Overcoming Java Microservices Deployment Challenges with Docker
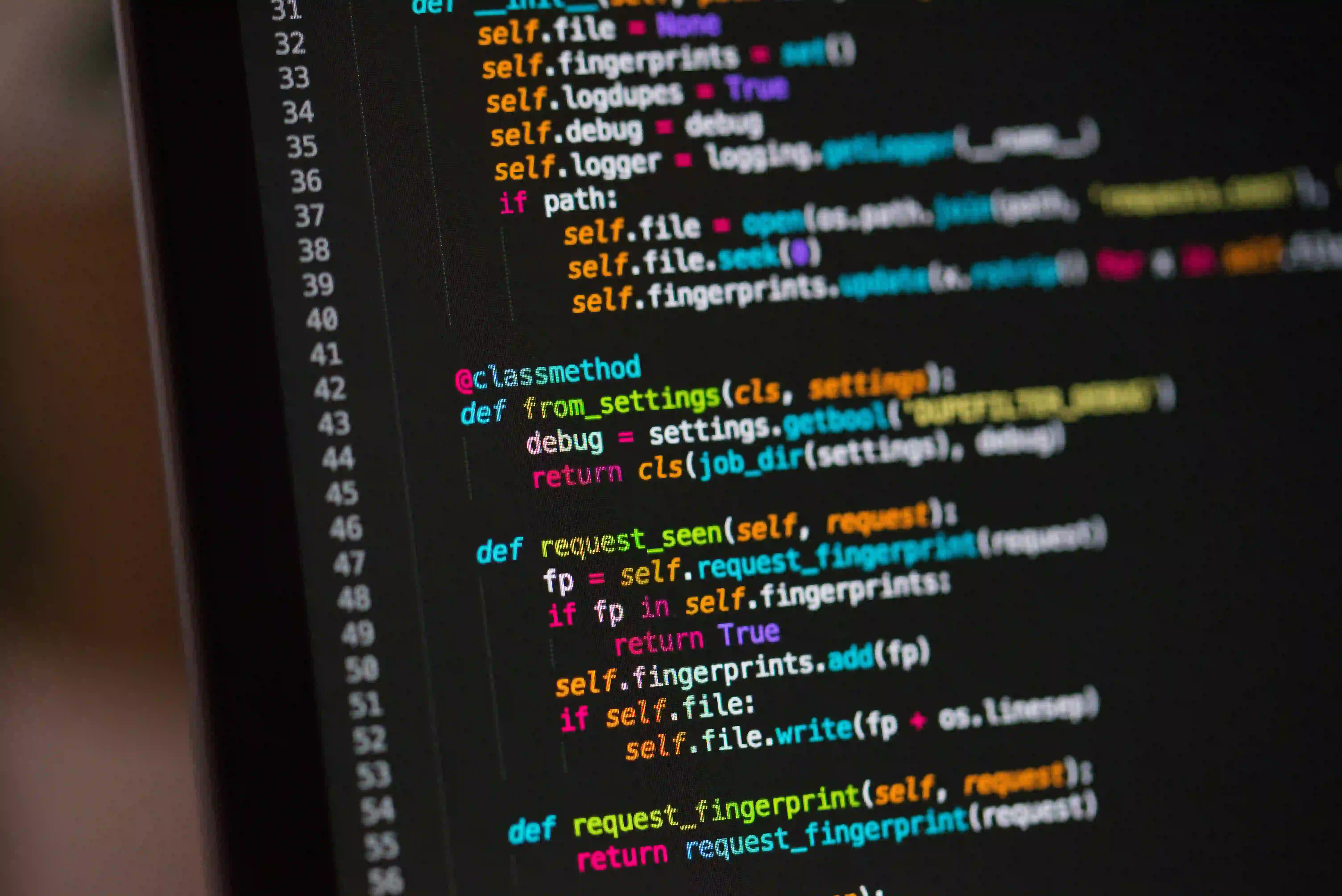
Overcoming Java Microservices Deployment Challenges with Docker
In the rapidly evolving landscape of software development, microservices architecture has emerged as a crucial pattern for building scalable and maintainable applications. Java is one of the most popular languages for implementing these microservices, given its robustness and ecosystem. However, deployment remains a key challenge. This is where Docker steps in as a powerful tool to streamline the deployment process of Java microservices.
In this post, we'll explore the common deployment challenges, how Docker can help, and showcase practical examples to solidify these concepts.
Understanding the Deployment Challenges
Deploying Java microservices can come with several hurdles:
-
Environment Consistency: Differences in development, testing, and production environments can lead to "it works on my machine" issues.
-
Dependency Management: Java applications often have multiple dependencies, leading to complex configurations for deployment.
-
Scalability and Load Balancing: As the application grows, managing instances of microservices becomes increasingly difficult.
-
Resource Utilization: Running multiple microservices can lead to inefficient use of server resources.
-
Monitoring and Logging: Tracking multiple microservice deployments separately can complicate debugging and performance tracking.
To tackle these issues, Docker can provide a consistent, portable solution.
What is Docker?
Docker is an open-source platform that enables developers to automate the deployment of applications inside lightweight containers. A container is a standardized unit of software that combines application code with its libraries and dependencies, allowing for seamless deployment across different environments.
Why Use Docker for Java Microservices?
- Environment Consistency: Docker containers provide a uniform platform irrespective of where they are deployed.
- Isolation: Each microservice runs in its container, isolating dependencies and preventing conflicts.
- Scalability: Docker makes it easy to replicate containers to handle traffic spikes.
- Resource Efficiency: Containers share the OS kernel and use fewer resources than traditional VMs.
Setting Up Docker for Java Microservices
Letβs consider a basic example of deploying a Java microservice using Docker. We will create a simple Spring Boot application as our microservice.
Step 1: Create a Simple Spring Boot Application
Start by generating a Spring Boot application. You can use the Spring Initializr (https://start.spring.io/) and select the following dependencies:
- Spring Web
- Spring Boot DevTools
- Spring Data JPA (optional for database interactions)
Directory Structure:
my-java-microservice
β-- src
β β-- main
β β-- java
β β-- com
β β-- example
β β-- myjavams
β β-- MyJavaMicroserviceApplication.java
β β-- controller
β β-- GreetingController.java
β-- Dockerfile
β-- pom.xml
Here is a simple example of the GreetingController.java
:
package com.example.myjavams.controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class GreetingController {
@GetMapping("/greet")
public String greet() {
return "Hello, Welcome to the Java Microservice!";
}
}
This controller maps the /greet
endpoint and returns a welcome message.
Step 2: Writing the Dockerfile
Next, within the root directory (my-java-microservice
), create a file named Dockerfile
.
# Use the official OpenJDK image as a parent image
FROM openjdk:11-jre-slim
# Set the working directory in the container
WORKDIR /app
# Copy the jar file from the target directory into the container
COPY target/my-java-microservice-0.0.1-SNAPSHOT.jar app.jar
# Specify the command to run the application
ENTRYPOINT ["java", "-jar", "app.jar"]
Commentary:
FROM openjdk:11-jre-slim
: This line specifies the base image, using a minimal version of Java JRE.WORKDIR /app
: Sets the working directory for subsequent instructions. This helps in organizing your application files.COPY
: Copies the compiled JAR file into the container.ENTRYPOINT
: Defines the command to run the Java application.
Step 3: Building the Docker Image
Navigate to the root directory of your project in the command line and build the Docker image:
mvn clean package
docker build -t my-java-microservice .
This command will compile your Spring Boot application and create a Docker image named my-java-microservice
.
Step 4: Running the Docker Container
Now that you have your Docker image, you can run your microservice with the following command:
docker run -p 8080:8080 my-java-microservice
Here, the -p
flag maps port 8080 of the host to port 8080 of the container, allowing us to access the microservice from the host machine.
Testing Your Microservice
Once the container is running, you can access your Java microservice by navigating to this URL in your web browser or with a tool like Postman:
http://localhost:8080/greet
You should see the response: "Hello, Welcome to the Java Microservice!"
Step 5: Orchestrating Containers with Docker Compose
As your architectures grow, managing multiple containers becomes necessary. This is where Docker Compose comes in.
Create a docker-compose.yml
file in your root directory:
version: '3'
services:
myjavams:
image: my-java-microservice
ports:
- "8080:8080"
Running Docker Compose:
To start your services defined in the docker-compose.yml
, run:
docker-compose up
This command builds and starts containers based on the configuration in the YAML file.
In Conclusion, Here is What Matters
Docker streamlines the deployment of Java microservices by providing consistency, scalability, and effective resource management. By containerizing your Java applications, you are set for smoother deployments, which is incredibly beneficial in an ever-changing development landscape.
For further reading on microservices and Docker, consider these resources:
- Spring Boot Microservices by Spring.
- Docker Official Documentation for more details on Docker's features.
Adopting Docker for your Java microservices will not only enhance your deployment strategy but also contribute to a more structured and efficient development lifecycle. As you embark on this journey, remember that the transition to microservices and containerization is iterative. Embrace the learning process and watch your applications thrive in the cloud!