Debugging Randomness: Common Pitfalls in Java's Math.random
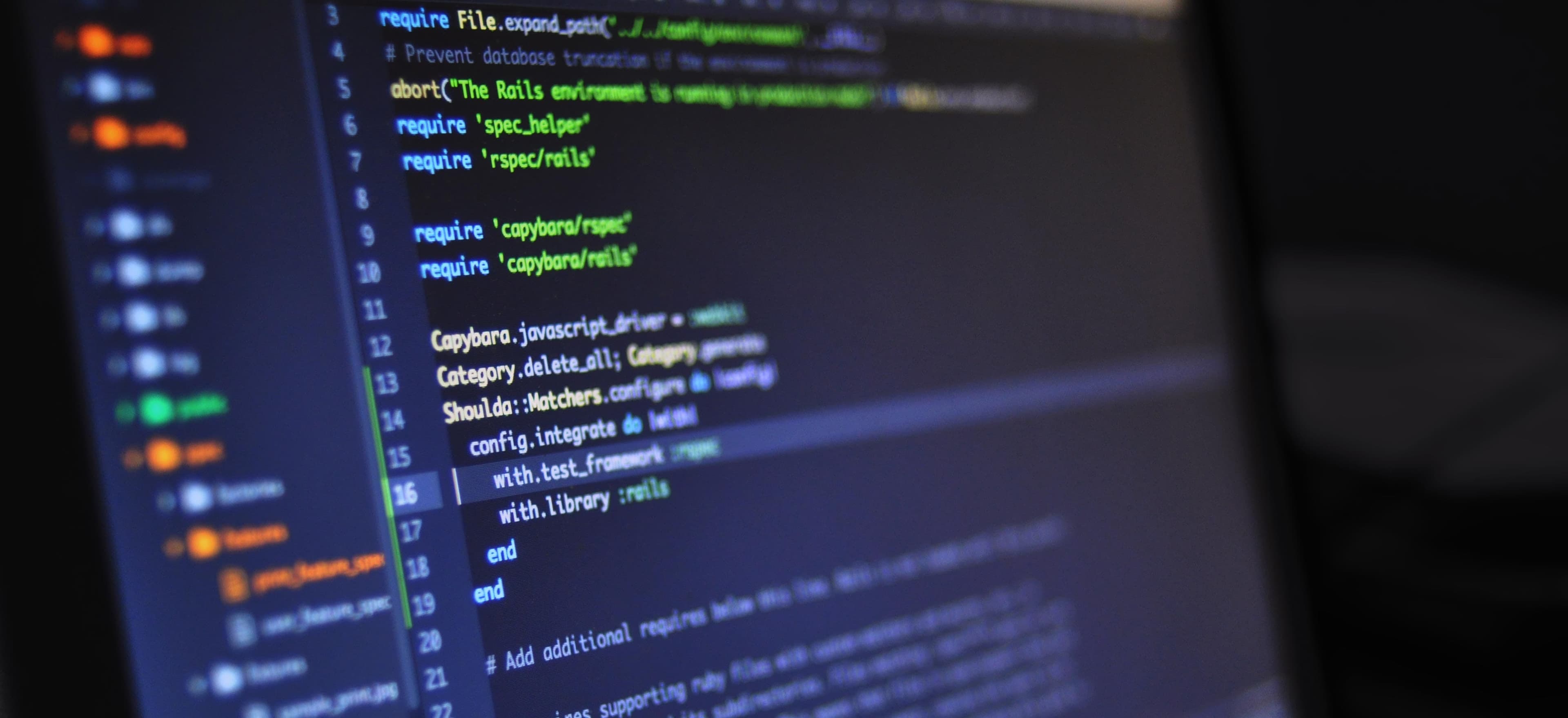
- Published on
Debugging Randomness: Common Pitfalls in Java's Math.random
When you're working with random numbers in Java, the first thing that might come to mind is Math.random()
. It's straightforward, it's convenient, and it's part of the standard library, making it a popular choice among developers. However, while this method may seem simple at first, various pitfalls can lead to unexpected behavior and frustrating bugs in your Java applications. This article aims to demystify the use of Math.random()
and show you how to navigate its common pitfalls effectively.
Understanding Math.random()
Before diving into typical issues, let’s clarify how Math.random()
works. This method generates a double value between 0.0 (inclusive) and 1.0 (exclusive). That means you will never get a result of 1.0, but you can get very close to it.
Here is a simple example of using Math.random()
:
public class RandomExample {
public static void main(String[] args) {
double randomValue = Math.random();
System.out.println("Random Value: " + randomValue);
}
}
The Why behind Math.random()
Why use Math.random()
? It’s built into Java, eliminates the need for external libraries for basic randomness, and is sufficiently random for non-secure applications. However, if you're attempting to use it for more complex scenarios, you may run into its limitations.
Common Pitfalls with Math.random()
1. Misunderstanding the Range of Values
A frequent mistake developers make is not understanding the range of values Math.random()
can generate. Since the method returns a double between 0.0 and 1.0, if you need a random integer in a different range, you must adjust the output appropriately.
Correct Usage:
For instance, if you want a random integer between 1 and 10, you can use this code:
int min = 1;
int max = 10;
int randomInt = (int) (Math.random() * (max - min + 1)) + min;
System.out.println("Random Integer: " + randomInt);
Why This Works
Why convert to int? The cast to int
truncates the decimal part, thus giving you a whole number. The multiplication scales the output to your desired range, and then the constant addition ensures that the minimum value is respected.
2. Lack of Seed Control
Another common misconception is the assumption that all random number generators (RNGs) behave the same way. Notably, Math.random()
does not allow you to control the seed, which means that you might get the same sequence of "random" numbers when your application runs multiple times.
If you need reproducible results (for testing, for example), a different approach is necessary.
Consider using the Random Class:
import java.util.Random;
public class RandomWithSeed {
public static void main(String[] args) {
Random random = new Random(12345); // Fixed seed
System.out.println("Random Integer: " + random.nextInt(10)); // Range 0-9
System.out.println("Random Integer: " + random.nextInt(10));
}
}
Why Use Random
?
This method offers a way to specify a seed, which results in generating the same sequence of numbers every time, making it invaluable for debugging and testing.
3. Overusing Randomness
Randomness isn't a magic solution for every problem. Some developers attempt to overuse random number generation in applications, which can lead to hard-to-read code and unexpected behavior.
Example of Overuse:
for (int i = 0; i < 10; i++) {
if (Math.random() > 0.5) {
// Do something
} else {
// Do something else
}
}
Why is it an Issue?
Uncontrolled randomness can lead to non-deterministic behavior that is challenging to debug. If you find yourself relying heavily on random values, reconsider the design and explore if there are more systematic, deterministic approaches you can implement.
4. Thread Safety
If you're working in a multi-threaded environment, keep in mind that Math.random()
is not thread-safe. Consequently, using it in concurrent contexts could lead to inconsistent results.
Using ThreadLocalRandom:
A more thread-safe alternative would be to utilize ThreadLocalRandom
:
import java.util.concurrent.ThreadLocalRandom;
public class ThreadSafeRandom {
public static void main(String[] args) {
// Generates a random integer in a thread-safe manner
int randomInt = ThreadLocalRandom.current().nextInt(1, 11); // Range 1-10
System.out.println("Thread-safe Random Integer: " + randomInt);
}
}
Why ThreadLocalRandom?
This class is designed for use in multi-threaded environments, providing better performance and avoiding contention among threads.
Real-World Applications of Math.random()
Despite its pitfalls, Math.random()
still holds value in many scenarios. Here are a few practical uses:
- Gaming: Randomly spawning enemies, loot generation, or dice rolls.
- Simulations: Creating randomized simulations in research or educational purposes.
- Simple Animations: Randomly modulating animation speeds or properties in visuals.
Additional Resources
To delve deeper into the randomness puzzle and explore potential issues further, it's worthwhile to look into the article titled Solving the Puzzle: Why Isn't Math.random Working?. This article offers additional insights into some problems related to Math.random()
and its nuances.
Final Considerations
Using Java's Math.random()
can be straightforward, but being aware of its limitations is crucial for writing effective and predictable code. Ensuring you understand its range, utilizing the Random
class for seeding, and being cautious in multi-threaded environments will help you make the most out of randomness in your applications.
Whether you decide to stick with Math.random()
or opt for a robust class like Random
or ThreadLocalRandom
, the key is understanding how each option fits your specific use case. Through proper application and awareness of potential pitfalls, you’ll navigate Java’s randomness landscape with confidence and success.