Conquering JVM's Stop-The-World Pauses in Logging Systems
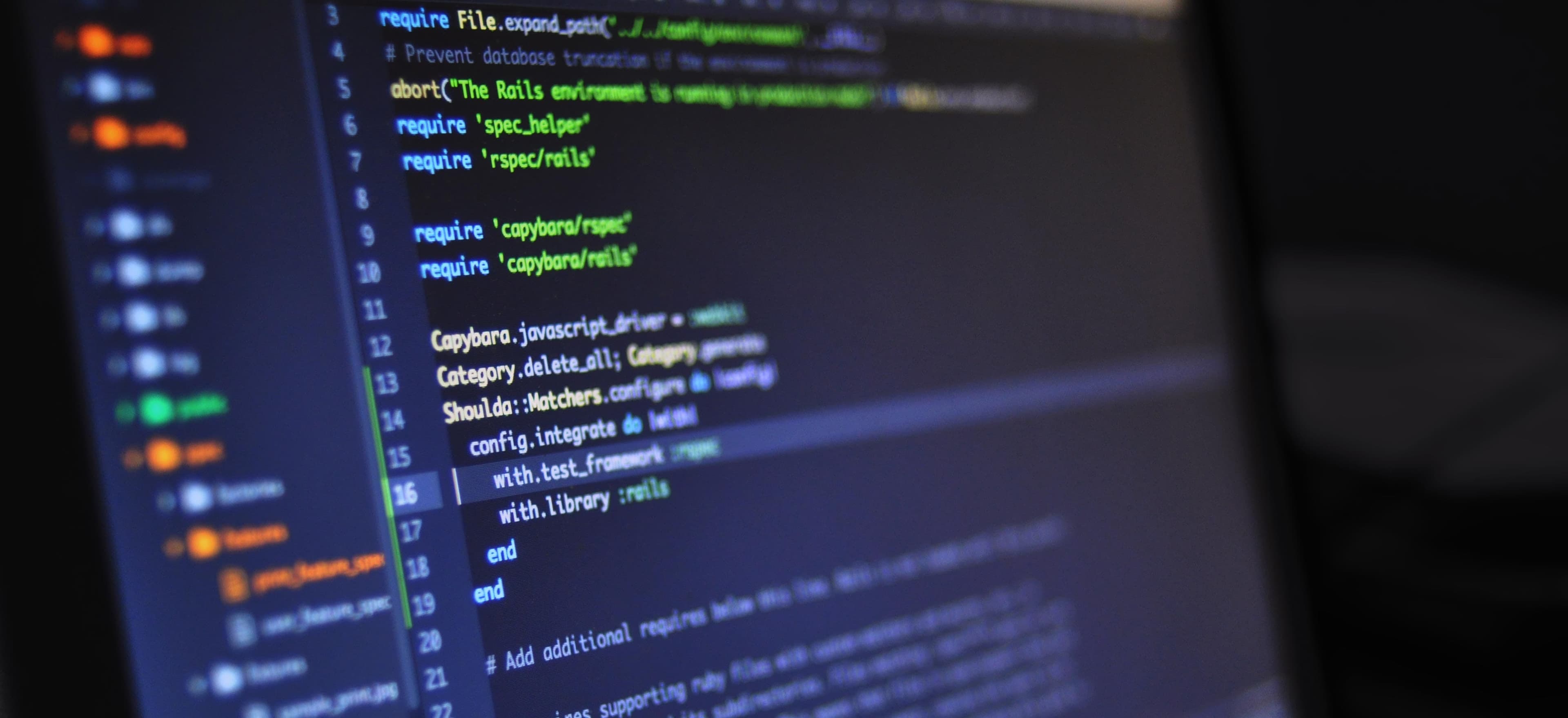
- Published on
Conquering JVM's Stop-The-World Pauses in Logging Systems
Java is known for its robustness and performance, but one of its Achilles' heels is the dreaded Stop-The-World (STW) pauses in the Java Virtual Machine (JVM). This can particularly wreak havoc in logging systems, where timely data recording is critical for performance monitoring and debugging. In this blog post, we will delve into the nature of STW pauses, how they impact logging systems, and what strategies can be employed to mitigate their effects.
Understanding Stop-The-World (STW) Pauses
Before we dive deeper, it’s essential to understand what STW pauses are. The JVM operates on a garbage collection (GC) mechanism, and during this process, it periodically frees up memory by reclaiming unused objects. A Stop-The-World pause occurs when the application freezes to allow the JVM to perform certain operations, like garbage collection.
Why Are STW Pauses Problematic?
In most systems, especially in scalable applications, frequent STW pauses can cause issues such as:
- Latency: Long delays may cause significant latency spikes, affecting performance.
- Loss of Data: In a logging context, events may be lost during the pause.
- Thread Disruption: All threads are halted, leading to irregular application behavior.
The Impact on Logging
Both application performance and user experience can be severely impacted by STW pauses in logging systems. For example, suppose your application logs critical user actions or system events. If a STW pause occurs, it could mean that important logs are not captured in real-time.
This is particularly concerning for applications in sectors like finance or healthcare, where regulatory compliance and audit logs must be meticulously maintained.
// Example of logging code with potential STW issues
import java.io.FileWriter;
import java.io.IOException;
import java.util.logging.Logger;
public class LoggingExample {
private static final Logger logger = Logger.getLogger(LoggingExample.class.getName());
public static void logMessage(String message) {
// The following could trigger Stop-The-World pauses
try (FileWriter fileWriter = new FileWriter("log.txt", true)) {
fileWriter.write(message + "\n");
} catch (IOException e) {
e.printStackTrace();
}
}
}
The above code may lead to STW pauses due to file I/O operations, which block threads while waiting for the disk access.
Strategies to Mitigate STW Pauses
To reduce STW impacts, consider the following strategies:
1. Asynchronous Logging
Using asynchronous logging can dramatically minimize the effect of STW pauses on your application.
Log4j 2 Async Logger
Log4j 2 comes with an asynchronous logger option that allows log messages to be queued and then written to the logging destination on separate threads. This approach offloads log writing from the application thread.
<!-- Log4j 2 Configuration Example -->
<Configuration status="WARN">
<Appenders>
<Async name="AsyncAppender">
<AppenderRef ref="File"/>
</Async>
<File name="File" fileName="logs/app.log">
<PatternLayout pattern="%d{HH:mm:ss.SSS} [%t] %-5level %logger{36} - %msg%n"/>
</File>
</Appenders>
<Loggers>
<Root level="info">
<AppenderRef ref="AsyncAppender"/>
</Root>
</Loggers>
</Configuration>
This way, even when a STW pause happens during garbage collection, log entries are queued and do not block your application threads.
2. Use Efficient Garbage Collectors
Choosing the right garbage collector for your application can minimize the frequency and duration of STW pauses.
G1 Garbage Collector
The G1 Garbage Collector is designed for applications that require low latency. It breaks up the heap into smaller regions and can perform concurrent collection, thereby reducing STW pauses.
You can activate G1 by adding the following JVM option:
-XX:+UseG1GC
3. Logging Level Management
Minimizing the amount of log data generated can also help alleviate STW pauses.
Log Level Tuning
Set the logging level appropriately based on the environment. In production, for instance, you might want to log only warnings and errors:
logger.setLevel(Level.WARNING);
This will keep the logging overhead low and reduce the frequency of logging operations that may trigger STW pauses.
4. Buffered Logging
Implementing buffered logging can also relieve the pressure during high log events. For instance, rather than writing each log entry immediately, you can collect multiple entries and write them in bulk.
import java.io.BufferedWriter;
import java.io.FileWriter;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
public class BufferedLogExample {
private List<String> logBuffer = new ArrayList<>();
private static final int BUFFER_SIZE = 50;
public void log(String message) {
logBuffer.add(message);
if (logBuffer.size() >= BUFFER_SIZE) {
flush();
}
}
public void flush() {
try (BufferedWriter writer = new BufferedWriter(new FileWriter("buffered_log.txt", true))) {
for (String message : logBuffer) {
writer.write(message);
writer.newLine();
}
logBuffer.clear();
} catch (IOException e) {
e.printStackTrace();
}
}
}
In this code, messages are buffered and written asynchronously. If a STW pause does occur, it is less catastrophic.
5. Customizable Log Destinations
Investigate alternatives to local disk I/O. For instance, utilizing remote logging services such as ELK Stack, Graylog, or custom message brokers like Kafka can offer non-blocking logging methods.
These services typically handle significant traffic and can aggregate logs from multiple sources, all while avoiding STW concerns related to local I/O.
Monitoring and Tuning Your System
It's vital to continuously monitor your logging system and JVM performance. Tools like Java VisualVM or JConsole can provide insights into GC behavior and help you understand how your system reacts to load.
Also, consider integrating application performance monitoring (APM) solutions like New Relic or Dynatrace for complete surveillance of performance metrics.
Lessons Learned
STW pauses can be a considerable challenge in logging systems, yet by implementing strategies such as asynchronous logging, using efficient garbage collectors, managing log levels, and setting up buffered logging, you can significantly mitigate their impact.
Embrace these practices as you build robust and resilient Java applications. For more on JVM optimization, feel free to check out additional resources like the Oracle Java Documentation and community resources.
By adopting these strategies, you ensure that your logging system is resilient against the impact of Stop-The-World pauses. Happy coding!
Checkout our other articles