Mastering Java Dynamic Proxies: Common Pitfalls to Avoid
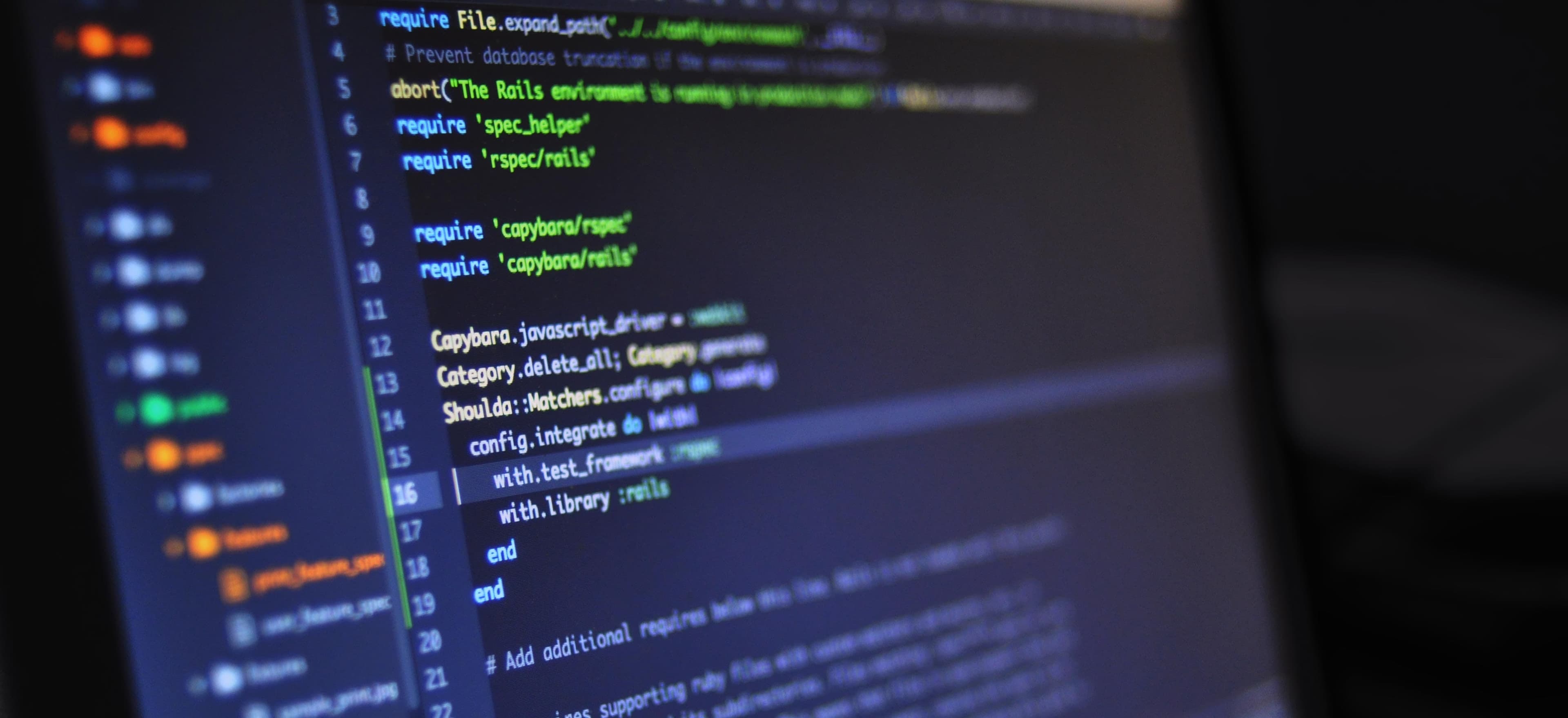
- Published on
Mastering Java Dynamic Proxies: Common Pitfalls to Avoid
Java is a powerful, versatile language, and part of its allure is its capability for dynamic proxies. Dynamic proxies provide a way to create proxy instances at runtime, allowing for programmatic behavior modification and enhancing the flexibility of your code. However, while Java dynamic proxies can be incredibly useful, they aren't without their common pitfalls. In this blog post, we will explore what dynamic proxies are, how to use them effectively, and highlight some common mistakes to watch out for.
What are Java Dynamic Proxies?
Dynamic proxies in Java are part of the Reflection API and allow you to create proxy instances for interfaces. A dynamic proxy can forward method calls to a central handler, which can implement various behaviors, such as logging, security checks, or transaction management.
Simple Example of a Dynamic Proxy
For a practical understanding, let’s start with a simple example of a dynamic proxy. Consider an interface Greeting
:
public interface Greeting {
void sayHello(String name);
}
Next, we can create a handler that uses the InvocationHandler
interface to control the way the method gets executed. Here's how you can set it up:
import java.lang.reflect.InvocationHandler;
import java.lang.reflect.Method;
import java.lang.reflect.Proxy;
class GreetingInvocationHandler implements InvocationHandler {
private final Object target;
public GreetingInvocationHandler(Object target) {
this.target = target;
}
@Override
public Object invoke(Object proxy, Method method, Object[] args) throws Throwable {
System.out.println("Before invoking " + method.getName());
Object result = method.invoke(target, args);
System.out.println("After invoking " + method.getName());
return result;
}
}
Creating the Proxy Instance
Now, let’s create a simple implementation of the Greeting
interface:
public class SimpleGreeting implements Greeting {
@Override
public void sayHello(String name) {
System.out.println("Hello, " + name + "!");
}
}
Now to create the proxy:
public class ProxyExample {
public static void main(String[] args) {
SimpleGreeting simpleGreeting = new SimpleGreeting();
Greeting proxyInstance = (Greeting) Proxy.newProxyInstance(
Greeting.class.getClassLoader(),
new Class[]{Greeting.class},
new GreetingInvocationHandler(simpleGreeting)
);
proxyInstance.sayHello("World");
}
}
Output
When you run the ProxyExample
class, you will observe:
Before invoking sayHello
Hello, World!
After invoking sayHello
This output demonstrates how the dynamic proxy captures the invocation of the method and allows you to encapsulate behavior before and after the call.
Common Pitfalls When Using Dynamic Proxies
While dynamic proxies are a powerful tool, they come with some potential pitfalls. Let's explore these in more detail.
1. Only Interfaces Can Be Proxied
Java dynamic proxies can only delegate calls for interfaces, not concrete classes. This often leads developers to inadvertently attempt to proxy a class instead of its interface.
Solution: Always ensure that you create an interface for the behavior you want to proxy. This encapsulates your behavior better and avoids the direct coupling to a concrete implementation.
2. Performance Overhead
Dynamic proxies come with a performance cost due to reflection. Each method call results in a level of indirection, which can lead to slower execution times in performance-critical applications.
Solution: Use dynamic proxies judiciously. If performance is a concern, method interception frameworks such as AspectJ might be more suitable.
3. Non-public Interfaces
Dynamic proxies can only be created for interfaces that are visible to the class loader. This means that if you're trying to proxy a non-public interface, it may not work as expected.
Solution: Ensure that all interfaces that you want to use with dynamic proxies are public and designed to work interactively with each other.
4. Misunderstanding Method Invocation
A common mistake is misunderstanding invoke
behavior where the target method may throw exceptions. If you don’t manage this properly in your invocation handler, you may inadvertently swallow exceptions or fail to log critical information about method failures.
Solution: Make sure to handle exceptions properly in the invoke
method. For example:
@Override
public Object invoke(Object proxy, Method method, Object[] args) throws Throwable {
try {
// logging and processing logic
return method.invoke(target, args);
} catch (Exception e) {
// Handle exception
System.err.println("An error occurred: " + e.getMessage());
throw e; // rethrow the exception
}
}
5. Lack of Type Safety
Since dynamic proxies rely on type casting while creating instances, this can lead to ClassCastException
at runtime if not used properly.
Solution: Always check the type and make sure to manage type safety carefully. Use generics where applicable to limit risks.
6. More Complexity
Using dynamic proxies can make code less readable. The added layer of indirection can be hard for other developers to follow, which might lead to maintenance difficulties.
Solution: Document the use of proxies well. Consider whether employing an interface or framework like Spring AOP would be more appropriate for your specific case, as they often enhance readability.
Closing the Chapter
Dynamic proxies in Java are a powerful feature but come with their own set of challenges. While they can effectively provide centralized behavior, developers must heed the common pitfalls we've discussed. Always prioritize performance, type-safety, and maintainability when designing your solutions.
For a deeper dive into reflective programming in Java, consider checking Java Reflection Basics.
As a best practice, keep your interface definitions clear, document your proxy usage well, and choose the right tools for the job. Proxies can greatly enhance your code's flexibility and modularity when wielded with care and precision.
Feel free to try out the code snippets we've shared, experiment with your enhancements, and explore the rich world of Java dynamic proxies! Happy coding!