How to Effectively Throttle Download Speed on Your Server
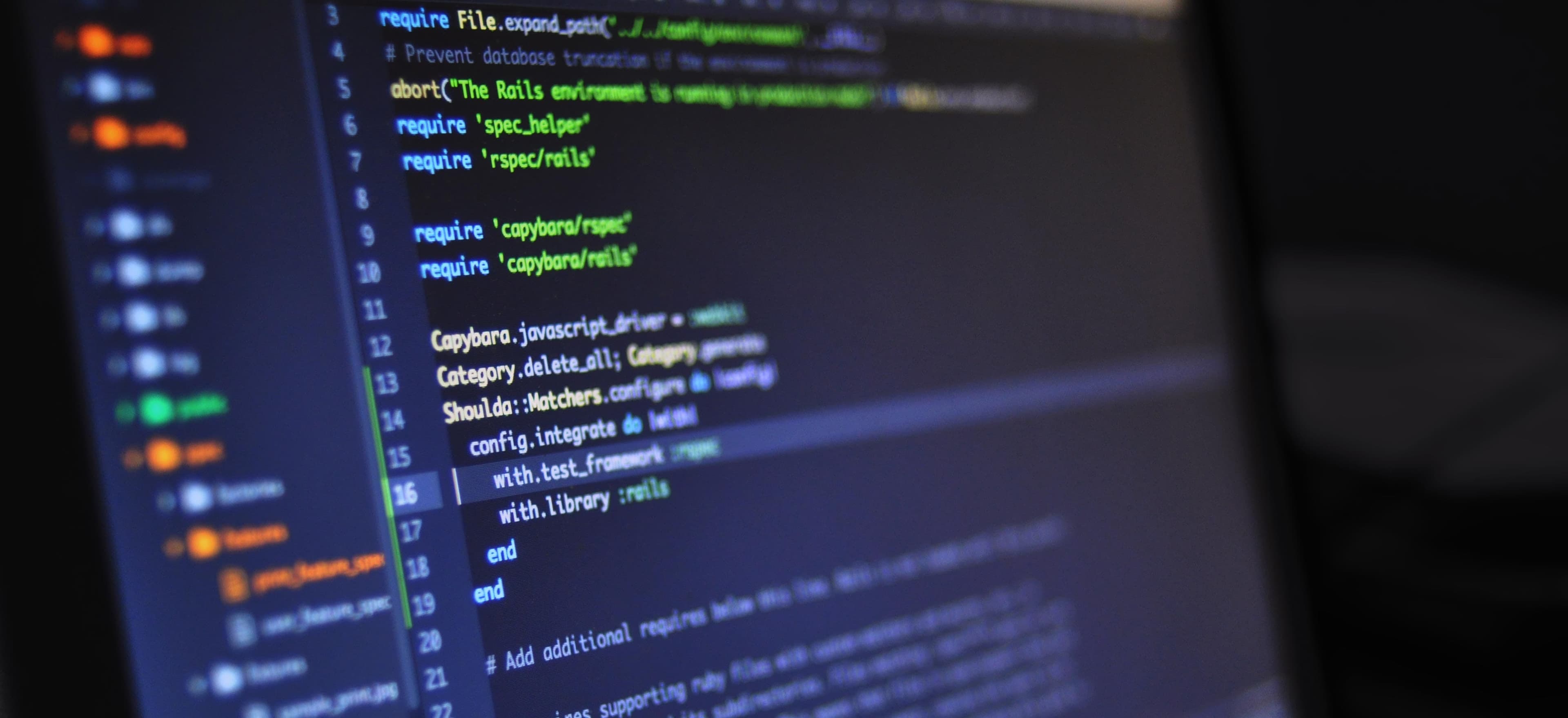
- Published on
How to Effectively Throttle Download Speed on Your Server
In an era where data consumption is soaring, managing and controlling download speeds on your server is crucial. Throttling can help balance load, prevent abuse, and ensure fair usage. This guide will delve into the various techniques of throttling download speeds, specific implementations using Java, and best practices to enhance your server's performance.
Understanding Throttling
Throttling refers to the deliberate slowing down of the data transfer rate over a network. It can be beneficial in scenarios where:
- Managing Bandwidth: Preventing certain users from consuming too much bandwidth.
- Load Balancing: Distributing available resources more evenly among users.
- User Experience: Ensuring that all users have equitable access to server resources without congestion.
Now that we have a basic understanding of throttling, let’s explore how to implement this in a Java-based application effectively.
Implementing Download Speed Throttling in Java
To throttle download speeds in Java, you can achieve it using various methods, including streams, threading, or libraries specifically designed for throttle control. Below, we’ll explore an example implementation using Java’s InputStream
and OutputStream
.
Example Code Snippet
Here’s a simple Java method to illustrate throttling download speeds:
import java.io.*;
public class ThrottledDownload {
private static final int BUFFER_SIZE = 4096; // Buffer size for copying
private static final long THROTTLE_DELAY_MS = 100; // Delay in milliseconds
public void downloadFile(InputStream inputStream, OutputStream outputStream) throws IOException {
byte[] buffer = new byte[BUFFER_SIZE];
int bytesRead;
while ((bytesRead = inputStream.read(buffer)) != -1) {
outputStream.write(buffer, 0, bytesRead);
// Throttle download by sleeping
try {
Thread.sleep(THROTTLE_DELAY_MS);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new IOException("Download interrupted", e);
}
}
}
}
Explanation of the Code
- BUFFER_SIZE: This is set to 4096 bytes, which represents the amount of data read into memory at a time. A larger buffer size may improve performance but can also consume more memory. Adjust based on your server’s capabilities.
- THROTTLE_DELAY_MS: The delay (in milliseconds) to be introduced after each buffer is read. Adjust this to control the download speed effectively.
- InputStream & OutputStream: These streams facilitate the reading and writing of data from and to the server.
Why Throttle?
Throttling is not just about restricting access; rather, it’s about smart utilization of resources. For instance, if five users are trying to download a sizable file simultaneously, it could lead to network congestion. Throttling helps manage such scenarios to maintain optimal speed for all users.
Alternatives and Advanced Techniques
While the aforementioned method is effective, there are other sophisticated techniques to control download speed.
Using Rate Limiting Libraries
Libraries such as Guava provide built-in methods to implement rate limiting. It allows for precise control over how many bytes can be processed in a given timeframe.
Here’s an example using Guava's RateLimiter
:
import com.google.common.util.concurrent.RateLimiter;
import java.io.*;
public class RateLimitedDownload {
private static final double RATE = 1.0; // Allow 1 MB per second
private RateLimiter rateLimiter;
public RateLimitedDownload() {
this.rateLimiter = RateLimiter.create(RATE); // Create a rate limiter
}
public void downloadFile(InputStream inputStream, OutputStream outputStream) throws IOException {
byte[] buffer = new byte[1024 * 1024]; // 1 MB buffer
int bytesRead;
while ((bytesRead = inputStream.read(buffer)) != -1) {
rateLimiter.acquire(); // Wait for the cup of speed
outputStream.write(buffer, 0, bytesRead);
}
}
}
Key Advantages of Using RateLimiter
- Precision: Provides a straightforward way to achieve fine-grained control.
- Thread Handling: Manages download rates even when multiple threads are involved.
- Flexibility: Can be adjusted easily to accommodate various bandwidth requirements.
Best Practices for Throttling
Implementing throttling should be approached thoughtfully, considering user experience and system performance. Here are some best practices:
- Monitor Performance: Continuously evaluate the impact of throttling on server performance.
- User Feedback: Clearly communicate any throttling measures to users, enhancing their experience and support.
- Adaptive Throttling: Implement algorithms that can dynamically adjust throttling based on server load and network conditions.
- Logging: Keep track of usage patterns, which can inform future adjustments to throttling conditions.
In Conclusion, Here is What Matters
Throttling is a vital component of server management, and using Java to implement it provides flexible, powerful solutions. Whether you choose to throttle downloads manually through input streams or leverage libraries like Guava for rate limiting, the result is a smoother, more equitable service for all users.
For more detailed reading on server management and optimization techniques, check out these resources:
- Apache Tomcat Performance Tuning
- Effective Java Programming
By effectively throttling download speeds, you can ensure that your server operates efficiently while providing a fair user experience. Take the time to assess your specific needs and employ the techniques discussed to create an optimized environment.
Checkout our other articles