Common Pitfalls When Using JUnit with EasyMock
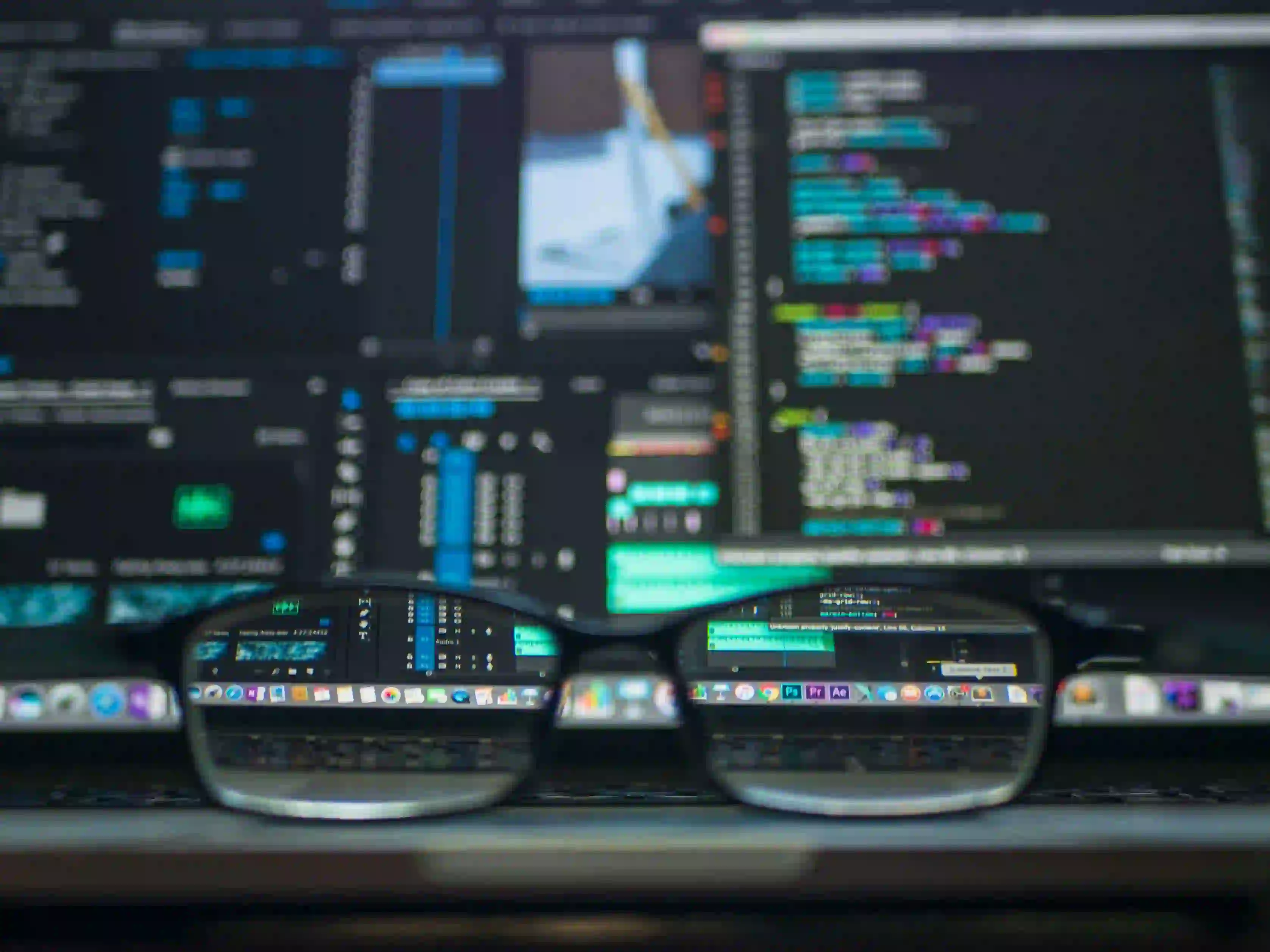
Common Pitfalls When Using JUnit with EasyMock
In the world of Java testing, JUnit and EasyMock are two powerful tools that developers often use together to create reliable unit tests. JUnit helps us structure and run our tests, while EasyMock allows us to create mock objects easily and flexibly. While both tools are fantastic for unit testing, integrating them smoothly can be challenging. This blog post will guide you through some common pitfalls developers encounter when using JUnit with EasyMock, and I'll provide advice on how to avoid them.
Understanding JUnit and EasyMock
Before diving into common pitfalls, let's briefly review both frameworks.
JUnit
JUnit is a simple, open-source testing framework for Java. It allows you to efficiently write and run repeatable tests. It follows the 'Arrange-Act-Assert' pattern, which helps ensure tests are structured and easy to read.
EasyMock
EasyMock is a framework that simplifies the creation of mock objects, which are essential for unit testing. Mocks allow you to isolate the class you are testing by simulating the behavior of its dependencies. This can help avoid slow tests, external dependencies, and side effects.
Both tools play vital roles in the testing process, but using them together requires attention to detail.
Common Pitfalls
1. Not Initializing Mocks Properly
A prevalent mistake is to forget to initialize mocks before using them in tests. In EasyMock, you have to call the EasyMock.createMock()
method, but it's crucial to follow this by calling EasyMock.replay(mock)
to indicate that the mock is ready for testing.
Example:
import static org.easymock.EasyMock.*;
import org.junit.Before;
import org.junit.Test;
public class MyServiceTest {
private MyService myService;
private MyDependency mockDependency;
@Before
public void setUp() {
mockDependency = createMock(MyDependency.class);
myService = new MyService(mockDependency);
}
@Test
public void testMyServiceMethod() {
expect(mockDependency.someMethod()).andReturn("mocked value");
replay(mockDependency);
String result = myService.myMethod();
assertEquals("Expected result not found", "mocked value", result);
verify(mockDependency);
}
}
In the code above, we correctly initialize the mock and prepare it for testing. If you forget the replay()
call, the test will fail.
2. Forgetting to Verify Interactions
Verification of interactions is critical to ensure that the expected methods on mocks were called during the execution of your code. Developers often forget to call EasyMock.verify(mock)
at the end of their tests.
Example:
@Test
public void testAnotherServiceMethod() {
mockDependency.performAction();
replay(mockDependency);
myService.anotherMethod();
// Verification is crucial here
verify(mockDependency);
}
Failing to call verify()
means that even if the test passes, it won't confirm that the mock behaved as expected. Consequently, you might miss bugs in your logic.
3. Stubbing Multiple Returns Incorrectly
Another common pitfall is trying to stub methods that return different values in successive calls without proper understanding. EasyMock supports this, but it requires correctly chaining the expect
statements.
Example:
@Test
public void testSequentialReturns() {
expect(mockDependency.fetchValue()).andReturn("first").andReturn("second");
replay(mockDependency);
assertEquals("first", mockDependency.fetchValue());
assertEquals("second", mockDependency.fetchValue());
verify(mockDependency);
}
In this example, we correctly set up two returns for our mock method. Failing to do this would result in an expectation mismatch
error.
4. Using Real Objects Instead of Mocks
One serious mistake is using real objects instead of mocks. This defeats the purpose of unit tests, as you lose the isolation you need to test a single unit of code without worrying about its dependencies.
A developer might accidentally forget to mock a dependency or use a shared state that could affect other tests. Always ensure to isolate your tests to maintain their reliability.
5. Mixing Test Frameworks
JUnit is often used with other frameworks and libraries (like TestNG, Mockito, etc.). Mixing them, especially when dealing with mocks, can cause confusion and unpredictable results. Stick with a consistent choice throughout the testing process.
For instance, if you're using EasyMock, make sure that you aren't inadvertently introducing Mockito or a different mocking framework into the same test scope:
Example:
import static org.mockito.Mockito.*;
@Test
public void testConflictingFrameworks() {
MyDependency mockDependency = mock(MyDependency.class); // This is Mockito
// EasyMock used below would conflict
MyDependency easyMockDependency = createMock(MyDependency.class);
}
This code snippet shows conflicting usages. Maintain clarity and consistency throughout your test suite.
6. Cleansing the State Between Tests
JUnit creates a new instance of the test class for each test case when using the @Before
annotation. Some developers mistakenly believe that setting state in @Before
will interfere with subsequent tests, leading to shared state issues.
The solution here is straightforward: always ensure your tests are stateless or that you're initializing all necessary state during the @Before
setup.
7. Using Annotations Incorrectly
In JUnit 4, several annotations can make setting up tests easier. However, misusing or forgetting these annotations can lead to confusion. For example, mistakenly dancing between @BeforeClass
and @Before
can create issues, as @BeforeClass
is called only once for the entire class.
Example:
@Before // Correct use
public void setUp() {
mockDependency = createMock(MyDependency.class);
replay(mockDependency);
}
The Closing Argument
Using JUnit with EasyMock can enhance your Java testing, making it easier to create reliable, maintainable, and swift unit tests. The pitfalls discussed above are common, but they can be avoided with careful practice.
Further Reading
- To dive deeper into the specifics of JUnit, check out JUnit 5 User Guide.
- For more on mocking practices and guidelines, consider reading EasyMock Documentation.
By being aware of these pitfalls and understanding the best practices, you will create more robust and effective unit tests. Embrace both JUnit and EasyMock's strengths, and your testing process will improve significantly. Happy testing!