Common Redis Pitfalls: Avoiding Key-Value Mismanagement
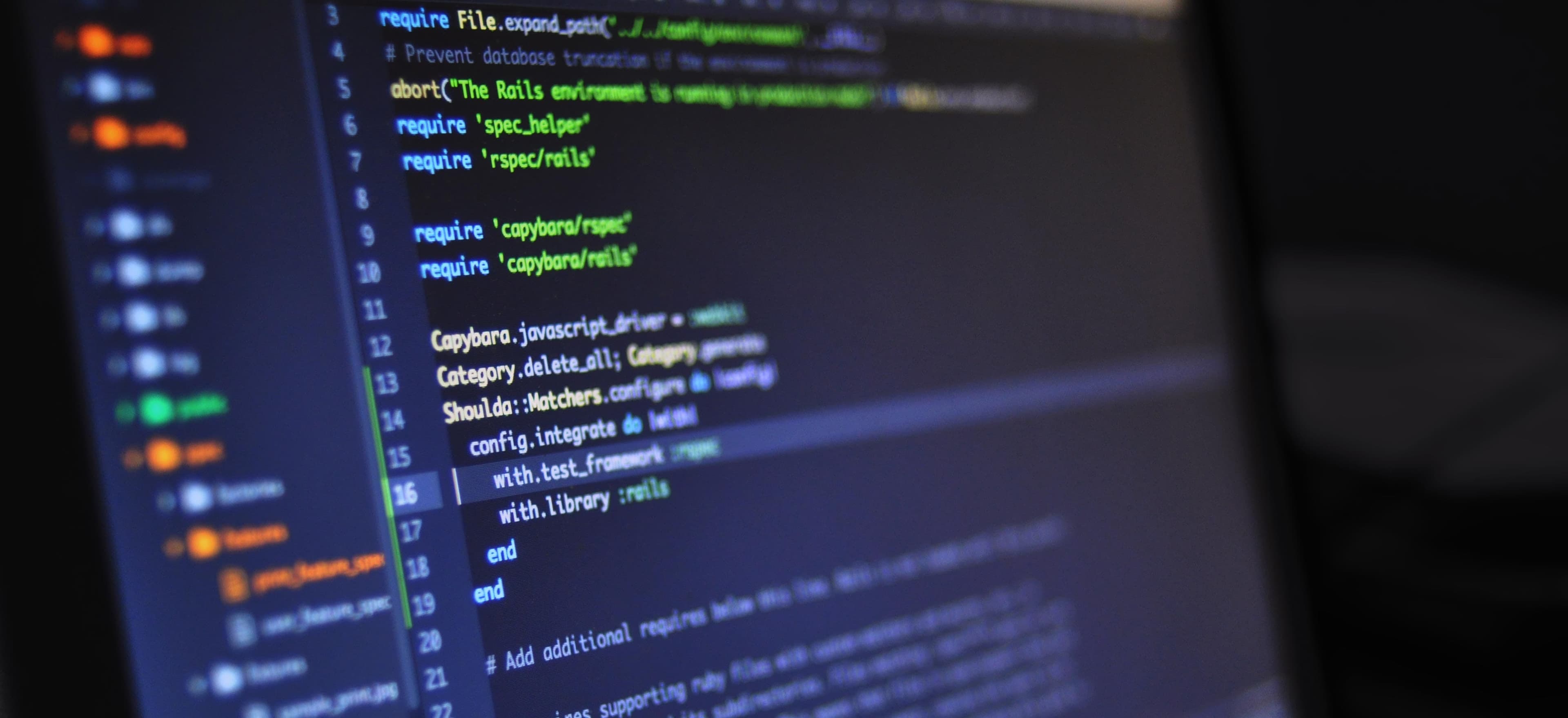
- Published on
Common Redis Pitfalls: Avoiding Key-Value Mismanagement
Redis, renowned for its speed and versatility, serves as a preferred key-value store for various applications, from caching solutions to real-time analytics. However, as with any tool, mismanagement can lead to inefficiencies and complications. In this blog post, we'll explore common pitfalls in Redis usage and how to avoid them to ensure your key-value store remains optimized.
Understanding Redis
Before we delve into the pitfalls, it is crucial to have a firm grasp of what Redis is. Redis (REmote DIctionary Server) is an in-memory data structure store that can be used as a database, cache, and message broker. Redis supports various data structures such as strings, hashes, lists, sets, and sorted sets, and it does so with remarkable speed.
Despite its advantages, improper handling can lead to several challenges. Let's look at some of the most common pitfalls and how to steer clear of them.
Pitfall #1: Improper Key Naming Conventions
Why It Matters
Key naming conventions are vital for the maintainability of your Redis database. Poorly named keys can lead to confusion, making it difficult to manage the data over time.
Best Practices
-
Use a Natural Hierarchy: Adopt a hierarchical naming structure. For instance, if you are storing user-related data, use prefixes like
user:123:profile
anduser:123:settings
. This method helps categorize data effectively. -
Be Descriptive: Descriptive names help convey the data type and its contents. This is especially useful for teams when they need to understand what each key represents.
-
Avoid Generic Names: Generic key names like
data1
,data2
provide no context. Avoid them.
Code Sample
// Correct way to create keys
String userId = "123";
String profileKey = "user:" + userId + ":profile";
String settingsKey = "user:" + userId + ":settings";
redisTemplate.opsForValue().set(profileKey, userProfileData);
redisTemplate.opsForValue().set(settingsKey, userSettingsData);
This structure allows you to manage keys easily, and the context-driven naming enhances readability.
Pitfall #2: Ignoring Expiry Policies
Why It Matters
Keys in Redis do not have to persist indefinitely. If certain data is temporary, failing to set an expiry can waste memory and affect performance.
Best Practices
-
Set Expiry for Temporary Data: Whenever you store temporary data, consider using the
EXPIRE
command or set an expiration time when storing the key. -
Monitor Memory Usage: Use tools like
INFO memory
to keep track of memory utilization.
Code Sample
String sessionKey = "session:" + userId;
redisTemplate.opsForValue().set(sessionKey, sessionData, 30, TimeUnit.MINUTES); // Set key with expiration
This ensures that the session data is automatically removed after 30 minutes, preventing memory bloat.
Pitfall #3: Using Blocking Operations Without Due Care
Why It Matters
Blocking operations, such as BRPOP
or BLPOP
, can cause your application to hang indefinitely if not handled carefully.
Best Practices
-
Set Timeouts: Always set a timeout for blocking operations to prevent indefinite blocking.
-
Error Handling: Ensure that your code has proper error handling in place.
Code Sample
String queueKey = "taskQueue";
List<String> task = redisTemplate.opsForList().range(queueKey, 0, -1);
// Safe blocking operation
try {
String task = redisTemplate.getConnectionFactory().getConnection().brPop(5, queueKey.getBytes()).toString();
} catch (Exception e) {
System.err.println("Error while consuming from queue: " + e.getMessage());
}
In this snippet, a blocking pop operation is performed with a timeout of 5 seconds, ensuring that the application does not hang indefinitely.
Pitfall #4: Overusing the Redis Cache as Primary Database
Why It Matters
Redis is excellent for caching, but relying on it as a primary database can lead to data loss, especially in cases of crashes.
Best Practices
-
Understand Data Durability: Know the persistence options that Redis offers, such as RDB and AOF (Append Only File). Choose the one that best fits your data durability requirements.
-
Use Redis for Temporary Data: Store temporary or frequently accessed data in Redis while using a more persistent database for critical data.
Code Sample
// Configuring Redis for persistence
@Bean
public RedisConnectionFactory redisConnectionFactory() {
RedisStandaloneConfiguration configuration = new RedisStandaloneConfiguration("localhost", 6379);
configuration.setDatabase(0);
configuration.setPassword(RedisPassword.of("your_password"));
JedisConnectionFactory factory = new JedisConnectionFactory(configuration);
factory.setUsePool(true);
return factory;
}
In this setup, we are using a connection factory with proper configuration for secure access to Redis, ensuring a balance between performance and data safety.
Pitfall #5: Neglecting to Monitor Performance
Why It Matters
Monitoring Redis performance is essential to prevent bottlenecks and availability issues. Without monitoring, it's hard to diagnose slow commands or memory issues.
Best Practices
-
Utilize Redis Monitoring Tools: Tools like
Redis Monitor
,Redis Insights
, or built-in commands likeMONITOR
,SLOWLOG
, allow you to keep track of performance metrics. -
Set Alerts: Implement alerting for high memory usage or long response times.
Code Sample
# Use the Redis CLI to monitor commands in real-time
redis-cli MONITOR
# Monitor slow queries
redis-cli SLOWLOG get 10
These commands allow developers to actively monitor operational performance, making diagnosing slow queries much easier.
A Final Look
While Redis offers unparalleled speed and flexibility for application data management, is essential to avoid common pitfalls in key-value mismanagement. By instilling proper key naming conventions, setting expiry policies, managing blocking operations wisely, understanding appropriate use of caching, and continuously monitoring performance, you can leverage Redis effectively.
Redis remains a powerful tool when used correctly. Implement these best practices and transform your Redis strategies, ensuring a robust, high-performing application.
For more information on Redis, check out the official Redis documentation and start implementing these best practices today!