Debugging Java's Math.random: Common Issues Explained
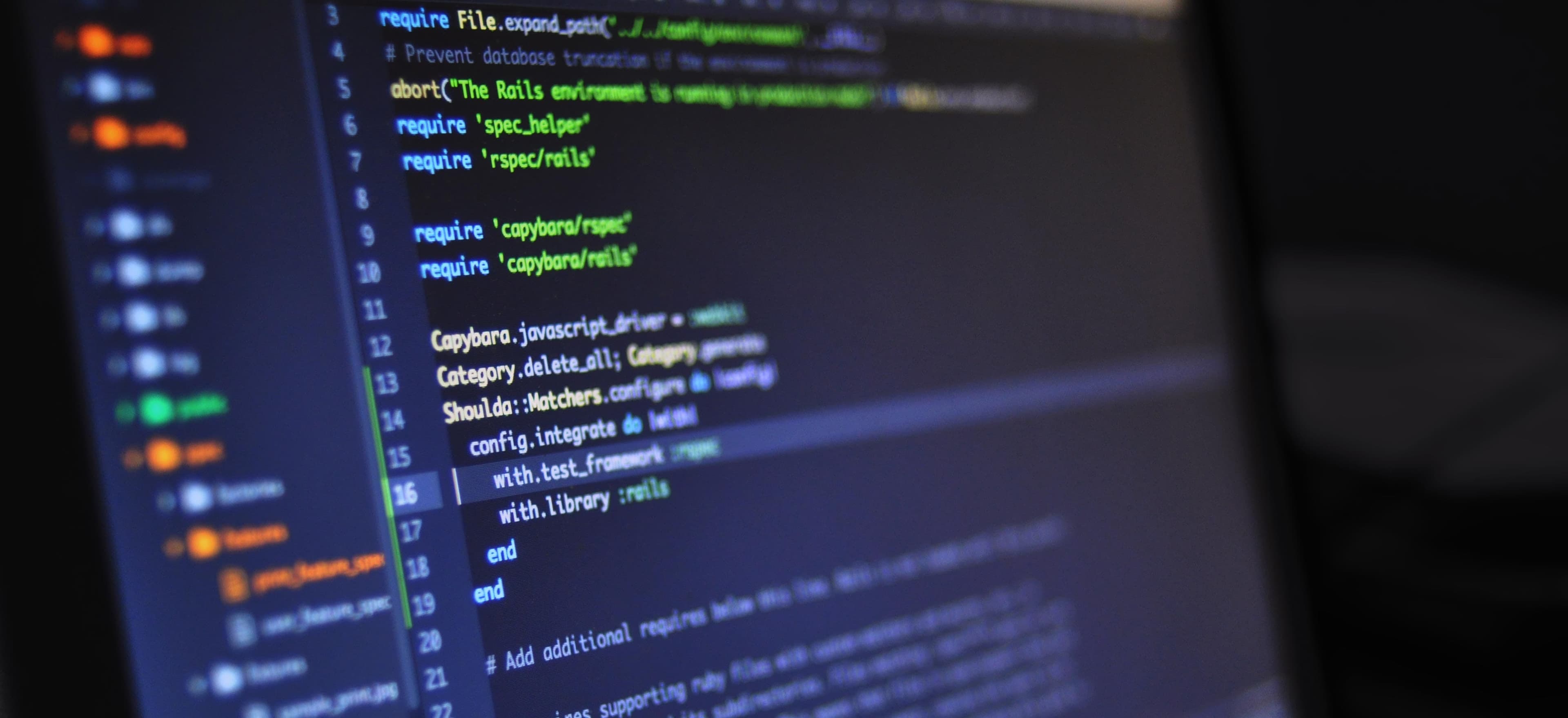
- Published on
Debugging Java's Math.random: Common Issues Explained
Java provides a straightforward way to generate random numbers using the Math.random
method. Although it's simple and effective, developers often encounter issues that lead to unexpected results. In this post, we'll delve into common pitfalls and how to debug them to ensure that your random number generation works as intended.
Understanding Math.random
The Math.random
method generates a double value in the range of 0.0 (inclusive) to 1.0 (exclusive). This is achieved through a pseudo-random number generator. The numbers returned are not genuinely random but rather determined by an algorithm, which, therefore, can reproduce the same sequence under identical conditions.
To use it effectively:
double randomValue = Math.random();
System.out.println(randomValue);
This code snippet outputs a random double value between 0.0 and 1.0. However, issues may arise in different scenarios, leading to confusion.
Common Issues with Math.random
1. Unexpected Range of Values
One of the most frequent errors comes from misunderstanding the range. If you need a random integer within a specific range, such as from 1 to 10, you need to adjust the output accordingly:
int min = 1;
int max = 10;
int randomInt = (int)(Math.random() * (max - min + 1)) + min;
System.out.println(randomInt);
Why this adjustment is necessary: Math.random()
alone produces a range from 0.0
to 1.0
, and multiplying by (max - min + 1)
scales this to the needed range. The cast to int
ensures we only deal with whole numbers.
2. Lack of Seed Control
A common misconception is that the random values are entirely random. The reality is that the sequence can repeat if the same seed is supplied to the random number generator. If your application calls Math.random
without a seed, it will generate numbers based on the system time when the JVM started.
Using java.util.Random
directly gives you better control over the randomness:
import java.util.Random;
Random rand = new Random();
int seededRandomInt = rand.nextInt(10) + 1; // Random integer from 1 to 10
System.out.println(seededRandomInt);
Using new Random(seed)
allows you to produce deterministic outputs for consistent testing.
3. Using it in Multithreaded Environments
When using Math.random
in a multithreaded context, problems can arise. The method is not thread-safe, which means if multiple threads call it at the same time, unpredictable results may occur.
To avoid this, use java.util.concurrent.ThreadLocalRandom
:
import java.util.concurrent.ThreadLocalRandom;
int threadSafeRandomInt = ThreadLocalRandom.current().nextInt(1, 11); // 1 to 10
System.out.println(threadSafeRandomInt);
This approach ensures each thread has its own instance of the random number generator, reducing contention and enhancing performance.
4. Understanding Repetition and Distribution
Even with good implementations, you may notice patterns or repetitions within the random numbers returned. This is a byproduct of the underlying algorithm of the random number generator. To address these issues, you can implement checks for number distributions.
If your application requires high-quality random numbers, consider using more advanced libraries like Apache Commons Math or similar.
Debugging Steps
When encountering unexpected behavior from Math.random
, follow these debugging steps:
- Check the Range: Ensure that you're adjusting the output for your desired range.
- Thread Safety: Re-evaluate how you're using
Math.random
in concurrent contexts. - Look for Patterns: Consider logging the output to examine patterns in the sequence of random numbers generated.
- Review Previous Articles: You might find insights from previous discussions, such as in "Solving the Puzzle: Why Isn't Math.random Working?" at tech-snags.com/articles/solving-puzzle-why-isnt-math-random-working.
Additional Resources
For a deeper understanding of generating random numbers in Java, check out this article on Java Generating Random Numbers. It provides a broad specter of options beyond Math.random
for different scenarios.
The Closing Argument
Although Math.random
serves as an easy-to-use tool for generating random numbers in Java, understanding its limitations is vital. Using techniques like proper range adjustment, seed management, and thread-safe alternatives can help address common pitfalls. By following the provided debugging steps, you can troubleshoot your issues effectively and ensure the random numbers generated enhance your applications' functionality.
Embrace randomness with confidence! Happy coding!
Checkout our other articles