Maximize Your Goal Achievement with Performance Tuning Tips
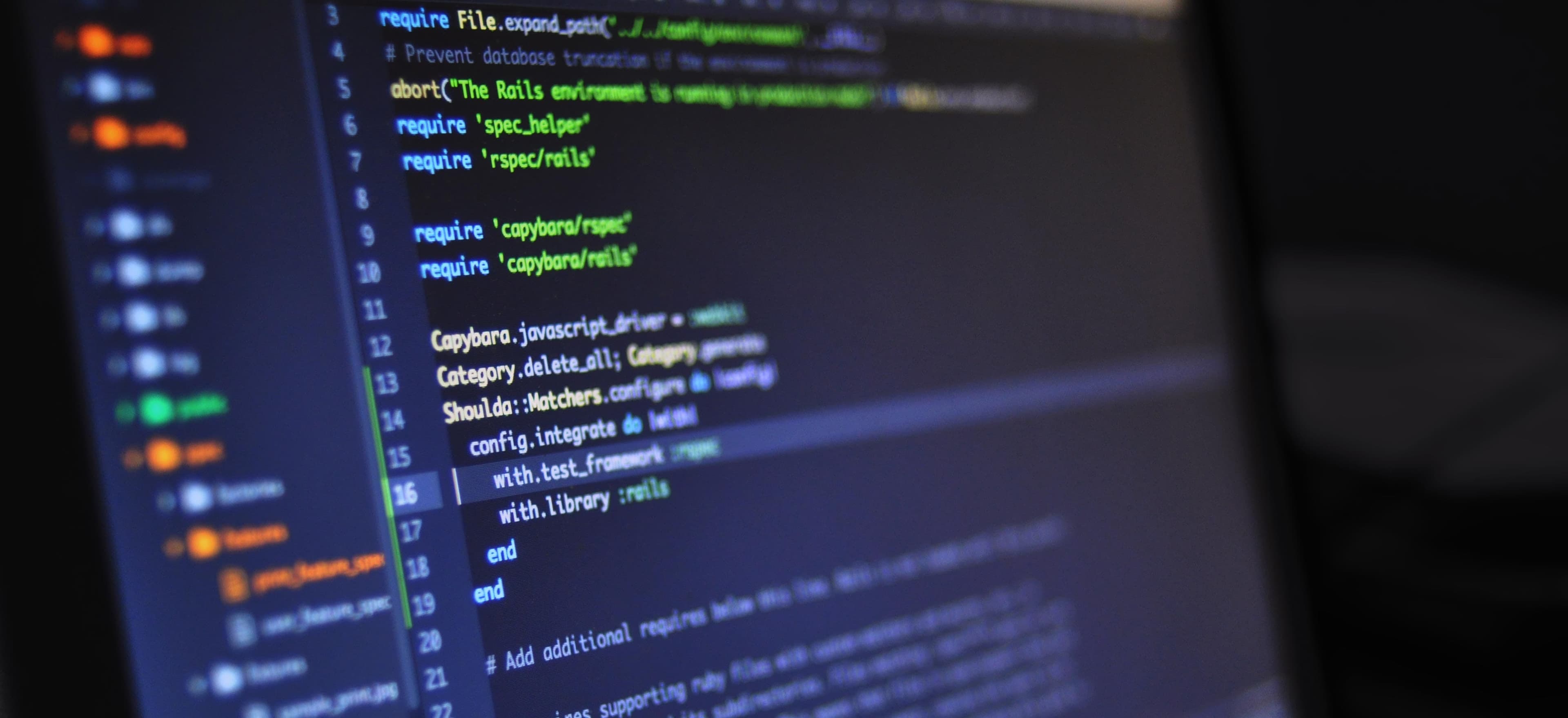
- Published on
Maximize Your Goal Achievement with Performance Tuning Tips
Performance tuning is an essential part of software optimization that directly impacts the effectiveness and efficiency of applications. Whether you are a beginner stepping into the world of coding or an experienced developer looking to enhance your skills, mastering performance tuning techniques in Java can significantly improve your application's overall performance and user experience. This comprehensive guide will walk you through the most effective strategies for optimizing performance in Java applications.
Understanding Performance Tuning
Before diving into the specifics, it's crucial to grasp what performance tuning entails. It refers to the process of optimizing a system's performance (be it hardware or software) to enhance response times, throughput, and overall efficiency. In the context of Java, this may involve:
- Reducing response times
- Minimizing resource consumption
- Increasing scalability
Here’s a simple analogy: Imagine your Java application as a racing car. Performance tuning is akin to adjusting the engine tuning, optimizing the aerodynamics, and fine-tuning the tire pressure. Every adjustment you make aims to enhance speed and handling, just as tuning aims to enhance the performance of your code.
Key Areas of Focus for Performance Tuning
- Algorithm Optimization
- Garbage Collection Management
- Thread Management
- I/O Optimization
- Database Optimization
- Profiling and Monitoring Tools
Let’s explore each area in depth.
1. Algorithm Optimization
The choice of algorithm can have a significant impact on performance. Choosing efficient algorithms is essential for improving the speed and responsiveness of your application.
Example of Algorithm Improvement:
Consider a simple problem: finding the maximum number in an array.
public int findMax(int[] array) {
int max = array[0];
for (int i = 1; i < array.length; i++) {
if (array[i] > max) {
max = array[i];
}
}
return max;
}
In the example above, the time complexity is O(n), which means the performance scales linearly with the input size. If we intentionally choose a less efficient algorithm, such as using nested loops, performance deteriorates dramatically to O(n^2).
public int findMaxWithNestedLoops(int[] array) {
int max = Integer.MIN_VALUE;
for (int i = 0; i < array.length; i++) {
for (int j = 0; j < array.length; j++) {
if (array[j] > max) {
max = array[j];
}
}
}
return max;
}
Why Optimize Algorithms?
Optimizing algorithms can vastly improve performance, especially with large data sets. It is crucial to revisit algorithms as application requirements change to maintain efficiency.
2. Garbage Collection Management
Java uses automatic garbage collection (GC) to manage memory, which is both a blessing and a curse. While it alleviates memory management burdens from developers, improper management can lead to performance bottlenecks.
Choosing the Right Garbage Collector
There are several GC implementations in Java, such as:
- Serial Garbage Collector
- Parallel Garbage Collector
- Concurrent Mark-Sweep (CMS)
- G1 Garbage Collector
Choosing the appropriate collector for your application can minimize pauses and optimize memory management based on the application's workload.
// For example, to enable the G1 Garbage Collector during JVM startup
-XX:+UseG1GC
Why is GC Important?
Efficient garbage collection settings can significantly reduce the time spent on memory management, thereby avoiding long pauses in applications where performance is critical.
3. Thread Management
Java provides a rich set of concurrency utilities, which can be both powerful and tricky to manage. Poor thread management can lead to contention, deadlocks, and other performance issues.
Use of Executors
Instead of managing threads manually, using the ExecutorService
can streamline the process.
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
ExecutorService executor = Executors.newFixedThreadPool(10);
executor.submit(() -> {
// Task implementation
});
executor.shutdown();
Why Use Executors?
Utilizing ExecutorService
reduces the complexities associated with thread creation and offers better resource management. This means you can focus more on business logic rather than worrying about thread lifecycle intricacies.
4. I/O Optimization
Input/Output operations are often slow. By minimizing I/O operations and using buffered streams, you can significantly boost performance.
import java.io.*;
public void readFileWithBuffering(String fileName) {
try (BufferedReader reader = new BufferedReader(new FileReader(fileName))) {
String line;
while ((line = reader.readLine()) != null) {
// Process the line
}
} catch (IOException e) {
e.printStackTrace();
}
}
Why Buffering?
Buffering reads larger chunks of data at once, reducing the number of I/O operations and enhancing performance.
5. Database Optimization
Database interactions often provide the most significant bottlenecks in an application. Consider:
- Using prepared statements to minimize query compilation overhead.
- Indexing your database tables effectively to speed up search operations.
String sql = "SELECT * FROM users WHERE email = ?";
try (PreparedStatement pstmt = connection.prepareStatement(sql)) {
pstmt.setString(1, userEmail);
ResultSet rs = pstmt.executeQuery();
// Process results
}
Why Use Prepared Statements?
They reduce the parsing time involved in executing queries and also enhance security by preventing SQL injection attacks.
6. Profiling and Monitoring Tools
Every performance tuning effort needs to be backed by data. Profiling tools provide insights into where the time is being utilized within your application.
Recommended Tools
- VisualVM: An all-in-one tool for monitoring, troubleshooting, and profiling Java applications.
- Java Mission Control: Designed for monitoring and profiling Java applications in production.
Using these tools, you can identify hotspots and bottlenecks in your code, enabling targeted optimization efforts.
The Last Word
Performance tuning in Java is a multifaceted endeavor aimed at enhancing the responsiveness and efficiency of applications. Understanding the nuances of algorithm optimization, effective garbage collection, thread management, I/O optimization, and database interaction can significantly boost your application's performance.
By utilizing profiling tools, you can continually monitor and refine your strategies, ensuring that your application not only meets but exceeds user expectations.
For further reading on optimizing Java applications, check out Java Performance Tuning and learn more about Java Concurrency in Practice. Dive into these resources to deepen your understanding of performance tuning strategies and elevate your Java skills to the next level.