Taming the Beast: How to Improve Legacy Code Quality
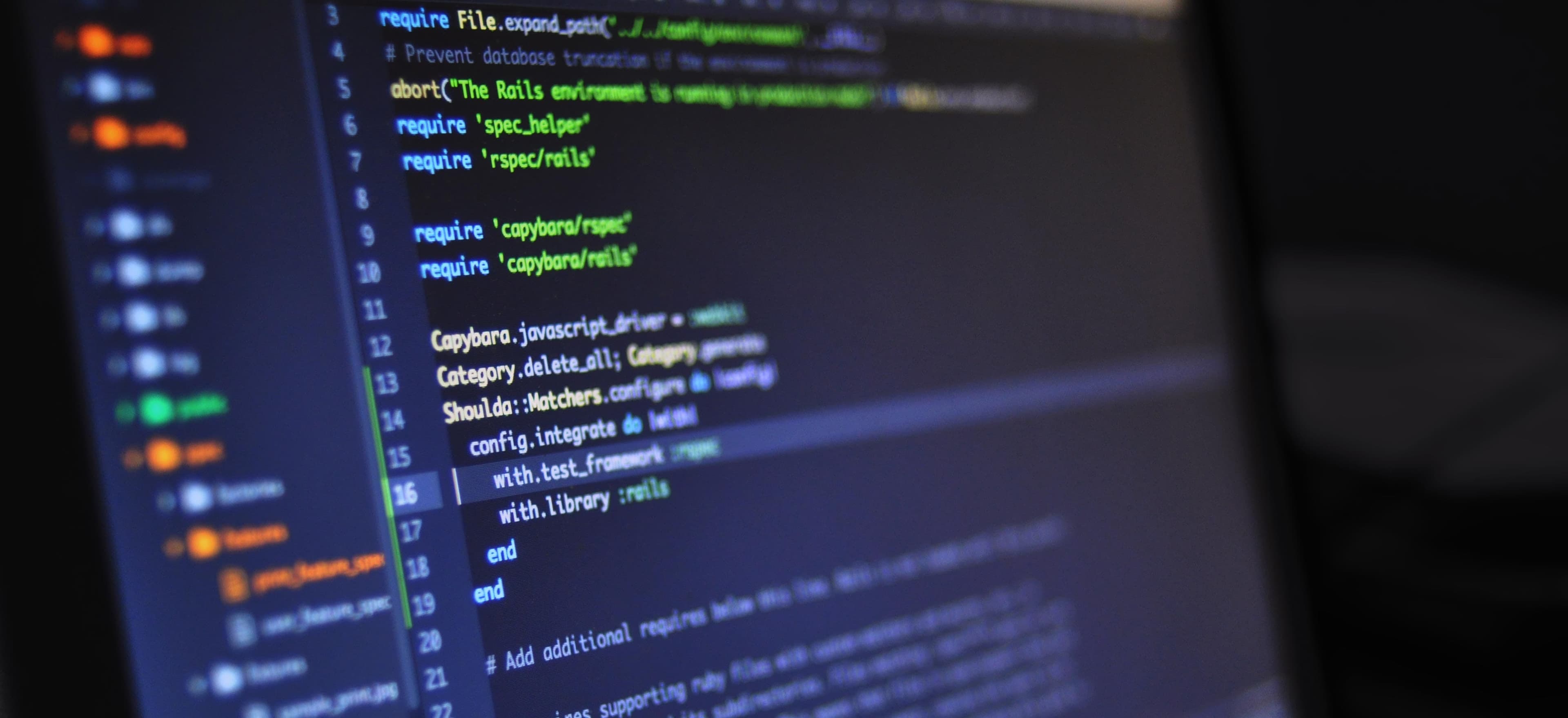
- Published on
Taming the Beast: How to Improve Legacy Code Quality
Legacy code can often feel like a beast that has gotten out of control. This complex, outdated code can be daunting for developers, especially when there’s little to no documentation available. Yet, improving the quality of legacy code is pivotal for maintaining the integrity of software applications. By adopting systematic approaches, developers can tame this code beast effectively.
In this blog post, we will explore various techniques to improve legacy code quality. We will unravel strategies including refactoring, automated testing, and code reviews, backed by Java code snippets to illustrate best practices. Let’s dive in.
Understanding Legacy Code
Before we delve into improving legacy code, it's essential to understand what constitutes legacy code. The phrase was popularized by Michael Feathers in his book on legacy code. Feathers defined legacy code as any code without tests. Essentially, if it’s hard to understand and modify, it's often deemed as "legacy."
Common Issues with Legacy Code
- Lack of Documentation: Code that predates current standards often lacks sufficient documentation, making it hard for new developers to grasp.
- Tightly Coupled Components: Components are often so intertwined that modifying one causes cascading effects across the application.
- Obsolete Technologies: Legacy code may rely on outdated libraries or frameworks, which can introduce security vulnerabilities.
Each of these issues can create significant hurdles. However, by applying best practices tailored for Java development, we can not only improve code quality but also enhance maintainability.
Refactoring: The Path to Quality
Refactoring is the process of restructuring existing code without changing its external behavior. The goal is to improve the code's structure, readability, and maintainability. Here’s how to approach refactoring legacy Java code:
1. Identify Code Smells
Code smells are indicators of potential problems in the code. Common Java code smells include large classes, long methods, and duplicated code.
Example: Extracting a Method
public class OrderProcessor {
public void processOrder(Order order) {
// Validation goes here
if (order.isValid()) {
// Processing logic here...
sendConfirmation(order);
}
}
private void sendConfirmation(Order order) {
// Sending confirmation logic...
}
}
In the above snippet, we refactored the processOrder
method by extracting the confirmation logic into a separate method. This makes processOrder
cleaner and emphasizes its primary purpose.
2. Use Meaningful Names
Naming is crucial. Meaningful and descriptive names make your code self-documenting.
Example: Improving Variable Names
public class EmployeeManager {
public void manageEmp(int empId, String empName) {
// Business logic...
}
}
Can be refactored into:
public class EmployeeManager {
public void manageEmployee(int employeeId, String employeeName) {
// Business logic...
}
}
Here, changing empId
to employeeId
and empName
to employeeName
makes it clear what the method parameters represent.
3. Extract Classes and Interfaces
When classes become too large or complex, consider breaking them into smaller, more manageable pieces.
Example: Extracting a Class
public class Report {
public void generate() {
// Logic to generate a report
}
public void print() {
// Logic to print a report
}
}
This can be refactored into:
public class Report {
public void generate() {
// Logic to generate a report
}
}
public class ReportPrinter {
public void print(Report report) {
// Logic to print a report
}
}
By separating concern, you can improve both readability and testability.
Leveraging Automated Testing
Testing is the safety net that allows you to refactor with confidence. Implementing automated tests is particularly important when dealing with legacy code.
1. Write Unit Tests
When encountering legacy code, your first priority should be to write unit tests for existing functionalities. This can help uncover bugs while providing a safety mechanism as you refactor.
Example: JUnit Test Case
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.*;
class OrderProcessorTest {
@Test
void testProcessOrder_ValidOrder() {
Order order = new Order(true);
OrderProcessor processor = new OrderProcessor();
processor.processOrder(order);
// Assertions to verify behavior
}
}
With this test case, you can verify that your OrderProcessor
behaves correctly without breaking existing functionality.
2. Use Test-Driven Development (TDD)
If you’re introducing new features or modules, consider using TDD. Write the test first, followed by the implementation.
Example: TDD Implementation
- Write a failing test first:
@Test
void testCalculateDiscount() {
Order order = new Order();
order.addItem(new Item(100.0));
double discount = order.calculateDiscount();
assertEquals(10.0, discount);
}
- Implement the feature until the test passes:
public class Order {
private List<Item> items = new ArrayList<>();
public void addItem(Item item) {
items.add(item);
}
public double calculateDiscount() {
// assume 10% discount
return items.stream().mapToDouble(Item::getPrice).sum() * 0.1;
}
}
By following this cycle, you ensure the feature is well-tested, and you slowly built a robust codebase.
Code Reviews: The Additional Layer
A thorough code review process adds a significant layer of quality to legacy systems. Engaging with peers can yield invaluable insights and collaborative improvement.
1. Engage Peers
Encourage developers to review each other’s code. Look for areas where legacy practices can be improved. This encourages a culture of learning and teaches best practices.
2. Use Automated Tools
Consider leveraging static analysis tools. Tools like SonarQube or Checkstyle can catch common issues before code is reviewed, saving time and effort.
Final Considerations: Your Roadmap Ahead
Improving legacy code quality is a journey that requires strategic thinking, planning, and a systematic approach. It is a process enriched by teamwork and the incorporation of testing at all stages.
Refactoring, leveraging automated testing, and reinforcing code reviews are pivotal components of that process. As much as it may feel like the beast cannot be tamed, patience and persistence will yield results over time.
By implementing the strategies discussed in this blog post, you will not only enhance the quality of your legacy code but also lay down a robust foundation for future enhancements.
Remember: an investment in code quality today will save you time, money, and headaches down the road.
For more insights on software development best practices, check out Java Code Geeks and Stack Overflow.
Happy coding!