Unlocking the Potential of jcmd: Mastering JDK 13 VM Events
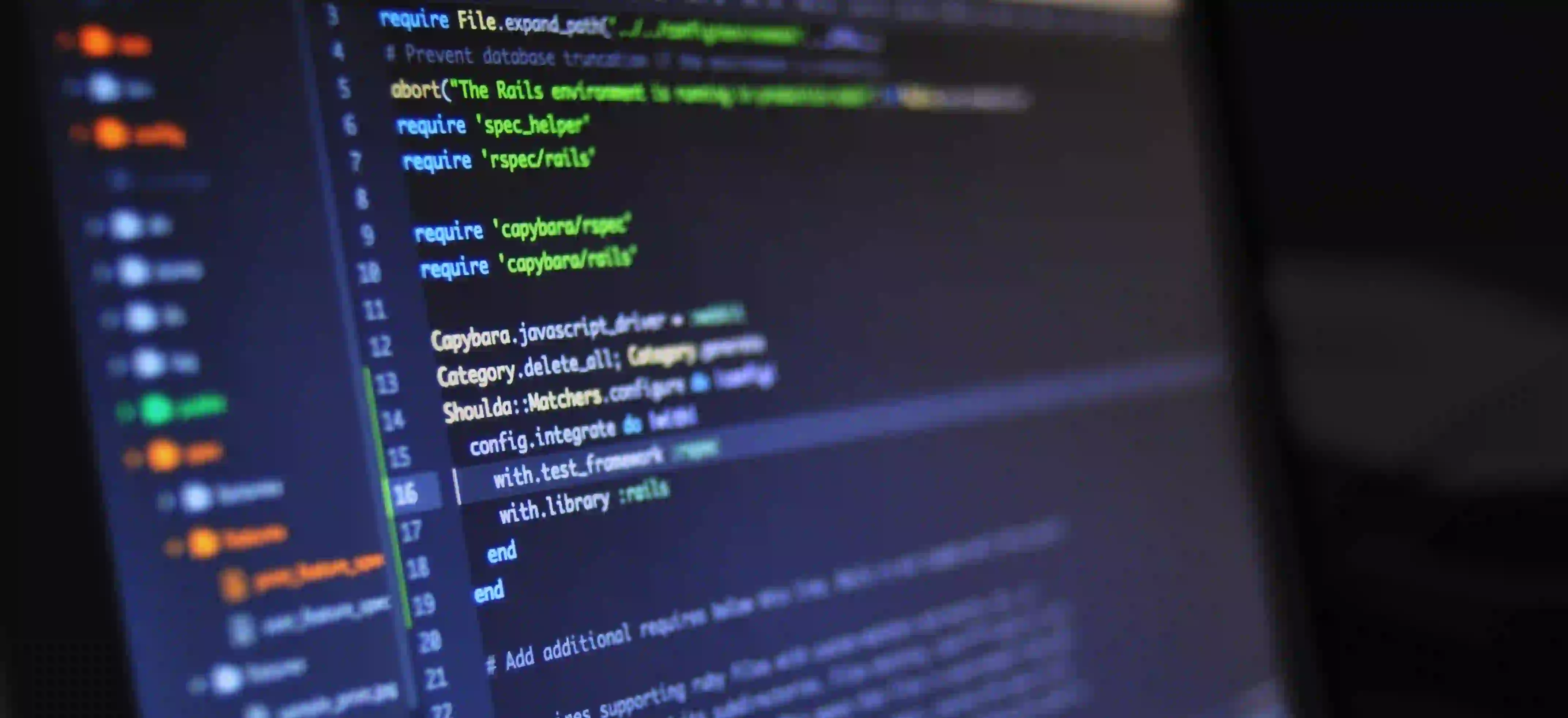
Unlocking the Potential of jcmd: Mastering JDK 13 VM Events
In the ever-evolving world of Java development, the ability to monitor and manage applications is crucial for efficient performance tuning and debugging. One of the most powerful yet often underutilized tools in the Java Development Kit (JDK) is jcmd
. Introduced in earlier Java versions and enhanced in JDK 13, jcmd
provides developers a command-line interface to interact with the Java Virtual Machine (JVM). This article will explore how to leverage jcmd
for managing JVM events, enhancing your ability to diagnose and resolve performance issues effectively.
What is jcmd?
jcmd
stands for "Java Command" and serves as a versatile command-line tool to send commands to a running JVM. This utility allows you to interact with the JVM to request diagnostic commands, including capturing heap dumps, generating thread stacks, and monitoring garbage collection. Unlike other tools in the JDK, such as jconsole
or JVisualVM
, jcmd
operates from the command line, making it suitable for automation and scripting.
Getting Started with jcmd
Before diving into the functionality offered by jcmd
, ensure that JDK 13 is installed and properly set up on your machine. You can check your Java version using the following command:
java -version
If you have JDK 13 installed, you should see output similar to:
openjdk version "13.0.2" 2020-01-14
OpenJDK Runtime Environment (build 13.0.2+8)
OpenJDK 64-Bit Server VM (build 13.0.2+8, mixed mode)
To see a list of running Java processes and their respective process IDs (PIDs), execute:
jcmd
This will output something like:
12345 MyJavaApp
67890 AnotherJavaApp
You can choose the appropriate PID to send commands for that specific running instance.
Working with VM Events
One of the most fascinating aspects of jcmd
is its ability to listen to and manage JVM events. These events can serve as crucial indicators of your application’s health, enabling timely and informed interventions.
Key Commands for VM Events
JDK 13 enhances the functionality of jcmd
, providing a range of commands. Here are some key commands related to VM events:
-
GC (Garbage Collection) Information:
🔧snippet.shjcmd <pid> GC.heap_info
This command retrieves information about the current state of the heap memory, which is crucial for understanding memory utilization.
-
Thread Dump:
🔧snippet.shjcmd <pid> Thread.print
Use this command to obtain a snapshot of all thread states, helping identify blocking threads or deadlock situations.
-
Heap Dump:
🔧snippet.shjcmd <pid> GC.heap_dump <file-path>
It generates a heap dump file at the specified location, which can be analyzed with tools like Eclipse MAT to identify memory leaks.
-
Enable/Disable Verbose GC:
🔧snippet.shjcmd <pid> VM.flag -XX:+PrintGCDetails
This command enables detailed logging of garbage collection events, providing deeper insights into GC activities.
Handling Specific VM Events
Let’s dive deeper into handling specific VM events using jcmd
. For instance, suppose you wish to monitor garbage collection activity and inspect heap sizes in real-time.
Example: Monitoring Garbage Collection
jcmd <pid> GC.run
This will trigger a garbage collection cycle and provide insights into the immediate effects on heap memory.
The output from this command could include:
Garbage collection 0
young generation: used=51MB, committed=60MB
Old generation: used=300MB, committed=300MB
Enabling JMX Events with jcmd
Java Management Extensions (JMX) can also be managed using jcmd
. JMX is a technology that lets you manage and monitor applications. With JMX, you can receive notifications for particular events such as memory threshold crossing.
Example: Setting Up JMX Notifications
First, ensure that JMX is enabled when you start your Java application:
java -Dcom.sun.management.jmxremote ...
You can then listen for notifications using jcmd
:
jcmd <pid> Monitoring.start <mbean_name>
Replace <mbean_name>
with the specific MBean you want to monitor (like a memory pool).
Advanced JVM Event Management
With jcmd
, you can create more sophisticated monitoring scripts. By combining multiple commands and using shell scripting or batch files, you can automate performance assessments.
Example Script: Automated Monitoring
Here’s a basic script that will take a heap dump based on a memory threshold (assuming you’re on a Unix-like system):
#!/bin/bash
THRESHOLD=20000000 # 20 MB
PID=<your_java_app_pid>
while true; do
MEM_USAGE=$(jcmd $PID VM.native_memory summary | grep "Total:" | awk '{print $2}')
if [ "$MEM_USAGE" -gt $THRESHOLD ]; then
jcmd $PID GC.heap_dump /path/to/dump/hotspotHeapDump.hprof
echo "Heap dump created at /path/to/dump/hotspotHeapDump.hprof due to memory threshold breach."
break
fi
sleep 60 # Sleep for 60 seconds
done
This script will continuously monitor the memory usage of your Java application. If the usage exceeds 20 MB, it will take a heap dump.
Final Considerations
jcmd is a powerful tool that provides immense capabilities for managing JVM events. In JDK 13, its features have been refined to help developers gain greater insights into application performance and health. By mastering jcmd
, you unlock the potential to proactively manage your Java applications, thereby ensuring that they operate smoothly and efficiently.
For further reading on JVM monitoring and management, refer to the official Java documentation.
Whether you are a developer looking to debug your application or a systems administrator trying to keep your production systems healthy, leveraging jcmd
will undoubtedly enhance your toolkit for managing JVM events effectively.