Securing Java Web Apps Against Session Hijacking Risks
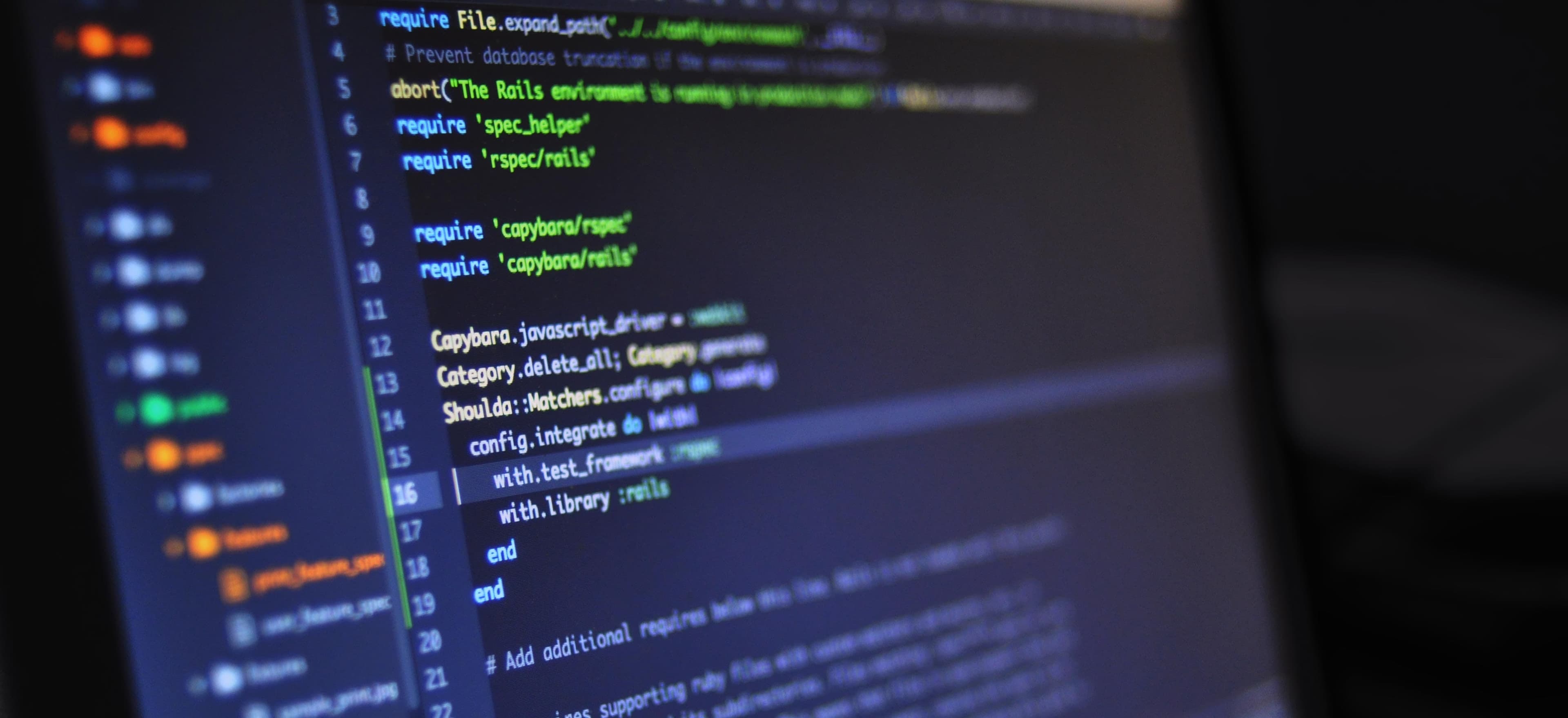
- Published on
Securing Java Web Apps Against Session Hijacking Risks
In an era where web applications dominate the digital landscape, security has never been more critical. As developers, we must safeguard our applications against various threats, one of the most concerning being session hijacking. In this post, we will delve into session hijacking risks, exploring practical ways to secure Java web applications against these vulnerabilities.
Understanding Session Hijacking
Session hijacking occurs when an attacker takes control of a user session after they have authenticated themselves in a web application. During this hijacking, the attacker can impersonate the user, gaining unauthorized access to sensitive data and functionality.
How Do Attacks Happen?
Attackers employ various techniques to hijack sessions, including:
- Session fixation: The attacker sets a user's session identifier (ID) on a victim's machine before the user logs in.
- Cross-site scripting (XSS): Malicious scripts can be injected into a web page, allowing attackers to steal session cookies.
- Network sniffing: Insecure connections can lead to intercepted session data.
Why Is It Important?
According to the article Is Your Login Safe? Unveiling Session Security Flaws, session hijacking can lead to severe consequences, including data breaches, loss of credibility, and potential legal ramifications for organizations. Thus, ensuring strong session security is paramount.
Best Practices for Securing Java Web Applications
1. Use HTTPS
The first line of defense against session hijacking is always to enforce HTTPS. This ensures data transmitted between the client and server remains encrypted.
// Enforce HTTPS in your web XML file or configuration
<security-constraint>
<web-resource-collection>
<web-resource-name>Protected Area</web-resource-name>
<url-pattern>/*</url-pattern>
</web-resource-collection>
<auth-constraint />
<user-data-constraint>
<transport-guarantee>CONFIDENTIAL</transport-guarantee>
</user-data-constraint>
</security-constraint>
Why this matters: By encrypting data over the network, you reduce the risk of interception by unauthorized parties, safeguarding session identifiers and other sensitive information.
2. Implement Secure Cookie Attributes
Setting the right cookie attributes plays a crucial role in making your application more secure.
// Creating a secure session cookie
HttpSession session = request.getSession();
session.setAttribute("user", user);
Cookie cookie = new Cookie("JSESSIONID", session.getId());
cookie.setHttpOnly(true); // Prevent XSS
cookie.setSecure(true); // Only sent over HTTPS
cookie.setMaxAge(3600); // Set cookie expiry
response.addCookie(cookie);
Why this matters: The
HttpOnly
attribute protects against XSS attacks by preventing JavaScript from accessing the cookie. TheSecure
attribute ensures the cookie is only sent over HTTPS, reducing the risk of theft.
3. Session Regeneration
Regenerating session IDs during sensitive actions (like logging in or changing passwords) can prevent session fixation attacks.
if (user.isAuthenticated()) {
request.getSession().invalidate(); // Invalidate the old session
HttpSession newSession = request.getSession(true); // Create a new session
newSession.setAttribute("user", user);
}
Why this matters: This technique ensures that even if an attacker had control of a previous session ID, it will become useless when the session is regenerated.
4. Use Short Session Timeouts
Implementing a short session timeout can prevent potential hijacking. Here’s an example of how to configure your sessions in Java:
<session-config>
<session-timeout>15</session-timeout> <!-- Duration in minutes -->
</session-config>
Why this matters: Shorter session lifespans reduce the window of opportunity for an attacker to hijack a session. Users can be prompted to re-authenticate if their session has expired.
5. Implement CSRF Protection
Cross-Site Request Forgery (CSRF) attacks can lead to unauthorized actions being taken with a user’s session. Utilizing CSRF tokens can mitigate this risk.
// Generate CSRF Token on form load
String csrfToken = UUID.randomUUID().toString();
session.setAttribute("csrfToken", csrfToken);
request.setAttribute("csrfToken", csrfToken);
// In your form
<form action="/submit" method="post">
<input type="hidden" name="csrfToken" value="${csrfToken}" />
...
</form>
// Validate CSRF Token on form submission
String formToken = request.getParameter("csrfToken");
String sessionToken = (String) session.getAttribute("csrfToken");
if (!formToken.equals(sessionToken)) {
throw new SecurityException("Invalid CSRF Token");
}
Why this matters: CSRF tokens ensure that requests are coming from authenticated and legitimate users. If the token is missing or invalid, the request can be rejected.
6. Monitor and Log User Activity
Implementing monitoring and logging mechanisms can be essential in detecting and responding to suspicious activity.
// Log user activity
Logger logger = Logger.getLogger(MyServlet.class.getName());
logger.info("User " + user.getUsername() + " accessed " + request.getRequestURI());
Why this matters: Monitoring user actions can help identify unauthorized access or unusual behavior, allowing you to take timely action.
Bringing It All Together
Securing Java web applications against session hijacking requires an integration of several best practices. From using HTTPS and setting secure cookie attributes to implementing CSRF protection and monitoring, each step is vital in establishing a robust security posture.
For more in-depth discussions about web security, particularly regarding login security, consider reading the article Is Your Login Safe? Unveiling Session Security Flaws.
By implementing these practices, developers can significantly reduce the risk of session hijacking and provide a safer environment for their users. Always remember, security is an ongoing process that demands attention, vigilance, and continuous improvement.