Solving CORS Issues in Java Applications on Azure
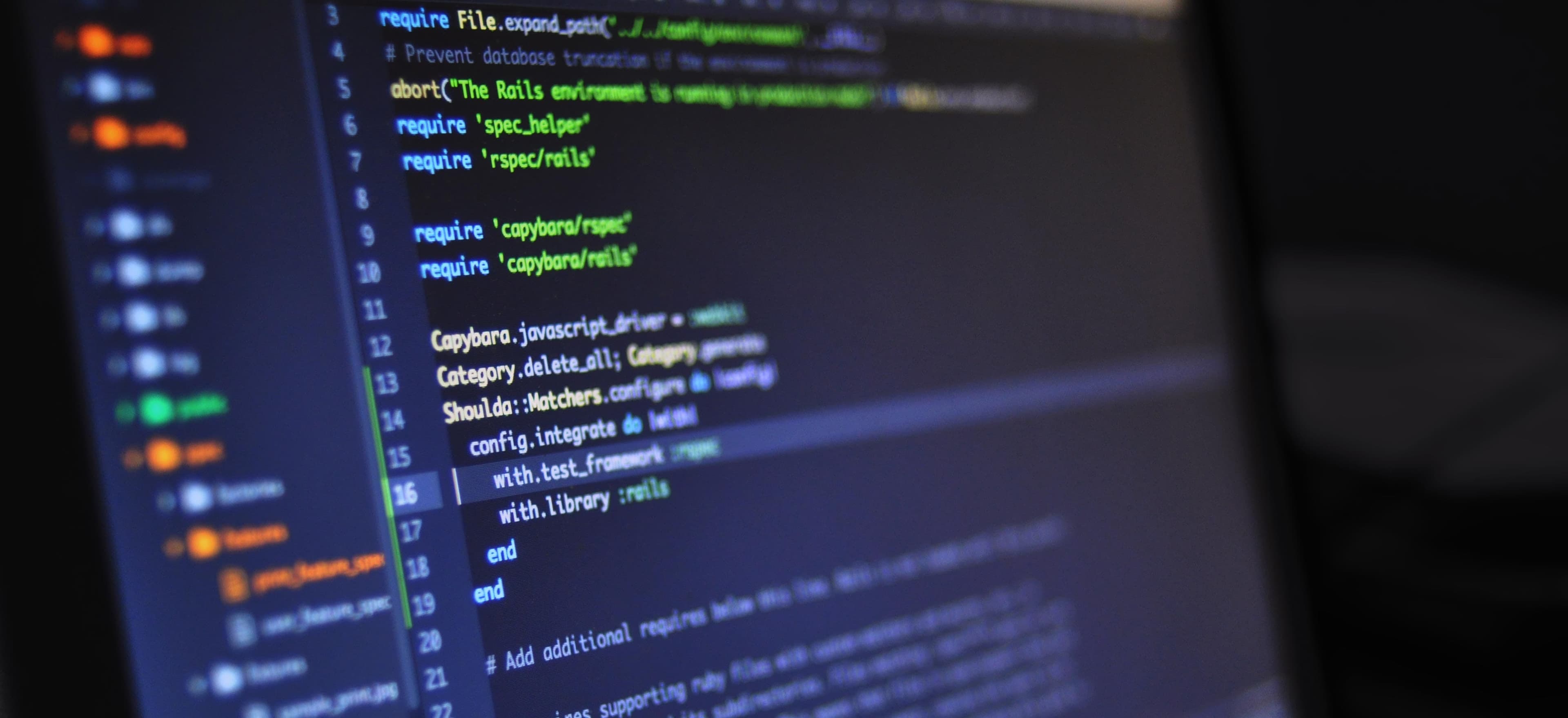
- Published on
Solving CORS Issues in Java Applications on Azure
When developing web applications, Cross-Origin Resource Sharing (CORS) can often seem like a complicated topic. This is especially true when you're deploying Java applications on Azure. As a developer, understanding how to properly manage CORS is essential for ensuring your application communicates effectively without compromising security. This blog post will guide you through common CORS issues, how to configure them in Java, and best practices to follow when deploying your application on Azure.
What is CORS?
CORS is a security feature implemented by browsers to prevent malicious websites from accessing resources from another domain without permission. Simply put, CORS specifies which web applications are allowed to interact with resources. It is controlled via HTTP headers, such as Access-Control-Allow-Origin
, which indicate which domains are permitted.
Why Are CORS Issues Common?
CORS issues arise when:
- The browser sends a request from one origin (domain) to another, such as a frontend application hosted on
example.com
trying to access resources onapi.example.com
. - The server does not respond with the appropriate CORS headers, resulting in the browser blocking the request.
Understanding CORS Errors
When we talk about errors in CORS, you might face issues like:
No 'Access-Control-Allow-Origin' header is present on the requested resource
The Same Origin Policy disallows reading the remote resource at [URL]
These errors can disrupt your application's functionality on Azure, leading to a frustrating experience for both developers and users alike.
Setting Up CORS in a Java Application
To remedy CORS issues in your Java application, you first need to configure your server to include the right headers. Below, we will look at several approaches depending on the framework you are using.
Using Spring Boot
Spring Boot is a popular framework for building web applications in Java. It allows you to manage CORS easily and efficiently.
Configuration Class
You can create a configuration class that implements WebMvcConfigurer
:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.config.annotation.CorsRegistry;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurer;
@Configuration
public class WebConfig implements WebMvcConfigurer {
@Override
public void addCorsMappings(CorsRegistry registry) {
registry.addMapping("/**") // Apply to all endpoints
.allowedOrigins("https://example.com") // Allowed origin
.allowedMethods("GET", "POST", "PUT", "DELETE") // Allowed methods
.allowedHeaders("*") // Allowed headers
.allowCredentials(true); // Allow credentials
}
}
Why This Code?
- The
addCorsMappings
method is where you define which endpoints should allow CORS requests. - The
allowedOrigins
method takes a string or an array of origins, specifying where the requests can come from. - The
allowedMethods
method defines which HTTP methods are supported for cross-origin requests. allowedHeaders
permits specific headers to be sent in requests.allowCredentials(true)
allows cookies and HTTP authentication to be included in CORS requests if needed.
Configuring CORS in a Servlet
If you are not using Spring Boot, you can also configure CORS in a Java Servlet like this:
import javax.servlet.Filter;
import javax.servlet.FilterChain;
import javax.servlet.FilterConfig;
import javax.servlet.ServletException;
import javax.servlet.ServletRequest;
import javax.servlet.ServletResponse;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
public class CORSFilter implements Filter {
@Override
public void doFilter(ServletRequest request, ServletResponse response, FilterChain chain)
throws IOException, ServletException {
HttpServletResponse httpResponse = (HttpServletResponse) response;
httpResponse.setHeader("Access-Control-Allow-Origin", "https://example.com");
httpResponse.setHeader("Access-Control-Allow-Methods", "GET, POST, PUT, DELETE");
httpResponse.setHeader("Access-Control-Allow-Headers", "Content-Type");
httpResponse.setHeader("Access-Control-Allow-Credentials", "true");
chain.doFilter(request, response);
}
@Override
public void init(FilterConfig filterConfig) throws ServletException {}
@Override
public void destroy() {}
}
Why Is This Important?
- This filter applies CORS settings to every response.
- You can flexibly modify allowed origins, methods, and headers as per application requirements.
Deployment on Azure
When deploying your Java application on Azure, ensure you have configured CORS both in Java and Azure settings. Azure provides a way to allow CORS in web applications through the portal or the Azure CLI.
Configuring CORS in Azure
-
Azure Portal: Navigate to your App Service >
CORS
in the settings menu. Here, you can add your allowed origins.!Azure CORS settings (hypothetical screenshot link)
-
Azure CLI: Use the following command to allow an origin using Azure CLI:
az webapp cors add --name myapp --resource-group myresourcegroup --allowed-origins https://example.com
Why It's Essential
Configuring CORS at the Azure level allows for an additional layer of security and flexibility, ensuring that your application can handle cross-origin requests appropriately.
Testing CORS
After setting up CORS, it's crucial to test it to ensure everything operates as intended.
- Browser Developer Tools: Use the Network tab in Developer Tools to check the response headers.
- Postman: Though not strictly mimicking a browser’s CORS policy, you can still send requests to test your API's response.
Best Practices for CORS
- Only allow origins that you control or trust.
- Avoid using wildcard
*
forallowedOrigins
in a production environment. - Regularly review allowed origins and methods as your application evolves.
- Log CORS requests to monitor potential attacks or misconfigurations.
To Wrap Things Up
Managing CORS issues in Java applications deployed on Azure is an essential skill for any web developer. By utilizing the techniques discussed above – using Spring Boot CORS configuration or implementing a custom filter for servlets – you can ensure a smoother experience for users while maintaining security. For further reading on related topics, consider checking out "Understanding CORS Issues in Azure: A Simple Guide".
With thorough attention to CORS settings at both the application and Azure level, you can build robust API-driven applications that seamlessly interact with front-end interfaces across domains. Happy coding!