Overcoming Common Challenges in RabbitMQ Integration with Spring
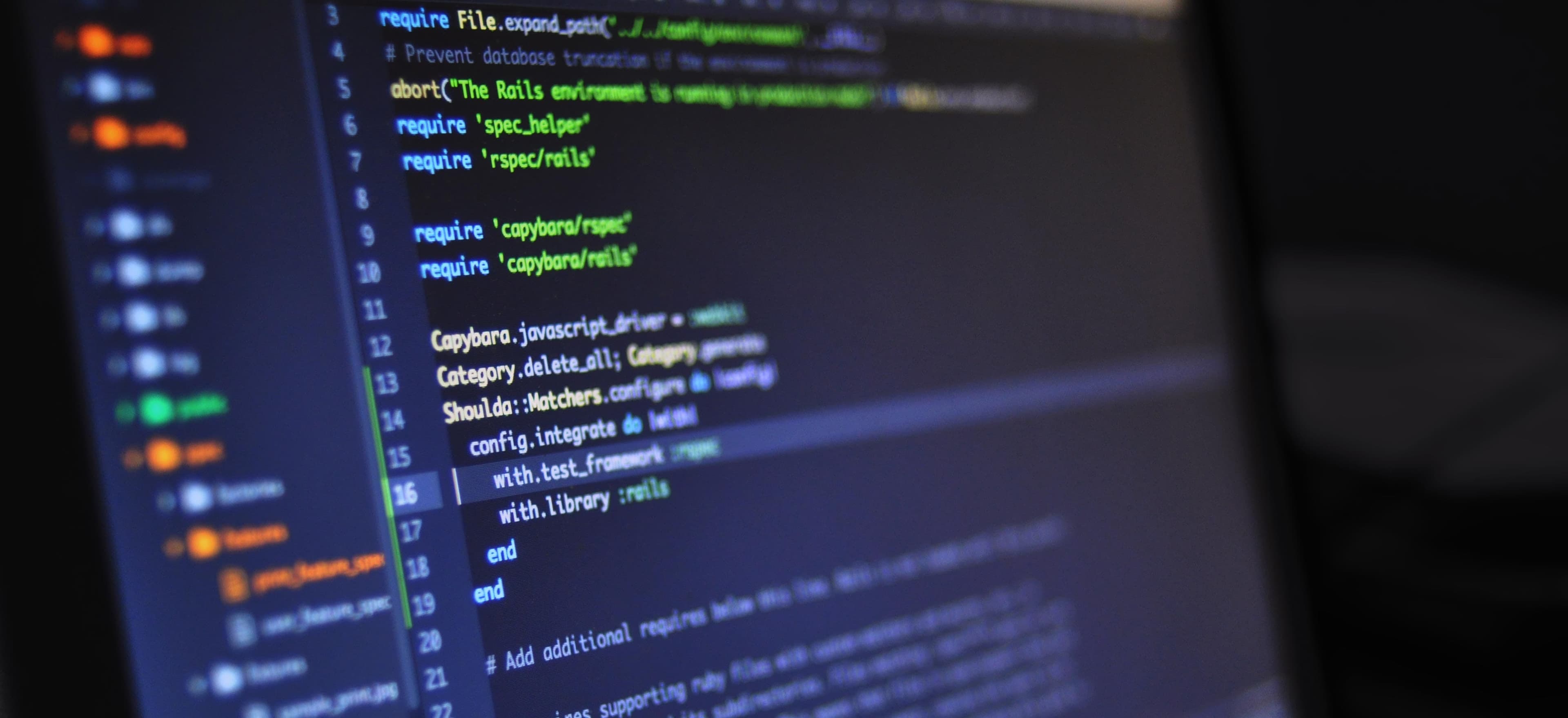
- Published on
Overcoming Common Challenges in RabbitMQ Integration with Spring
RabbitMQ is a robust messaging broker widely used in microservices architecture for message queuing. Integrating RabbitMQ with Spring can significantly improve the scalability and reliability of your applications. However, developers often face common challenges during this integration. In this blog post, we'll explore these challenges and offer solutions, equipping you with the knowledge to integrate RabbitMQ seamlessly with Spring.
Table of Contents
- Understanding RabbitMQ and Spring Integration
- Common Challenges Encountered
- Best Practices for Integration
- Example Project Setup
- Conclusion
Understanding RabbitMQ and Spring Integration
RabbitMQ is an open-source message broker that uses the Advanced Message Queuing Protocol (AMQP). In contrast, Spring Framework provides powerful abstractions to interact with RabbitMQ smoothly. The Spring AMQP subproject encapsulates the RabbitMQ client and simplifies integration.
Using RabbitMQ allows your applications to handle asynchronous communications, promoting a decoupled and highly scalable design. By integrating RabbitMQ with Spring, you can utilize its Beans and Annotations to simplify messaging patterns and reduce boilerplate code.
Common Challenges Encountered
Misconfiguration Issues
One of the most common challenges in RabbitMQ integration is misconfiguration. This can arise due to incorrect settings, which can lead to runtime issues.
Solution
Make sure your RabbitMQ settings in the application.properties
file are correctly defined:
spring.rabbitmq.host=localhost
spring.rabbitmq.port=5672
spring.rabbitmq.username=user
spring.rabbitmq.password=pass
Why It's Important: Simple typos or incorrect ports can prevent your application from connecting to RabbitMQ. Always ensure the configuration matches the RabbitMQ installation.
Message Serialization/Deserialization
Another frequent challenge is the serialization and deserialization of messages. RabbitMQ sends messages as bytes, which may lead to issues with data interpretation.
Solution
Spring AMQP provides built-in support for various serialization mechanisms. You can define a custom message converter for your needs. Here’s how to set up a Jackson JSON message converter:
import org.springframework.amqp.support.converter.Jackson2JsonMessageConverter;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class RabbitConfig {
@Bean
public Jackson2JsonMessageConverter jsonMessageConverter() {
return new Jackson2JsonMessageConverter();
}
}
Why It's Important: Using the appropriate message converter ensures that your sender and receiver can communicate seamlessly, regardless of the data structure.
Error Handling
Errors during message processing can lead to a loss of valuable information. RabbitMQ clients may silently handle exceptions or drop messages, leading to data loss.
Solution
Spring offers various error handling strategies, such as ErrorHandler
and MessageListener
. You can implement a custom error handler to manage exceptions effectively.
import org.springframework.amqp.rabbit.listener.api.ChannelAwareMessageListener;
import com.rabbitmq.client.Channel;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class CustomMessageListener implements ChannelAwareMessageListener {
private static final Logger logger = LoggerFactory.getLogger(CustomMessageListener.class);
@Override
public void onMessage(Message message, Channel channel) throws Exception {
try {
// Process the message
String msgBody = new String(message.getBody(), StandardCharsets.UTF_8);
logger.info("Received message: " + msgBody);
// Process the message...
channel.basicAck(message.getMessageProperties().getDeliveryTag(), false);
} catch (Exception e) {
logger.error("Error processing message: " + e.getMessage());
channel.basicNack(message.getMessageProperties().getDeliveryTag(), false, true);
}
}
}
Why It's Important: Implementing proper error handling ensures that messages are either processed or appropriately retried. This can be critical for maintaining system integrity.
Scaling Issues
As applications grow, performance bottlenecks can arise, particularly regarding message throughput.
Solution
Consider implementing consumer scaling. Spring’s SimpleMessageListenerContainer
is designed to facilitate concurrent message consumption from RabbitMQ.
import org.springframework.amqp.rabbit.listener.SimpleMessageListenerContainer;
import org.springframework.amqp.rabbit.listener.adapter.MessageListenerAdapter;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class RabbitConfig {
@Autowired
private MessageListener myMessageListener;
@Bean
public SimpleMessageListenerContainer messageListenerContainer(ConnectionFactory connectionFactory) {
SimpleMessageListenerContainer container = new SimpleMessageListenerContainer();
container.setConnectionFactory(connectionFactory);
container.setQueueNames("myQueue");
container.setMessageListener(new MessageListenerAdapter(myMessageListener));
container.setConcurrentConsumers(5); // Scale up
container.setMaxConcurrentConsumers(10); // Max scaling
return container;
}
}
Why It's Important: Properly utilizing concurrency can drastically improve throughput and application responsiveness, especially when dealing with heavy workloads.
Best Practices for Integration
- Regular Monitoring: Always monitor RabbitMQ's performance and connectivity to address potential bottlenecks or downtimes.
- Configure Timeouts: Set appropriate connection and communication timeouts in your configuration.
- Use Fanout/Direct Exchanges Wisely: Depending on your use case, leverage the right type of exchange to optimize message routing.
- Implement Idempotency: This prevents issues that can arise from reprocessing messages in case of retries or failures.
Example Project Setup
To demonstrate RabbitMQ and Spring integration, you may want to set up a simple Spring Boot project with RabbitMQ.
-
Create a Spring Boot Application: Use Spring Initializr and include dependencies for Spring Web and Spring AMQP.
-
Add RabbitMQ Dependency: Ensure you have the RabbitMQ dependency in your
pom.xml
:<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-amqp</artifactId> </dependency>
-
Configure RabbitMQ: Ensure you have the RabbitMQ server running and correctly configure your application properties.
-
Implement Messaging Logic: Write producer and consumer code as outlined in the sections above, including error handling and custom message conversion.
-
Run Your Application: Start your Spring Boot application and verify that messages are being sent and received correctly.
Final Considerations
Integrating RabbitMQ with Spring can streamline your message-driven architecture and significantly enhance your application's efficiency. By understanding and overcoming common challenges such as configuration issues, serialization problems, error handling, and scaling, you can harness the full power of these technologies.
Consider implementing the best practices discussed and regularly review your integration to ensure optimal performance. For a more in-depth understanding of RabbitMQ functionalities, refer to the RabbitMQ documentation and Spring AMQP guide.
Now it's your turn! What challenges have you faced in RabbitMQ integration? Share your thoughts in the comments below!