Java vs Native Agents: Understanding Performance Trade-offs
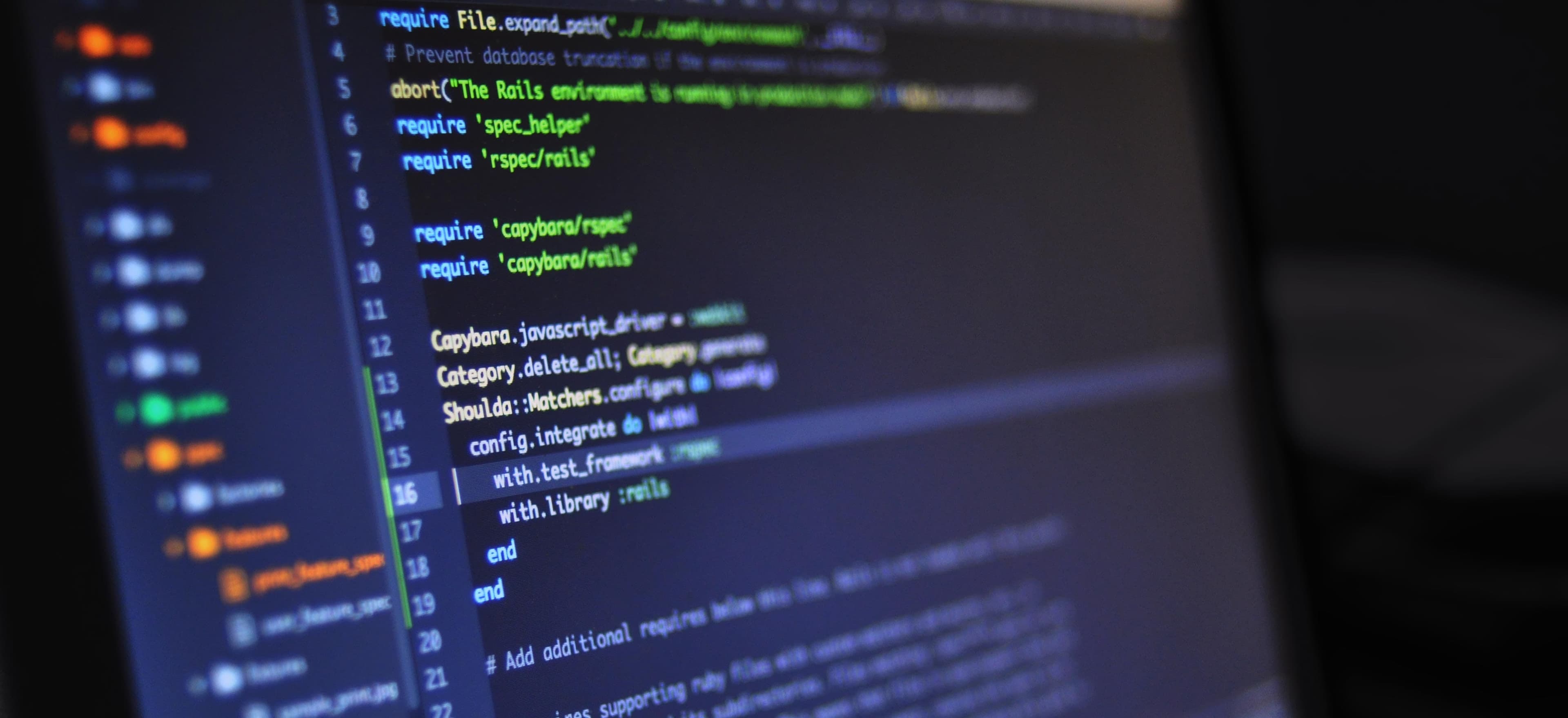
- Published on
Java vs Native Agents: Understanding Performance Trade-offs
Java is a robust, versatile, and widely used programming language. Its platform independence and vast ecosystem make it a strong contender for various applications, from simple console programs to complex enterprise-level systems. However, what happens when performance is a critical factor? Enter native agents, a term that often stirs debate among developers and system architects. In this article, we will delve into performance trade-offs between Java and native agents, examining the strengths and weaknesses of each approach.
Table of Contents
- What are Native Agents?
- The Java Ecosystem
- Performance Comparison
- Use Cases for Java vs Native Agents
- Making the Right Choice
- Conclusion
What are Native Agents?
Native agents refer to programs that are executed natively by the operating system. These programs are often written in languages like C or C++, which are closer to the machine code. Because of this, native agents can sometimes perform operations more quickly than their Java counterparts.
For instance, developing a performance-intensive application that manipulates large datasets might benefit significantly from a native agent. The direct interaction with system resources can yield a much faster execution time, especially in applications that require low-level memory management.
The Java Ecosystem
Java is well-regarded for its "write once, run anywhere" philosophy due to its built-in Just-In-Time (JIT) compiler and robust Garbage Collection (GC) mechanisms. Here are some advantages Java brings to the table:
- Platform Independence: Java programs compile to bytecode, which the Java Virtual Machine (JVM) can execute on any machine with a compatible JVM.
- Memory Management: Java's garbage collection handles memory allocation and deallocation automatically, which helps prevent memory leaks.
- Rich Libraries: The Java ecosystem boasts a wealth of libraries and frameworks, such as Spring and Hibernate, to accelerate development.
Yet, these features come with a performance cost. The additional layers of abstraction in Java can create overhead that slows down execution speed compared with native applications.
Performance Comparison
Performance is a multifaceted concept, comprising aspects like execution speed, memory footprint, and resource management. Here’s how Java compares to native agents in each of these categories:
Execution Speed
While Java has advanced over the years and the JIT compiler makes bytecode execution faster than ever, it still may not match the raw execution speed of native code.
// Java example: Quick sort algorithm
public class QuickSort {
public static void quickSort(int[] array, int low, int high) {
if (low < high) {
int pivotIndex = partition(array, low, high);
quickSort(array, low, pivotIndex - 1);
quickSort(array, pivotIndex + 1, high);
}
}
private static int partition(int[] array, int low, int high) {
int pivot = array[high];
int i = low - 1;
for (int j = low; j < high; j++) {
if (array[j] <= pivot) {
i++;
// Swap array[i] and array[j]
int temp = array[i];
array[i] = array[j];
array[j] = temp;
}
}
// Swap array[i + 1] and array[high] (or pivot)
int temp = array[i + 1];
array[i + 1] = array[high];
array[high] = temp;
return i + 1;
}
}
In the above Java example, while the code is readable and concise, executing a quick sort on a large dataset may not be as fast as its native counterpart written in C.
Memory Footprint
Java consumes more memory due to the JVM's runtime requirements and the overhead of garbage collection. Native agents generally have smaller memory footprints because they don’t require a runtime environment.
Resource Management
Java’s automatic memory management is a double-edged sword. On the one hand, it reduces the risk of memory leaks; on the other hand, it introduces pauses due to garbage collection. Native agents offer more precise control over memory management, allowing developers to optimize resource allocation based on specific needs.
Use Cases for Java vs Native Agents
Java Use Cases
- Web Applications: Java's inherent scalability and ecosystem (like Spring Boot) make it ideal for web applications.
- Enterprise Applications: Organizations with complex business logic and requirements often prefer Java for its robust architecture.
Native Agent Use Cases
- Performance-Critical Applications: Applications in finance, gaming, or image processing often use native agents for speed.
- Device Drivers and Embedded Systems: Here, low-level access to hardware and performance is paramount.
Making the Right Choice
Understanding your project's needs is crucial when deciding between Java and native agents. Consider the following factors:
- Performance Requirements: If speed is the utmost priority and the application interacts closely with hardware, you might lean towards native agents.
- Development Speed and Maintenance: Java can significantly reduce development time through its vast libraries and ease of use.
- Team Expertise: If your team excels in Java, it may be better to leverage their strengths, even if native code offers performance benefits.
The Bottom Line
In the ongoing debate between Java and native agents, it’s essential to recognize that both have their pros and cons. While Java offers significant benefits in terms of portability and development speed, native agents may offer superior performance for specific use cases. Ultimately, the choice should be guided by the unique requirements of your project.
Remember, just because one technology excels in a particular area does not mean it is suitable for every application. Always align your choice with your project goals, team skills, and performance benchmarks. By making informed decisions, you can harness the strength of both Java and native agents effectively.
For more insights on Java development and performance optimization, check out Oracle's Java Tutorials and Performance Tuning Guide.
Whether you're team Java or team Native, the world of programming offers countless tools to fit any challenge. Explore, experiment, and find what works best for you!