Resolving Dropdown Menu Bugs in Java Web Applications
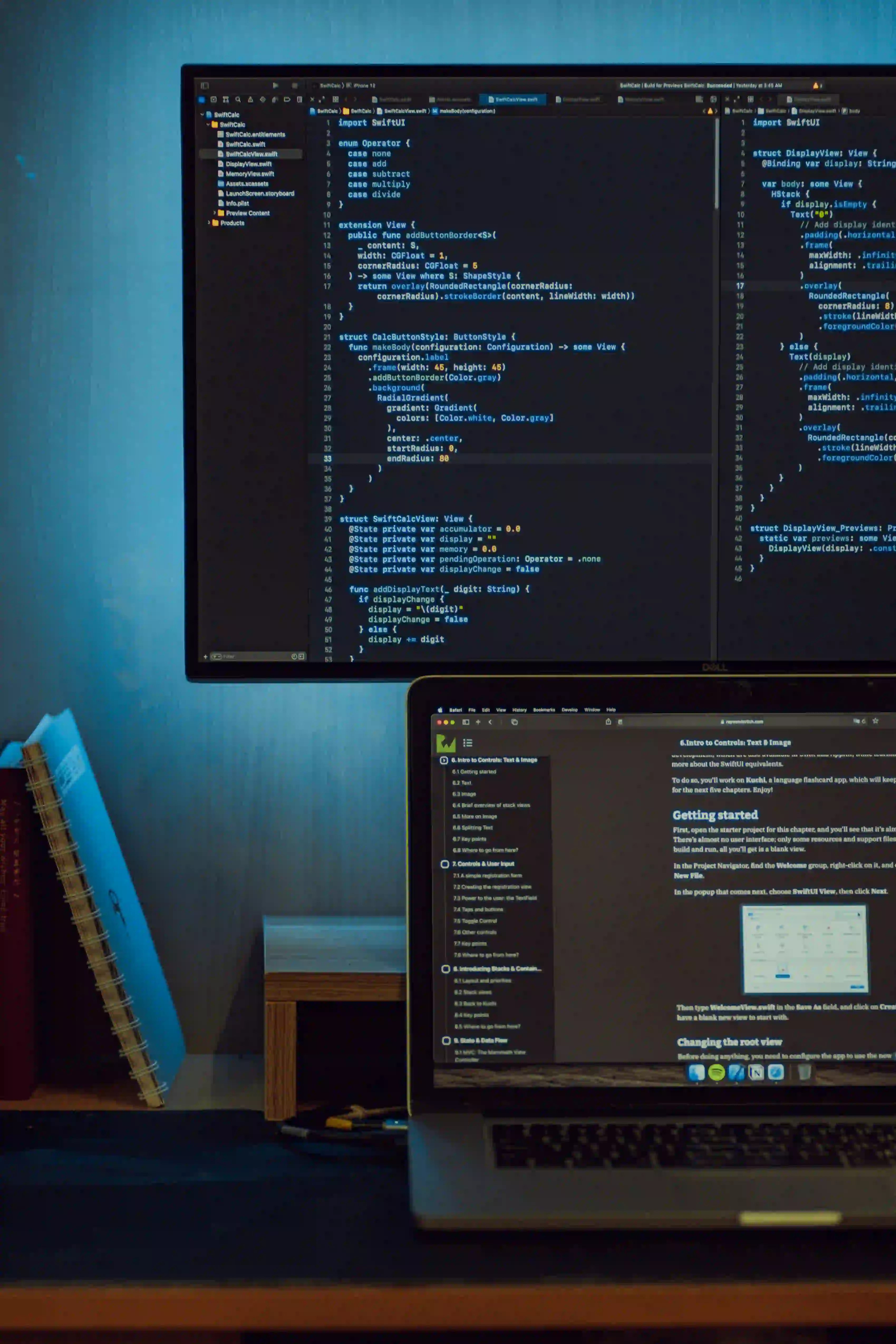
Resolving Dropdown Menu Bugs in Java Web Applications
Dropdown menus are a fundamental component of web applications, providing users with a simple means to navigate through various options. However, ensuring their smooth functionality across all browsers can be quite daunting, especially when dealing with inconsistencies inherent in various browser engines. In this post, we will explore common issues with dropdown menus in Java web applications and provide solutions to enhance user experience.
Understanding Dropdown Menus
Dropdown menus are crucial for enhancing user navigation and interaction. They can be implemented using HTML, CSS, and JavaScript, forming an integral part of the front end of your Java web application. This interaction layer can sometimes lead to problems, especially when the state of the menu does not render as expected across different browsers.
Basic Dropdown Menu Structure
Here's a simple example of a dropdown menu implemented in HTML:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Simple Dropdown Menu</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div class="dropdown">
<button class="dropbtn">Dropdown</button>
<div class="dropdown-content">
<a href="#link1">Link 1</a>
<a href="#link2">Link 2</a>
<a href="#link3">Link 3</a>
</div>
</div>
</body>
</html>
Why this structure matters: It outlines a basic dropdown menu, setting the stage for more complex functionalities as your application grows. This structure allows for straightforward CSS styling and JavaScript interactions.
Common Issues Faced with Dropdown Menus
-
Browser Incompatibility
Different browsers may render dropdowns inconsistently, leading to layout issues or unexpected behaviors. A prevalent example is seen in Internet Explorer, which may fail to show the dropdown content when the parent element is positioned absolutely. -
Z-Index Problems
Z-index issues can result in dropdown menus being hidden behind other elements. Properly managing the stacking order is critical. -
Event Handling
JavaScript-based dropdowns may face problems if click events aren’t captured properly, leading to menus that won’t open or close as expected.
Fixing Browser Incompatibility
A significant issue arises when browsers like Internet Explorer render dropdown elements differently. To mitigate these problems, a robust approach is to encapsulate dropdown menus inside a controlled environment.
CSS Resets
One of the most effective techniques to ensure consistency across browsers is using CSS resets. Here’s a simple reset you can integrate:
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
Why use a CSS reset?
This ensures that all browsers start from the same baseline, making it easier to manage your styles consistently.
Responsive Styling with Flexbox
By employing Flexbox, dropdown menus can be styled to adapt better to different screen sizes, which is crucial for maintaining usability.
.dropdown {
position: relative;
display: inline-flex;
}
.dropdown-content {
display: none;
position: absolute;
z-index: 1;
background-color: white;
border: 1px solid #ccc;
}
.dropdown:hover .dropdown-content {
display: block;
}
Flexbox Insights
Using Flexbox for dropdown menus gives you more control over the alignment and spacing, enhancing the look without compromising functionality.
Handling Z-Index Issues
Z-index plays a vital role in rendering dropdowns. It determines the stack level of elements. Always ensure that your dropdown has a higher z-index than any overlapping content.
.dropdown-content {
z-index: 9999;
}
Why is this important?
A high z-index ensures that your dropdown remains on top of all other elements, allowing for better visibility and functionality.
JavaScript for Dynamic Dropdowns
Sometimes, CSS alone isn’t enough. Therefore, implementing JavaScript for dynamic behavior is critical to troubleshooting dropdown issues.
JavaScript Click Event
document.querySelector('.dropbtn').addEventListener('click', function() {
const dropdown = document.querySelector('.dropdown-content');
dropdown.style.display = (dropdown.style.display === 'block') ? 'none' : 'block';
});
Why use this approach?
This JavaScript enables toggling the dropdown visibility based on user interaction, addressing any event handling issues across browsers.
Accessibility Considerations
While aesthetic and functional aspects are imperative, don't overlook accessibility. Make sure your dropdowns are usable with keyboard navigation.
Adding Keyboard Support
document.querySelector('.dropbtn').addEventListener('keydown', function(event) {
if (event.key === 'Enter' || event.key === 'Space') {
// Trigger dropdown toggle
const dropdown = document.querySelector('.dropdown-content');
dropdown.style.display = (dropdown.style.display === 'block') ? 'none' : 'block';
event.preventDefault(); // Prevent default behavior
}
});
Why is keyboard support critical?
Ensuring users can navigate your dropdowns using keyboard commands enhances the overall accessibility, catering to all users, including those with disabilities.
Additional Resources
For further reading on fixing issues related to dropdown menus in different contexts, you can refer to helpful articles like Fixing Transparent Dropdown Menu Issues in Internet Explorer. This resource provides additional insight into resolving problems that may arise when dealing with legacy browsers.
To Wrap Things Up
Dropdown menus are essential for creating interactive, user-friendly web applications. However, tackling the bugs that may arise across different browsers is equally important to ensure a seamless experience for users. By implementing best practices like CSS resets, employing Flexbox for layout, and considering accessibility, you can significantly enhance user interaction.
Navigating the complexities of dropdown menus can seem daunting, but with attention to detail and a systematic approach, these challenges can be successfully overcome.
Armed with the strategies we discussed, you're now better equipped to handle dropdown menu bugs in your Java web applications. Happy coding!