Unlocking the Secrets of SAX Parsing for XML Data
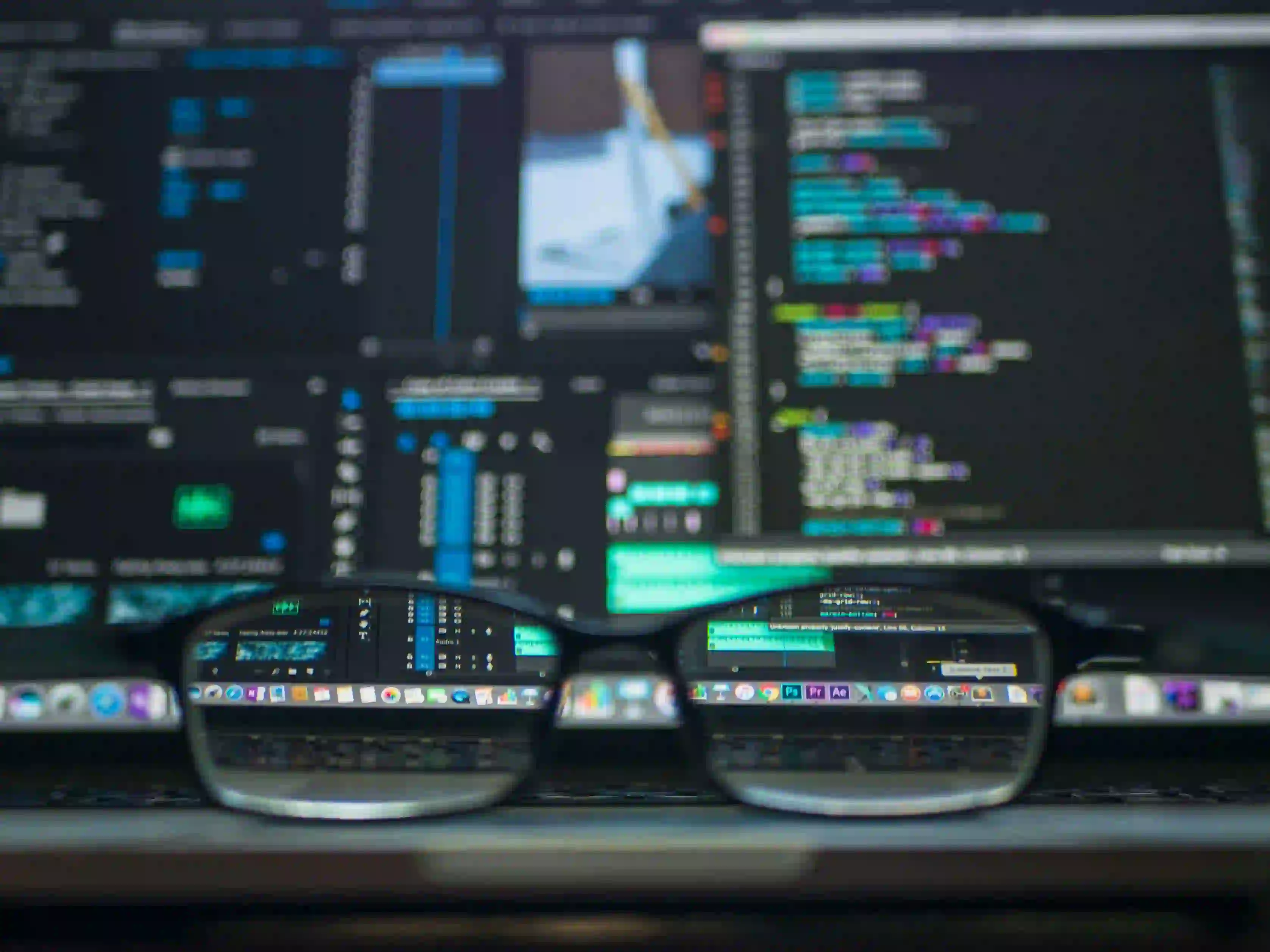
Unlocking the Secrets of SAX Parsing for XML Data
When it comes to processing XML in Java, developers have a variety of options at their disposal. Two of the most common parsing methods are DOM (Document Object Model) and SAX (Simple API for XML). While DOM is widely used for its ease of navigation, SAX offers advantages that are particularly valuable in scenarios of large datasets where memory efficiency is paramount. In this blog post, we will delve deep into SAX parsing, highlighting its advantages, providing code snippets to illustrate its use, and discussing best practices.
Why Choose SAX Parsing?
Before we dive into the mechanics of SAX parsing, let's quickly discuss why you may want to choose this method over others:
-
Memory Efficiency: SAX parses the XML stream without loading the entire document into memory. This is especially beneficial for large XML files.
-
Speed: SAX can read and parse XML data quickly since it processes data in streaming fashion β it stops reading as soon as it gets the information it needs.
-
Event-driven: SAX parser generates events as it encounters elements in the XML file, triggering specific actions via user-defined handlers.
-
Low Overhead: Because SAX consumes less memory, it is well-suited for applications where system resources might be constrained.
The Basics of SAX Parsing
SAX works by triggering events when it encounters specific parts of the XML document, such as the start and end of elements and character data.
The SAX parser interfaces you typically use in Java include:
org.xml.sax.helpers.DefaultHandler
: A helper class to simplify event handling.org.xml.sax.ErrorHandler
: An interface to handle errors during parsing.
To begin with SAX parsing, you will need to add the necessary libraries to your project. If you are using Maven, the following dependency is already included in the Java Standard Library:
<dependency>
<groupId>xml-apis</groupId>
<artifactId>xml-apis</artifactId>
<version>1.4.01</version>
</dependency>
Creating Your SAX Parser
Here is a step-by-step guide to create a SAX parser.
Step 1: Create a Handler
The first step in using SAX involves creating a handler class that extends DefaultHandler
. This class will contain methods that respond to various events.
import org.xml.sax.Attributes;
import org.xml.sax.SAXException;
import org.xml.sax.helpers.DefaultHandler;
public class MySAXHandler extends DefaultHandler {
@Override
public void startElement(String uri, String localName, String qName, Attributes attributes) throws SAXException {
// Triggered at the start of an element.
System.out.println("Start Element: " + qName);
// Attributes can be accessed here
for (int i = 0; i < attributes.getLength(); i++) {
System.out.println("Attribute: " + attributes.getQName(i) + " = " + attributes.getValue(i));
}
}
@Override
public void endElement(String uri, String localName, String qName) throws SAXException {
// Triggered at the end of an element.
System.out.println("End Element: " + qName);
}
@Override
public void characters(char[] ch, int start, int length) throws SAXException {
// Triggered when character data is encountered.
System.out.println("Characters: " + new String(ch, start, length).trim());
}
}
Step 2: Parse the XML File
Next, we will set up the SAX parser and use it to read an XML file using the handler defined above.
import org.xml.sax.SAXException;
import javax.xml.parsers.SAXParser;
import javax.xml.parsers.SAXParserFactory;
import java.io.File;
import java.io.IOException;
public class SAXParserExample {
public static void main(String[] args) {
try {
SAXParserFactory factory = SAXParserFactory.newInstance();
SAXParser saxParser = factory.newSAXParser();
MySAXHandler handler = new MySAXHandler();
saxParser.parse(new File("example.xml"), handler); // Adjust the filepath as necessary
} catch (SAXException | IOException | ParserConfigurationException e) {
e.printStackTrace();
}
}
}
Explanation of Code Choice
-
SAXParserFactory: This factory creates the SAX parser instance that will be used to parse the XML data.
-
MySAXHandler: When we create an instance of this handler, we enable the SAX parser to utilize our event-driven methods to process XML elements.
-
Parse Method: The
parse()
method takes in a File and a handler, allowing us to link our event-driven logic directly to the XML structure.
Sample XML
To test the above code, you can use the following simplistic XML format, saved as example.xml
:
<?xml version="1.0"?>
<note>
<to>Tove</to>
<from>Jani</from>
<heading>Reminder</heading>
<body>Don't forget me this weekend!</body>
</note>
Running the Parser
Compile and run your Java application, and you should see output similar to:
Start Element: note
Start Element: to
Characters: Tove
End Element: to
Start Element: from
Characters: Jani
End Element: from
Start Element: heading
Characters: Reminder
End Element: heading
Start Element: body
Characters: Don't forget me this weekend!
End Element: body
End Element: note
Best Practices for SAX Parsing
-
Use Efficient Memory Management: Be aware that excessive string concatenation within character handlers can lead to performance bottlenecks. Consider using
StringBuilder
for large datasets. -
Error Handling: Implement a robust error handling strategy by extending
ErrorHandler
. This way you can gracefully handle issues without crashing your application.βsnippet.javapublic class MyErrorHandler implements ErrorHandler { @Override public void warning(SAXParseException exception) throws SAXException { System.err.println("Warning: " + exception.getMessage()); } @Override public void error(SAXParseException exception) throws SAXException { System.err.println("Error: " + exception.getMessage()); } @Override public void fatalError(SAXParseException exception) throws SAXException { System.err.println("Fatal Error: " + exception.getMessage()); throw exception; } }
-
Use Named Constants: If your application needs to parse specific types of XML, consider using named constants for element and attribute names for maintainability.
-
Choose the Right XPath: If you find yourself doing a lot of element/query work, consider using
XPath
along with your SAX parser for more complex XML structures.
Final Thoughts
SAX parsing is an integral part of XML processing in Java, especially when working with large files where traditional DOM parsing can be inefficient. With its event-driven model, SAX allows you to handle input in a zero-sum manner, ensuring that your applications remain responsive and efficiently handle XML data.
Whether youβre a seasoned Java developer or just starting, mastering SAX parsing will undoubtedly enhance your abilities to manage and process XML effectively. For further reading, consider looking into Java SE Documentation or W3C XML Specifications to deepen your understanding of XML parsing tools.
Happy coding!