Troubleshooting Common Issues in Spring SOAP Web Services
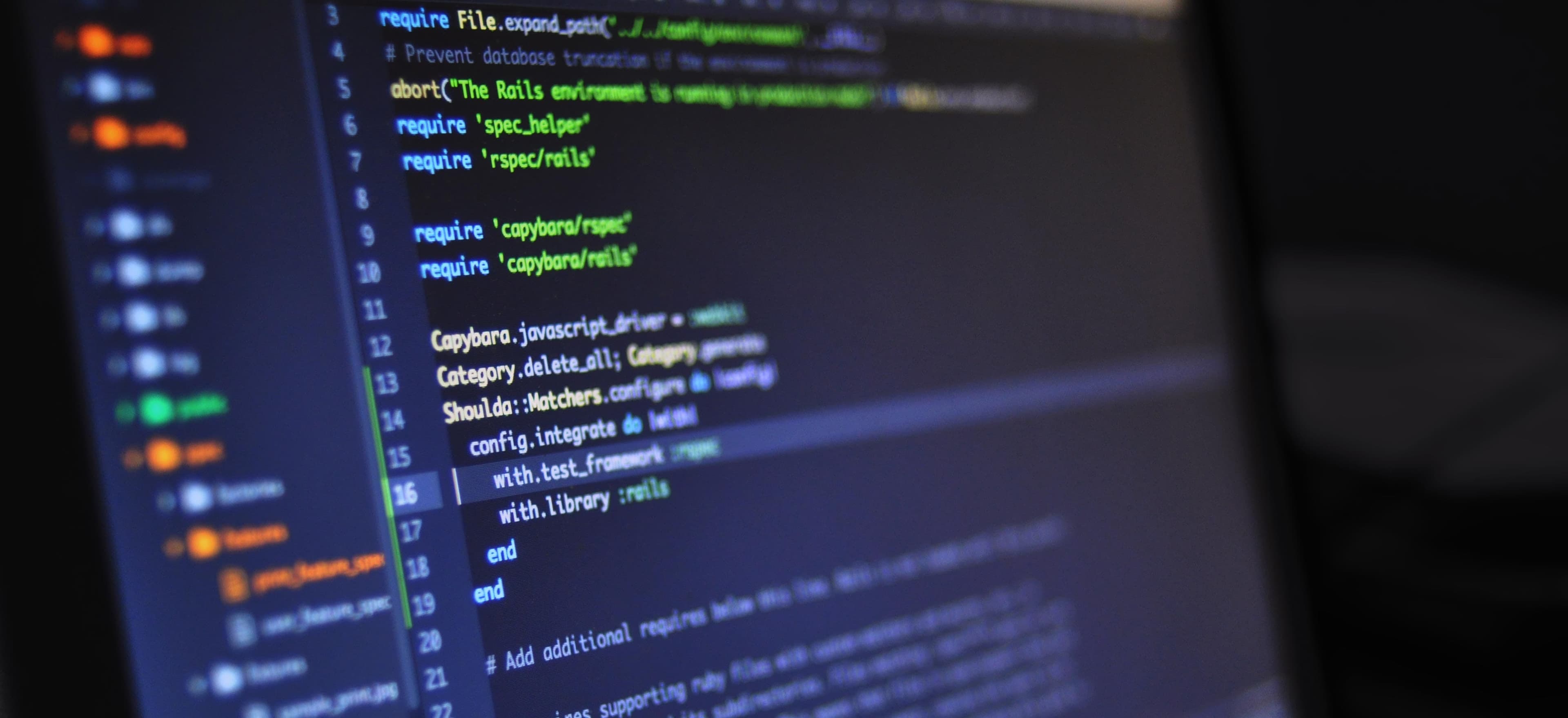
- Published on
Troubleshooting Common Issues in Spring SOAP Web Services
Spring Web Services (Spring-WS) is a powerful framework that facilitates the creation and consumption of SOAP-based web services. While it offers numerous advantages, developers often encounter challenges during development and deployment. This blog post will outline common issues in Spring SOAP web services and provide actionable solutions.
Table of Contents
- Understanding SOAP Web Services
- Setting Up Your Environment
- Common Issues in Spring SOAP Web Services
- Summary
Understanding SOAP Web Services
SOAP (Simple Object Access Protocol) is a protocol for exchanging structured information in web services. It relies on XML and offers features such as:
- Interoperability: SOAP web services can work across different programming platforms.
- Security: SOAP provides standards for message integrity and confidentiality.
In Spring, you can build SOAP web services using the spring-ws
module, which allows you to define message contracts and handle requests effortlessly.
Setting Up Your Environment
Before you start troubleshooting, ensure that your development environment is correctly configured. Here are some key components you'll need:
- Java Development Kit (JDK): Make sure you have JDK 8 or above installed.
- Spring Framework: The latest version of Spring (5+ recommended) should be included in your project.
- Spring Web Services: Add the Spring WS dependencies in your
pom.xml
if you are using Maven:
<dependency>
<groupId>org.springframework.ws</groupId>
<artifactId>spring-ws-core</artifactId>
<version>3.0.10</version>
</dependency>
This dependency is essential to use Spring Web Services functionalities in your project.
Common Issues in Spring SOAP Web Services
WSDL Validation Errors
Problem: You may encounter validation errors while testing your SOAP service.
Solution: Ensure that your WSDL file is correctly defined. A common mistake is not adhering to the XSD schema. Use tools like SoapUI to validate your WSDL against the provided XSD.
Example:
<wsdl:definitions ...>
<wsdl:types>
<xsd:schema>
<xsd:element name="YourRequestType" type="xsd:...
</xsd:schema>
</wsdl:types>
</wsdl:definitions>
Why: This section defines the message format and helps SOAP clients understand how to communicate with the service.
Incorrect Endpoint URLs
Problem: Clients cannot access your SOAP service due to incorrect endpoint URLs.
Solution: Verify that your @Endpoint
annotation is correctly configured. For example:
@Endpoint
public class YourServiceEndpoint {
private static final String NAMESPACE_URI = "http://example.com/your-service";
@PayloadRoot(namespace = NAMESPACE_URI, localPart = "YourRequest")
@ResponsePayload
public YourResponse handleRequest(@RequestPayload YourRequest request) {
YourResponse response = new YourResponse();
// handle business logic
return response;
}
}
You should ensure that your client is using the correct URL to access the service. Often, the endpoint URL should be the following pattern:
http://<server>:<port>/spring-ws/<context-path>
Why: The @PayloadRoot annotation specifies which request should be handled. If the client sends a request that does not match this, it will receive a 404 error.
Faulty XML Payloads
Problem: You receive a SOAP Fault
due to incorrect XML payloads.
Solution: Double-check the XML structure that you are sending in your request. Use an XML validator to compare against the expected structure defined in your WSDL.
Example:
<soapenv:Envelope xmlns:soapenv="http://schemas.xmlsoap.org/soap/envelope/" xmlns:ex="http://example.com/your-service">
<soapenv:Header/>
<soapenv:Body>
<ex:YourRequest>
<ex:field1>YourValue</ex:field1>
<ex:field2>YourValue</ex:field2>
</ex:YourRequest>
</soapenv:Body>
</soapenv:Envelope>
Why: If the XML does not match the expected schema, the service cannot process the request properly, leading to errors.
Authentication Issues
Problem: Authentication failures while accessing secured SOAP services.
Solution: Ensure that your Spring configuration is set up for the type of authentication you are using, be it Basic Authentication, WS-Security, or another method.
For Basic Authentication in Spring WS, you might configure it as follows:
<http:security>
<http:basic-authentication/>
</http:security>
Why: Properly managing authentication is crucial for maintaining the integrity and security of your web services.
Summary
Spring SOAP web services offer a robust framework for building and consuming SOAP-based applications. However, as with any technology, challenges may arise. By understanding common issues—such as WSDL validation, endpoint configurations, XML payloads, and authentication—you can mitigate potential pitfalls and develop more effective services.
For further reading, check out the official Spring WS Documentation to deepen your understanding.
If you have experienced other issues not covered in this post or have additional tips, please share them in the comments below. Happy coding!