Performance Showdown: Sort vs ParallelSort in Arrays
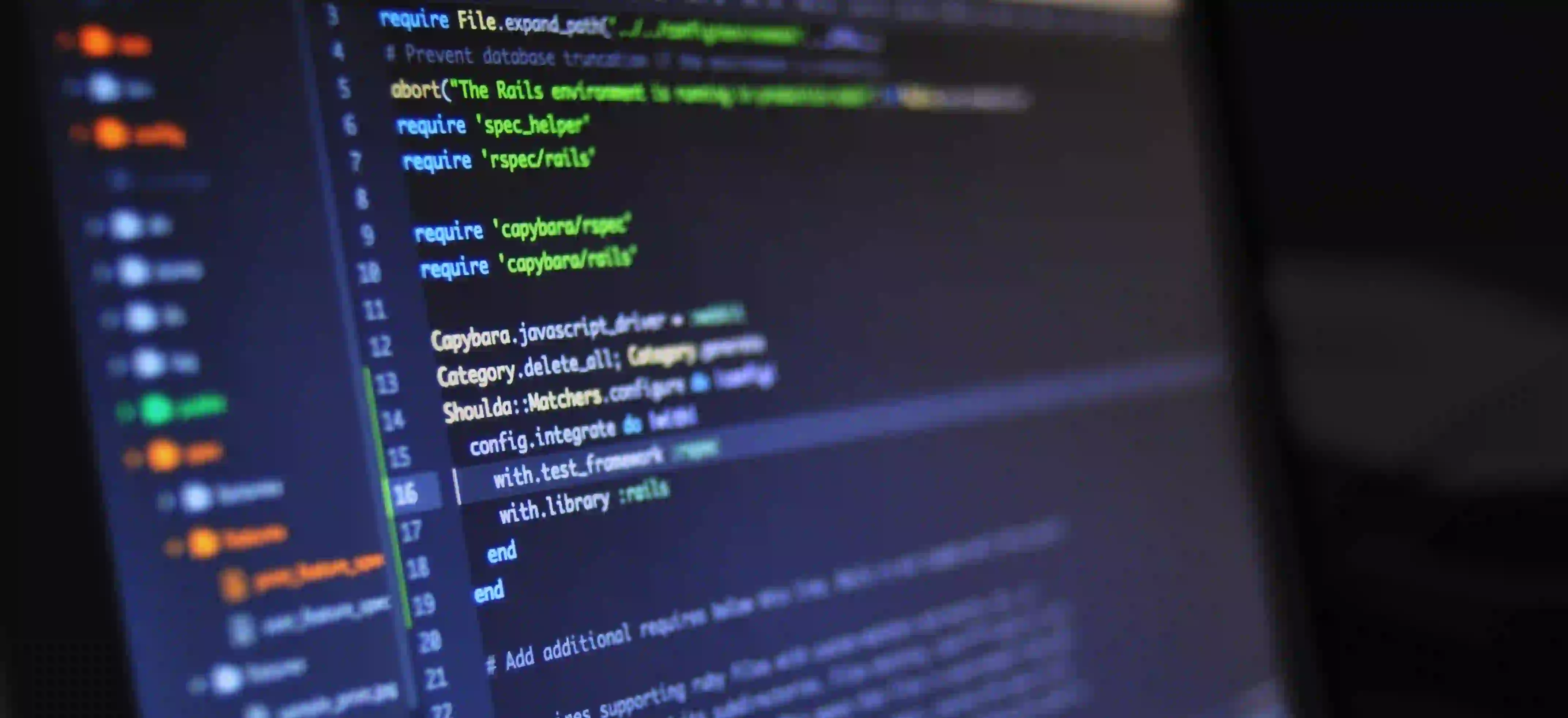
Performance Showdown: sort
vs parallelSort
in Arrays
When handling large datasets in Java, the performance of sorting algorithms can significantly impact the efficiency of an application. Java provides two primary mechanisms for sorting arrays: the traditional Arrays.sort()
and the more modern Arrays.parallelSort()
. This blog post delves into the intricacies of these two methods, comparing their performance, use cases, and advantages.
Understanding the Basics
Before we dive deep into performance comparisons, let's establish a solid understanding of the two sorting methods.
Arrays.sort()
The Arrays.sort()
method is a part of the Java standard library that provides a quick and efficient way to sort arrays. Under the hood, it uses a dual-pivot Quicksort algorithm, which is known for its efficiency in average cases.
Here’s a simple code snippet demonstrating its usage:
import java.util.Arrays;
public class SortExample {
public static void main(String[] args) {
int[] numbers = {5, 3, 8, 1, 2};
Arrays.sort(numbers);
System.out.println("Sorted Array: " + Arrays.toString(numbers));
}
}
Why Use Arrays.sort()
?
- Simplicity: It’s straightforward to use, requiring only a single line.
- Efficiency: For smaller datasets,
Arrays.sort()
is often faster due to lower overhead.
Arrays.parallelSort()
Introduced in Java 8, Arrays.parallelSort()
utilizes the Fork/Join framework to sort large arrays in parallel. This method takes advantage of multi-core processors, splitting the array into smaller chunks, sorting them concurrently, and then merging the results.
Here’s how you can implement parallelSort()
:
import java.util.Arrays;
public class ParallelSortExample {
public static void main(String[] args) {
int[] numbers = {5, 3, 8, 1, 2};
Arrays.parallelSort(numbers);
System.out.println("Sorted Array: " + Arrays.toString(numbers));
}
}
Why Use Arrays.parallelSort()
?
- Performance on Large Datasets: It excels with large arrays, where parallelism can significantly reduce sort time.
- Leverage Multi-Core Processors: By utilizing multiple CPU cores, it harnesses greater computational power.
Performance Comparison
To make an informed choice between sort
and parallelSort
, it's crucial to understand their performance characteristics.
Time Complexity
Both algorithms have the same time complexity in terms of Big O notation:
- Best Case: O(n log n)
- Average Case: O(n log n)
- Worst Case: O(n log n)
However, the constants involved can differ significantly due to overheads associated with parallelSort()
.
Empirical Performance Testing
The best way to evaluate performance is by running tests under various conditions. Below is a benchmark example comparing the two methods with varying array sizes.
import java.util.Arrays;
public class PerformanceBenchmark {
public static void main(String[] args) {
int size = 1000000; // Array size
int[] array1 = generateRandomArray(size);
int[] array2 = Arrays.copyOf(array1, array1.length); // Copy for fair comparison
long startTime = System.nanoTime();
Arrays.sort(array1);
long durationSort = System.nanoTime() - startTime;
startTime = System.nanoTime();
Arrays.parallelSort(array2);
long durationParallelSort = System.nanoTime() - startTime;
System.out.println("Time taken by Arrays.sort(): " + durationSort + " ns");
System.out.println("Time taken by Arrays.parallelSort(): " + durationParallelSort + " ns");
}
private static int[] generateRandomArray(int size) {
int[] array = new int[size];
for (int i = 0; i < size; i++) {
array[i] = (int) (Math.random() * size);
}
return array;
}
}
Expected Results
- Small Sizes:
Arrays.sort()
typically performs better due to lower overhead. - Medium to Large Sizes:
Arrays.parallelSort()
starts to dominate as the size grows, especially on multi-core machines.
Memory Considerations
While parallelSort()
can yield performance gains, it also incurs greater memory overhead due to its use of additional threads and the need to maintain temporary arrays. This factor is crucial in environments with limited memory resources.
Garbage Collection Impact
When using parallel sort, the system forks additional threads. Java's garbage collector may need to work harder, leading to longer pause times in certain scenarios, especially if the application is heavily dependent on real-time performance.
When to Use Each Method
Choose Arrays.sort()
when:
- Working with small to medium arrays.
- Memory usage is a concern.
- Simplicity and quick implementation are critical.
Choose Arrays.parallelSort()
when:
- Handling large datasets where performance is paramount.
- The application runs in a multi-core environment.
- You're prepared to manage the increased memory consumption.
To Wrap Things Up
Both Arrays.sort()
and Arrays.parallelSort()
serve essential roles in Java's sorting capabilities. Understanding their strengths and limitations allows developers to leverage the best method for their specific use case.
Whether your application is crunching large data tables or sorting small collections, choosing the right sorting algorithm can make a tangible difference in performance. Benchmark your specific use case to find the most efficient solution for your needs.
For more insights on performance optimization and Java best practices, visit Oracle's Java documentation.
Additional Resources
- Learn more about the Java Collection Framework.
- For more about Fork/Join framework, check out Java's Fork/Join Framework.
- Explore advanced sorting algorithms like Merge Sort and Quick Sort.
With a clear understanding of these sorting techniques, you’ll be well-equipped to make efficient choices in your Java applications!