Mastering JaCoCo: Avoiding Common Code Coverage Pitfalls
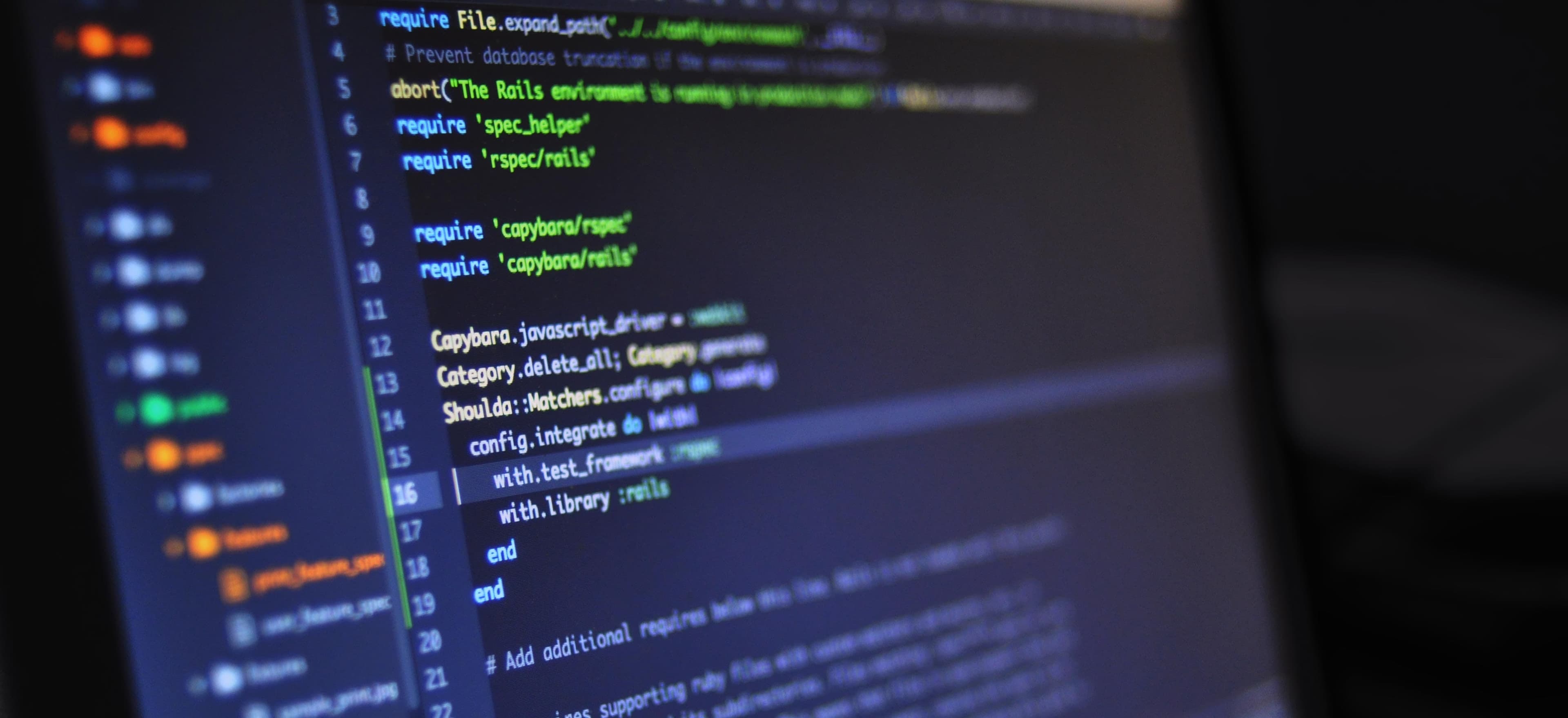
- Published on
Mastering JaCoCo: Avoiding Common Code Coverage Pitfalls
In the ever-evolving landscape of software development, ensuring the quality and reliability of your code is paramount. One way to achieve this is through code coverage, which provides insights into the percentage of your code being tested by automated tests. JaCoCo (Java Code Coverage) is a popular library used for this purpose in the Java ecosystem. However, many developers fall into common pitfalls when working with JaCoCo, which can lead to misleading results or gaps in test coverage. In this blog post, we will explore JaCoCo in-depth, helping you identify and avoid these pitfalls.
What is JaCoCo?
JaCoCo is a robust code coverage library designed for Java applications. It integrates seamlessly into build tools like Maven and Gradle and provides various reports regarding code coverage metrics, such as line coverage, branch coverage, and instruction coverage.
Key Features of JaCoCo
- Integration: Quickly integrates with various build systems like Maven, Gradle, and Ant.
- Reporting Formats: Generates different reporting formats, including HTML, XML, and CSV.
- Versatility: Works with any Java-based project, including those with complex frameworks.
- Support for Java Versions: Compatible with modern Java versions, including Java 8 and beyond.
Basic JaCoCo Setup
To get started with JaCoCo, you need to add the necessary dependencies to your project. Below are configurations for both Maven and Gradle.
Maven Setup
<dependencies>
<dependency>
<groupId>org.jacoco</groupId>
<artifactId>org.jacoco.agent</artifactId>
<version>0.8.8</version>
<scope>runtime</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.jacoco</groupId>
<artifactId>jacoco-maven-plugin</artifactId>
<version>0.8.8</version>
<executions>
<execution>
<goals>
<goal>prepare-agent</goal>
</goals>
</execution>
<execution>
<id>report</id>
<phase>test</phase>
<goals>
<goal>report</goal>
</goals>
</execution>
</executions>
</plugin>
</plugins>
</build>
Gradle Setup
plugins {
id 'jacoco'
}
jacoco {
toolVersion = "0.8.8"
}
test {
finalizedBy jacocoTestReport
}
jacocoTestReport {
reports {
xml.enabled true
html.enabled true
}
}
With JaCoCo integrated, you can now run your tests and check the coverage reports generated.
Common Pitfalls in JaCoCo Usage
While JaCoCo is a powerful tool, there are several pitfalls that developers often encounter:
1. Ignoring the Uncovered Code
One of the most common pitfalls is neglecting the uncovered code sections. Many developers may look at coverage reports and become complacent, believing that as long as their coverage percentage looks good, their code is well-tested.
Why This Matters
High coverage does not equate to well-tested code. Just because a line of code is executed does not mean it has been tested for all possible scenarios.
Example
Suppose you have a function that processes user data:
public String processUserData(User user) {
if (user != null) {
return "Processed: " + user.getName();
}
return "No user data";
}
You may have written a test that covers the scenario with a user:
@Test
public void testProcessUserDataWithValidUser() {
User user = new User("John Doe");
String result = processUserData(user);
assertEquals("Processed: John Doe", result);
}
However, if you don't test the case when user
is null
, your code is not being thoroughly tested. Always ensure that edge cases are accounted for — they are just as crucial as the 'happy path' scenarios.
2. Single Test for Multiple Scenarios
Another common issue is writing a single test case to cover multiple scenarios. While this approach may initially seem efficient, it can lead to false sense of security regarding your code coverage.
Why This Matters
If a single test fails, you may not immediately know which scenario it corresponds to, making debugging harder.
Example Code
@Test
public void testUserData() {
// Testing scenario with null
assertEquals("No user data", processUserData(null));
// Testing valid user
User user = new User("Jane Doe");
assertEquals("Processed: Jane Doe", processUserData(user));
}
Here, two scenarios are covered in one test. It is better to separate these into distinct test methods, increasing clarity:
@Test
public void testProcessUserDataWithNull() {
assertEquals("No user data", processUserData(null));
}
@Test
public void testProcessUserDataWithValidUser() {
User user = new User("Jane Doe");
assertEquals("Processed: Jane Doe", processUserData(user));
}
3. Misinterpreting Coverage Metrics
Coverage metrics are often misunderstood. For instance, a common misconception is that 100% line coverage means code quality is perfect.
Why This Matters
Achieving high code coverage does not necessarily imply that the application is free from bugs or that the tests are high quality.
Focus on Quality over Quantity
Instead of striving for a perfect score, focus on writing meaningful tests that validate your application's key functionalities comprehensively. Prioritize tests that cover critical paths in your code.
4. Neglecting Branch Coverage
Branch coverage measures whether all branches of control structures have been executed. Focusing solely on line coverage can cause you to miss logical errors.
Why This Matters
Branch coverage provides a deeper understanding of how different paths in your code perform. It's possible to have high line coverage but very low branch coverage.
Example
Consider the following method:
public String validateUserRole(String role) {
if ("admin".equals(role)) {
return "Admin Access granted.";
} else if ("user".equals(role)) {
return "User Access granted.";
}
return "No Access.";
}
Testing only one condition:
@Test
public void testAdminAccess() {
assertEquals("Admin Access granted.", validateUserRole("admin"));
}
Here, only the admin scenario is tested. You should cover every possible role, including "user" and any other non-matching string:
@Test
public void testUserAccess() {
assertEquals("User Access granted.", validateUserRole("user"));
}
@Test
public void testNoAccess() {
assertEquals("No Access.", validateUserRole("guest"));
}
5. Dependency on Code Coverage Tools
Some developers overly depend on tools like JaCoCo to ensure code quality. This can lead to the neglect of best practices in testing.
Why This Matters
Tools are just aids; they don't replace the necessity for robust testing strategies. Relating testing goals explicitly with business logic should remain at the forefront of development efforts.
Lessons Learned
While JaCoCo is an excellent tool for measuring code coverage, it is essential to be aware of common pitfalls that can lead to misleading results. Focus on writing meaningful tests that cover both the happy path and edge cases, and ensure your coverage metrics are interpreted correctly.
Strive for quality in your tests over quantity. Remember, a high coverage percentage does not equate to well-tested code, and it is your responsibility to ensure your application meets the highest standards of quality.
For more resources on JaCoCo and code coverage in Java, visit the JaCoCo Documentation.
By mastering these principles and avoiding common mistakes, you can leverage JaCoCo effectively and ensure your Java applications are robust and well-tested. Happy coding!
Checkout our other articles