Fixing JDK 12's Files Mismatch Method: Common Pitfalls
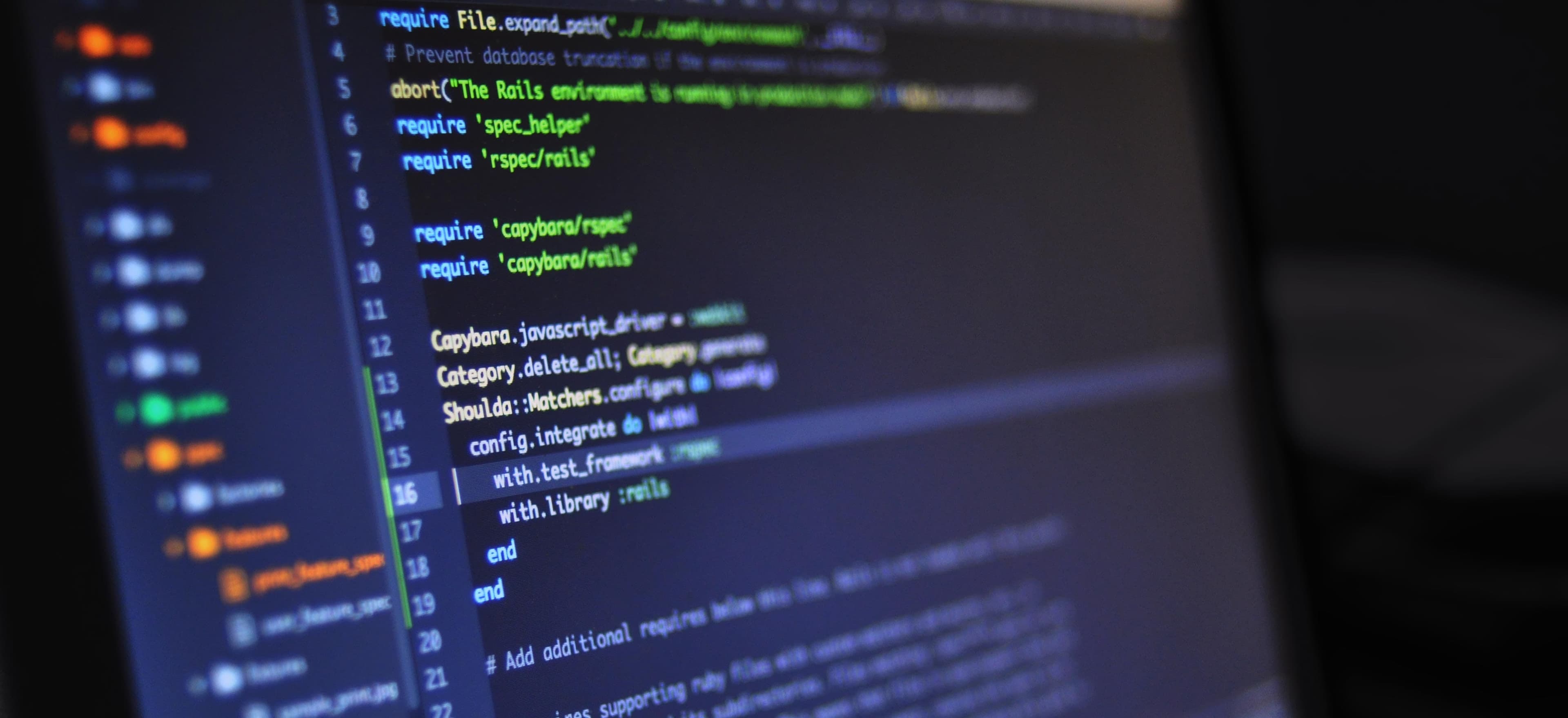
- Published on
Fixing JDK 12's Files Mismatch Method: Common Pitfalls
Java has continued to evolve with each new version, enhancing the standard library and offering better ways to handle various tasks. With the advent of JDK 12, the Files
class introduced the mismatch
method, allowing developers to efficiently compare two files. While this method provides a robust utility, developers frequently encounter pitfalls when using it.
In this blog post, we will discuss the mismatch
method, common pitfalls faced by developers, and provide fixes and best practices to avoid these issues. We will also include code snippets to illustrate the discussed points, ensuring you grasp the concepts thoroughly.
Understanding the Files Mismatch Method
The Files.mismatch(Path first, Path second)
method in JDK 12 is designed to find the position of the first differing byte between two files. If the files are identical, it returns -1
. On the other hand, if the method encounters an issue (like one of the files not existing), it throws an IOException
.
Example of the Mismatch Method
Here’s a simple example to demonstrate the use of the mismatch
method:
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.io.IOException;
public class FileMismatchExample {
public static void main(String[] args) {
Path file1 = Paths.get("example1.txt");
Path file2 = Paths.get("example2.txt");
try {
long mismatchPosition = Files.mismatch(file1, file2);
if (mismatchPosition == -1) {
System.out.println("The files are identical.");
} else {
System.out.println("Files differ at byte position: " + mismatchPosition);
}
} catch (IOException e) {
System.err.println("An I/O error occurred: " + e.getMessage());
}
}
}
Key Takeaways from the Example
- Path Representation: We use
Paths.get()
to createPath
objects representing our files. - Error Handling: Always handle
IOException
to deal with potential issues such as missing files. - Return Value: The return value using
mismatch
helps you know where the files differ.
Common Pitfalls
Despite its simplicity, using Files.mismatch
can lead to several common pitfalls. Let’s dive into these issues and explore solutions and best practices.
1. Handling Non-existent Files
One of the most common pitfalls is failing to check if the files exist before calling Files.mismatch
. If either file does not exist, an IOException
will be thrown, and it can lead to unexpected crashes in your application.
Solution
Always verify the existence of files using Files.exists()
:
if (Files.exists(file1) && Files.exists(file2)) {
// Proceed with mismatch
} else {
System.err.println("One or both files do not exist.");
}
2. Not Considering File Permissions
Another issue arises when dealing with files that do not have the appropriate permissions. Even if the files exist, lack of read permissions can also lead to IOException
.
Solution
Check file permissions before attempting to call mismatch
:
if (Files.isReadable(file1) && Files.isReadable(file2)) {
// Proceed with mismatch
} else {
System.err.println("Cannot read one or both files.");
}
3. Large Files Performance
When comparing large files, performance can become a concern. Since Files.mismatch
reads through files byte by byte until it finds a difference, larger files can take longer.
Solution
When dealing with very large files, consider implementing a more efficient algorithm or using other libraries to improve performance, such as Apache Commons IO for other file comparisons.
You can read more on file operations with Apache Commons here.
4. Handling Different File Encodings
When comparing text files, it’s essential to understand that differences in character encoding can cause unexpected results. For instance, a file saved in UTF-8 may differ from one saved in ISO-8859-1.
Solution
If you are comparing text files, ensure they share the same encoding. You can use Java’s Files.readAllLines()
to read files as lines and perform string comparisons explicitly. It is ideal for text content as it allows for encoding specification.
import java.nio.charset.StandardCharsets;
// Read text files with proper encoding
List<String> lines1 = Files.readAllLines(file1, StandardCharsets.UTF_8);
List<String> lines2 = Files.readAllLines(file2, StandardCharsets.UTF_8);
// Compare lines
for (int i = 0; i < Math.min(lines1.size(), lines2.size()); i++) {
if (!lines1.get(i).equals(lines2.get(i))) {
System.out.println("Files differ at line: " + (i + 1));
}
}
5. Ignoring Possible Errors and Exceptions
A common mistake is to ignore exceptions, particularly when writing code to perform file comparisons. If a potential error occurs, not handling it can lead to silent failures.
Solution
Always log exceptions appropriately. It’s often helpful to use a logging framework rather than merely printing stack traces. A logging framework provides more flexibility and control over your error handling strategy.
My Closing Thoughts on the Matter
In summary, while JDK 12's Files.mismatch
method provides a streamlined way to compare files, understanding its common pitfalls is essential for developers. Always check for file existence and permissions, consider performance implications, be mindful of character encodings, and handle exceptions properly.
By following best practices and learned from common pitfalls, you'll improve both the reliability and performance of your file comparison logic. For deeper insights into I/O operations, check out the official Java documentation on the Java NIO (New Input/Output).
Happy coding!
Checkout our other articles