider and the consumer. Before diving into pitfalls, let’s set the stage with an exemplary API design.
Basic Structure
Imagine you are designing a RESTful API for managing a simple task list. Below is a basic example of how you might define endpoints:
public class TaskController {
// Endpoint to create a new task
@POST
@Path("/tasks")
public Response createTask(Task task) {
// Logic to save the task
}
// Endpoint to retrieve all tasks
@GET
@Path("/tasks")
public List<Task> getTasks() {
// Logic to fetch all tasks
}
}
This example sets a solid foundation by clearly defining endpoints using HTTP methods. However, let’s explore the common pitfalls you might encounter in API design.
Common API Design Pitfalls
1. Inconsistent Naming Conventions
Pitfall: Using varying naming conventions can confuse developers.
When creating an API, ensure consistent naming across endpoints, methods, and data models.
Avoidance Strategy: Stick to widely accepted conventions such as:
- Use nouns for endpoints:
/tasks
,/users
- Use HTTP verbs: GET for retrieval, POST for creation, etc.
// Inconsistent naming leading to confusion
@GET
@Path("/getAllTasks") // Not recommended
@GET
@Path("/tasks") // Preferred
2. Lack of Versioning
Pitfall: Not versioning your API can lead to breaking changes down the line.
Avoidance Strategy: Always version your API to maintain backward compatibility. A common way to do this is through the URL.
@GET
@Path("/v1/tasks")
public List<Task> getTasksV1() {
// Logic for version 1
}
@GET
@Path("/v2/tasks")
public List<Task> getTasksV2() {
// Logic for version 2 (with enhancements)
}
Versioning allows you to introduce changes without disrupting existing users. For further reading on API versioning best practices, visit Versioning APIs.
3. Overcomplicated Responses
Pitfall: Overloading API responses can lead to confusion and larger payloads.
Keep responses simple. Return only the necessary fields to the client.
Avoidance Strategy: Use DTOs (Data Transfer Objects) to encapsulate the required data.
public class TaskDTO {
private Long id;
private String title;
// Getters and setters
}
@GET
@Path("/tasks")
public List<TaskDTO> getTasks() {
List<Task> tasks = fetchAllTasks();
return tasks.stream()
.map(task -> new TaskDTO(task.getId(), task.getTitle()))
.collect(Collectors.toList());
}
4. Not Using Appropriate HTTP Status Codes
Pitfall: Misusing HTTP status codes can lead to ambiguity in responses.
Avoidance Strategy: Use the appropriate status codes that convey the right information.
@POST
@Path("/tasks")
public Response createTask(Task task) {
// Assume the task is created successfully
return Response.status(Response.Status.CREATED).build();
}
@GET
@Path("/tasks/{id}")
public Response getTask(@PathParam("id") Long id) {
Task task = findTaskById(id);
if (task == null) {
return Response.status(Response.Status.NOT_FOUND).build();
}
return Response.ok(task).build();
}
Security First: Common Security Pitfalls
5. Insecure Authentication
Pitfall: Inadequate authentication mechanisms can expose your API to vulnerabilities.
Ensure that your API enforces strong authentication protocols.
Avoidance Strategy: Use OAuth 2.0 and JWT (JSON Web Tokens) for securing your APIs.
@GET
@Path("/tasks")
@RolesAllowed({"USER", "ADMIN"})
public List<TaskDTO> getTasks() {
// Fetch and return tasks for authenticated users
}
By restricting access based on roles, you contribute to a more secure API environment.
6. Poor Rate Limiting
Pitfall: Not implementing rate limiting can expose your API to abuse.
Avoidance Strategy: Employ rate limiting to ensure that users do not overwhelm your API with requests.
Consider using libraries or frameworks such as Bucket4j for rate limiting.
@GET
@Path("/tasks")
public Response getTasks() {
// Implement rate limiting logic
if (userExceedsAllowedRequests()) {
return Response.status(Response.Status.TOO_MANY_REQUESTS).build();
}
return Response.ok(fetchTasks()).build();
}
Key Takeaways
When it comes to API design, avoiding common pitfalls is essential for creating a reliable and user-friendly interface. Consistency in naming, proper versioning, simplifying responses, utilizing correct HTTP status codes, ensuring security, and implementing rate limiting are integral steps to follow.
By adhering to these guidelines and best practices, you not only enhance the usability of your API but also ensure a better experience for developers who will interact with it. For more in-depth insights into API best practices, be sure to check out Rest API Design Guide.
In a world where APIs fuel the interactions between various software applications, investing time in thoughtful API design will always pay dividends. Happy coding, and may your APIs thrive!
Checkout our other articles