Mastering Pagination in Spring Data Solr: A Step-by-Step Guide
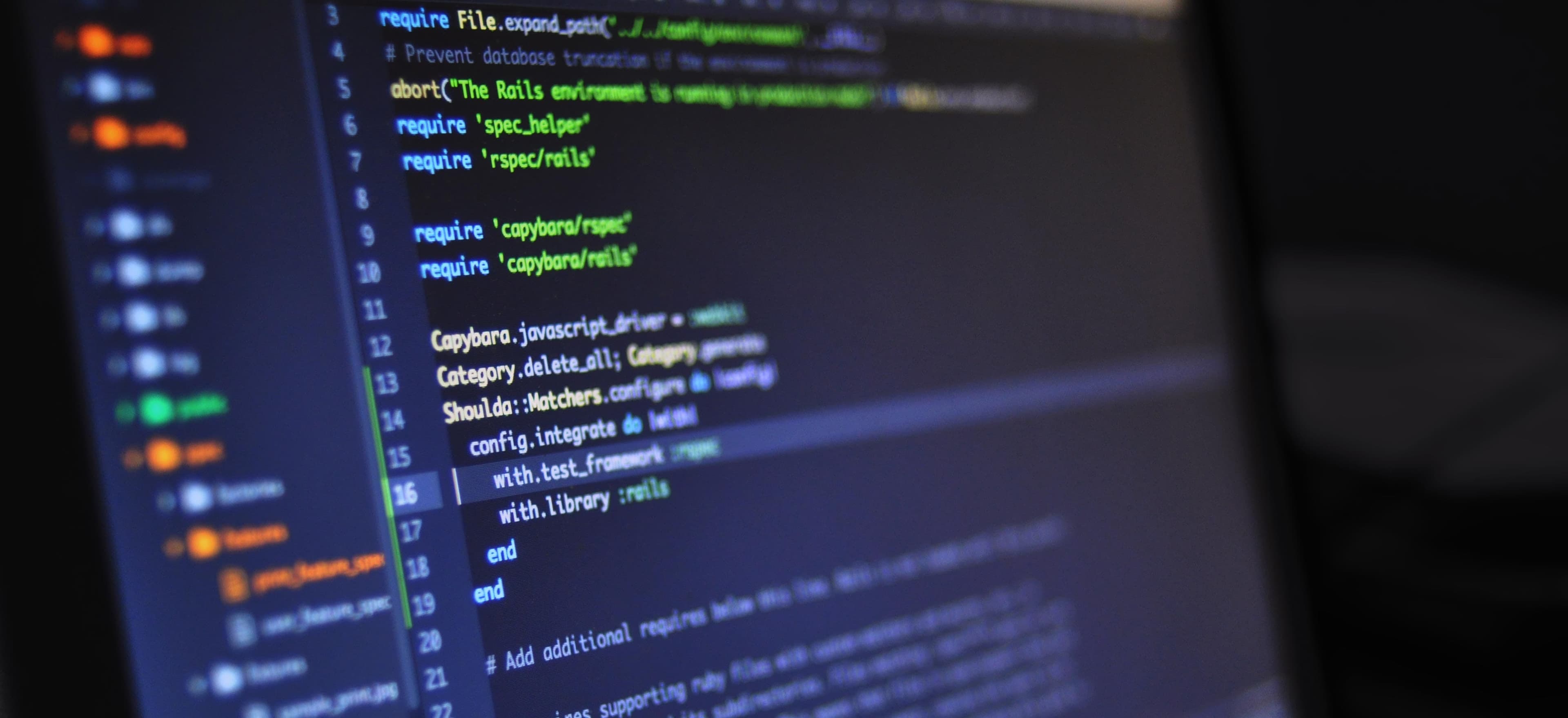
- Published on
Mastering Pagination in Spring Data Solr: A Step-by-Step Guide
Pagination is a crucial aspect of modern web applications. It enhances user experience by reducing loading times and making data more manageable. With the rise of big data, users expect seamless access to information. In this guide, we’ll explore pagination within Spring Data Solr, a powerful framework for integrating Apache Solr with Spring applications.
What is Spring Data Solr?
Spring Data Solr is a part of the Spring Data project that aims to provide a simplified data access strategy for Solr. It allows you to easily build applications that interact with Solr repositories using a familiar Spring Data programming model.
Why Use Solr for Pagination?
Apache Solr is a search platform built on Apache Lucene. It provides robust features including full-text search, faceting, distributed searching, and, importantly, pagination. When dealing with large datasets, efficient pagination is critical to maintaining performance and usability.
Understanding Pagination in Solr
In Solr, pagination can be achieved primarily through the start
and rows
parameters. The start
parameter indicates the offset (or position) from which Solr should begin returning results, while the rows
parameter specifies the number of results to return.
Example:
To fetch the second page of results, with 10 results per page, you would set:
start
= 10 (skip the first 10 results)rows
= 10 (retrieve the next 10 results)
Step-by-Step Guide to Implement Pagination in Spring Data Solr
Step 1: Set Up Your Spring Boot Project
First, create a new Spring Boot project. You can do this by using Spring Initializr to generate a base project with the necessary dependencies for Spring Data Solr.
- Select
Spring Web
,Spring Data Solr
, andSpring Boot DevTools
in the dependency section. - Download and extract the project.
Step 2: Configure Solr in Your Application
Once your project is set up, configure your Solr connection in the application.properties
file:
spring.data.solr.host=http://localhost:8983/solr
spring.data.solr.repositories.enabled=true
Here, replace the Solr host URL with your server details if necessary.
Step 3: Create Your Domain Model
Define an entity that Solr will index; for example, let's use a simple Product
class:
import org.springframework.data.annotation.Id;
import org.springframework.data.solr.core.mapping.SolrDocument;
@SolrDocument
public class Product {
@Id
private String id;
private String name;
private double price;
// Getters and Setters here
}
The @SolrDocument
annotation indicates that this class is a domain object for Solr.
Step 4: Create a Repository Interface
Next, create a repository interface for your Solr entity:
import org.springframework.data.solr.repository.SolrCrudRepository;
public interface ProductRepository extends SolrCrudRepository<Product, String> {
}
This interface extends SolrCrudRepository
and provides CRUD operations without requiring any additional code.
Step 5: Implement Pagination in Your Service
Construct a service class to handle pagination logic. Here’s how:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.domain.PageRequest;
import org.springframework.data.domain.Pageable;
import org.springframework.data.domain.Page;
import org.springframework.stereotype.Service;
@Service
public class ProductService {
@Autowired
private ProductRepository productRepository;
public Page<Product> getProducts(int page, int size) {
Pageable pageable = PageRequest.of(page, size);
return productRepository.findAll(pageable);
}
}
Here, the getProducts
method receives page
and size
values, sets up a Pageable
object, and retrieves the paginated results using Spring Data's built-in support.
Step 6: Create a Controller
Finally, set up a controller that will expose an HTTP endpoint for fetching paginated results:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.domain.Page;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class ProductController {
@Autowired
private ProductService productService;
@GetMapping("/products")
public Page<Product> getProducts(
@RequestParam(defaultValue = "0") int page,
@RequestParam(defaultValue = "10") int size) {
return productService.getProducts(page, size);
}
}
The ProductController
handles GET requests made to /products
, allowing the user to specify pagination parameters.
Step 7: Testing Your Implementation
You can use tools like Postman or simply a web browser to test your endpoint. Navigate to:
http://localhost:8080/products?page=1&size=10
This URL will return the second page of products (assuming zero-based indexing) containing 10 products.
Additional Considerations
Performance
When implementing pagination, performance can become a concern if you are not careful about the start
parameter. It's essential to maintain efficient indexing and search queries, especially with very large datasets.
Sorting
You can enhance your pagination further by allowing sort order in your application. Modify your repository method as follows:
import org.springframework.data.solr.core.query.Sort;
import org.springframework.data.solr.core.query.Query;
import org.springframework.data.solr.core.query.SimpleQuery;
public Page<Product> getProducts(int page, int size, String sortBy) {
Pageable pageable = PageRequest.of(page, size, Sort.by(sortBy));
return productRepository.findAll(pageable);
}
Now the end-user can also specify how they'd like results sorted.
The Last Word
Pagination is a vital component when retrieving data for web applications. With Spring Data Solr, implementing efficient pagination is straightforward and can lead to significant enhancements in the performance and usability of your applications.
For more detailed insights into Spring Data Solr, you can visit their official documentation to dive deeper into advanced features, querying, and configuring your Solr server.
By mastering pagination with Spring Data Solr, you not only optimize your application but also provide a better user experience. So take the plunge and implement pagination in your next project!
Feel free to leave a comment if you have questions or insights about pagination in Spring Data Solr.