Understanding Mocks vs. Stubs: A Quality Assurance Dilemma
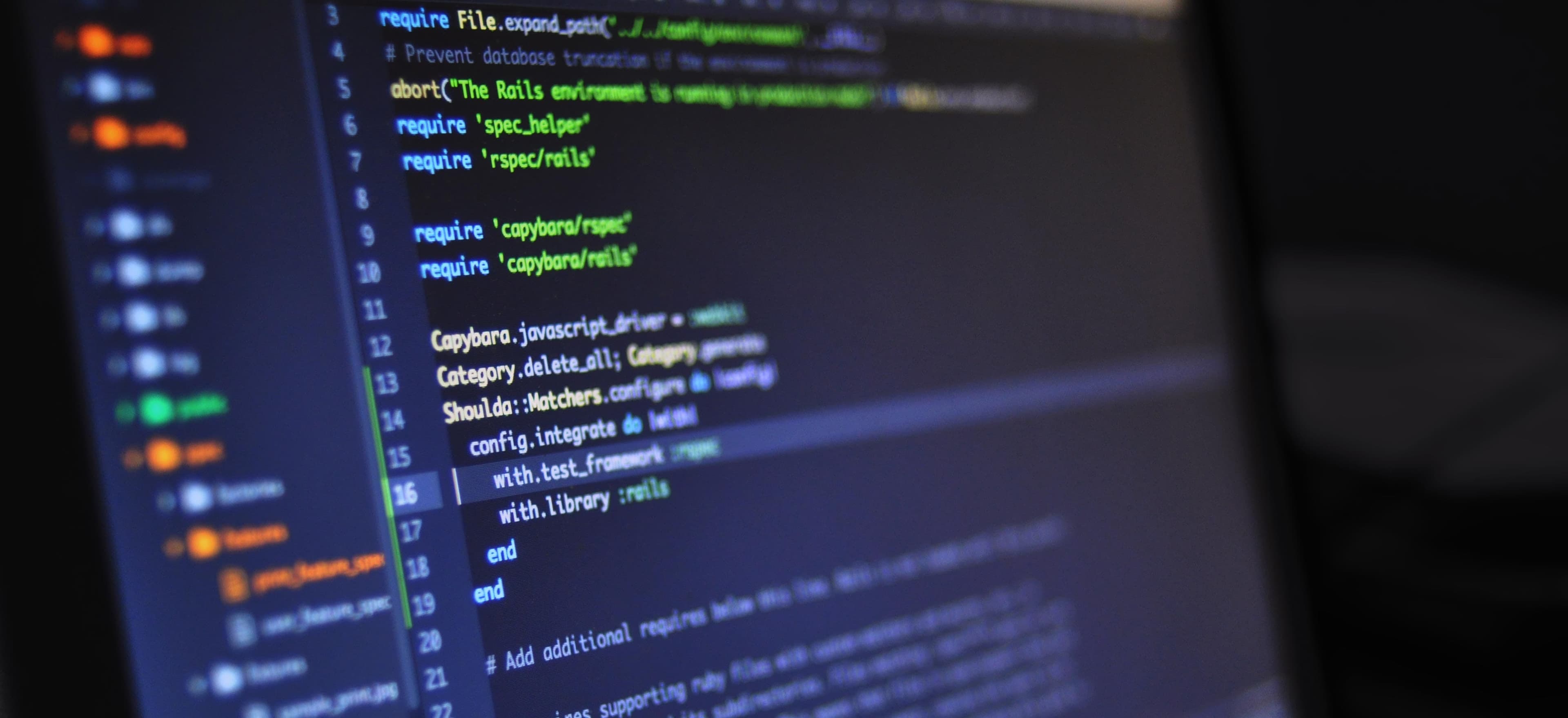
- Published on
Understanding Mocks vs. Stubs: A Quality Assurance Dilemma
Quality Assurance (QA) plays a critical role in ensuring software quality, functionality, and customer satisfaction. In the realm of testing, two essential concepts often arise: mocks and stubs. While both serve specific purposes in testing, they exhibit distinct differences. In this blog post, we will dissect the concepts of mocks and stubs, their use cases, and best practices for incorporating them into your Java development process.
What are Stubs?
In testing, a stub is a simplistic implementation of an interface or a function that simulates the behavior of a component. Stubs are used when you want to isolate a unit under test without necessitating dependencies on other components. While they provide predefined responses, they do not have logic for any interaction.
Why Use Stubs?
- Isolation: They allow you to test the component in isolation.
- Control: You can easily control the input and output of the stubbed methods.
- Eliminate Dependencies: You can remove the need for complex setup of dependencies in your tests.
Example of Stubs in Java
Let’s consider an example of a service that interacts with an external API to fetch user data.
public interface UserService {
User getUser(String userId);
}
public class UserController {
private final UserService userService;
public UserController(UserService userService) {
this.userService = userService;
}
public User getUser(String userId) {
return userService.getUser(userId);
}
}
// Stub Implementation
class UserServiceStub implements UserService {
@Override
public User getUser(String userId) {
// Return a fixed user regardless of input
return new User(userId, "John Doe");
}
}
// Test Case
public class UserControllerTest {
@Test
public void testGetUser() {
// Arrange
UserService stub = new UserServiceStub();
UserController userController = new UserController(stub);
// Act
User user = userController.getUser("123");
// Assert
assertEquals("John Doe", user.getName());
}
}
In the code above, UserService
is the interface we want to test, and UserController
is the component that depends on it. The UserServiceStub
class simulates UserService
, giving us complete control over the output of getUser()
.
What are Mocks?
On the other hand, a mock is a specialized version of a stub that records the interactions with the component being tested. Mocks not only replace the dependencies but also allow for verification of how they were used. They are more complex than stubs as they facilitate behavior verification.
Why Use Mocks?
- Verification: Mocks can confirm if a method was called and how many times.
- Behavior Testing: Facilitates testing of how code interacts with external components.
- Fail Fast: You can easily identify issues in behavior during testing.
Example of Mocks in Java
Let’s extend our previous example by introducing a mock:
import static org.mockito.Mockito.*;
public class UserServiceTest {
@Test
public void testGetUser() {
// Create a mock object
UserService mockedService = mock(UserService.class);
// Define behavior
when(mockedService.getUser("123")).thenReturn(new User("123", "Jane Doe"));
UserController userController = new UserController(mockedService);
// Act
User user = userController.getUser("123");
// Verify the interaction
verify(mockedService).getUser("123");
// Assert
assertEquals("Jane Doe", user.getName());
}
}
In this example, we make use of the well-known Mockito framework, which allows us to create mock objects seamlessly. Here, mock(UserService.class)
creates a mock of UserService
, and we set an expectation using when(...).thenReturn(...)
.
Key Differences Between Mocks and Stubs
Understanding the core differences will help you decide when to use which.
| Feature | Stubs | Mocks | |----------------|-------------------------------------|------------------------------------------| | Purpose | Simplifies other components | Records interactions for verification | | Behavior | Fixed responses | Flexible response can be defined | | Verification | No verification | Interaction verification | | Complexity | Simpler to implement | More complex to implement and manage |
Best Practices for Using Mocks and Stubs
1. Use Stubs for Stateless Components
When testing a component relying on services that don't change state, stubs are usually sufficient. They provide a clean and maintainable way to ensure that functionality remains intact.
2. Use Mocks for Interaction Testing
When the behavior of the component is crucial to its functionality, it’s wise to utilize mocks. This increases your test robustness as you can confirm that interactions happen as expected.
3. Don't Overuse Mocks
While mocks are handy, overusing them can lead to fragile tests. Ideally, rely on them when necessary, and if interaction isn’t critical, opt for stubs.
4. Maintain Clear Tests
Remember, your tests should be understandable. If your solution is convoluted and requires intricate mocks, consider whether your design can be simplified to make testing more straightforward.
Final Considerations
Mocks and stubs are both essential tools in the testing toolbox. Each serves a distinct yet essential role in the quality assurance process. They not only help to isolate components for testing but also verify that components interact as expected.
As you continue to work with Java and navigate testing frameworks, consider incorporating mocks and stubs strategically into your tests. This will not only improve code adherence to specifications but also facilitate smoother integration and deployment.
Further Reading
By understanding and leveraging these tools, you can enhance the quality of your Java applications significantly. Happy coding and testing!